@ethereum-waffle/mock-contract
Advanced tools
Comparing version 4.0.0-alpha.b94c36 to 4.0.0-dev.0e1e2e5
{ | ||
"name": "@ethereum-waffle/mock-contract", | ||
"description": "Mock smart contracts in a smart way.", | ||
"version": "4.0.0-alpha.b94c36", | ||
"version": "4.0.0-dev.0e1e2e5", | ||
"author": "Marek Kirejczyk <account@ethworks.io> (http://ethworks.io)", | ||
@@ -11,4 +11,8 @@ "repository": "git@github.com:EthWorks/Waffle.git", | ||
"access": "public", | ||
"tag": "alpha" | ||
"tag": "dev" | ||
}, | ||
"files": [ | ||
"dist", | ||
"src" | ||
], | ||
"keywords": [ | ||
@@ -32,2 +36,21 @@ "ethereum", | ||
"types": "dist/esm/index.d.ts", | ||
"engines": { | ||
"node": ">=10.0" | ||
}, | ||
"dependencies": { | ||
"@ethersproject/abi": "^5.6.1", | ||
"ethers": "5.6.2" | ||
}, | ||
"devDependencies": { | ||
"@ethereum-waffle/chai": "4.0.0-dev.0e1e2e5", | ||
"@ethereum-waffle/compiler": "4.0.0-dev.0e1e2e5", | ||
"solc": "^0.6.3", | ||
"@ethereum-waffle/provider": "4.0.0-dev.0e1e2e5", | ||
"typechain": "^8.0.0", | ||
"mocha": "^8.2.1", | ||
"rimraf": "^3.0.2", | ||
"typescript": "^4.6.2", | ||
"eslint": "^7.14.0", | ||
"ts-node": "^9.0.0" | ||
}, | ||
"scripts": { | ||
@@ -44,16 +67,3 @@ "test": "export NODE_ENV=test && yarn test:build && mocha", | ||
}, | ||
"engines": { | ||
"node": ">=10.0" | ||
}, | ||
"dependencies": { | ||
"@ethersproject/abi": "^5.6.0", | ||
"ethers": "5.6.1" | ||
}, | ||
"devDependencies": { | ||
"@ethereum-waffle/chai": "workspace:*", | ||
"@ethereum-waffle/compiler": "workspace:*", | ||
"solc": "^0.6.3", | ||
"@ethereum-waffle/provider": "workspace:*", | ||
"typechain": "^8.0.0" | ||
} | ||
} | ||
"readme": "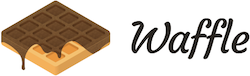\n\n\n[](https://www.npmjs.com/package/@ethereum-waffle/mock-contract)\n\n# @ethereum-waffle/mock-contract\n\nLibrary for mocking smart contract dependencies during unit testing.\n\n## Installation\nIn the current version of waffle (v3.x.x) you will install this package as a dependency of the main waffle package - `ethereum-waffle`.\n\n```\nyarn add --dev ethereum-waffle\nnpm install --save-dev ethereum-waffle\n```\n\nIf you want to use this package directly please install it via:\n```\nyarn add --dev @ethereum-waffle/mock-contract\nnpm install --save-dev @ethereum-waffle/mock-contract\n```\n\n## Usage\n\nCreate an instance of a mock contract providing the ABI/interface of the smart contract you want to mock:\n\n```js\nconst {deployMockContract} = require('@ethereum-waffle/mock-contract');\n\n...\n\nconst mockContract = await deployMockContract(wallet, contractAbi);\n```\n\nMock contract can now be passed into other contracts by using the `address` attribute.\n\nReturn values for mocked functions can be set using:\n\n```js\nawait mockContract.mock.<nameOfMethod>.returns(<value>)\nawait mockContract.mock.<nameOfMethod>.withArgs(<arguments>).returns(<value>)\n```\n\nMethods can also be set up to be reverted using:\n\n```js\nawait mockContract.mock.<nameOfMethod>.reverts()\nawait mockContract.mock.<nameOfMethod>.withArgs(<arguments>).reverts()\n```\n\nSometimes you may have an overloaded function name:\n\n```solidity\ncontract OverloadedFunctions is Ownable {\n function burn(uint256 amount) external returns (bool) {\n // ...\n }\n\n function burn(address user, uint256 amount) external onlyOwner returns (bool) {\n // ...\n }\n}\n```\n\nYou may choose which function to call by using its signature:\n\n```js\nawait mockContract.mock['burn(uint256)'].returns(true)\nawait mockContract.mock['burn(address,uint256)'].withArgs('0x1234...', 1000).reverts()\n```\n\nYou may wish to execute another contract through a mock. Given the \"AmIRichAlready\" code below, you could call constant functions using `staticcall`:\n\n```js\nconst contractFactory = new ContractFactory(AmIRichAlready.abi, AmIRichAlready.bytecode, sender);\nconst amIRich = await contractFactory.deploy()\nconst mockERC20 = await deployMockContract(sender, IERC20.abi);\n\nlet result = await mockERC20.staticcall(amIRich, 'check()')\n// you may also just use the function name\nresult = await mockERC20.staticcall(amIRich, 'check')\nexpect(result).to.equal(true) // result will be true if you have enough tokens\n```\n\nYou may also execute transactions through the mock, using `call`:\n\n```js\nconst contractFactory = new ContractFactory(AmIRichAlready.abi, AmIRichAlready.bytecode, sender);\nconst amIRich = await contractFactory.deploy()\nconst mockERC20 = await deployMockContract(sender, IERC20.abi);\n\nlet result = await mockERC20.call(amIRich, 'setRichness(uint256)', 1000)\n// you may also just use the function name\nresult = await mockERC20.call(amIRich, 'setRichness', 1000)\nexpect(await amIRich.richness()).to.equal('1000') // richness was updated\n```\n\n## Example\n\nThe example below illustrates how `mock-contract` can be used to test the very simple `AmIRichAlready` contract.\n\n```Solidity\npragma solidity ^0.6.0;\n\ninterface IERC20 {\n function balanceOf(address account) external view returns (uint256);\n}\n\ncontract AmIRichAlready {\n IERC20 private tokenContract;\n uint public richness = 1000000 * 10 ** 18;\n\n constructor (IERC20 _tokenContract) public {\n tokenContract = _tokenContract;\n }\n\n function check() public view returns (bool) {\n uint balance = tokenContract.balanceOf(msg.sender);\n return balance > richness;\n }\n\n function setRichness(uint256 _richness) public {\n richness = _richness;\n }\n}\n```\n\nWe are mostly interested in the `tokenContract.balanceOf` call. Mock contract will be used to mock exactly this call with values that are significant for the return of the `check()` method.\n\n```js\nimport {use, expect} from 'chai';\nimport {Contract, ContractFactory, utils, Wallet} from 'ethers';\nimport {MockProvider} from '@ethereum-waffle/provider';\nimport {waffleChai} from '@ethereum-waffle/chai';\nimport {deployMockContract} from '@ethereum-waffle/mock-contract';\n\nimport IERC20 from './helpers/interfaces/IERC20.json';\nimport AmIRichAlready from './helpers/interfaces/AmIRichAlready.json';\n\nuse(waffleChai);\n\ndescribe('Am I Rich Already', () => {\n let contractFactory: ContractFactory;\n let sender: Wallet;\n let receiver: Wallet;\n let mockERC20: Contract;\n let contract: Contract;\n\n beforeEach(async () => {\n [sender, receiver] = new MockProvider().getWallets();\n mockERC20 = await deployMockContract(sender, IERC20.abi);\n contractFactory = new ContractFactory(AmIRichAlready.abi, AmIRichAlready.bytecode, sender);\n contract = await contractFactory.deploy(mockERC20.address);\n });\n\n it('returns false if the wallet has less then 1000000 coins', async () => {\n await mockERC20.mock.balanceOf.returns(utils.parseEther('999999'));\n expect(await contract.check()).to.be.equal(false);\n });\n\n it('returns true if the wallet has at least 1000000 coins', async () => {\n await mockERC20.mock.balanceOf.returns(utils.parseEther('1000001'));\n expect(await contract.check()).to.equal(true);\n });\n\n it('reverts if the ERC20 reverts', async () => {\n await mockERC20.mock.balanceOf.reverts();\n await expect(contract.check()).to.be.revertedWith('Mock revert');\n });\n\n it('returns 1000001 coins for my address and 0 otherwise', async () => {\n await mockERC20.mock.balanceOf.returns('0');\n await mockERC20.mock.balanceOf.withArgs(sender.address).returns(utils.parseEther('1000001'));\n\n expect(await contract.check()).to.equal(true);\n expect(await contract.connect(receiver.address).check()).to.equal(false);\n });\n});\n```\n\n# Special thanks\n\nSpecial thanks to @spherefoundry for creating the original [Doppelganger](https://github.com/EthWorks/Doppelganger) project.\n" | ||
} |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Native code
Supply chain riskContains native code (e.g., compiled binaries or shared libraries). Including native code can obscure malicious behavior.
Found 1 instance in 1 package
230689
10
11
1234
+ Added@ethersproject/bytes@5.6.1(transitive)
+ Added@ethersproject/networks@5.6.1(transitive)
+ Added@ethersproject/providers@5.6.2(transitive)
+ Addedethers@5.6.2(transitive)
- Removed@ethersproject/bytes@5.6.0(transitive)
- Removed@ethersproject/networks@5.6.0(transitive)
- Removed@ethersproject/providers@5.6.1(transitive)
- Removedethers@5.6.1(transitive)
Updated@ethersproject/abi@^5.6.1
Updatedethers@5.6.2