@fastify/swagger-ui
Advanced tools
Comparing version 1.5.0 to 1.6.0
'use strict' | ||
const fp = require('fastify-plugin') | ||
const { readFile } = require('fs/promises') | ||
function fastifySwaggerUi (fastify, opts, next) { | ||
async function fastifySwaggerUi (fastify, opts) { | ||
fastify.decorate('swaggerCSP', require('./static/csp.json')) | ||
@@ -14,6 +15,5 @@ | ||
theme: opts.theme || {}, | ||
logo: opts.logo || { type: 'image/svg+xml', content: await readFile(require.resolve('./static/logo.svg')) }, | ||
...opts | ||
}) | ||
next() | ||
} | ||
@@ -20,0 +20,0 @@ |
@@ -6,2 +6,5 @@ 'use strict' | ||
function swaggerInitializer (opts) { | ||
const logoBase64 = Buffer.from(opts.logo.content).toString('base64') | ||
const logoData = `data:${opts.logo.type};base64,${logoBase64}` | ||
return `window.onload = function () { | ||
@@ -32,3 +35,9 @@ function resolveUrl(url) { | ||
const ui = SwaggerUIBundle(resConfig) | ||
window.ui = ui | ||
const logoData = '${logoData}' | ||
if (logoData) { | ||
const img = document.querySelector('#swagger-ui > section > div.topbar > div > div > a > img') | ||
img.src = logoData | ||
} | ||
ui.initOAuth(${serialize(opts.initOAuth)}) | ||
@@ -35,0 +44,0 @@ }` |
{ | ||
"name": "@fastify/swagger-ui", | ||
"version": "1.5.0", | ||
"version": "1.6.0", | ||
"description": "Serve Swagger-ui for Fastify", | ||
@@ -56,5 +56,5 @@ "main": "index.js", | ||
"standard": "^17.0.0", | ||
"swagger-ui-dist": "4.16.1", | ||
"swagger-ui-dist": "4.18.1", | ||
"tap": "^16.3.2", | ||
"tsd": "^0.25.0" | ||
"tsd": "^0.28.0" | ||
}, | ||
@@ -61,0 +61,0 @@ "dependencies": { |
@@ -11,2 +11,4 @@ # @fastify/swagger-ui | ||
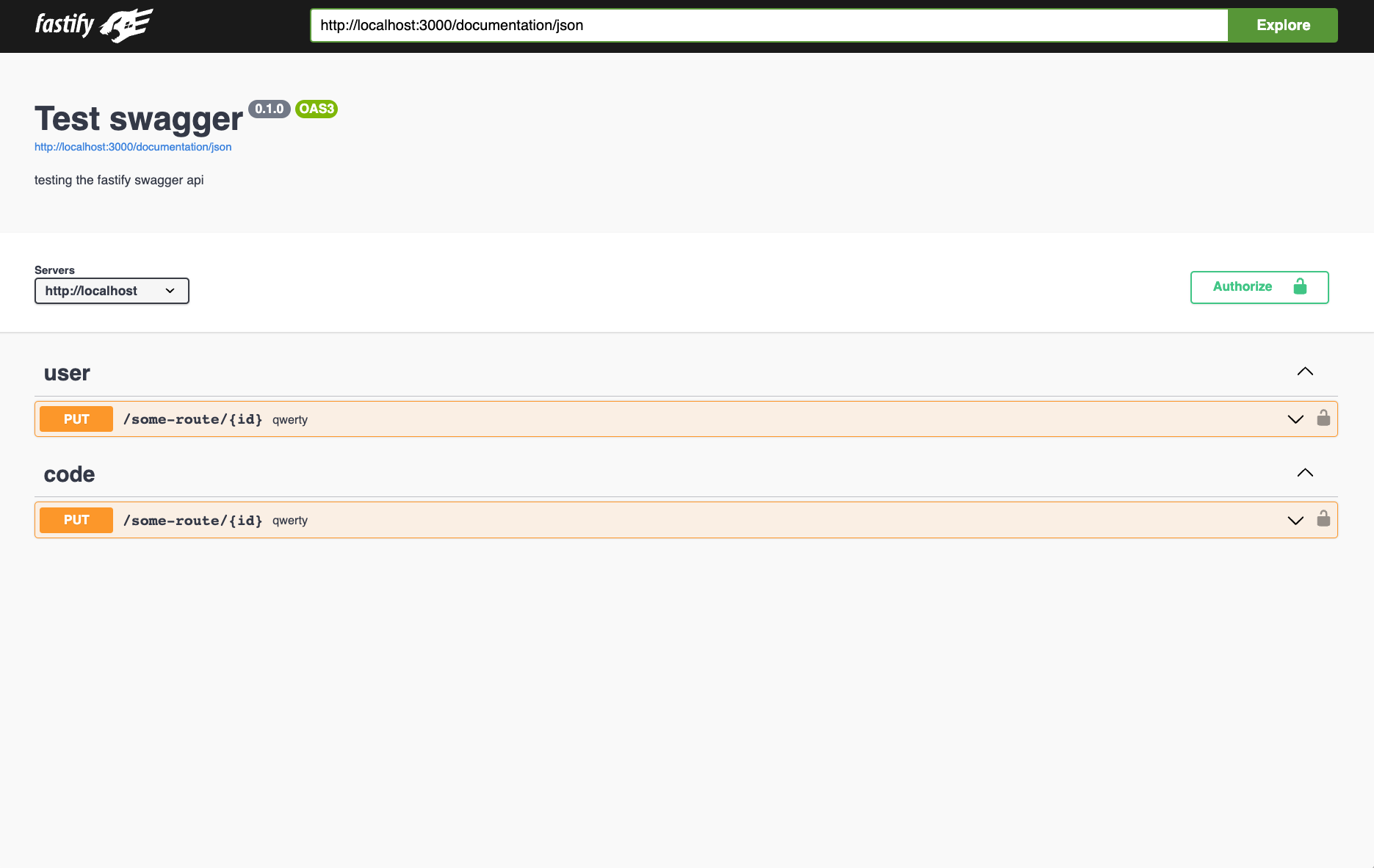 | ||
<a name="install"></a> | ||
@@ -113,3 +115,3 @@ ## Install | ||
| uiConfig | {} | Configuration options for [Swagger UI](https://github.com/swagger-api/swagger-ui/blob/master/docs/usage/configuration.md). | | ||
| uiHooks | {} | Additional hooks for the documentation's routes. You can provide the `onRequest` and `preHandler` hooks with the same [route's options](https://www.fastify.io/docs/latest/Routes/#options) interface.| | ||
| uiHooks | {} | Additional hooks for the documentation's routes. You can provide the `onRequest` and `preHandler` hooks with the same [route's options](https://www.fastify.io/docs/latest/Reference/Routes/#routes-options) interface.| | ||
| theme | {} | Add custom JavaScript and CSS to the Swagger UI web page | | ||
@@ -204,2 +206,3 @@ | logLevel | info | Allow to define route log level. | | ||
##### Example | ||
```js | ||
@@ -231,2 +234,74 @@ const fastify = require('fastify')() | ||
You can add custom JavaScript and CSS to the Swagger UI web page by using the theme option. | ||
#### logo | ||
It's possible to override the logo displayed in the top bar by specifying: | ||
``` | ||
await fastify.register(require('@fastify/swagger-ui'), { | ||
logo: { | ||
type: 'image/png', | ||
content: Buffer.from('iVBOR...', 'base64') | ||
}, | ||
theme: { | ||
favicon: [ | ||
{ | ||
filename: 'favicon.png', | ||
rel: 'icon', | ||
sizes: '16x16', | ||
type: 'image/png', | ||
content: Buffer.from('iVBOR...', 'base64') | ||
} | ||
] | ||
} | ||
}) | ||
``` | ||
#### Protect your documentation routes | ||
You can protect your documentation by configuring an authentication hook. | ||
Here is an example using the [`@fastify/basic-auth`](https://github.com/fastify/fastify-basic-auth) plugin: | ||
##### Example | ||
```js | ||
const fastify = require('fastify')() | ||
const crypto = require('crypto') | ||
fastify.register(require('@fastify/swagger')) | ||
// perform constant-time comparison to prevent timing attacks | ||
function compare (a, b) { | ||
a = Buffer.from(a) | ||
b = Buffer.from(b) | ||
if (a.length !== b.length) { | ||
// Delay return with cryptographically secure timing check. | ||
crypto.timingSafeEqual(a, a) | ||
return false | ||
} | ||
return crypto.timingSafeEqual(a, b) | ||
} | ||
await fastify.register(require('@fastify/basic-auth'), { | ||
validate (username, password, req, reply, done) { | ||
let result = true | ||
result = compare(username, validUsername) && result | ||
result = compare(password, validPassword) && result | ||
if (result) { | ||
done() | ||
} else { | ||
done(new Error('Access denied')) | ||
} | ||
}, | ||
authenticate: true | ||
}) | ||
await fastify.register(require('@fastify/swagger-ui', { | ||
uiHooks: { | ||
onRequest: fastify.basicAuth | ||
} | ||
}) | ||
``` | ||
<a name="license"></a> | ||
@@ -233,0 +308,0 @@ ## License |
@@ -13,4 +13,2 @@ const fs = require('fs') | ||
const filesToCopy = [ | ||
'favicon-16x16.png', | ||
'favicon-32x32.png', | ||
'index.html', | ||
@@ -32,2 +30,11 @@ 'index.css', | ||
const overrides = [ | ||
'favicon-16x16.png', | ||
'favicon-32x32.png', | ||
'logo.svg' | ||
] | ||
overrides.forEach(filename => { | ||
fse.copySync(`./${filename}`, resolve(`./static/${filename}`)) | ||
}) | ||
const sha = { | ||
@@ -34,0 +41,0 @@ script: [], |
@@ -79,1 +79,17 @@ 'use strict' | ||
}) | ||
test('customize logo', async (t) => { | ||
const config = { | ||
mode: 'static', | ||
specification: { | ||
path: './examples/example-static-specification.yaml' | ||
} | ||
} | ||
const fastify = Fastify() | ||
await fastify.register(fastifySwagger, config) | ||
await fastify.register(fastifySwaggerUi, { logo: { type: 'image/png', content: 'foobar' } }) | ||
const res = await fastify.inject('/documentation/static/swagger-initializer.js') | ||
t.equal(res.body.indexOf(Buffer.from('foobar').toString('base64')) > -1, true) | ||
}) |
@@ -56,2 +56,4 @@ import { FastifyPluginCallback, FastifyReply, FastifyRequest, onRequestHookHandler, preHandlerHookHandler } from 'fastify'; | ||
logo?: FastifySwaggerUILogo | ||
transformSpecification?: (swaggerObject: Readonly<Record<string, any>>, request: FastifyRequest, reply: FastifyReply) => Record<string, any> | ||
@@ -67,2 +69,7 @@ transformSpecificationClone?: boolean | ||
type FastifySwaggerUILogo = { | ||
type: string; | ||
content: string | Buffer; | ||
} | ||
type SupportedHTTPMethods = "get" | "put" | "post" | "delete" | "options" | "head" | "patch" | "trace"; | ||
@@ -69,0 +76,0 @@ |
@@ -142,1 +142,8 @@ import fastify, { FastifyReply, FastifyRequest } from 'fastify'; | ||
}) | ||
app.register(fastifySwaggerUi, { | ||
logo: { | ||
type: 'image/png', | ||
content: 'somethingsomething' | ||
} | ||
}) |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Filesystem access
Supply chain riskAccesses the file system, and could potentially read sensitive data.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
4484044
66
10495
307
5