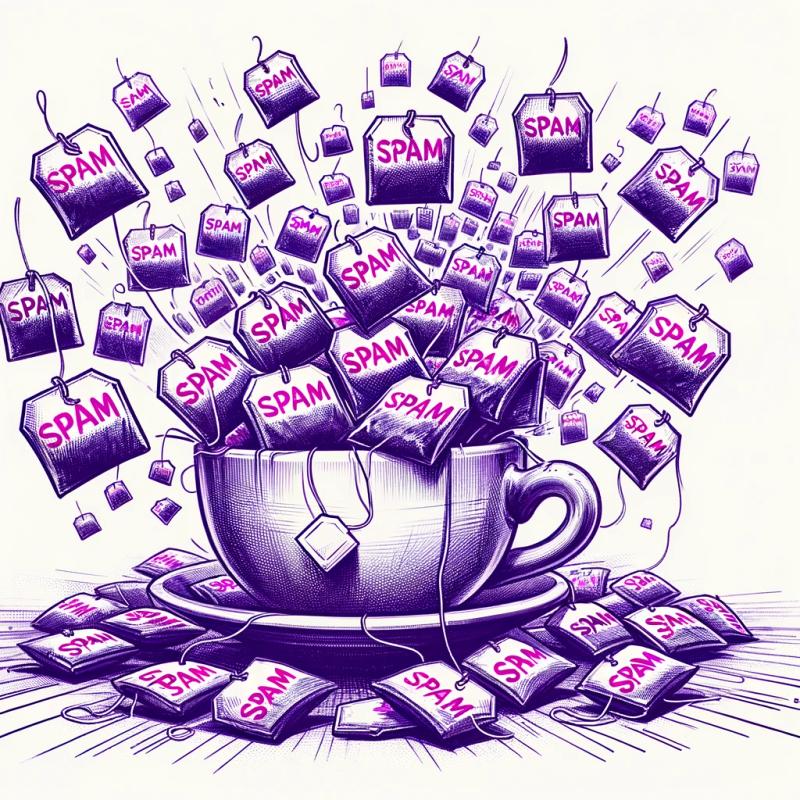
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@gcorevideo/rtckit
Advanced tools
Gcore real-time streaming platform is a cloud [SFU](https://bloggeek.me/webrtcglossary/sfu/) for WebRTC.
Readme
Gcore real-time streaming platform is a cloud SFU for WebRTC.
A very basic example. We will use Vue.js 3.
<template>
<main class="room-container room">
<div class="peers-container">
<div
class="peer-card peer-card_self"
:class="{'peer-card_joined': joined}"
v-if="room && !room.me.hidden"
>
<video ref="selfVideo" autoplay playsinline :hidden="!cameraOn"></video>
<div class="peer-card__name" v-if="room">
{{ room.me.displayName }}
(you)
</div>
</div>
<div v-for="peer of visiblePeers" :key="peer.id" ref="peerCards" class="peer-card">
<video :hidden="!peersWithVideo.has(peer.id)" autoplay playsinline />
<div class="peer-card__name">
{{ peer.displayName }}
</div>
</div>
</div>
</main>
</template>
<script setup>
import { onMounted, ref } from "vue";
import { computed, onMounted, ref, watch } from "vue";
import { RoomClient } from "@gcorevideo/rtckit";
// Your hostname or just use "meet.gcorelabs.com" for testing
const CLIENT_HOSTNAME = "first.video.international";
const ROOM_ID = "live0123";";
const cameraOn = ref(false);
const myName = ref("Alice");
const peers = ref([]);
const peerCards = ref([]);
const peersWithVideo = ref(new Set());
const readyToJoin = ref(false);
const selfVideo = ref();
const visiblePeers = computed(() => {
return peers.value.filter((peer) => !peer.hidden);
});
let room;
let mediaStream = null;
let rtcStream;
onMounted(async () => {
const client = new RoomClient({
clientHost: CLIENT_HOSTNAME,
})
room = await client.connect(ROOM_ID);
room.me.setName(myName.value);
room.on("ready", () => {
console.log("room ready to join");
startCamera();
room.join();
});
room.peers.on("newpeer", (peer) => {
console.log("new peer", peer.id);
if (!findPeer(peer.id)) {
addPeer(peer);
}
});
room.peers.on("peerleft", (id) => {
console.log("peer left", id);
removePeer(id);
});
});
// Start streaming the video from own camera
async function startCamera() {
console.log("startCamera");
cameraOn.value = true;
if (!mediaStream) {
mediaStream = await navigator.mediaDevices.getUserMedia({ video: true });
}
rtcStream = await room.me.sendVideo(mediaStream.getVideoTracks()[0], {
disableTrackOnPause: true,
});
if (selfVideo.value) {
selfVideo.value.srcObject = mediaStream;
selfVideo.value.play();
}
}
// Add peer card to the view
function addPeer(peer) {
peers.value.push(peer);
peer.on("stream", ({ label, track }) => {
console.log("peer stream available peerId:%s label:%s", peer.id, label);
if (label !== "video") {
return;
}
showPeerVideo(peer.id, track);
});
peer.on("nostream", ({ label }) => {
console.log("peer stream unavailable peerId:%s label:%s", peer.id, label);
if (label !== "video") {
return;
}
hidePeerVideo(peer.id);
});
peer.on("left", () => removePeer(peer.id));
}
// Show video on peer's card
function showPeerVideo(peerId, track) {
console.log("setPeerVideo peerId:%s", peerId);
const index = peers.value.findIndex((peer) => peer.id === peerId);
const videoElem = peerCards.value[index].querySelector("video");
const newTrack = track.clone();
const srcObject = videoElem.srcObject;
if (srcObject) {
stopPeerVideo(videoElem);
srcObject.addTrack(newTrack);
} else {
const stream = new MediaStream([newTrack]);
videoElem.srcObject = stream;
}
videoElem.play();
peersWithVideo.value.add(peerId);
}
function removePeer(peerId) {
console.log("removePeer peerId:%s", peerId);
hidePeerVideo(peerId);
const index = peers.value.findIndex((peer) => peer.id === peerId);
peers.value.splice(index, 1);
}
function hidePeerVideo(peerId) {
console.log("hidePeerVideo peerId:%s", peerId);
const index = peers.value.findIndex((peer) => peer.id === peerId);
peersWithVideo.value.delete(peerId);
const videoElem = peerCards.value[index].querySelector("video");
stopPeerVideo(videoElem);
}
</script>
Full example is in the [apps/basic].
More elaborated usage example can be found in [apps/gvcdemo]
audio
, video
, share
Client tokens are used to restrict access to a media session for users depending on possession of a token. A client token can encode media access permissions as well as higher application level roles. Client tokens are JWTs signed with a client's key. Client token attributes: TODO
Session token is what is used to authenticate a user connection to a media server. Session tokens are issued by the API backend and carry all the necessary information about the session and the user connecting to it. Some attributes of a session tokens can be controlled with a client token. This way you can, for example, provide a client token with stream producing rights to a dedicated user, while letting everybody else only consume the streams.
This is a client for Gcore real-time streaming API. It allows you, among other things, to initiate a session. You will need a client account to access the API. For testing purposes it should be sufficient to use the demo account. See the detailed API description here
import { ApiClient } from "@gcorevideo/rtckit"
const apiClient = new ApiClient({
clientKey: "XXX",
clientHost: "a0eb.gvideo.co"
})
apiClient.initSession({
peerId: "peer1"
roomId: "welcome",
}).then((sessionInfo) => {
console.log("Session info", sessionInfo)
}, (e) => {
console.error("Init session failed", e)
})
TODO API method link
This is the main interface for controlling a running session. It is created by
...
const sessionInfo = await apiClient.initSession(...)
const conn = new SocketConnection(sessionInfo.server, sessionInfo.token)
const rtcClient = new RtcClient(conn)
...
After that you can send and receive media streams within the session.
npm install
npm run build
npm run lint
Once you've made the desired changes:
$ npm version [major|minor|patch]
$ npm run build
$ npm publish --access public
FAQs
Gcore real-time streaming platform is a cloud [SFU](https://bloggeek.me/webrtcglossary/sfu/) for WebRTC.
The npm package @gcorevideo/rtckit receives a total of 7 weekly downloads. As such, @gcorevideo/rtckit popularity was classified as not popular.
We found that @gcorevideo/rtckit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.