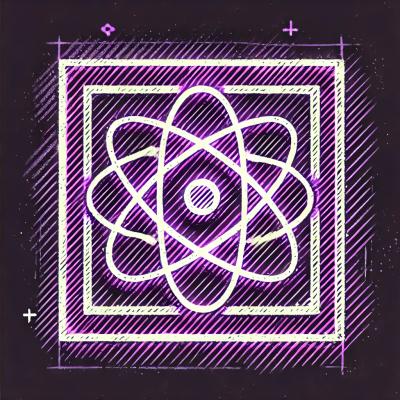
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@germinal-io/redux-as-event-bus
Advanced tools
`redux-as-event-bus` turns your redux store into a fully-typed event bus.
redux-as-event-bus
turns your redux store into a fully-typed event bus.
Doing so it allows you to manage your side effects in a clean and testable way.
$ npm i redux-as-event-bus
import { prepareEventBus } from 'redux-as-event-bus'
import { applyMiddleware, createStore, Store } from 'redux'
type AppEvent = FirstEvent | SecondEvent
type AppStore = Store<AppState, AppEvent>
const { eventBus, eventBusMiddleware } = prepareEventBus<AppEvent>()
const store: AppStore = createStore(rootReducer, applyMiddleware(eventBusMiddleware))
eventBus.onEvent('FirstEvent', (event: FirstEvent) => {
console.log(event.type) // 'FirstEvent'
})
eventBus.onAllEvents((event: AppEvent) => {
console.log(event.type) // 'FirstEvent' or 'SecondEvent'
})
interface Dependencies {
getState: () => AppState
dispatch: Dispatch<AppEvent>
userGateway: UserGateway
}
const deps: Dependencies = {
...store,
userGateway: new GraphQlUserGateway()
}
eventBus.onEvent('CreateProject', handler(deps))
function handler(deps: Dependencies) {
return async (event: CreateProject) => {
// do async logic using store values, then dispatch new event
const userId = userIdSelector(deps.getState())
const project = prepareProjectToCreate(userId)
await deps.userGateway.createProject(project)
deps.dispatch(ProjectCreated(project))
}
}
eventBus.onError((error: Error) => {
console.log(error) // Some error
})
type HandlerResult = AppEvent[]
const { eventBus, eventBusMiddleware } = prepareEventBus<
AppEvent,
HandlerResult // to ensure typing
>()
eventBus.onResult((result: HandlerResult) => {
result.map(store.dispatch) // if results are Event you may dispatch them
})
MIT
FAQs
`redux-as-event-bus` turns your redux store into a fully-typed event bus.
The npm package @germinal-io/redux-as-event-bus receives a total of 2 weekly downloads. As such, @germinal-io/redux-as-event-bus popularity was classified as not popular.
We found that @germinal-io/redux-as-event-bus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.