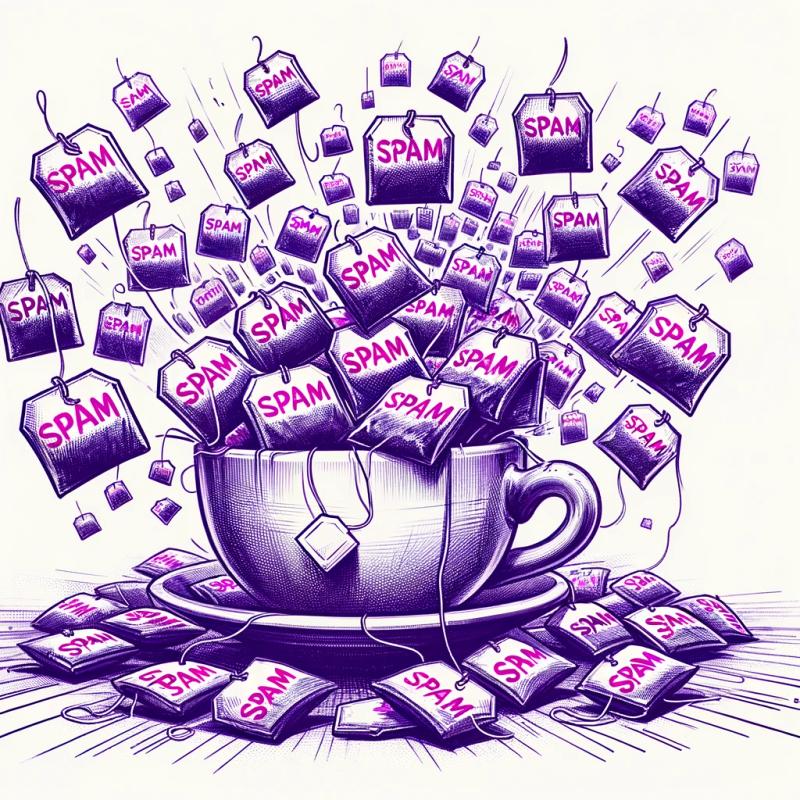
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@giosg/realtime-sdk
Advanced tools
Readme
RealtimeSDK is a JavaScript library, written in TypeScript, that combines the new giosg v5 API endpoints and the channel broadcasting system together. It allows you to observe RESTful resources and resource collections, automatically updating their state in real-time. The observing is done with Observables
from RxJS library.
RealtimeSDK manages authentication of the user or visitor that is currently using the web app. The recommended way to authorize users is to use OpenID Connect (OAuth 2) authentication. Alternatively, for visitors, there is an AJAX-based authentication method (which will be replaced with a better method in the future).
To get the RealtimeSDK to work on a web app you need to import the library, instantiate the required objects, and connect them to an OpenID Connect compatible authorization endpoint.
import { RealtimeSDK } from '@giosg/realtime-sdk';
import { OpenIDAuthorizer } from '@giosg/realtime-sdk';
// Instantiate the SDK
const sdk = new RealtimeSDK();
// Set up the authentication instance depending on the desired method
const authorizer = new OpenIdAuthorizer({
// The OAuth authorization endpoint URL
authUrl: 'https://service.giosg.com/identity/authorize',
// The `client_id` as specified in OAuth
clientId: 'chats.giosg.com',
// Prefix for the keys when storing authentication infor to session storage
storagePrefix: 'giosg-auth',
// Scopes required by the app, as specified in OAuth
scopes: [
'openid',
'profile',
'email',
/* and then any number of following: 'settings', 'reports', 'users' */
],
// Wait at least half of the expiration time of the token before renewal
minRenewalFactor: 0.5,
// Wait at most 3/4 of the expiration time of the token before renewal
maxRenewalFactor: 0.75,
});
// When your app starts, call this to start the authentication process and socket connections
sdk.connect(authorizer);
You can immediately start subscribing resources and collections after the SDK has been instantiated. HTTP requests and socket connections are only done after connect
has been called!
When running unit tests, you should probably call setAuthentication
method instead of connect
. See "Authentication in tests" section for more details.
import { RealtimeSDK } from '@giosg/realtime-sdk';
import { AjaxAuthorizer } from '@giosg/realtime-sdk';
// Instantiate the SDK
const sdk = new RealtimeSDK();
// The ID of the organization, who owns this app and the visitor data
const orgId = 'ebd8dc9d-483c-49ad-9bb8-4a80f3e2c281'
// Set up the authentication for the SDK using a *deprecated* AJAX API endpoint
const authorizer = new AjaxAuthorizer(`/api/v5/public/orgs/${orgId}/auth`, {
visitor_global_id: localStorage.getItem('visitor_global_id'),
visitor_secret_id: localStorage.getItem('visitor_secret_id'),
});
// When your app starts, call this to start the authentication process and socket connections
sdk.connect(authorizer);
For now, there is an alternative way of authenticating the user with an AJAX call to a deprecated API endpoint.
Currently, this is the only way to authenticate visitors. Do not use this method for users but use OpenID Connect (OAuth2) instead!
Also note that this method only works on service.giosg.com
domain!
/api/v5/auth
. This is DEPRECATED in favor of OpenID Connect!/api/v5/public/orgs/<organization_id>/auth
. You should provide an object with the second parameter containing the visitor_global_id
("gid") and visitor_secret_id
("sgid") that are stored locally.You usually want to do something with the "currently authenticated user". The auth
method returns an Observable that emits the latest authentication information of the user.
sdk.auth().subscribe(auth => {
console.log(`Currently authenticated with user ${auth.user_id}`);
console.log(`The user belongs to organization ${auth.organization_id}`);
});
The Observable won't emit the authentication information until the user is successfully authenticated.
The auth
parameter is an object having the following attributes, based on the information received from the authorization endpoint (e.g. OAuth2):
Attribute | Description |
---|---|
organization_id | The UUID of the organization to which the authentication is related. For authenticated users, this is the organization of the user. For visitors, this is the organiztion to which the visitor ID (CID) relates to. |
user_id | If the authentication is for an authenticated user account, then is will be the UUID of the user |
visitor_id | If the authentication is for an anonymous visitor, then this will be the ID (CID) of the visitor |
expires_at | The ISO formatted date/time when the authentication will expire. SDK will attempt to renew the access token before it expires |
access_token | The access token string that SDK will use internally for all the HTTP requests (with Authorization: Bearer <token> HTTP header) and WebSocket connection (as token URL parameter) |
See also the example how to stream resources of the authenticated user
The most important feature of the RealtimeSDK is that you can load the immediate state and then all the future updates for one specific resource. The stream completes only if the resource ever gets deleted.
// Start listening changes to a specific resource
sdk.streamResource('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b')
.subscribe(organization => {
// Emits the resource immediately when loaded, and later
// all the changes to the resource.
})
;
If connection to the server breaks, the SDK will automatically reload the resource when the connection is re-established, and any missed updates will be emitted!
The stream will result in an error if the immediate load of the resource fails. However, after that the SDK will recover from connection problems automatically, reloading and emitting the resource if necessary.
SDK will automatically handle real-time channel listening under-the-hood. The client monitors changes to the resource as long as the user of SDK is being subscribed to a resource stream. Therefore, you should unsubscribe when you do not need it any more, in order to prevent unnecessary network traffic and memory load.
// Start listening changes to the resource
let resourceStream = sdk.streamResource('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b');
let subscription = resourceStream.subscribe(organization => { /* ... */ });
// You should cancel the subscription when you are not interested in the changes any more
subscription.unsubscribe();
In many cases you may want to use the information of the authenticated user to determine which resources are observed. A common recipe is to use the observable returned by the auth
method and Observable.switchMap
.
// Observe the details of the current user
sdk.auth()
.map(auth => auth.user_id)
.distinctUntilChanged()
.switchMap(userId => sdk.streamResource('/api/v5/users/' + userId))
.subscribe(user => {
console.log("My name is " + user.full_name);
})
;
// Observe the details of the current user's organization
sdk.auth()
.map(auth => auth.organization_id)
.distinctUntilChanged()
.switchMap(orgId => sdk.streamResource('/api/v5/orgs/' + orgId))
.subscribe(organization => {
console.log("My organization's name is " + organization.name);
})
;
However, there is a shortcut helper method for this named switchUser
:
// Observe the details of the current user
sdk.switchUser(userId => sdk.streamResource('/api/v5/users/' + userId))
.subscribe(user => {
console.log("My name is " + user.full_name);
})
;
// Observe the details of the current user's organization
sdk.switchUser((userId, orgId) => sdk.streamResource('/api/v5/orgs/' + orgId))
.subscribe(organization => {
console.log("My organization's name is " + organization.name);
})
;
In advanced use, if you want to optimize the number of channel subscriptions when streaming idividual resources, you may additionally define a custom channel from which the resource updates are listened. If not defined, this defaults to the resource URL:
// Start listening changes to a specific resource, using a channel of the parent collection
sdk.streamResource('/api/v5/users/xxxxx/chats/yyyyy', { channel: '/api/v5/users/xxxxx/chats' })
.subscribe(chat => {
// ...
})
;
In some cases you may be interested in just the current state of the resource but not any future changes. You can do this by calling getResource
method. This just makes a GET request, without listening real-time changes.
Note that this still returns an Observable that needs to be subscribed.
// Start listening changes to a specific resource
sdk.getResource('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b')
.subscribe(organization => {
// Emits the resource's latest state and then completes.
// Does not emit any further changes to the resource.
}, error => {
// Failed to load the resource.
})
;
This is similar than calling Observable.first method of the Observable returned by streamResource
, but it is more efficient, as it does not use real-time socket connection at all.
In this case, the subscription does not need to be manually unsubscribed, unless you want to abort the request.
You can get an observable for creating new resource by calling postResource
method. By subscribing to it, the resource will be POSTed to the collection. The Observable emits exactly one value: the created resource object. It then completes. In other words, this does not subscribe to any future changes of the resource.
// Create a new resource by posting to a collection
let userData = {
first_name: "John",
last_name: "Smith",
/* etc... */
};
sdk.postResource('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users', userData)
.subscribe(createdUser => {
// Emits the resource when created successfully. It then completes.
}, error => {
// The creation failed!
})
;
If the creation fails, the observable results in an error.
NOTE: The HTTP request starts only after the observable is actually subscribed. This is because of the anatomy of an observable. In other words, to create a resource, even if you are not interested in the results, you need to call sdk.postResource(...).subscribe();
.
Also, by default it makes a new request for each subscriber. If this behavior is not desired, you can multicast the observable, e.g by calling Observable.share
.
Also note that the SDK will automatically "add" the newly created resource to the collection (see below) it was posted! Therefore, if you are streaming the collection to which te resource was POSTed, then SDK will automatically ensure that the resource is added to the collection (and therefore e.g. shown in the UI).
You can update an existing resource by calling putResource
for a full update or patchResource
for a partial update. Similar to postResource
, this only emits the updated resource and then completes.
// Update a new resource by putting
let updatedUserData = {
first_name: "John",
last_name: "Doe",
/* etc... */
};
sdk.putResource('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users/6437bd00-5c92-4310-b4b6-1cedb35aa3c9', updatedUserData)
.subscribe(updatedUser => {
// Emits the resource when updated successfully. It then completes.
}, error => {
// The update failed!
})
;
NOTE: As with postResource
, these observables needs to be subscribed in order to start the HTTP request, and each subscription results in a new request. See the notes above.
You can destroy an existing resource by calling deleteResource
for a resource endpoint. Unlike other methods, this only emits a single undefined value when successful. It does not emit any changes (because the resource does not exist any more).
// Destroy the resource
sdk.deleteResource('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users/6437bd00-5c92-4310-b4b6-1cedb35aa3c9')
.subscribe(() => {
// Destroyed successfully!
}, error => {
// The destroying failed!
})
;
NOTE: As with postResource
, this observable needs to be subscribed in order to start the HTTP request, and each subscription results in a new request. See the notes above.
Another core feature of the Giosg SDK is that it can be used to observe collections of resources and all the changes made to it in real-time.
The simplest way to observe a collection of resources is to stream arrays of resources.
// Start listening changes to the collection
sdk.streamCollectionArray('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users')
.subscribe(users => {
// Emits the array of ALL the resources in the collection, and then
// all the changed arrays.
})
;
However, you should only use streamCollectionArray
method if you know that your collection is relatively small by its size. This is because all the pagination chunks needs to be loaded from the server before the complete array can be built. For large collections, let's say, more than 100 items, this can be a performance issue, and you should use the streamCollection
method instead, descibed below.
For large arrays, it is strongly recommended to use lower-level streamCollection
method instead. Instead of arrays, it emits higher-order observables, that is, "observables of observables". The emitted "inner" observables emits all the resources in the collection, in order.
// Start listening changes to the collection
sdk.streamCollection('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users')
.subscribe(userStream => {
// Emits an Observable for the users in the collection, according to their current
// state in the collection. If the contents of the collection is changed, then
// another Observable is emitted.
})
;
Here's an example, how to observe max. 10 manager users, using an endpoint that lists all the users.
// Observe the first 10 manager users from the users collection
sdk.streamCollection('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users')
.switchMap(userStream => {
// Take the 10 first resources matching the giving criteria, ignoring the rest
return userStream
// Include only if the user is a manager
.filter(user => user.is_manager)
// Only max. 10 managers.
// SDK only loads the minimum number of required page chunks from the server.
.take(10)
// Emit as an array
.toArray()
;
})
.subscribe(firstManagers => {
// Emits the first 10 manager users as an array, and then
// a new array whenever the collection changes.
})
;
The collection pagination chunks are loaded lazily on-demand. That is, the pages are only actually loaded from the server if there are any subscribers interested in their contents! For example, you can use Observable.take
to take only the given number of first resources from the collection. The latter pages are not loaded from the server at all, making the operation fast even for very large collections.
Please be careful when working with higher-order observables. It is likely that the underlying collection sometimes changes before a "inner" Observable is completed, making it obsolete. For example, a resource is added to the collection before all the page chunks are loaded from the server. Luckily, Observable has handly methods to manage inner observables! For example, you can use Observable.switchMap which is useful to only use the latest emitted inner stream of resources.
As with any stream returned by the SDK, you should unsubscribe your subscription when you are not interest in the changes any more!
// Start listening changes to the collection
let collectionStream = sdk.streamCollection('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users');
// Subscribe to the stream
let subscription = collectionStream.subscribe(userStream => { /* ... */ });
// You should cancel the subscription when you are not interested in the changes any more
subscription.unsubscribe();
// Returns an observable for the current contents of the collection
sdk.getCollection('/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users')
.subscribe(user => {
// Calls this for EACH RESOURCE in the collection and then completes!
}, error => {
// Loading one of the collection page loads failed!
})
;
Sometimes you just want to get all the resources in the collection without observing the changes to the content. In this case you can use getCollection
method that just retrieves the current resources in the collection as a single observable. It emits the items until the end of the collection is reached, and then completes.
The main difference to earlier methods is that the observable emits each resource from the current state of the collection. This is roughly equivalent to: sdk.streamCollection(...).first().mergeAll()
but is more efficient, because it does not use the real-time socket connection at all.
// Observe the collection in a specific order
let collectionStream = sdk.streamCollection(
'/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users',
{ordering: '-created_at'}
)
// Convert to arrays of max. 10 most recently created users
.switchMap(userStream => userStream.take(10).toArray())
.subscribe(recentlyCreatedUsers => {
// Emits max. 10 most recently created users as an array,
// and then a new array whenever a new user is created!
})
;
The streamCollection
, streamCollectionArray
and getCollection
methods accepts additional options. You can use the ordering
option to define the order of the resources in the collection. The option must be supported by the HTTP endpoint.
As with the HTTP endpoint, you may use the -
prefix to reverse the order. You can also separate secondary sorting criterias with commas (,
).
All the collection streaming and loading methods described above (streamCollection
, streamCollectionArray
and getCollection
) support filtering options:
// Start listening changes to the collection
sdk.streamCollectionArray(
'/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users',
{ is_manager: true }
)
.subscribe(managerUsers => {
// Emits the array of filtered resources in the collection
})
;
This will load the resources from the following URL:
/api/v5/orgs/c0c329ce-bd7f-41e3-b79b-af2f7f9f334b/users?is_manager=true
For the best performance, it is recommended that the backend supports the filtering GET parameters. However, the filtering will be also done client-side, in case the backend does not support the filtering parameter.
You may provide multiple filtering criterias, in which case all of them must match.
The following filtering types are supported:
Type | Description |
---|---|
string | Added to the URL request as URI-encoded GET parameter |
integer | Added to the URL request as stringified GET parameter |
boolean | Added to the URL reqeust as ether true or false GET parameter |
Note that currently, for simplicity, only exact value filtering is supported with URL GET parameters. Negations or comparisons may be added in the future. You may perform any custom filtering on the client-side. Also, no "or" condition in currently supported.
In some cases you may only be interested in resources that are added to a collection. For example, you may want to play a sound or show a small notification whenever a new chat message is received, but you would like to ignore any initial chat messages for this purpose.
In these cases, you can use streamCollectionAdditions
helper method:
// Start listening additions to the collections
sdk.streamCollectionAdditions(
'/api/v5/users/xxxxx/chats/yyyyy/messages',
{ ordering: '-created_at' }
)
.subscribe(newMessage => {
// Called for each chat message that is added to the collection
// while this collection is being subscribed!
})
;
Please note the following characteristics of the returned observable:
.distinctKey("id")
, but consider memory usage.streamCollection
does, and therefore you can (and should) provide the same options, including filtering criteria. The ordering
option will affect the order in which any "simulatenously" added resources are emitted. If the ordering does not matter and you are streaming the same collection elsewhere with any specific ordering, using the same ordering with this method will preserve a bunch of unnecessary HTTP requests!In unit tests you probably do not want to call connect
method of RealtimeSDK instance, as this tries to redirect the browser or make an AJAX request to the authentication endpoint and create an actual Websocket connection. Instead, you may initialize the SDK with a mock authentication object with setAuthentication
method like this:
import { RealtimeSDK } from '@giosg/realtime-sdk';
const sdk = new RealtimeSDK();
// Set mock authentication for the SDK
sdk.setAuthentication({
access_token: "<MY_ACCESS_TOKEN>",
user_id: "b8aca5a7-4c73-4de1-bcf4-d3614029db1f",
organization_id: "4ee0c512-7fb5-4b00-bfec-a6c6cd7f94e3",
});
It also accepts an Observable that emits authentication objects (to mimic changes in the authentication state):
import { Subject } from 'rxjs/Subject';
// ...
// Set up authentication from an Observable of authentication objects
let mockAuth = new Subject();
sdk.setAuthentication(mockAuth);
mockAuth.next({ access_token: "<FIRST_ACCESS_TOKEN>", /* ... */ });
mockAuth.next({ access_token: "<SECOND_ACCESS_TOKEN>", /* ... */ });
The has a bunch of methods that allows to to mock the resources and collections. This means than whenever a certain resource or collection is streamed, the "fake" objects are emitted instead.
This is useful for testing components that use SDK, as you do not have to fake HTTP requests or socket traffic at all! You can also use these methods runtime for debugging.
Mock to always return a certain value:
// Mock the resource
sdk.mockResource("/api/v5/users/xxxx", {
id: "xxxx",
first_name: "John",
last_name: "Smith",
// ...
});
It also allows you to mock with an Observable. By using a Subject, you can fake changes to a resource:
// Fake the changes to the resource
let fakeStream = new Subject<Resource>();
sdk.mockResource("/api/v5/users/xxxx", fakeStream);
// ...
fakeStream.next({
id: "xxxx",
is_online: false,
// ...
});
// ...
fakeStream.next({
id: "xxxx",
is_online: true,
// ...
});
You can reset the mocking for the resource, which re-enables retrieving the resource via HTTP and listening changes via socket:
// Reset the mocking for the resource
sdk.mockResource("/api/v5/users/xxxx", null);
Mock to always return a certain array of resources:
sdk.mockCollectionArray("/api/v5/users", [
{ /* User 1 */ },
{ /* User 2 */ },
{ /* User 3 */ },
]);
You can fake changes to the collection by mocking with an Observable of arrays, for example, by using a Subject. It also allows you to mock with an Observable. By using a Subject, you can fake changes to a resource:
let fakeStream = new Subject<Resource[]>();
sdk.mockCollection("/api/v5/users", fakeStream);
// ...
fakeStream.next([
{ /* User 1 */ },
{ /* User 2 */ },
]);
// ...
fakeStream.next([
{ /* User 1 */ },
{ /* User 2 */ },
{ /* User 3 */ },
]);
You can also mock the responses to POST, PUT, PATCH and DELETE actions done to resources via RealtimeSDK. For POST, PUT and PATCH, you can set a static response object.
// Mock POST response
sdk.mockResourcePost("/api/v5/users", {
id: "xxxx",
first_name: "John",
last_name: "Smith",
// ...
});
// Mock PUT response
sdk.mockResourcePut("/api/v5/users/xxxx", {
id: "xxxx",
first_name: "John",
last_name: "Smith",
// ...
});
// Mock PATCH response
sdk.mockResourcePatch("/api/v5/users/xxxx", {
id: "xxxx",
first_name: "John",
last_name: "Smith",
// ...
});
Alternatively, for faking asyncronous responses, you can provide an Observable, such as a Subject. This applies any of the action mocking methods:
// Mock asyncronous POST response
let fakeResponse = new Subject<Resource>();
sdk.mockResourcePost("/api/v5/users/xxxx", fakeResponse);
// ...
fakeResponse.next({
id: "xxxx",
first_name: "John",
last_name: "Smith",
// ...
});
fakeResponse.complete();
In tests, you can also provide a function as the mock. The function will be called with the payload and the access token. Its return value, which can be an object or an Observable, will be handled similar to previous examples.
// Test that the code POSTs a new resource
let postHandler = jasmine.createSpy('postHandler').and.returnValue({
id: "xxxx",
first_name: "John",
last_name: "Smith",
// ...
});
sdk.mockResourcePost("/api/v5/users", postHandler);
// ...
expect(postHandler).toHaveBeenCalledWith({ /* POSTed object */ }, "<ACCESS_TOKEN>");
By default, SDK will only fake resources and collections that are specifically mocked with the mocking methods. Any other resources and collections will be loaded remotely. In tests, however, this is not usually what you want.
You can prevent the SDK to make any actual HTTP requests or socket traffic by switching it to the test mode. For example, in Jasmine tests you can do this:
// Disallow actual network traffic during the test
beforeEach(() => sdk.startTestMode());
afterEach(() => sdk.stopTestMode());
This is recommended to be done in each test that uses an SDK.
RealtimeSDK supports optimistic resource updates and deletions.
By default, any updates and removals are applied immediately to any related resource or collection stream! The actual operation is then run in background. If the operation fails, then the optimistic changes are rolled back.
NOTE: The support for optimistic additions could be added later.
When using putResource
, patchResource
or deleteResource
, you may optionally define any optimistic side-effects yourself.
sdk.putResource(
`/api/v5/users/${userId}`,
{
first_name: "John",
last_name: "Smith",
// ...
},
{
// Which other resources should be updated?
optimisticUpdates: [{
resourceUrl: `/api/v5/orgs/${organizationId}/users/${userId}`,
resource: {
full_name: "John Smith",
}
}],
// Which resources should be removed from their collections?
optimisticRemovals: [`/api/v5/orgs/${organizationId}/cool_guys/${userId}`],
}
).subscribe();
To publish newer version of this package to npm ypu need to:
package.json
npm install
to install all dependencies and update package-lock.json
npm login --scope=@giosg
to our organizationnpm publish -–access=public
FAQs
SDK for streaming Giosg resources in real-time
The npm package @giosg/realtime-sdk receives a total of 91 weekly downloads. As such, @giosg/realtime-sdk popularity was classified as not popular.
We found that @giosg/realtime-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 18 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.