@google/clasp
Advanced tools
Comparing version 1.1.1 to 1.1.2
848
index.js
#!/usr/bin/env node | ||
"use strict"; | ||
var __awaiter = (this && this.__awaiter) || function (thisArg, _arguments, P, generator) { | ||
return new (P || (P = Promise))(function (resolve, reject) { | ||
function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } } | ||
function rejected(value) { try { step(generator["throw"](value)); } catch (e) { reject(e); } } | ||
function step(result) { result.done ? resolve(result.value) : new P(function (resolve) { resolve(result.value); }).then(fulfilled, rejected); } | ||
step((generator = generator.apply(thisArg, _arguments || [])).next()); | ||
}); | ||
}; | ||
var __generator = (this && this.__generator) || function (thisArg, body) { | ||
var _ = { label: 0, sent: function() { if (t[0] & 1) throw t[1]; return t[1]; }, trys: [], ops: [] }, f, y, t, g; | ||
return g = { next: verb(0), "throw": verb(1), "return": verb(2) }, typeof Symbol === "function" && (g[Symbol.iterator] = function() { return this; }), g; | ||
function verb(n) { return function (v) { return step([n, v]); }; } | ||
function step(op) { | ||
if (f) throw new TypeError("Generator is already executing."); | ||
while (_) try { | ||
if (f = 1, y && (t = y[op[0] & 2 ? "return" : op[0] ? "throw" : "next"]) && !(t = t.call(y, op[1])).done) return t; | ||
if (y = 0, t) op = [0, t.value]; | ||
switch (op[0]) { | ||
case 0: case 1: t = op; break; | ||
case 4: _.label++; return { value: op[1], done: false }; | ||
case 5: _.label++; y = op[1]; op = [0]; continue; | ||
case 7: op = _.ops.pop(); _.trys.pop(); continue; | ||
default: | ||
if (!(t = _.trys, t = t.length > 0 && t[t.length - 1]) && (op[0] === 6 || op[0] === 2)) { _ = 0; continue; } | ||
if (op[0] === 3 && (!t || (op[1] > t[0] && op[1] < t[3]))) { _.label = op[1]; break; } | ||
if (op[0] === 6 && _.label < t[1]) { _.label = t[1]; t = op; break; } | ||
if (t && _.label < t[2]) { _.label = t[2]; _.ops.push(op); break; } | ||
if (t[2]) _.ops.pop(); | ||
_.trys.pop(); continue; | ||
} | ||
op = body.call(thisArg, _); | ||
} catch (e) { op = [6, e]; y = 0; } finally { f = t = 0; } | ||
if (op[0] & 5) throw op[1]; return { value: op[0] ? op[1] : void 0, done: true }; | ||
} | ||
}; | ||
var _this = this; | ||
exports.__esModule = true; | ||
@@ -12,2 +48,3 @@ var anymatch = require("anymatch"); | ||
var http = require("http"); | ||
var isOnline = require('is-online'); | ||
var mkdirp = require("mkdirp"); | ||
@@ -102,3 +139,3 @@ var OAuth2 = google.auth.OAuth2; | ||
CLONING: 'Cloning files...', | ||
CREATE_PROJECT_FINISH: function (scriptId) { return "Created new script: " + getScriptURL(scriptId) + "."; }, | ||
CREATE_PROJECT_FINISH: function (scriptId) { return "Created new script: " + getScriptURL(scriptId); }, | ||
CREATE_PROJECT_START: function (title) { return "Creating new script: " + title + "..."; }, | ||
@@ -140,2 +177,3 @@ DEPLOYMENT_CREATE: 'Creating deployment...', | ||
LOGGED_OUT: "Please login. (" + PROJECT_NAME + " login)", | ||
OFFLINE: 'Error: Looks like you are offline.', | ||
ONE_DEPLOYMENT_CREATE: 'Currently just one deployment can be created at a time.', | ||
@@ -250,3 +288,5 @@ READ_ONLY_DELETE: 'Unable to delete read-only deployment.', | ||
'https://www.googleapis.com/auth/script.projects', | ||
] | ||
], | ||
code_challenge_method: 'S256', | ||
code_challenge: codes.codeChallenge | ||
}; | ||
@@ -292,3 +332,3 @@ var authCode = useLocalhost ? | ||
server.listen(0, function () { | ||
oauth2Client.redirectUri = 'http://localhost:' + server.address().port; | ||
oauth2Client.redirectUri = "http://localhost:" + server.address().port; | ||
var authUrl = oauth2Client.generateAuthUrl(opts); | ||
@@ -347,2 +387,20 @@ console.log(LOG.AUTHORIZE(authUrl)); | ||
/** | ||
* Checks if the network is available. Gracefully exits if not. | ||
*/ | ||
function checkIfOnline() { | ||
return __awaiter(this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, isOnline()]; | ||
case 1: | ||
if (!(_a.sent())) { | ||
logError(null, ERROR.OFFLINE); | ||
process.exit(1); | ||
} | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
} | ||
/** | ||
* Saves the script ID in the project dotfile. | ||
@@ -379,5 +437,13 @@ * @param {string} scriptId The script ID | ||
console.warn(ERROR.LOGGED_IN); | ||
})["catch"](function (err) { | ||
authorize(cmd.localhost); | ||
}); | ||
})["catch"](function (err) { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
authorize(cmd.localhost); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); }); | ||
}); | ||
@@ -412,21 +478,29 @@ /** | ||
else { | ||
getAPICredentials(function () { | ||
spinner.setSpinnerTitle(LOG.CREATE_PROJECT_START(title)); | ||
spinner.start(); | ||
script.projects.create({ title: title, parentId: parentId }, {}, function (error, _a) { | ||
var data = _a.data; | ||
var scriptId = data.scriptId; | ||
spinner.stop(true); | ||
if (error) { | ||
logError(error, ERROR.CREATE); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
spinner.setSpinnerTitle(LOG.CREATE_PROJECT_START(title)); | ||
spinner.start(); | ||
script.projects.create({ title: title, parentId: parentId }, {}, function (error, _a) { | ||
var data = _a.data; | ||
var scriptId = data.scriptId; | ||
spinner.stop(true); | ||
if (error) { | ||
logError(error, ERROR.CREATE); | ||
} | ||
else { | ||
console.log(LOG.CREATE_PROJECT_FINISH(scriptId)); | ||
saveProjectId(scriptId); | ||
if (!manifestExists()) { | ||
fetchProject(scriptId); // fetches appsscript.json, o.w. `push` breaks | ||
} | ||
} | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
else { | ||
console.log(LOG.CREATE_PROJECT_FINISH(scriptId)); | ||
saveProjectId(scriptId); | ||
if (!manifestExists()) { | ||
fetchProject(scriptId); // fetches appsscript.json, o.w. `push` breaks | ||
} | ||
} | ||
}); | ||
}); | ||
}); }); | ||
} | ||
@@ -439,43 +513,54 @@ }); | ||
* @param {string?} rootDir The directory to save the project files to. Defaults to `pwd` | ||
* @param {number?} versionNumber The version of files to fetch. | ||
*/ | ||
function fetchProject(scriptId, rootDir) { | ||
function fetchProject(scriptId, rootDir, versionNumber) { | ||
var _this = this; | ||
if (rootDir === void 0) { rootDir = ''; } | ||
spinner.start(); | ||
getAPICredentials(function () { | ||
script.projects.getContent({ | ||
scriptId: scriptId | ||
}, {}, function (error, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (error) { | ||
if (error.statusCode === 404) | ||
return logError(null, ERROR.SCRIPT_ID_INCORRECT(scriptId)); | ||
return logError(error, ERROR.SCRIPT_ID); | ||
} | ||
else { | ||
if (!data.files) { | ||
return logError(null, ERROR.SCRIPT_ID_INCORRECT(scriptId)); | ||
} | ||
// Create the files in the cwd | ||
console.log(LOG.CLONE_SUCCESS(data.files.length)); | ||
var sortedFiles = data.files.sort(function (file) { return file.name; }); | ||
sortedFiles.map(function (file) { | ||
var filePath = file.name + "." + getFileType(file.type); | ||
var truePath = (rootDir || '.') + "/" + filePath; | ||
mkdirp(path.dirname(truePath), function (err) { | ||
if (err) | ||
return logError(err, ERROR.FS_DIR_WRITE); | ||
if (!file.source) | ||
return; // disallow empty files | ||
fs.writeFile(truePath, file.source, function (err) { | ||
if (err) | ||
return logError(err, ERROR.FS_FILE_WRITE); | ||
}); | ||
// Log only filename if pulling to root (Code.gs vs ./Code.gs) | ||
console.log("\u2514\u2500 " + (rootDir ? truePath : filePath)); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
script.projects.getContent({ | ||
scriptId: scriptId, | ||
versionNumber: versionNumber | ||
}, {}, function (error, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (error) { | ||
if (error.statusCode === 404) | ||
return logError(null, ERROR.SCRIPT_ID_INCORRECT(scriptId)); | ||
return logError(error, ERROR.SCRIPT_ID); | ||
} | ||
else { | ||
if (!data.files) { | ||
return logError(null, ERROR.SCRIPT_ID_INCORRECT(scriptId)); | ||
} | ||
// Create the files in the cwd | ||
console.log(LOG.CLONE_SUCCESS(data.files.length)); | ||
var sortedFiles = data.files.sort(function (file) { return file.name; }); | ||
sortedFiles.map(function (file) { | ||
var filePath = file.name + "." + getFileType(file.type); | ||
var truePath = (rootDir || '.') + "/" + filePath; | ||
mkdirp(path.dirname(truePath), function (err) { | ||
if (err) | ||
return logError(err, ERROR.FS_DIR_WRITE); | ||
if (!file.source) | ||
return; // disallow empty files | ||
fs.writeFile(truePath, file.source, function (err) { | ||
if (err) | ||
return logError(err, ERROR.FS_FILE_WRITE); | ||
}); | ||
// Log only filename if pulling to root (Code.gs vs ./Code.gs) | ||
console.log("\u2514\u2500 " + (rootDir ? truePath : filePath)); | ||
}); | ||
}); | ||
} | ||
}); | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); }); | ||
} | ||
@@ -486,9 +571,17 @@ /** | ||
commander | ||
.command('clone <scriptId>') | ||
.command('clone <scriptId> [versionNumber]') | ||
.description('Clone a project') | ||
.action(function (scriptId) { | ||
spinner.setSpinnerTitle(LOG.CLONING); | ||
saveProjectId(scriptId); | ||
fetchProject(scriptId); | ||
}); | ||
.action(function (scriptId) { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
spinner.setSpinnerTitle(LOG.CLONING); | ||
saveProjectId(scriptId); | ||
fetchProject(scriptId); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); }); | ||
/** | ||
@@ -500,11 +593,19 @@ * Fetches a project from either a provided or saved script id. | ||
.description('Fetch a remote project') | ||
.action(function () { | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId, rootDir = _a.rootDir; | ||
if (scriptId) { | ||
spinner.setSpinnerTitle(LOG.PULLING); | ||
fetchProject(scriptId, rootDir); | ||
.action(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId, rootDir = _a.rootDir; | ||
if (scriptId) { | ||
spinner.setSpinnerTitle(LOG.PULLING); | ||
fetchProject(scriptId, rootDir); | ||
} | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); }); | ||
/** | ||
@@ -520,97 +621,111 @@ * Force writes all local files to the script management server. | ||
.description('Update the remote project') | ||
.action(function () { | ||
spinner.setSpinnerTitle(LOG.PUSHING); | ||
spinner.start(); | ||
getAPICredentials(function () { | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId, rootDir = _a.rootDir; | ||
if (!scriptId) | ||
return; | ||
// Read all filenames as a flattened tree | ||
recursive(rootDir || './', function (err, filePaths) { | ||
if (err) | ||
return logError(err); | ||
// Filter files that aren't allowed. | ||
filePaths = filePaths.filter(function (name) { return !name.startsWith('.'); }); | ||
DOTFILE.IGNORE().then(function (ignorePatterns) { | ||
filePaths = filePaths.sort(); // Sort files alphanumerically | ||
var abortPush = false; | ||
// Match the files with ignored glob pattern | ||
readMultipleFiles(filePaths, 'utf8', function (err, contents) { | ||
if (err) | ||
return console.error(err); | ||
var nonIgnoredFilePaths = []; | ||
// Check if there are any .gs files | ||
// We will prompt the user to rename files | ||
var canRenameToJS = false; | ||
filePaths.map(function (name, i) { | ||
if (path.extname(name) === '.gs') { | ||
canRenameToJS = true; | ||
} | ||
}); | ||
// Check if there are files that will conflict if renamed .gs to .js | ||
filePaths.map(function (name) { | ||
var fileNameWithoutExt = name.slice(0, -path.extname(name).length); | ||
if (filePaths.indexOf(fileNameWithoutExt + '.js') !== -1 && | ||
filePaths.indexOf(fileNameWithoutExt + '.gs') !== -1) { | ||
// Can't rename, conflicting files | ||
abortPush = true; | ||
if (path.extname(name) === '.gs') { | ||
logError(null, ERROR.CONFLICTING_FILE_EXTENSION(fileNameWithoutExt)); | ||
} | ||
} | ||
else if (path.extname(name) === '.gs') { | ||
// rename file to js | ||
console.log(LOG.RENAME_FILE(fileNameWithoutExt + '.gs', fileNameWithoutExt + '.js')); | ||
fs.renameSync(fileNameWithoutExt + '.gs', fileNameWithoutExt + '.js'); | ||
} | ||
}); | ||
if (abortPush) | ||
return spinner.stop(true); | ||
var files = filePaths.map(function (name, i) { | ||
var nameWithoutExt = name.slice(0, -path.extname(name).length); | ||
// Formats rootDir/appsscript.json to appsscript.json. | ||
// Preserves subdirectory names in rootDir | ||
// (rootDir/foo/Code.js becomes foo/Code.js) | ||
var formattedName = nameWithoutExt; | ||
if (rootDir) { | ||
formattedName = nameWithoutExt.slice(rootDir.length + 1, nameWithoutExt.length); | ||
} | ||
if (getAPIFileType(name) && !anymatch(ignorePatterns, name)) { | ||
nonIgnoredFilePaths.push(name); | ||
var file = { | ||
name: formattedName, | ||
type: getAPIFileType(name), | ||
source: contents[i] //the file contents | ||
}; | ||
return file; | ||
} | ||
else { | ||
return; // Skip ignored files | ||
} | ||
}).filter(Boolean); // remove null values | ||
script.projects.updateContent({ | ||
scriptId: scriptId, | ||
resource: { files: files } | ||
}, {}, function (error, res) { | ||
spinner.stop(true); | ||
if (error) { | ||
console.error(LOG.PUSH_FAILURE); | ||
error.errors.map(function (err) { | ||
console.error(err.message); | ||
.action(function () { return __awaiter(_this, void 0, void 0, function () { | ||
var _this = this; | ||
return __generator(this, function (_a) { | ||
spinner.setSpinnerTitle(LOG.PUSHING); | ||
spinner.start(); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId, rootDir = _a.rootDir; | ||
if (!scriptId) | ||
return; | ||
// Read all filenames as a flattened tree | ||
recursive(rootDir || './', function (err, filePaths) { | ||
if (err) | ||
return logError(err); | ||
// Filter files that aren't allowed. | ||
filePaths = filePaths.filter(function (name) { return !name.startsWith('.'); }); | ||
DOTFILE.IGNORE().then(function (ignorePatterns) { | ||
filePaths = filePaths.sort(); // Sort files alphanumerically | ||
var abortPush = false; | ||
// Match the files with ignored glob pattern | ||
readMultipleFiles(filePaths, 'utf8', function (err, contents) { | ||
if (err) | ||
return console.error(err); | ||
var nonIgnoredFilePaths = []; | ||
// Check if there are any .gs files | ||
// We will prompt the user to rename files | ||
var canRenameToJS = false; | ||
filePaths.map(function (name, i) { | ||
if (path.extname(name) === '.gs') { | ||
canRenameToJS = true; | ||
} | ||
}); | ||
// Check if there are files that will conflict if renamed .gs to .js | ||
filePaths.map(function (name) { | ||
var fileNameWithoutExt = name.slice(0, -path.extname(name).length); | ||
if (filePaths.indexOf(fileNameWithoutExt + '.js') !== -1 && | ||
filePaths.indexOf(fileNameWithoutExt + '.gs') !== -1) { | ||
// Can't rename, conflicting files | ||
abortPush = true; | ||
if (path.extname(name) === '.gs') { | ||
logError(null, ERROR.CONFLICTING_FILE_EXTENSION(fileNameWithoutExt)); | ||
} | ||
} | ||
else if (path.extname(name) === '.gs') { | ||
// rename file to js | ||
console.log(LOG.RENAME_FILE(fileNameWithoutExt + '.gs', fileNameWithoutExt + '.js')); | ||
fs.renameSync(fileNameWithoutExt + '.gs', fileNameWithoutExt + '.js'); | ||
} | ||
}); | ||
if (abortPush) | ||
return spinner.stop(true); | ||
var files = filePaths.map(function (name, i) { | ||
var nameWithoutExt = name.slice(0, -path.extname(name).length); | ||
// Replace OS specific path separator to common '/' char | ||
nameWithoutExt = nameWithoutExt.replace('\\', '/'); | ||
// Formats rootDir/appsscript.json to appsscript.json. | ||
// Preserves subdirectory names in rootDir | ||
// (rootDir/foo/Code.js becomes foo/Code.js) | ||
var formattedName = nameWithoutExt; | ||
if (rootDir) { | ||
formattedName = nameWithoutExt.slice(rootDir.length + 1, nameWithoutExt.length); | ||
} | ||
if (getAPIFileType(name) && !anymatch(ignorePatterns, name)) { | ||
nonIgnoredFilePaths.push(name); | ||
var file = { | ||
name: formattedName, | ||
type: getAPIFileType(name), | ||
source: contents[i] //the file contents | ||
}; | ||
return file; | ||
} | ||
else { | ||
return; // Skip ignored files | ||
} | ||
}).filter(Boolean); // remove null values | ||
script.projects.updateContent({ | ||
scriptId: scriptId, | ||
resource: { files: files } | ||
}, {}, function (error, res) { | ||
spinner.stop(true); | ||
if (error) { | ||
console.error(LOG.PUSH_FAILURE); | ||
error.errors.map(function (err) { | ||
console.error(err.message); | ||
}); | ||
} | ||
else { | ||
nonIgnoredFilePaths.map(function (filePath) { | ||
console.log("\u2514\u2500 " + filePath); | ||
}); | ||
console.log(LOG.PUSH_SUCCESS(nonIgnoredFilePaths.length)); | ||
} | ||
}); | ||
}); | ||
}); | ||
} | ||
else { | ||
nonIgnoredFilePaths.map(function (filePath) { | ||
console.log("\u2514\u2500 " + filePath); | ||
}); | ||
console.log(LOG.PUSH_SUCCESS(nonIgnoredFilePaths.length)); | ||
} | ||
}); | ||
}); | ||
}); | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); }); | ||
return [2 /*return*/]; | ||
}); | ||
}); | ||
}); }); | ||
/** | ||
@@ -625,11 +740,20 @@ * Opens the script editor in the user's browser. | ||
var scriptId = _a.scriptId; | ||
if (scriptId) { | ||
console.log(LOG.OPEN_PROJECT(scriptId)); | ||
if (scriptId.length < 30) { | ||
logError(null, ERROR.SCRIPT_ID_INCORRECT(scriptId)); | ||
} | ||
else { | ||
open(getScriptURL(scriptId)); | ||
} | ||
} | ||
return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_b) { | ||
switch (_b.label) { | ||
case 0: | ||
if (!scriptId) return [3 /*break*/, 3]; | ||
console.log(LOG.OPEN_PROJECT(scriptId)); | ||
if (!(scriptId.length < 30)) return [3 /*break*/, 1]; | ||
logError(null, ERROR.SCRIPT_ID_INCORRECT(scriptId)); | ||
return [3 /*break*/, 3]; | ||
case 1: return [4 /*yield*/, checkIfOnline()]; | ||
case 2: | ||
_b.sent(); | ||
open(getScriptURL(scriptId)); | ||
_b.label = 3; | ||
case 3: return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); | ||
@@ -644,34 +768,42 @@ }); | ||
.action(function () { | ||
getAPICredentials(function () { | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
if (!scriptId) | ||
return; | ||
spinner.setSpinnerTitle(LOG.DEPLOYMENT_LIST(scriptId)); | ||
spinner.start(); | ||
script.projects.deployments.list({ | ||
scriptId: scriptId | ||
}, {}, function (error, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (error) { | ||
logError(error); | ||
} | ||
else { | ||
var deployments = data.deployments; | ||
var numDeployments = deployments.length; | ||
var deploymentWord = pluralize('Deployment', numDeployments); | ||
console.log(numDeployments + " " + deploymentWord + "."); | ||
deployments.map(function (_a) { | ||
var deploymentId = _a.deploymentId, deploymentConfig = _a.deploymentConfig; | ||
var versionString = !!deploymentConfig.versionNumber ? | ||
"@" + deploymentConfig.versionNumber : '@HEAD'; | ||
var description = deploymentConfig.description ? | ||
'- ' + deploymentConfig.description : ''; | ||
console.log("- " + deploymentId + " " + versionString + " " + description); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
if (!scriptId) | ||
return; | ||
spinner.setSpinnerTitle(LOG.DEPLOYMENT_LIST(scriptId)); | ||
spinner.start(); | ||
script.projects.deployments.list({ | ||
scriptId: scriptId | ||
}, {}, function (error, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (error) { | ||
logError(error); | ||
} | ||
else { | ||
var deployments = data.deployments; | ||
var numDeployments = deployments.length; | ||
var deploymentWord = pluralize('Deployment', numDeployments); | ||
console.log(numDeployments + " " + deploymentWord + "."); | ||
deployments.map(function (_a) { | ||
var deploymentId = _a.deploymentId, deploymentConfig = _a.deploymentConfig; | ||
var versionString = !!deploymentConfig.versionNumber ? | ||
"@" + deploymentConfig.versionNumber : '@HEAD'; | ||
var description = deploymentConfig.description ? | ||
'- ' + deploymentConfig.description : ''; | ||
console.log("- " + deploymentId + " " + versionString + " " + description); | ||
}); | ||
} | ||
}); | ||
}); | ||
} | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); }); | ||
}); | ||
@@ -687,54 +819,62 @@ /** | ||
description = description || ''; | ||
getAPICredentials(function () { | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
if (!scriptId) | ||
return; | ||
spinner.setSpinnerTitle(LOG.DEPLOYMENT_START(scriptId)); | ||
spinner.start(); | ||
function createDeployment(versionNumber) { | ||
spinner.setSpinnerTitle(LOG.DEPLOYMENT_CREATE); | ||
script.projects.deployments.create({ | ||
scriptId: scriptId, | ||
resource: { | ||
versionNumber: versionNumber, | ||
manifestFileName: PROJECT_MANIFEST_BASENAME, | ||
description: description | ||
} | ||
}, {}, function (err, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (err) { | ||
console.error(ERROR.DEPLOYMENT_COUNT); | ||
} | ||
else { | ||
console.log("- " + data.deploymentId + " @" + versionNumber + "."); | ||
} | ||
}); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
if (!scriptId) | ||
return; | ||
spinner.setSpinnerTitle(LOG.DEPLOYMENT_START(scriptId)); | ||
spinner.start(); | ||
function createDeployment(versionNumber) { | ||
spinner.setSpinnerTitle(LOG.DEPLOYMENT_CREATE); | ||
script.projects.deployments.create({ | ||
scriptId: scriptId, | ||
resource: { | ||
versionNumber: versionNumber, | ||
manifestFileName: PROJECT_MANIFEST_BASENAME, | ||
description: description | ||
} | ||
}, {}, function (err, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (err) { | ||
console.error(ERROR.DEPLOYMENT_COUNT); | ||
} | ||
else { | ||
console.log("- " + data.deploymentId + " @" + versionNumber + "."); | ||
} | ||
}); | ||
} | ||
// If the version is specified, update that deployment | ||
var versionRequestBody = { | ||
description: description | ||
}; | ||
if (version) { | ||
createDeployment(version); | ||
} | ||
else { | ||
script.projects.versions.create({ | ||
scriptId: scriptId, | ||
resource: versionRequestBody | ||
}, {}, function (err, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (err) { | ||
logError(null, ERROR.ONE_DEPLOYMENT_CREATE); | ||
} | ||
else { | ||
console.log(LOG.VERSION_CREATED(data.versionNumber)); | ||
createDeployment(data.versionNumber); | ||
} | ||
}); | ||
} | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
// If the version is specified, update that deployment | ||
var versionRequestBody = { | ||
description: description | ||
}; | ||
if (version) { | ||
createDeployment(version); | ||
} | ||
else { | ||
script.projects.versions.create({ | ||
scriptId: scriptId, | ||
resource: versionRequestBody | ||
}, {}, function (err, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (err) { | ||
logError(null, ERROR.ONE_DEPLOYMENT_CREATE); | ||
} | ||
else { | ||
console.log(LOG.VERSION_CREATED(data.versionNumber)); | ||
createDeployment(data.versionNumber); | ||
} | ||
}); | ||
} | ||
}); | ||
}); | ||
}); }); | ||
}); | ||
@@ -749,23 +889,31 @@ /** | ||
.action(function (deploymentId) { | ||
getAPICredentials(function () { | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
if (!scriptId) | ||
return; | ||
spinner.setSpinnerTitle(LOG.UNDEPLOYMENT_START(deploymentId)); | ||
spinner.start(); | ||
script.projects.deployments["delete"]({ | ||
scriptId: scriptId, | ||
deploymentId: deploymentId | ||
}, {}, function (err, res) { | ||
spinner.stop(true); | ||
if (err) { | ||
logError(null, ERROR.READ_ONLY_DELETE); | ||
} | ||
else { | ||
console.log(LOG.UNDEPLOYMENT_FINISH(deploymentId)); | ||
} | ||
}); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
if (!scriptId) | ||
return; | ||
spinner.setSpinnerTitle(LOG.UNDEPLOYMENT_START(deploymentId)); | ||
spinner.start(); | ||
script.projects.deployments["delete"]({ | ||
scriptId: scriptId, | ||
deploymentId: deploymentId | ||
}, {}, function (err, res) { | ||
spinner.stop(true); | ||
if (err) { | ||
logError(null, ERROR.READ_ONLY_DELETE); | ||
} | ||
else { | ||
console.log(LOG.UNDEPLOYMENT_FINISH(deploymentId)); | ||
} | ||
}); | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); }); | ||
}); | ||
@@ -779,26 +927,34 @@ /** | ||
.action(function (deploymentId, version, description) { | ||
getAPICredentials(function () { | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
script.projects.deployments.update({ | ||
scriptId: scriptId, | ||
deploymentId: deploymentId, | ||
resource: { | ||
deploymentConfig: { | ||
versionNumber: version, | ||
manifestFileName: PROJECT_MANIFEST_BASENAME, | ||
description: description | ||
} | ||
} | ||
}, {}, function (error, res) { | ||
spinner.stop(true); | ||
if (error) { | ||
logError(null, error); // TODO prettier error | ||
} | ||
else { | ||
console.log(LOG.REDEPLOY_END); | ||
} | ||
}); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
script.projects.deployments.update({ | ||
scriptId: scriptId, | ||
deploymentId: deploymentId, | ||
resource: { | ||
deploymentConfig: { | ||
versionNumber: version, | ||
manifestFileName: PROJECT_MANIFEST_BASENAME, | ||
description: description | ||
} | ||
} | ||
}, {}, function (error, res) { | ||
spinner.stop(true); | ||
if (error) { | ||
logError(null, error); // TODO prettier error | ||
} | ||
else { | ||
console.log(LOG.REDEPLOY_END); | ||
} | ||
}); | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); }); | ||
}); | ||
@@ -814,28 +970,36 @@ /** | ||
spinner.start(); | ||
getAPICredentials(function () { | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
script.projects.versions.list({ | ||
scriptId: scriptId | ||
}, {}, function (error, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (error) { | ||
logError(error); | ||
} | ||
else { | ||
if (data && data.versions && data.versions.length) { | ||
var numVersions = data.versions.length; | ||
console.log(LOG.VERSION_NUM(numVersions)); | ||
data.versions.map(function (version) { | ||
console.log(LOG.VERSION_DESCRIPTION(version)); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
script.projects.versions.list({ | ||
scriptId: scriptId | ||
}, {}, function (error, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (error) { | ||
logError(error); | ||
} | ||
else { | ||
if (data && data.versions && data.versions.length) { | ||
var numVersions = data.versions.length; | ||
console.log(LOG.VERSION_NUM(numVersions)); | ||
data.versions.map(function (version) { | ||
console.log(LOG.VERSION_DESCRIPTION(version)); | ||
}); | ||
} | ||
else { | ||
console.error(LOG.DEPLOYMENT_DNE); | ||
} | ||
} | ||
}); | ||
} | ||
else { | ||
console.error(LOG.DEPLOYMENT_DNE); | ||
} | ||
} | ||
}); | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); }); | ||
}); | ||
@@ -851,23 +1015,31 @@ /** | ||
spinner.start(); | ||
getAPICredentials(function () { | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
script.projects.versions.create({ | ||
scriptId: scriptId, | ||
description: description | ||
}, {}, function (error, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (error) { | ||
logError(error); | ||
} | ||
else { | ||
console.log(LOG.VERSION_CREATED(data.versionNumber)); | ||
} | ||
}); | ||
})["catch"](function (err) { | ||
spinner.stop(true); | ||
logError(err); | ||
getAPICredentials(function () { return __awaiter(_this, void 0, void 0, function () { | ||
return __generator(this, function (_a) { | ||
switch (_a.label) { | ||
case 0: return [4 /*yield*/, checkIfOnline()]; | ||
case 1: | ||
_a.sent(); | ||
getProjectSettings().then(function (_a) { | ||
var scriptId = _a.scriptId; | ||
script.projects.versions.create({ | ||
scriptId: scriptId, | ||
description: description | ||
}, {}, function (error, _a) { | ||
var data = _a.data; | ||
spinner.stop(true); | ||
if (error) { | ||
logError(error); | ||
} | ||
else { | ||
console.log(LOG.VERSION_CREATED(data.versionNumber)); | ||
} | ||
}); | ||
})["catch"](function (err) { | ||
spinner.stop(true); | ||
logError(err); | ||
}); | ||
return [2 /*return*/]; | ||
} | ||
}); | ||
}); | ||
}); }); | ||
}); | ||
@@ -874,0 +1046,0 @@ /** |
{ | ||
"name": "@google/clasp", | ||
"version": "1.1.1", | ||
"version": "1.1.2", | ||
"description": "Develop Apps Script Projects locally", | ||
@@ -41,2 +41,3 @@ "main": "index.js", | ||
"http-shutdown": "^1.2.0", | ||
"is-online": "^7.0.0", | ||
"mkdirp": "^0.5.1", | ||
@@ -43,0 +44,0 @@ "open": "^0.0.5", |
# clasp | ||
Develop [Apps Script](https://developers.google.com/apps-script/) projects locally usingclasp (*C*ommand *L*ine *A*pps *S*cript *P*rojects). | ||
Develop [Apps Script](https://developers.google.com/apps-script/) projects locally using clasp (*C*ommand *L*ine *A*pps *S*cript *P*rojects). | ||
@@ -5,0 +5,0 @@ 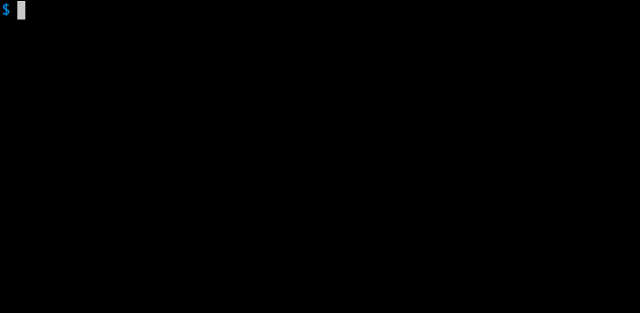 |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
69236
1168
22
+ Addedis-online@^7.0.0
+ Added@sindresorhus/is@0.7.0(transitive)
+ Addedaggregate-error@1.0.0(transitive)
+ Addedcacheable-request@2.1.4(transitive)
+ Addedcapture-stack-trace@1.0.2(transitive)
+ Addedclean-stack@1.3.0(transitive)
+ Addedclone-response@1.0.2(transitive)
+ Addedcore-util-is@1.0.3(transitive)
+ Addedcreate-error-class@3.0.2(transitive)
+ Addeddecode-uri-component@0.2.2(transitive)
+ Addeddecompress-response@3.3.0(transitive)
+ Addeddns-packet@1.3.4(transitive)
+ Addeddns-socket@1.6.3(transitive)
+ Addedduplexer3@0.1.5(transitive)
+ Addedfrom2@2.3.0(transitive)
+ Addedget-stream@3.0.0(transitive)
+ Addedgot@6.7.18.3.2(transitive)
+ Addedhas-symbol-support-x@1.4.2(transitive)
+ Addedhas-to-string-tag-x@1.4.1(transitive)
+ Addedhttp-cache-semantics@3.8.1(transitive)
+ Addedindent-string@3.2.0(transitive)
+ Addedinto-stream@3.1.0(transitive)
+ Addedip@1.1.9(transitive)
+ Addedip-regex@2.1.0(transitive)
+ Addedis-ip@2.0.0(transitive)
+ Addedis-object@1.0.2(transitive)
+ Addedis-online@7.0.0(transitive)
+ Addedis-plain-obj@1.1.0(transitive)
+ Addedis-redirect@1.0.0(transitive)
+ Addedis-retry-allowed@1.2.0(transitive)
+ Addedis-stream@1.1.0(transitive)
+ Addedisurl@1.0.0(transitive)
+ Addedjson-buffer@3.0.0(transitive)
+ Addedkeyv@3.0.0(transitive)
+ Addedlowercase-keys@1.0.01.0.1(transitive)
+ Addedmimic-response@1.0.1(transitive)
+ Addednormalize-url@2.0.1(transitive)
+ Addedp-any@1.1.0(transitive)
+ Addedp-cancelable@0.4.1(transitive)
+ Addedp-finally@1.0.0(transitive)
+ Addedp-is-promise@1.1.0(transitive)
+ Addedp-some@2.0.1(transitive)
+ Addedp-timeout@1.2.12.0.1(transitive)
+ Addedprepend-http@1.0.42.0.0(transitive)
+ Addedprocess-nextick-args@2.0.1(transitive)
+ Addedpublic-ip@2.5.0(transitive)
+ Addedquery-string@5.1.1(transitive)
+ Addedreadable-stream@2.3.8(transitive)
+ Addedresponselike@1.0.2(transitive)
+ Addedsafe-buffer@5.1.2(transitive)
+ Addedsort-keys@2.0.0(transitive)
+ Addedstrict-uri-encode@1.1.0(transitive)
+ Addedstring_decoder@1.1.1(transitive)
+ Addedtimed-out@4.0.1(transitive)
+ Addedunzip-response@2.0.1(transitive)
+ Addedurl-parse-lax@1.0.03.0.0(transitive)
+ Addedurl-to-options@1.0.1(transitive)
+ Addedutil-deprecate@1.0.2(transitive)