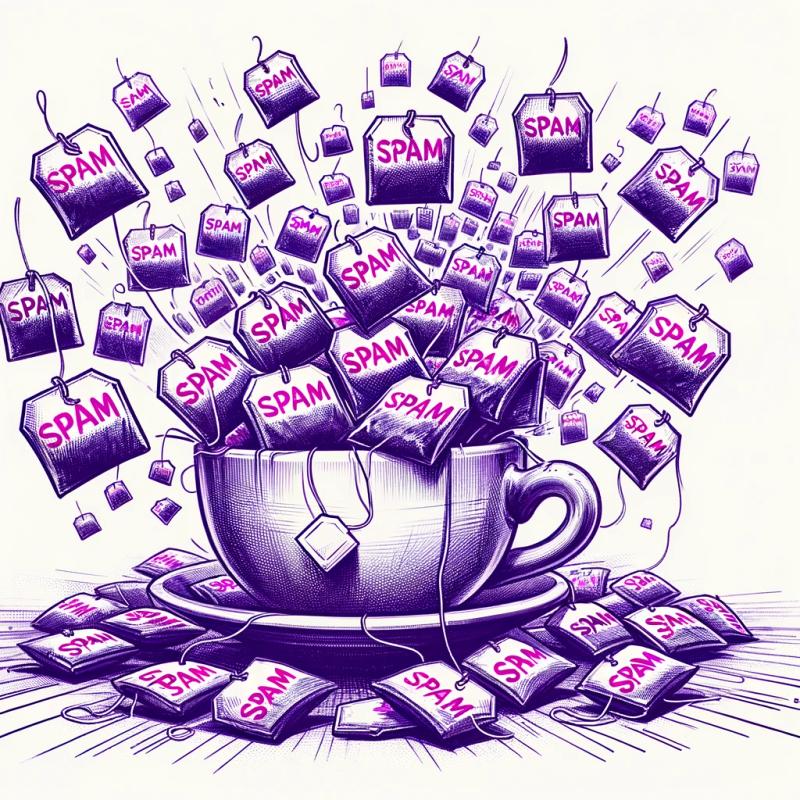
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@haensl/google-analytics
Advanced tools
Changelog
1.1.1
Readme
Google Analytics 4 JavaScript abstraction for ga4
(gtag) and measurement protocol
(API).
@haensl/google-analytics
is build with different runtime platforms (browser vs. Node.js) in mind:
On the client:
Use window.gtag
(default): @haensl/google-analytics
import { init, event } from '@haensl/google-analytics'
;
On the server:
Use measurement protocol: @haensl/google-analytics/measurement-protocol
import { init, event } from '@haensl/google-analytics/measurement-protocol'
;
Attention: The measurement protocol abstraction requires a fetch
implementation to work, e.g. node-fetch
, cross-fetch
, or similar. See init(config)
.
npm
$ npm install -S @haensl/google-analytics
yarn
$ yarn add @haensl/google-analytics
Client/tag and measurement protocol offer similar methods, but differ in initialization and dependencies.
init(confg)
: Initialize the gtag module.install
: Installs gtag stubs on window
.consent(granted)
: Consent to tracking.event(data)
: Track an event.exception(data)
: Track an exception.pageView(data)
: Track a page view.setUserId({ id })
: Set the user id.setUserProperty({ name, value })
init(confg)
: Initialize the measurement protocol module.clientId(cookies)
: Get a client id.async event(data)
: Track an event.async exception(data)
: Track an exception.async pageView(data)
: Track a page view.async setUserId({ id })
: Set the user id.async setUserProperty({ name, value })
@haensl/google-analytics
The tag implementation relies on the existence of window.gtag
. Please ensure that your page loads the Google Analytics 4 tag
, e.g. by including a <script src="...">
to fetch gtag.js
or via a component like @haensl/next-google-analytics
init(config)
import { init } from '@haensl/google-analytics'
Initializes the tracking module. You only need to call this once, e.g. when your app boots. This also calls install()
.
Tracking consent is defaulted to false
for EU region. See consent()
.
init({
/**
* Google measurement id.
*
* E.g. 'G-123ABC456D'
*/
measurementId,
/**
* Run gtag in debug mode.
*/
debug = false,
/**
* Anonymize IP setting.
*/
anonymizeIp = true,
/**
* Automatically send page views.
*/
sendPageViews = false,
/**
* Whether the user has given her consent to track.
*/
trackingConsent = false
})
install()
import { install } from '@haensl/google-analytics'
Installs window.dataLayer
and window.gtag
stubs, i.e. Google Docs. Is called on init()
.
consent(granted = false)
import { consent } from '@haensl/google-analytics'
Grant or deny tracking consent.
Granting/Denying tracking consent toggles:
For more information on consent mode, see Google's docs.
consent(granted = false)
Example: User grants consent:
import { consent } from '@haensl/google-analytics';
// Consent button click handler.
const onConsentTap = () => {
consent(true);
};
// Attach to consent button.
button.onClick = onConsentTap;
event({ name, params })
import { event } from '@haensl/google-analytics'
Track an event.
event({
/**
* Event name. String.
*/
name,
/**
* Event parameters.
*
* An object mapping names to strings or numbers.
*/
params
})
Example: Sign up event
import { event } from '@haensl/google-analytics';
// track a sign up from the footer form.
event({
name: 'sign_up',
params: {
form: 'footer'
}
});
exception({ description, fatal })
import { exception } from '@haensl/google-analytics'
Tracks an exception. Alias for event({ name: 'exception', ... })
.
exception({
/**
* Error description. String.
*/
description,
/**
* Whether or not the error is fatal. Boolean.
*/
fatal = false
})
pageView({ location, title })
import { pageView } from '@haensl/google-analytics'
Tracks a page view. Alias for event({ name: 'page_view', ... })
.
pageView({
/**
* Page title. String.
*/
title,
/**
* Page location. String.
*/
location
})
setUserId({ id })
import { setUserId } from '@haensl/google-analytics'
Set the user id.
setUserId({
/**
* A user id. String.
*/
id
})
setUserProperty({ name, value })
import { setUserProperty } from '@haensl/google-analytics'
Set a user property (formerly known as dimension).
setUserProperty({
/**
* User property name. String.
*/
name,
/**
* User property value. String or number.
*/
value
})
Example: track the users color scheme:
import { setUserProperty } from '@haensl/google-analytics';
setUserProperty({
name: 'appearance',
value: 'dark'
});
@haensl/google-analytics/measurement-protocol
The measurement protocol implementation requires an API secret as well as a fetch
implementation at initialization.
init(config)
import { init } from '@haensl/google-analytics/measurement-protocol'
Initialize the measurement protocol module.
init({
/**
* Fetch implementation. Function.
*/
fetch,
/**
* Google measurement id. String.
*
* E.g. 'G-123ABC456D'
*/
measurementId,
/**
* Google measurement API secret. String.
*/
measurementSecret,
/**
* Measurement API URL. String.
*/
measurementUrl = 'https://www.google-analytics.com/mp/collect'
})
Example
import fetch from 'node-fetch';
import { init } from '@haensl/google-analytics/measurement-protocol';
init({
fetch,
measurementId: 'G-123ABC456D',
measurementSecret: 'super-secret'
});
clientId(cookies)
import { clientId } from '@haensl/google-analytics/measurement-protocol'
Tries to parse the Google Analytics client id from the given cookies object.
Generates a client id from timestamps if not found in cookies.
clientId(cookies = {}) => String
Example: Usage with next-cookies
import { cookies } from 'next-cookies';
import { clientId, pageView } from '@haensl/google-analytics/measurement-protocol';
export const getServerSideProps = async (ctx) => {
const requestCookies = cookies(ctx);
const requestClientId = clientId(requestCookies);
pageView({
title: 'my serverside page',
clientId: requestClientId,
location: ctx.req.url
});
};
async event({ name, params, clientId })
import { event } from '@haensl/google-analytics/measurement-protocol'
Track an event.
async event({
/**
* Event name. String.
*/
name,
/**
* Event parameters.
*
* An object mapping names to strings or numbers.
*/
params,
/**
* The client id. String.
*/
clientId
}) => Promise
Example: Sign up event
import { event, clientId } from '@haensl/google-analytics/measurement-protocol';
// track a sign up from the footer form.
await event({
name: 'sign_up',
params: {
form: 'footer'
},
// Extract client id from context
clientId: clientId(ctx.cookies)
});
async exception({ description, fatal clientId })
import { exception } from '@haensl/google-analytics/measurement-protocol'
Tracks an exception. Alias for event({ name: 'exception', ... })
.
async exception({
/**
* Error description. String.
*/
description,
/**
* Whether or not the error is fatal. Boolean.
*/
fatal = false,
/**
* The client id. String.
*/
clientId
}) => Promise
async pageView({ location, title, clientId })
import { pageView } from '@haensl/google-analytics/measurement-protocol'
Tracks a page view. Alias for event({ name: 'page_view', ... })
.
async pageView({
/**
* Page title. String.
*/
title,
/**
* Page location. String.
*/
location,
/**
* The client id. String.
*/
clientId
}) => Promise
async setUserId({ id, clientId })
import { setUserId } from '@haensl/google-analytics/measurement-protocol'
Set the user id.
async setUserId({
/**
* A user id. String.
*/
id,
/**
* The client id. String.
*/
clientId
}) => Promise
async setUserProperty({ name, value, clientId })
import { setUserProperty } from '@haensl/google-analytics/measurement-protocol'
Set a user property.
async setUserProperty({
/**
* User property name. String.
*/
name,
/**
* User property value. String or number.
*/
value,
/**
* The client id. String.
*/
clientId
}) => Promsie
Example: track the users color scheme:
import { clientId, setUserProperty } from '@haensl/google-analytics/measurement-protocol';
await setUserProperty({
name: 'appearance',
value: 'dark',
// Extract client id from context.
clientId: clientId(ctx.cookies)
});
FAQs
Google Analytics 4 service abstraction for gtag and measurement protocol.
The npm package @haensl/google-analytics receives a total of 121 weekly downloads. As such, @haensl/google-analytics popularity was classified as not popular.
We found that @haensl/google-analytics demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.