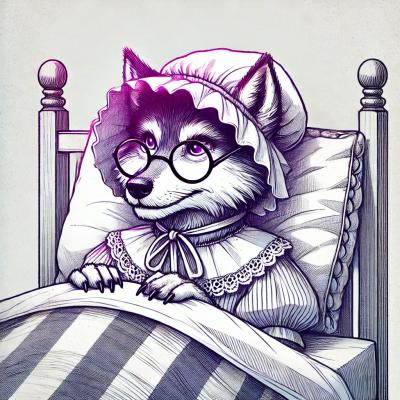
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@hapi/catbox
Advanced tools
@hapi/catbox is a multi-strategy caching library for Node.js, designed to work with the hapi.js framework but can be used independently. It provides a consistent API for various caching backends, making it easier to manage and optimize caching strategies.
Memory Caching
This code demonstrates how to use @hapi/catbox with an in-memory caching strategy. It initializes a Catbox client with the memory strategy, sets a value in the cache, and retrieves it.
const Catbox = require('@hapi/catbox');
const CatboxMemory = require('@hapi/catbox-memory');
const client = new Catbox.Client(CatboxMemory);
const start = async () => {
await client.start();
const key = { id: 'example', segment: 'examples' };
await client.set(key, 'myValue', 10000);
const value = await client.get(key);
console.log(value.item); // 'myValue'
};
start();
Redis Caching
This code demonstrates how to use @hapi/catbox with a Redis caching strategy. It initializes a Catbox client with the Redis strategy, sets a value in the cache, and retrieves it.
const Catbox = require('@hapi/catbox');
const CatboxRedis = require('@hapi/catbox-redis');
const client = new Catbox.Client(CatboxRedis, { host: '127.0.0.1', port: 6379 });
const start = async () => {
await client.start();
const key = { id: 'example', segment: 'examples' };
await client.set(key, 'myValue', 10000);
const value = await client.get(key);
console.log(value.item); // 'myValue'
};
start();
Custom Caching Strategy
This code demonstrates how to create and use a custom caching strategy with @hapi/catbox. It defines a custom cache class, initializes a Catbox client with this custom strategy, sets a value in the cache, and retrieves it.
const Catbox = require('@hapi/catbox');
class CustomCache {
constructor(options) {
this.cache = new Map();
}
async start() {}
async stop() {}
async get(key) {
return { item: this.cache.get(key.id) };
}
async set(key, value, ttl) {
this.cache.set(key.id, value);
}
async drop(key) {
this.cache.delete(key.id);
}
}
const client = new Catbox.Client(CustomCache);
const start = async () => {
await client.start();
const key = { id: 'example', segment: 'examples' };
await client.set(key, 'myValue', 10000);
const value = await client.get(key);
console.log(value.item); // 'myValue'
};
start();
node-cache is a simple and efficient in-memory caching module for Node.js. It provides basic caching functionalities with a straightforward API. Compared to @hapi/catbox, node-cache is more lightweight and easier to set up but lacks the multi-strategy support and flexibility.
cache-manager is a caching library for Node.js that supports multiple storage backends like memory, Redis, and more. It offers a similar level of flexibility as @hapi/catbox but with a different API design. cache-manager is a good alternative if you need a versatile caching solution with support for various storage backends.
lru-cache is a simple and efficient Least Recently Used (LRU) cache for Node.js. It is highly performant for scenarios where LRU caching is needed. Compared to @hapi/catbox, lru-cache is more specialized and optimized for LRU caching but does not offer the same level of flexibility in terms of different caching strategies.
catbox is part of the hapi ecosystem and was designed to work seamlessly with the hapi web framework and its other components (but works great on its own or with other frameworks). If you are using a different web framework and find this module useful, check out hapi – they work even better together.
FAQs
Multi-strategy object caching service
We found that @hapi/catbox demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.