@hello-pangea/dnd
Core characteristics
- Beautiful and natural movement of items 💐
- Accessible: powerful keyboard and screen reader support ♿️
- Extremely performant 🚀
- Clean and powerful api which is simple to get started with
- Plays extremely well with standard browser interactions
- Unopinionated styling
- No creation of additional wrapper dom nodes - flexbox and focus management friendly!
Get started 👩🏫
Alex Reardon has created a free course on egghead.io
🥚 (using react-beautiful-dnd) to help you get started with @hello-pangea/dnd
as quickly as possible.
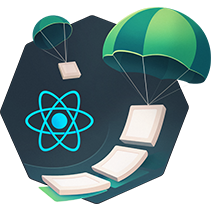
Currently supported feature set ✅
- Vertical lists ↕
- Horizontal lists ↔
- Movement between lists (▤ ↔ ▤)
- Virtual list support 👾 - unlocking 10,000 items @ 60fps
- Combining items
- Mouse 🐭, keyboard 🎹♿️ and touch 👉📱 (mobile, tablet and so on) support
- Multi drag support
- Incredible screen reader support ♿️ - we provide an amazing experience for english screen readers out of the box 📦. We also provide complete customisation control and internationalisation support for those who need it 💖
- Conditional dragging and conditional dropping
- Multiple independent lists on the one page
- Flexible item sizes - the draggable items can have different heights (vertical lists) or widths (horizontal lists)
- Add and remove items during a drag
- Compatible with semantic
<table>
reordering - table pattern - Auto scrolling - automatically scroll containers and the window as required during a drag (even with keyboard 🔥)
- Custom drag handles - you can drag a whole item by just a part of it
- Able to move the dragging item to another element while dragging (clone, portal) - Reparenting your
<Draggable />
- Create scripted drag and drop experiences 🎮
- Allows extensions to support for any input type you like 🕹
- 🌲 Tree support through the
@atlaskit/tree
package - A
<Droppable />
list can be a scroll container (without a scrollable parent) or be the child of a scroll container (that also does not have a scrollable parent) - Independent nested lists - a list can be a child of another list, but you cannot drag items from the parent list into a child list
- Server side rendering (SSR) compatible - see resetServerContext()
- Plays well with nested interactive elements by default
Motivation 🤔
@hello-pangea/dnd
exists to create beautiful drag and drop for lists that anyone can use - even people who cannot see. For a good overview of the history and motivations of the project you can take a look at these external resources:
Not for everyone ✌️
There are a lot of libraries out there that allow for drag and drop interactions within React. Most notable of these is the amazing react-dnd
. It does an incredible job at providing a great set of drag and drop primitives which work especially well with the wildly inconsistent html5 drag and drop feature. @hello-pangea/dnd
is a higher level abstraction specifically built for lists (vertical, horizontal, movement between lists, nested lists and so on). Within that subset of functionality @hello-pangea/dnd
offers a powerful, natural and beautiful drag and drop experience. However, it does not provide the breadth of functionality offered by react-dnd
. One shortcoming is that grid layouts are not supported (yet). So @hello-pangea/dnd
might not be for you depending on what your use case is.
Documentation 📖
About 👋
Sensors 🔉
The ways in which somebody can start and control a drag
API 🏋️
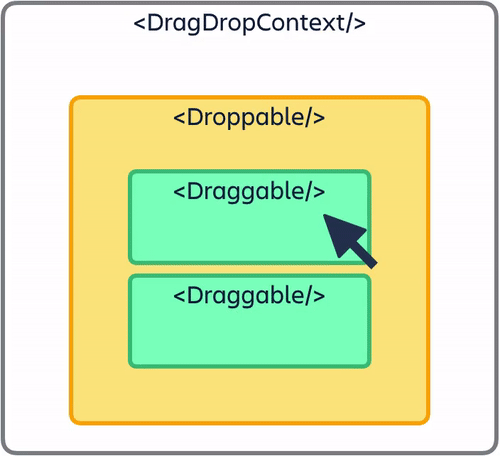
Guides 🗺
Patterns 👷
Support 👩⚕️
Read this in other languages 🌎
⚠️ These following translations are based on react-beautiful-dnd.
Creator ✍️
Alex Reardon @alexandereardon
Alex is no longer personally maintaning this project. The other wonderful maintainers are carrying this project forward.
Maintainers 🛠️
Collaborators 🤝
Thanks 🤗

Thanks to Chromatic for providing the visual testing platform that helps us review UI changes and catch visual regressions.