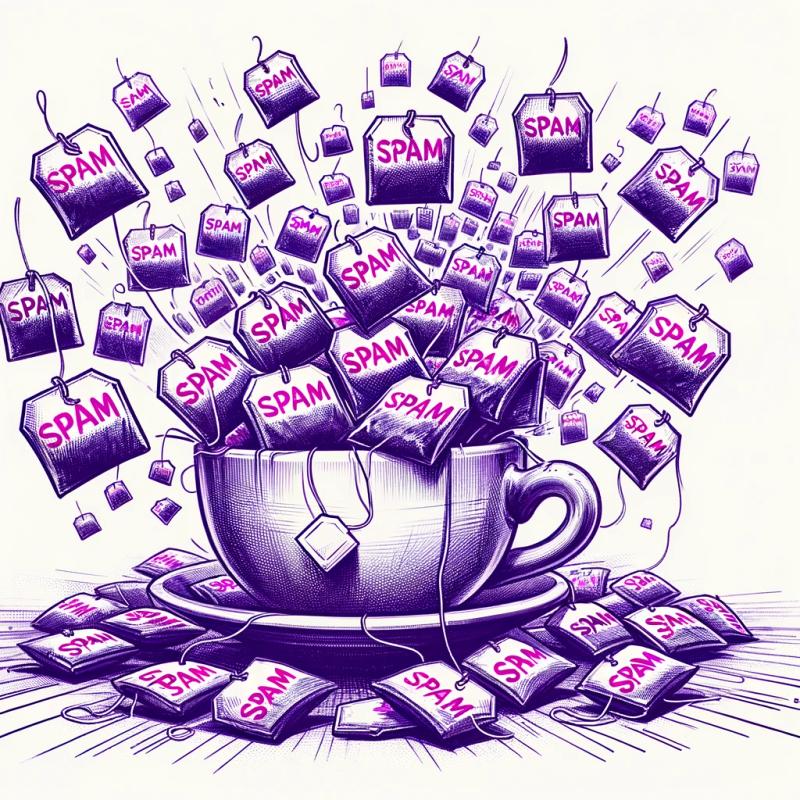
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@innovastudio/contentbox
Advanced tools
ContentBox.js is a web page designer. It uses ContentBuilder.js as its HTML editor with added features for page designing. You can use it to create your own CMS or online site builder.
Readme
ContentBox.js is a web page designer. It uses ContentBuilder.js as its HTML editor with added features for page designing. You can use it to create your own CMS or online site builder.
Include the ContentBuilder css files:
<link href="assets/minimalist-blocks/content.css" rel="stylesheet" type="text/css" /> <!-- for snippets -->
<link href="contentbuilder/contentbuilder.css" rel="stylesheet" type="text/css" />
and the main ContentBox css file:
<link href="contentbox/contentbox.css" rel="stylesheet" type="text/css" />
Include the js file:
<script src="contentbox/contentbox.min.js" type="text/javascript"></script>
npm install @innovastudio/contentbox
Then import into your project:
import ContentBox from '@innovastudio/contentbox';
For viewing content, additional includes are also needed.
On the head section:
<link href="box/box-flex.css" rel="stylesheet" type="text/css" />
Before the end of the body tag:
<script src="box/box-flex.js" type="text/javascript"></script>
(this is the same as in the previous version)
On the head section:
<link href="box/box.css" rel="stylesheet" type="text/css" />
Before the end of the body tag:
<script src="path-to/jquery.min.js"></script> /* for running the slider */
<script src="box/box.js" type="text/javascript"></script>
The JQuery include is needed here because the previous version of ContentBox includes predesigned Sliders (using Slick slider) that require JQuery to run. If you are not using the slider in your content, then you do not need JQuery include and you can change the box.css & box.js includes with the box-flex.css & box-flex.js which exclude the JQuery dependency.
<div class="is-wrapper">
</div>
const builder = new ContentBox({
wrapper: '.is-wrapper',
});
To get the edited content:
let html = builder.html();
let mainCss = builder.mainCss(); // Returns the default typography style for the page.
let sectioncss = builder.sectioncss(); // Returns the typography styles for specific sections on the page
Then you can do anything with the content, for example, posting it to the server for saving, etc.
In production, the saved HTML content should be rendered with its styles.
ContentBox.js is written in pure Javascript (ES6) so you can use it in most situations. Sample use in simple HTML, PHP, React and Vue projects are included.
React and Vue project examples are provided in separate downloads in the user area.
To start building a page, you can click the (+) button on the top left sidebar. This will open a selection of predesigned sections that you can add into your page.
There are 2 predesigned section categories:
The predesigned section files are located in the folder:
assets/designs/
It contains:
You can configure the file location by setting the designUrl1, designUrl2 and designPath parameters:
const builder = new ContentBox({
wrapper: '.is-wrapper',
designUrl1: 'assets/designs/basic.js',
designUrl2: 'assets/designs/examples.js',
designPath: 'assets/designs/',
});
In case of a different assets location, path adjustment may be needed. Here you can use the designPathReplace parameter.
Example:
const builder = new ContentBox({
wrapper: '.is-wrapper',
designUrl1: 'https://path-to/assets/designs/basic.js',
designUrl2: 'https://path-to/assets/designs/examples.js',
designPath: 'https://path-to/assets/designs/',
designPathReplace: ['assets/designs/', 'https://path-to/assets/designs/'], // replace the default path to the new location
});
In this example, the default location is changed to https://path-to/assets/designs/
With this, you can place all the assets in a separate server or different host (e.g. from a CDN).
Snippets are predesigned blocks that you can add or drag & drop into your content.
Snippet selection can be opened from the left sidebar.
Snippet files are located in the folder:
assets/minimalist-blocks/
It contains:
You can configure the snippets location by setting the snippetUrl and snippetPath parameters:
const builder = new ContentBox({
wrapper: '.is-wrapper',
snippetUrl: 'assets/minimalist-blocks/content.js', // Snippet file
snippetPath: 'assets/minimalist-blocks/', // Location of snippets' assets
});
In case of a different location, path adjustment may be needed. Here you can use the snippetPathReplace parameter.
Example:
const builder = new ContentBox({
wrapper: '.is-wrapper',
snippetUrl: 'https://path-to/assets/minimalist-blocks/content.js', // Snippet file
snippetPath: 'https://path-to/assets/minimalist-blocks/', // Location of snippets' assets
snippetPathReplace: ['assets/', 'https://path-to/assets/'], // replace the default path to the new location
});
In this example, the default location is changed to https://path-to/assets/minimalist-blocks/
With this, you can place all the snippet assets in a separate server or different host (e.g. from a CDN).
A selection of typography styles is provided for you to choose to format your page. The selection can be opened from the left sidebar.
The style can be used to format the entire page or just a specific section of your page.
To apply the typography style to a specific box area, open the Box Settings dialog, select the 'Text' tab and click 'Change Style'.
This will re-open the typography selection and your selection will be applied to the chosen box area only.
Typography style files are located in the folder:
assets/styles/
It contains all the css needed and its preview images. You can change its location using the contentStylePath parameter.
const builder = new ContentBox({
wrapper: '.is-wrapper',
contentStylePath: 'assets/styles/',
});
The new version includes predesigned slider templates (using Glide slider) that require some includes:
<link href="assets/scripts/glide/css/glide.core.css" rel="stylesheet" type="text/css" />
<link href="assets/scripts/glide/css/glide.theme.css" rel="stylesheet" type="text/css" />
<script src="assets/scripts/glide/glide.js" type="text/javascript"></script>
To enable the slider:
const builder = new ContentBox({
/*...*/
slider: 'glide' // default: 'slick' (old version slider)
});
Values:
With the Language file, you can translate the ContentBox.js interface into another language.
The language file is located in:
To enable the language file, you need to add the file before including ContentBox.js:
<script src="contentbox/lang/en.js" type="text/javascript"></script>
Here is the language file content as seen on en.js:
var _txt = new Array();
_txt['Bold'] = 'Bold';
_txt['Italic'] = 'Italic';
You can create your own language file by copying/modifying this file.
To add custom buttons to the sidebar, use the addButton method.
Here is an example of adding the Undo & Redo button. For the undo and redo operation, we call the undo() and redo() methods.
builder.addButton({
'pos': 2, // button position
'title': 'Undo', // title
'html': '<svg class="is-icon-flex" style="width:14px;height:14px;"><use xlink:href="#ion-ios-undo"></use></svg>', // icon
'onClick': ()=>{
builder.undo();
}
});
builder.addButton({
'pos': 3, // button position
'title': 'Redo', // title
'html': '<svg class="is-icon-flex" style="width:14px;height:14px;"><use xlink:href="#ion-ios-redo"></use></svg>', // icon
'onClick': ()=>{
builder.redo();
}
});
The addButton method has 4 parameters:
Here is another example for adding a Preview button. If clicked, the button will open a separate page (preview.html) that we use to preview our edited page as in production.
builder.addButton({
'pos': 5,
'title': 'Preview',
'html': '<svg class="is-icon-flex" style="width:16px;height:16px;"><use xlink:href="#ion-eye"></use></svg>',
'onClick': ()=>{
var html = builder.html();
localStorage.setItem('preview-html', html);
var mainCss = builder.mainCss();
localStorage.setItem('preview-maincss', mainCss);
var sectionCss = builder.sectionCss();
localStorage.setItem('preview-sectioncss', sectionCss);
window.open('/preview.html', '_blank').focus();
}
});
Here we get the content and its styles using the html(), mainCss(), and sectionCss() methods and save them into the browser's local storage. The content will then be used in the preview.html for viewing.
In the Box Settings, you can specify a box's cover or background image.
However, to quickly upload an image without opening the Box Settings, you can click the box image button. This allows you to browse local images and select an image to upload as a box image cover (background).
There are 2 methods for uploading cover image:
Here we use the coverImageHandler parameter to specify the upload handler for saving images on the server.
const builder = new ContentBox({
wrapper: '.is-wrapper',
coverImageHandler: 'savecover.php',
});
As examples, you can use the provided handler:
Here we use the onUploadCoverImage parameter to specify a custom upload function.
const builder = new ContentBox({
wrapper: '.is-wrapper',
onUploadCoverImage: (e) => {
uploadFile(e, (response)=>{
const uploadedImageUrl = response.url; // get saved image url
builder.boxImage(uploadedImageUrl); // change cover image
});
},
});
function uploadFile(e, callback) {
const selectedFile = e.target.files[0];
const formData = new FormData();
formData.append('file', selectedFile);
fetch('/upload', {
method: 'POST',
body: formData,
})
.then(response=>response.json())
.then(response=>{
console.log(response)
if(callback) callback(response);
});
}
The example above uses the boxImage(url) method to apply the image url as a background cover.
In the example, the image is posted to an endpoint: /upload If you’re using Node.js, you can implement the endpoint to save the image using:
const express = require('express');
const fs = require('fs');
const app = express();
const path = require('path');
const cors = require('cors');
const serveStatic = require('serve-static');
const formidable = require('formidable-serverless');
const sharp = require('sharp');
//Specify url path
var $path = __dirname + '/public/uploads/'; // Physical path
var $urlpath = 'uploads/'; // URL path
app.use(cors());
app.use(express.urlencoded({
extended: true
}));
app.use(express.json({ limit: '50mb' }));
app.use(serveStatic(path.join(__dirname, '/public')));
app.post('/upload', (req, res) => {
const form = new formidable.IncomingForm();
form.parse(req, async (err, fields, files) => {
if (err) return res.status(500).json({ ok:true, status: 500, error: 'Something went wrong.' });
let extension = path.extname(files.file.name).toLowerCase();
if(extension !== '.jpeg' && extension !== '.jpg' && extension !== '.png' && extension !== '.gif' && extension !== '.webp' && extension !== '.webm' && extension !== '.mp4') {
res.status(500).json({ ok:true, status: 500, error: 'File type not allowed.' });
return;
}
const file = fs.readFileSync(files.file.path);
let imageFile = file;
if(extension === '.jpeg' || extension === '.jpg') {
imageFile = await sharp(files.file.path).resize(1600, 1600, {
fit: sharp.fit.inside,
withoutEnlargement: true,
})
.jpeg({ quality: 80, progressive: true, force: false })
.toBuffer();
}
fs.writeFile($path + files.file.name, imageFile, async (err)=>{
if (err) {
res.status(500).json({ ok:true, status: 500, error: 'Something went wrong.' });
return
}
res.status(200).json({ ok:true, status: 200, url: $urlpath + files.file.name});
});
});
});
Content consists of HTML and its styles (e.g. typography styles/css includes). As explained previously, you get the edited content using the following methods:
let html = builder.html();
let mainCss = builder.mainCss(); // Returns the default typography style for the page.
let sectioncss = builder.sectioncss(); // Returns the typography styles for specific sections on the page
You can save the HTML and its styles above into a database. And when you need to load the content back for editing, use the loadHtml() and loadStyles methods.
builder.loadHtml(html);
builder.loadStyles(mainCss, sectionCss);
You can programmatically perform an undo or redo action.
To undo:
builder.undo();
To redo:
builder.redo();
To destroy the ContentBox:
builder.destroy();
ContentBox.js uses ContentBuilder.js as its HTML editor. So most of the ContentBuilder.js options/parameters can be accessed through the ContentBox.js object.
For example, to specify a custom file browser (or Asset Manager):
const builder = new ContentBox({
wrapper: '.is-wrapper',
imageSelect: 'assets.html',
fileSelect: 'assets.html',
videoSelect: 'assets.html',
});
And to specify custom upload functions:
const builder = new ContentBox({
wrapper: '.is-wrapper',
onMediaUpload: (e)=>{
...
},
onVideoUpload: (e)=>{
...
},
});
More configuration options can be found in the ContentBuilder.js documentation: https://innovastudio.com/docs/contentbuilder.pdf
FAQs
Unknown package
The npm package @innovastudio/contentbox receives a total of 128 weekly downloads. As such, @innovastudio/contentbox popularity was classified as not popular.
We found that @innovastudio/contentbox demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.