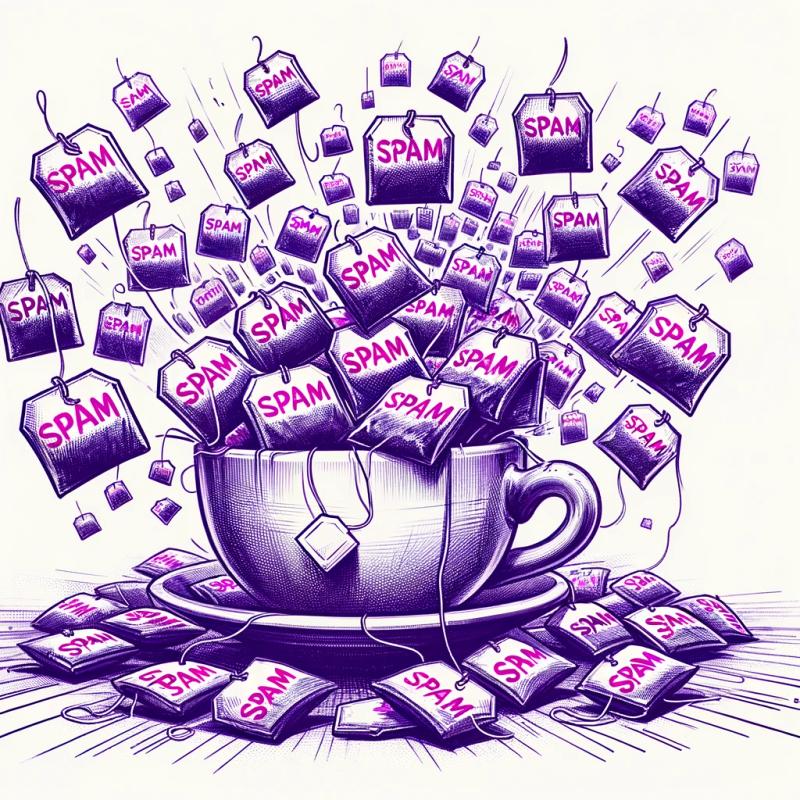
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@meedwire/react-native-video-manager
Advanced tools
Readme
Package for manager video
yarn add react-native-video-manager
import { getFramesVideo } from 'react-native-video-manager';
// ...
const App: React.FC = () => {
const [source, setSouce] = useState()
const getFrames = useCallback(async () => {
try {
if (!source) return;
const frames = await getVideoFrames(source);
setFrames(frames);
}catch(e){
console.error(e)
}
}, [source])
return (
<View>
<Button title="Retrieve Frames" onPress={getFrames} />
<View/>
)
}
import { compress } from 'react-native-video-manager';
// ...
const App: React.FC = () => {
const [source, setSouce] = useState()
const handleCropVideo = useCallback(async () => {
try {
if (!source) return;
const {
filePath,
fileSize,
originalFileSize
} = await compress(source);
setSouce(filePath);
}catch(e){
console.error(e)
}
}, [source])
return (
<View>
<Button title="Retrieve Frames" onPress={handleCropVideo} />
<View/>
)
}
import { cropVideo } from 'react-native-video-manager';
// ...
const App: React.FC = () => {
const [source, setSouce] = useState()
const handleCropVideo = useCallback(async () => {
try {
if (!source) return;
// Seconds
const startTime = 0;
// Seconds
const endTime = 10;
const source = await cropVideo(source, {startTime, endTime});
setSouce(source);
}catch(e){
console.error(e)
}
}, [source])
return (
<View>
<Button title="Retrieve Frames" onPress={handleCropVideo} />
<View/>
)
}
See the contributing guide to learn how to contribute to the repository and the development workflow.
MIT
Made with create-react-native-library
FAQs
Used to assist in video manipulation
The npm package @meedwire/react-native-video-manager receives a total of 1 weekly downloads. As such, @meedwire/react-native-video-manager popularity was classified as not popular.
We found that @meedwire/react-native-video-manager demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.