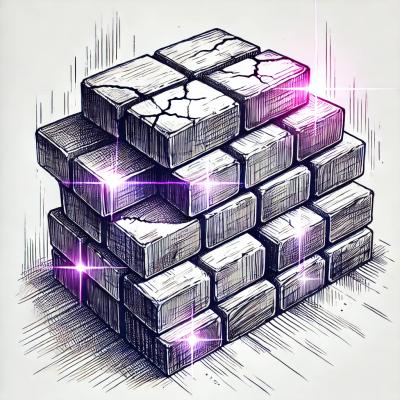
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@mirrorworld/evm.asset-minting
Advanced tools
@mirrorworld/evm.asset-minting
Client SDKThis SDK contains the client side methods for the Asset Minting EVM Smart Contract
🚨 Please make sure to add this NPM token in your
.npmrc
file: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
yarn add @mirrorworld/evm.asset-minting
Import the AssetMintingLib
instance into your client for using the Asset Minting 1155 Smart Contract. It expects
a contractAddress
and provider
instance.
Import the AssetMinting721Lib
instance into your client for using the Asset Minting 721 Smart Contract. It expects
a contractAddress
and provider
instance.
Import the TokenLib
instance into your client for using the ERC 1155 Token Smart Contract. It expects
a contractAddress
and provider
instance.
Import the Token721Lib
instance into your client for using the ERC 721 Token Smart Contract. It expects
a contractAddress
and provider
instance.
import {
AssetMintingLib,
TokenLib,
AssetMinting721Lib,
Token721Lib
} from "@mirrorworld/evm.asset-minting";
const RPC = "http://localhost:8500";
let provider = new ethers.providers.JsonRpcProvider(RPC);
const assetMinting1155Lib: AssetMintingLib = new AssetMintingLib("Contract Address", provider);
const token1155Lib: TokenLib = new TokenLib("Contract Address", provider);
const assetMinting721Lib: AssetMinting721Lib = new AssetMinting721Lib("Contract Address", provider);
const token721Lib: Token721Lib = new Token721Lib("Contract Address", provider);
Example: You can see example project in this repo for 721 and 1155
Deploy the new 721 token from the contract.
const messageHash = assetMintingLib.getDeployTokenMessageHash(salt, tokenOwnerAddress.address, baseUrl, name, symbol, trackMint, burnEnable, mintEnable, mintStartId, mintEndId);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createDeployTokenTransactionRequest(salt,
tokenOwnerAddress.address,
baseUrl,
name,
symbol,
trackMint,
burnEnable,
mintEnable,
mintStartId,
mintEndId,
sig,
gasPrice,
gasLimit);
const sigTx = await tokenOwnerAddress.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
tokenAddress = txRe.logs[0].address;
Deploy the new 1155 token from the contract.
const messageHash = assetMintingLib.getDeployTokenMessageHash(salt, tokenOwnerAddress.address, baseUrl, trackMint, burnEnable, mintEnable, mintStartId, mintEndId, mintAmount);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createDeployTokenTransactionRequest(salt,
tokenOwnerAddress.address,
baseUrl,
trackMint,
burnEnable,
mintEnable,
mintStartId,
mintEndId,
mintAmount,
sig,
gasPrice,
gasLimit);
const sigTx = await tokenOwnerAddress.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
tokenAddress = txRe.logs[0].address;
Mint method for minting the 721 token from the contract.
const messageHash = assetMintingLib.getMintTokenMessageHash(salt, tokenAddress, caller.address, toAddress, tokenId);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createMintTokenTransactionRequest(salt,
tokenAddress,
caller.address,
toAddress,
tokenId,
sig,
gasPrice,
gasLimit);
const sigTx = await caller.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Safe Mint method for minting the 721 token from the contract.
const messageHash = assetMintingLib.getSafeMintTokenMessageHash(salt, tokenAddress, caller.address, toAddress, tokenId);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createSafeMintTokenTransactionRequest(salt,
tokenAddress,
caller.address,
toAddress,
tokenId,
sig,
gasPrice,
gasLimit);
const sigTx = await caller.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Mint method for minting single 1155 token from the contract.
const messageHash = assetMintingLib.getMintTokenMessageHash(salt, tokenAddress, caller.address, toAddress, tokenId, amount, tokenData);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createMintTokenTransactionRequest(salt,
tokenAddress,
caller.address,
toAddress,
tokenId,
amount,
tokenData,
sig,
gasPrice,
gasLimit);
const sigTx = await caller.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Mint method for minting batch 1155 tokens from the contract.
const messageHash = assetMintingLib.getMintBatchTokenMessageHash(salt, tokenAddress, caller.address, toAddress, tokenIds, amounts, tokenData);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createMintBatchTokenTransactionRequest(salt,
tokenAddress,
caller.address,
toAddress,
tokenIds,
amounts,
tokenData,
sig,
gasPrice,
gasLimit);
const sigTx = await caller.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Enable or disable minting by token owner for asset minting 721 and 1155 contract.
const messageHash = assetMintingLib.getEnableOrDisableMintingByTokenOwnerMessageHash(salt, tokenAddress, tokenOwnerWallet.address, value);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createEnableOrDisableMintingByTokenOwnerTransactionRequest(salt,
tokenAddress,
tokenOwnerWallet.address,
value,
sig,
gasPrice,
gasLimit);
const sigTx = await tokenOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Enable or disable burn by token owner for asset minting 721 and 1155 contract.
const messageHash = assetMintingLib.getEnableOrDisableBurnByTokenOwnerMessageHash(salt, tokenAddress, tokenOwnerWallet.address, value);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createEnableOrDisableBurnByTokenOwnerTransactionRequest(salt,
tokenAddress,
tokenOwnerWallet.address,
value,
sig,
gasPrice,
gasLimit);
const sigTx = await tokenOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Token paused or unpaused by token owner for asset minting 721 and 1155 contract.
const messageHash = assetMintingLib.getTokenPausedOrUnpausedByTokenOwnerMessageHash(salt, tokenAddress, tokenOwnerWallet.address, value);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createTokenPausedOrUnpausedByTokenOwnerTransactionRequest(salt,
tokenAddress,
tokenOwnerWallet.address,
value,
sig,
gasPrice,
gasLimit);
const sigTx = await tokenOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Append mint end id by token owner for asset minting 721 and 1155 contract.
const messageHash = assetMintingLib.getAppendMintEndIdByTokenOwnerMessageHash(salt, tokenAddress, tokenOwnerWallet.address, value);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createAppendMintEndIdByTokenOwnerTransactionRequest(salt,
tokenAddress,
tokenOwnerWallet.address,
value,
sig,
gasPrice,
gasLimit);
const sigTx = await tokenOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Append mint amount by token owner for asset minting 1155 contract.
const messageHash = assetMintingLib.getAppendMintAmountByTokenOwnerMessageHash(salt, tokenAddress, tokenOwnerWallet.address, value);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createAppendMintAmountByTokenOwnerTransactionRequest(salt,
tokenAddress,
tokenOwnerWallet.address,
value,
sig,
gasPrice,
gasLimit);
const sigTx = await tokenOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Unlimited mint ids and amount by token owner for asset minting 721 and 1155 contract.
const messageHash = assetMintingLib.getUnlimitedMintIdsAndAmountByTokenOwnerMessageHash(salt, tokenAddress, tokenOwnerWallet.address);
const sig = await assetMintingLib.signMessage(ownerWallet, messageHash);
const tx: TransactionRequest = await assetMintingLib.createSetUnlimitedMintIdsAndAmountByTokenOwnerTransactionRequest(salt,
tokenAddress,
tokenOwnerWallet.address,
sig,
gasPrice,
gasLimit);
const sigTx = await tokenOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Set verify sig contract address for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createSetVerifySigAddressTransactionRequest(
contractOwnerWallet.address,
address,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Set messages contract address for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createSetMessagesAddressTransactionRequest(
contractOwnerWallet.address,
address,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Set token factory contract Address for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createSetTokenFactoryAddressTransactionRequest(
contractOwnerWallet.address,
address,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Deactivate token for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createDeactivateTokenTransactionRequest(
contractOwnerWallet.address,
tokenAddress,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Activate token for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createActivateTokenTransactionRequest(
contractOwnerWallet.address,
tokenAddress,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Deactivate and paused token for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createDeactivateAndPausedTokenTransactionRequest(
contractOwnerWallet.address,
tokenAddress,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Activate and unpaused token for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createActivateAndUnpausedTokenTransactionRequest(
contractOwnerWallet.address,
tokenAddress,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Enable or disable token minting for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createEnableOrDisableTokenMintingTransactionRequest(
contractOwnerWallet.address,
tokenAddress,
value,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Enable or disable token burn for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createEnableOrDisableTokenBurnTransactionRequest(
contractOwnerWallet.address,
tokenAddress,
value,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Pause or unpause token for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createPauseOrUnpauseTokenTransactionRequest(
contractOwnerWallet.address,
tokenAddress,
value,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
Pause or unpause contract for asset minting 721 and 1155 contract.
const tx: TransactionRequest = await assetMintingLib.createPauseOrUnpauseContractTransactionRequest(
contractOwnerWallet.address,
value,
gasPrice,
gasLimit);
const sigTx = await contractOwnerWallet.signTransaction(tx);
const sentTx = await provider.sendTransaction(sigTx);
const txRe = await sentTx.wait();
FAQs
Medusa SDK Core
The npm package @mirrorworld/evm.asset-minting receives a total of 0 weekly downloads. As such, @mirrorworld/evm.asset-minting popularity was classified as not popular.
We found that @mirrorworld/evm.asset-minting demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.