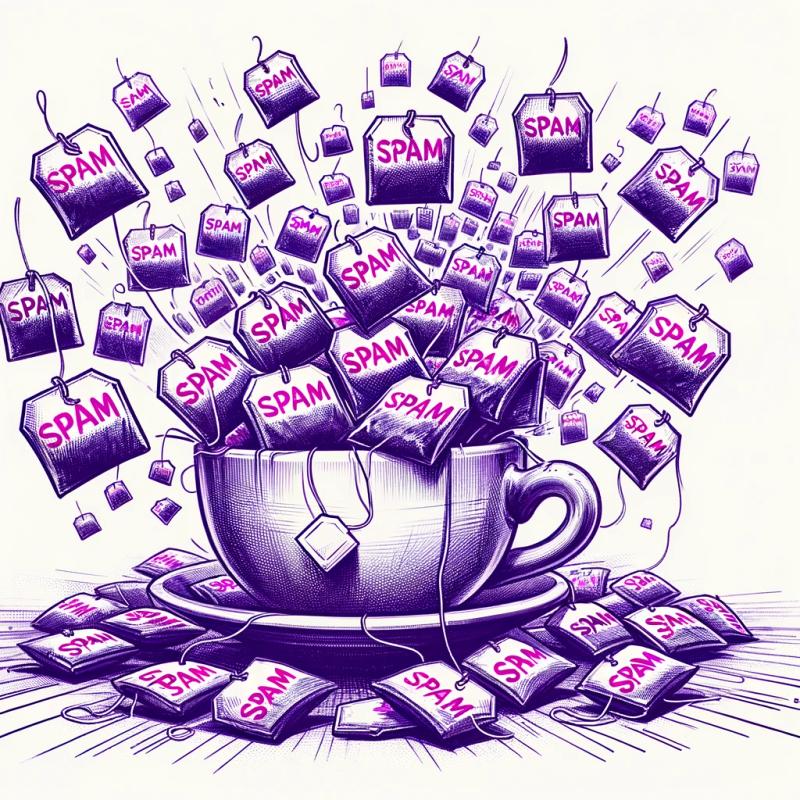
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@mxenabled/web-widget-sdk
Advanced tools
Changelog
[0.0.8] - 2022-06-16
Readme
The purpose of this project is to help simplify your integration experience. Giving you as few steps as possible to get up and running with an MX web widget.
After following the Getting Started instructions below, you will be able to import and load MX widgets in your web applications, and configure them for your specific needs.
Please refer to the official MX Docs for an in-depth
explanation of the MX platform. The general outline for incorporating the
web-widget-sdk
into your project is as follow:
npm
or yarn
.A developer account can be obtained by signing up on our Client Portal site.
Open a terminal and run the commands below at the root of your project to install the SDK:
Using npm
npm install --save @mxenabled/web-widget-sdk
Using yarn
yarn add @mxenabled/web-widget-sdk
The Widget SDK provides multiple modules that you can import and use in your application. There is currently a CommonJS module, an ES module, an AMD module, and an UMD module that you can use depending on your build process.
A CommonJS module is exported by this package and available by requiring
@mxenabled/web-widget-sdk
. Require this module and build your project with a
build tool that supports CommonJS modules (such as browserify):
const widgetSdk = require("@mxenabled/web-widget-sdk")
const widget = new widgetSdk.ConnectWidget({ /* options */ })
An ES module is exported by this package and available by importing
@mxenabled/web-widget-sdk
. Import this module and build your project with a
build tool that supports ES modules (such as webpack):
import * as widgetSdk from "@mxenabled/web-widget-sdk"
const widget = new widgetSdk.ConnectWidget({ /* options */ })
The AMD module can be found in
node_modules/@mxenabled/web-widget-sdk/dist/amd/index.js
. In order to serve
this file in your application, you will have to host it yourself. Transfer this
file into any location where it can be made publicly available from. Once the
file is available, you can import and use it like so:
<script src="https://requirejs.org/docs/release/2.3.6/minified/require.js"></script>
<script>
requirejs(["<path to MX Web Widget SDK AMD JavaScript file>"], function (widgetSdk) {
const widget = new widgetSdk.ConnectWidget({ /* options */ })
})
</script>
The UMD module can be found in
node_modules/@mxenabled/web-widget-sdk/dist/umd/index.js
. In order to serve
this file in your application, you will have to host it yourself. Transfer this
file into any location where it can be made publicly available from. Once the
file is available, you can import and use it like so:
<div id="container"></div>
<script src="<path to MX Web Widget SDK UMD JavaScript file>"></script>
<script>
const widget = new widgetSdk.ConnectWidget({ /* options */ })
</script>
See SSO Widget URL documentation for instructions. If
loading the Connect Widget, follow the instructions located in Connect SSO
Widget URL documentation. The SSO URL should be
passed to a Widget class via the url
option.
const options = {
container: "#widget",
url: "https://int-widgets.moneydesktop.com/md/connect/..."
}
The SDK also has the option of making the SSO request on your behalf to your
backend service that is able to make requests to our API. If used, the proxy
URL should passed to a Widget class via the proxy
option.
const options = {
container: "#widget",
proxy: "http://localhost:8089/{widget_type}/{user_guid}",
}
When you instantiate a widget with options, it will mount itself in the DOM, and set up various event listeners.
You'll need to call the unmount
method when closing the widget and before creating a new instance.
const options = {
container: "#widget",
widgetURL: "https://int-widgets.moneydesktop.com/md/connect/...."
}
// Calling `new sdk.ConnectWidget(...)` here will mount the widget in the DOM
const widget = new widgetSdk.ConnectWidget(options)
// When you are ready to close the widget, you'll want to call `unmount`. This
// will remove the element and any event listeners added.
widget.unmount()
You can listen to post message events by passing callback functions in the widget options object to the class. The option names follow this naming scheme:
on<event name>
,on<entity><action>
For example, the mx/connect/selectInstitution
event is made available via
onSelectInstitution
in the ConnectWidget
class. Refer to this
document for a list of events and their
payloads.
You can configure the state and behavior of the widget with the following class options:
language
: Load the widget in the specified language. Defaults to en-US
.
See language
options
for additional information.proxy
: SSO proxy server URL.style
: Styles applied to the view containing the widget.iframeTitle
: Allows for the title attribute of the iframe to be set.url
: Widget SSO URL. See Generating your Widget SSO
URL for additional information. This prop
is required.colorScheme
: Load the widget in the specified colorScheme; options are
light
and dark
. Defaults to light
.currentInstitutionCode
: Load the widget into the credential view for the
specified institution.currentInstitutionGuid
: Load the widget into the credential view for the
specified institution.currentMemberGuid
: Load to a specific member that contains an error or
requires MFA from the most recent job. currentMemberGuid
takes precedence
over currentInstitutionCode
.disableInstitutionSearch
: When set to true, the institution search feature
will be disabled and end users will not be able to navigate to it. Must be
used with currentInstitutionCode
, currentInstitutionGuid
, or
currentMemberGuid
.includeTransactions
: When set to false while creating or updating a member,
transaction data will not be automatically aggregated. Future manual or
background aggregations will not be affected. Defaults to true.uiMessageWebviewUrlScheme
: Used as the scheme that MX will redirect to at
the end of OAuth. This must be a scheme that your application responds to.
See OAuth redirects for additional information.updateCredentials
: Loads widget to the update credential view of a current
member. Optionally used with currentMemberGuid
. This option should be used
sparingly. The best practice is to use currentMemberGuid
and let the widget
resolve the issue.waitForFullAggregation
: Loads Connect, but forces the widget to wait until
any aggregation-type process is complete in order to fire a member connected
postMessage. This allows clients to have transactional data by the time the
widget is closed.This SDK exposes the following classes:
AccountsWidget
BudgetsWidget
ConnectAggregationWidget
ConnectVerificationWidget
ConnectWidget
ConnectionsWidget
DebtsWidget
FinstrongWidget
GoalsWidget
HelpWidget
MasterWidget
MiniBudgetsWidget
MiniFinstrongWidget
MiniPulseCarouselWidget
MiniSpendingWidget
PulseWidget
SettingsWidget
SpendingWidget
TransactionsWidget
TrendsWidget
FAQs
MX Web Widget SDK
The npm package @mxenabled/web-widget-sdk receives a total of 3,273 weekly downloads. As such, @mxenabled/web-widget-sdk popularity was classified as popular.
We found that @mxenabled/web-widget-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 23 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.