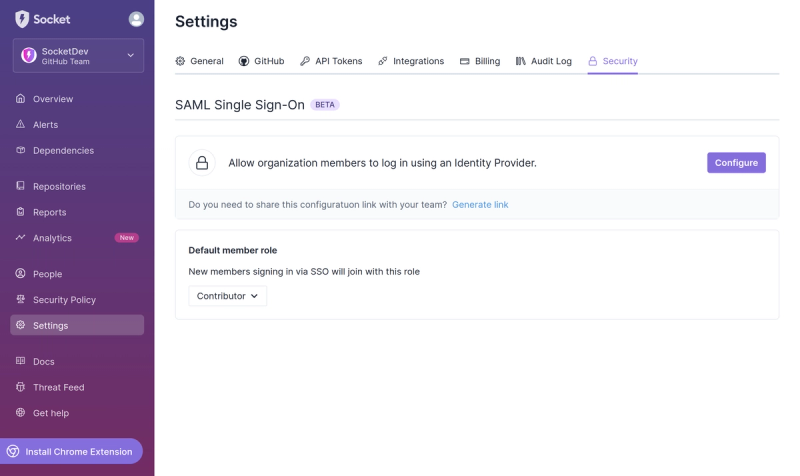
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@nestjs-hybrid-auth/facebook
Advanced tools
Readme
Implement facebook authentication in your NestJS application.
npm install @nestjs-hybrid-auth/facebook --save
OR
yarn add @nestjs-hybrid-auth/facebook
The package exports mainly a dynamic module and guard. The module should be imported in your app.module.ts and guards should be used on the route handlers of any controller.
Want to jump directly to the available options?
If you just want to provide the static values or have them handy, pass them as options to the forRoot
static method like below. The options object is type of FacebookAuthModuleOptions
.
import { FacebookAuthModule } from '@nestjs-hybrid-auth/facebook';
@Module({
imports: [
FacebookAuthModule.forRoot({
clientID: process.env.CLIENT_ID,
clientSecret: process.env.CLIENT_SECRET,
callbackURL: process.env.CALLBACK_URL,
}),
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
useFactory
to get the ConfigService injected.If you want to make use of nest's ConfigModule to get the auth configuration for a provider from .env
config files, use forRootAsync
static method. The options to this method are typeof FacebookAuthModuleAsyncOptions
which accepts a useFactory
property. useFactory
is a function which gets the instances injected whatever has been provided in inject
array. You can use those instances to prepare and return the actual FacebookAuthModuleOptions
object. ConfigService can be one of them as per your choice.
import { FacebookAuthModule } from '@nestjs-hybrid-auth/facebook';
@Module({
imports: [
ConfigModule.forRoot({
isGlobal: true,
cache: true,
expandVariables: true,
}),
FacebookAuthModule.forRootAsync({
imports: [ConfigModule],
inject: [ConfigService],
useFactory: (configService: ConfigService) => ({
clientID: configService.get('FACEBOOK_CLIENT_ID'),
clientSecret: configService.get('FACEBOOK_CLIENT_SECRET'),
callbackURL: configService.get('FACEBOOK_CALLBACK_URL'),
}),
}),
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
useClass
to get your auth config from a classIf the useFactory
makes your app module bloated with a lot of boilerplate code, you can useClass
to provide an existing config provider class. The class must implement FacebookAuthModuleOptionsFactory
interface and createModuleOptions
method. This method should return FacebookAuthModuleOptions
object. Similar to useFactory
, whatever you provide in inject
array, it will get injected in the constructor of your class. Follow the example:
hybrid-auth.config.ts
import { ConfigService } from '@nestjs/config';
import {
FacebookAuthModuleOptions,
FacebookAuthModuleOptionsFactory,
} from '@nestjs-hybrid-auth/facebook';
@Injectable()
class HybridAuthConfig implements FacebookAuthModuleOptionsFactory {
constructor(private configService: ConfigService) {}
createModuleOptions(): FacebookAuthModuleOptions {
return {
clientKey: this.configService.get('FACEBOOK_CLIENT_ID'),
clientSecret: this.configService.get('FACEBOOK_CLIENT_SECRET'),
callbackURL: this.configService.get('FACEBOOK_CALLBACK_URL'),
};
}
}
app.module.ts
import { FacebookAuthModule } from '@nestjs-hybrid-auth/facebook';
@Module({
imports: [
ConfigModule.forRoot({
isGlobal: true,
cache: true,
expandVariables: true,
}),
FacebookAuthModule.forRootAsync({
imports: [ConfigModule],
inject: [ConfigService],
useClass: HybridAuthConfig,
}),
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
Once you have setup the module properly in module file, its time to configure your route handlers to make the user properly redirected to appropriate identity provider's login page. @nestjs-hybrid-auth/facebook
provides a guard and result interface to make it enabled.
Each route will have two variants. One is to redirect to social login page and the other is to collect the response such as access/refresh tokens and user profile etc. The result will be attached to Request
object's hybridAuthResult
property as shown in the example below.
import {
UseFacebookAuth,
FacebookAuthResult,
} from '@nestjs-hybrid-auth/facebook';
@Controller()
export class AppController {
constructor(private readonly appService: AppService) {}
@UseFacebookAuth()
@Get('auth/facebook')
loginWithFacebook() {
return 'Login with Facebook';
}
@UseFacebookAuth()
@Get('auth/facebook-login/callback')
facebookCallback(@Request() req): Partial<FacebookAuthResult> {
const result: FacebookAuthResult = req.hybridAuthResult;
return {
accessToken: result.accessToken,
refreshToken: result.refreshToken,
profile: result.profile,
};
}
}
@nestjs-hybrid-auth/facebook
exports various decorators, interfaces and methods.
UseFacebookAuth
is NestJS Guard
which hijacks your nest request and redirects users to the appropriate login page of your configured identity provider (facebook in this case). The same guard can be used on callback
route also as shown in the example above. In the callback route handler, the req: Request
object will have a property hybridAuthResult
which is an object of type FacebookAuthResult
.
@UseFacebookAuth(options: FacebookAuthGuardOptions)
@Get('auth/facebook')
loginWithFacebook() {
return 'Login with Facebook';
}
This is a simple object to be passed into UseFacebookAuth
guard as shown in example above if you want to pass some extra parameters to query the facebook result. It can be left empty for default result.
This is the dynamic module which must be imported in your app's main module with forRoot
or forRootAsync
static methods whichever suits your need. Both will return a NestJS dynamic module.
interface FacebookAuthModule {
forRoot(options: FacebookAuthModuleOptions): DynamicModule;
forRootAsync(options: FacebookAuthModuleAsyncOptions): DynamicModule;
}
If you are configuring your module with forRoot
static method, pass in the module options given below. They can be called the facebook passport strategy options also.
interface FacebookAuthModuleOptions {
clientID: string;
clientSecret: string;
callbackURL: string;
scopeSeparator?: string | undefined;
enableProof?: boolean | undefined;
profileFields?: string[] | undefined;
authorizationURL?: string | undefined;
tokenURL?: string | undefined;
profileURL?: string | undefined;
graphAPIVersion?: string | undefined;
display?: 'page' | 'popup' | 'touch' | undefined;
authType?: 'reauthenticate' | undefined;
authNonce?: string | undefined;
}
If you want to configure the FacebookAuthModule
dynamically having the config or other services injected, pass in async options in the forRootAsync
static method. Please refer to the example above for useFactory
and useClass
properties.
interface FacebookAuthModuleAsyncOptions {
useExisting?: Type<FacebookAuthModuleOptionsFactory>;
useClass?: Type<FacebookAuthModuleOptionsFactory>;
useFactory?: (
...args: any[]
) => Promise<FacebookAuthModuleOptions> | FacebookAuthModuleOptions;
inject?: any[];
}
interface FacebookAuthModuleOptionsFactory {
createModuleOptions():
| Promise<FacebookAuthModuleOptions>
| FacebookAuthModuleOptions;
}
If you still have trouble setting up the workflow properly, please file an issue at Issues page.
FAQs
NestJS facebook authentication using passport
The npm package @nestjs-hybrid-auth/facebook receives a total of 35 weekly downloads. As such, @nestjs-hybrid-auth/facebook popularity was classified as not popular.
We found that @nestjs-hybrid-auth/facebook demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.