@nestjs/common
Advanced tools
Comparing version 3.0.3 to 3.1.0
@@ -17,1 +17,3 @@ export declare const metadata: { | ||
export declare const GUARDS_METADATA = "__guards__"; | ||
export declare const INTERCEPTORS_METADATA = "__interceptors__"; | ||
export declare const HTTP_CODE_METADATA = "__httpCode__"; |
@@ -19,1 +19,3 @@ "use strict"; | ||
exports.GUARDS_METADATA = '__guards__'; | ||
exports.INTERCEPTORS_METADATA = '__interceptors__'; | ||
exports.HTTP_CODE_METADATA = '__httpCode__'; |
export * from './utils'; | ||
export * from './enums'; | ||
export { NestModule, INestApplication, INestMicroservice, MiddlewareConfigProxy, MiddlewareConfiguration, NestMiddleware, ExpressMiddleware, MiddlewaresConsumer, OnModuleInit, ExceptionFilter, WebSocketAdapter, PipeTransform, Paramtype, ArgumentMetadata, OnModuleDestroy, CanActivate, RpcExceptionFilter, WsExceptionFilter } from './interfaces'; | ||
export { NestModule, INestApplication, INestMicroservice, MiddlewareConfigProxy, MiddlewareConfiguration, NestMiddleware, ExpressMiddleware, MiddlewaresConsumer, OnModuleInit, ExceptionFilter, WebSocketAdapter, PipeTransform, Paramtype, ArgumentMetadata, OnModuleDestroy, ExecutionContext, CanActivate, RpcExceptionFilter, WsExceptionFilter, NestInterceptor } from './interfaces'; | ||
export * from './services/logger.service'; |
import { Observable } from 'rxjs/Observable'; | ||
import { ExecutionContext } from './execution-context.interface'; | ||
export interface CanActivate { | ||
canActivate(request: any, controller: any, method: any): boolean | Promise<boolean> | Observable<boolean>; | ||
canActivate(request: any, context: ExecutionContext): boolean | Promise<boolean> | Observable<boolean>; | ||
} |
@@ -21,1 +21,3 @@ export * from './request-mapping-metadata.interface'; | ||
export * from './exceptions/ws-exception-filter.interface'; | ||
export * from './execution-context.interface'; | ||
export * from './nest-interceptor.interface'; |
import { MiddlewaresConsumer } from './middlewares-consumer.interface'; | ||
export interface MiddlewareConfigProxy { | ||
with: (...data) => MiddlewareConfigProxy; | ||
forRoutes: (...routes) => MiddlewaresConsumer; | ||
/** | ||
* Passes custom arguments to `resolve()` method of the middleware | ||
* | ||
* @param {} ...data | ||
* @returns MiddlewareConfigProxy | ||
*/ | ||
with(...data: any[]): MiddlewareConfigProxy; | ||
/** | ||
* Attaches passed routes / controllers to the processed middleware(s). | ||
* Single route can be defined as a literal object: | ||
* ``` | ||
* path: string; | ||
* method: RequestMethod; | ||
* ``` | ||
* | ||
* When you passed Controller class, Nest will attach middleware to every HTTP route handler inside this controller. | ||
* | ||
* @param {} ...routes | ||
* @returns MiddlewaresConsumer | ||
*/ | ||
forRoutes(...routes: any[]): MiddlewaresConsumer; | ||
} |
import { MiddlewareConfigProxy } from './middleware-config-proxy.interface'; | ||
export interface MiddlewaresConsumer { | ||
/** | ||
* Takes single middleware class or array of classes, | ||
* which subsequently can be attached to the passed routes / controllers. | ||
* | ||
* @param {any|any[]} middlewares | ||
* @returns MiddlewareConfigProxy | ||
*/ | ||
apply(middlewares: any | any[]): MiddlewareConfigProxy; | ||
} |
import { MiddlewaresConsumer } from '../middlewares/middlewares-consumer.interface'; | ||
export interface NestModule { | ||
configure?(consumer: MiddlewaresConsumer): MiddlewaresConsumer | void; | ||
configure(consumer: MiddlewaresConsumer): MiddlewaresConsumer | void; | ||
} |
@@ -5,12 +5,90 @@ import { MicroserviceConfiguration } from '@nestjs/microservices'; | ||
export interface INestApplication { | ||
/** | ||
* Initializes application. It is not necessary to call this method directly. | ||
* | ||
* @returns Promise | ||
*/ | ||
init(): Promise<void>; | ||
/** | ||
* The wrapper function around native `express.use()` method. | ||
* Example `app.use(bodyParser.json())` | ||
* | ||
* @param {} requestHandler Express Request Handler | ||
* @returns void | ||
*/ | ||
use(requestHandler: any): void; | ||
/** | ||
* Starts the application. | ||
* | ||
* @param {number} port | ||
* @param {Function} callback Optional callback | ||
* @returns Promise | ||
*/ | ||
listen(port: number, callback?: () => void): Promise<any>; | ||
/** | ||
* Starts the application and can be awaited. | ||
* | ||
* @param {number} port | ||
* @returns Promise | ||
*/ | ||
listenAsync(port: number): Promise<any>; | ||
/** | ||
* Setups the prefix for the every HTTP route path | ||
* | ||
* @param {string} prefix The prefix for the every HTTP route path (for example `/v1/api`) | ||
* @returns void | ||
*/ | ||
setGlobalPrefix(prefix: string): void; | ||
/** | ||
* Setup Web Sockets Adapter, which will be used inside Gateways. | ||
* Use, when you want to override default `socket.io` library. | ||
* | ||
* @param {WebSocketAdapter} adapter | ||
* @returns void | ||
*/ | ||
useWebSocketAdapter(adapter: WebSocketAdapter): void; | ||
/** | ||
* Connects microservice to the NestApplication instance. It transforms application to the hybrid instance. | ||
* | ||
* @param {MicroserviceConfiguration} config Microservice configuration objet | ||
* @returns INestMicroservice | ||
*/ | ||
connectMicroservice(config: MicroserviceConfiguration): INestMicroservice; | ||
/** | ||
* Returns array of the connected microservices to the NestApplication. | ||
* | ||
* @returns INestMicroservice[] | ||
*/ | ||
getMicroservices(): INestMicroservice[]; | ||
startAllMicroservices(callback: () => void): void; | ||
/** | ||
* Starts all the connected microservices asynchronously | ||
* | ||
* @param {Function} callback Optional callback function | ||
* @returns void | ||
*/ | ||
startAllMicroservices(callback?: () => void): void; | ||
/** | ||
* Starts all the connected microservices and can be awaited | ||
* | ||
* @returns Promise | ||
*/ | ||
startAllMicroservicesAsync(): Promise<void>; | ||
/** | ||
* Setups exception filters as a global filters (will be used inside every HTTP route path) | ||
* | ||
* @param {ExceptionFilter[]} ...filters | ||
*/ | ||
useGlobalFilters(...filters: ExceptionFilter[]): any; | ||
useGlobalPipes(...pipes: PipeTransform[]): any; | ||
/** | ||
* Setups pipes as a global pipes (will be used inside every HTTP route path) | ||
* | ||
* @param {PipeTransform[]} ...pipes | ||
*/ | ||
useGlobalPipes(...pipes: PipeTransform<any>[]): any; | ||
/** | ||
* Terminates the application (both NestApplication, Web Socket Gateways and every connected microservice) | ||
* | ||
* @returns void | ||
*/ | ||
close(): void; | ||
} |
import { WebSocketAdapter } from '@nestjs/common'; | ||
export interface INestMicroservice { | ||
/** | ||
* Starts the microservice. | ||
* | ||
* @param {Function} callback Callback called after instant | ||
* @returns Promise | ||
*/ | ||
listen(callback: () => void): any; | ||
/** | ||
* Setup Web Sockets Adapter, which will be used inside Gateways. | ||
* Use, when you want to override default `socket.io` library. | ||
* | ||
* @param {WebSocketAdapter} adapter | ||
* @returns void | ||
*/ | ||
useWebSocketAdapter(adapter: WebSocketAdapter): void; | ||
/** | ||
* Terminates the application (both NestMicroservice and every Web Socket Gateway) | ||
* | ||
* @returns void | ||
*/ | ||
close(): void; | ||
} |
@@ -5,7 +5,7 @@ import { Paramtype } from './paramtype.interface'; | ||
type: Paramtype; | ||
metatype?: any; | ||
data?: any; | ||
metatype?: new (...args) => any; | ||
data?: string; | ||
} | ||
export interface PipeTransform { | ||
transform: Transform<any>; | ||
export interface PipeTransform<T> { | ||
transform(value: T, metadata: ArgumentMetadata): any; | ||
} |
@@ -7,4 +7,4 @@ import { MessageMappingProperties } from '@nestjs/websockets/gateway-metadata-explorer'; | ||
bindClientDisconnect?(client: any, callback: (...args) => void): any; | ||
bindMessageHandlers(client: any, handlers: MessageMappingProperties[]): any; | ||
bindMessageHandler(client: any, handler: MessageMappingProperties, process: (data: any) => Promise<any>): any; | ||
bindMiddleware?(server: any, middleware: (socket, next) => void): any; | ||
} |
{ | ||
"name": "@nestjs/common", | ||
"version": "3.0.3", | ||
"version": "3.1.0", | ||
"description": "Nest - modern, fast, powerful node.js web framework (@common)", | ||
"author": "Kamil Mysliwiec", | ||
"license": "MIT", | ||
"scripts": { | ||
"compile": "tsc -p tsconfig.json" | ||
}, | ||
"dependencies": { | ||
@@ -8,0 +11,0 @@ "cli-color": "1.1.0" |
104
Readme.md
@@ -1,23 +0,56 @@ | ||
[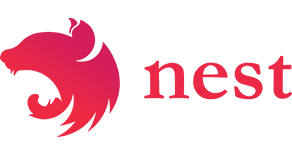](http://kamilmysliwiec.com/) | ||
<p align="center"> | ||
<a href="http://nestjs.com/" target="blank"><img src="http://kamilmysliwiec.com/public/nest-logo.png" alt="Nest Logo" /></a> | ||
</p> | ||
Modern, powerful web application framework for [Node.js](http://nodejs.org). | ||
[travis-image]: https://api.travis-ci.org/kamilmysliwiec/nest.svg?branch=master | ||
[travis-url]: https://travis-ci.org/kamilmysliwiec/nest | ||
[linux-image]: https://img.shields.io/travis/kamilmysliwiec/nest/master.svg?label=linux | ||
[linux-url]: https://travis-ci.org/kamilmysliwiec/nest | ||
<p align="center">Modern, powerful web application framework for <a href="http://nodejs.org" target="blank">Node.js</a>.</p> | ||
<p align="center"> | ||
<a href="https://www.npmjs.com/~nestjscore"><img src="https://img.shields.io/npm/v/@nestjs/core.svg" alt="NPM Version" /></a> | ||
<a href="https://www.npmjs.com/~nestjscore"><img src="https://img.shields.io/npm/l/@nestjs/core.svg" alt="Package License" /></a> | ||
<a href="https://www.npmjs.com/~nestjscore"><img src="https://img.shields.io/npm/dm/@nestjs/core.svg" alt="NPM Downloads" /></a> | ||
<a href="https://travis-ci.org/kamilmysliwiec/nest"><img src="https://api.travis-ci.org/kamilmysliwiec/nest.svg?branch=master" alt="Travis" /></a> | ||
<a href="https://travis-ci.org/kamilmysliwiec/nest"><img src="https://img.shields.io/travis/kamilmysliwiec/nest/master.svg?label=linux" alt="Linux" /></a> | ||
<a href="https://coveralls.io/github/kamilmysliwiec/nest?branch=master"><img src="https://coveralls.io/repos/github/kamilmysliwiec/nest/badge.svg?branch=master" alt="Coverage Status" /></a> | ||
<a href="https://gitter.im/nestjs/nestjs?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=body_badge"><img src="https://badges.gitter.im/nestjs/nestjs.svg" alt="Gitter" /></a> | ||
<a href="https://opencollective.com/nest#backer"><img src="https://opencollective.com/nest/backers/badge.svg" alt="Backers on Open Collective" /></a> | ||
<a href="https://opencollective.com/nest#sponsor"><img src="https://opencollective.com/nest/sponsors/badge.svg" alt="Sponsors on Open Collective" /></a> | ||
</p> | ||
<!--[](https://opencollective.com/nest#backer) | ||
[](https://opencollective.com/nest#sponsor)--> | ||
[![NPM Version][npm-image]][npm-url] | ||
[![NPM Downloads][downloads-image]][downloads-url] | ||
[![Travis][travis-image]][travis-url] | ||
[![Linux][linux-image]][linux-url] | ||
[](https://coveralls.io/github/kamilmysliwiec/nest?branch=master) | ||
[](https://opencollective.com/nest#backer) | ||
[](https://opencollective.com/nest#sponsor) | ||
[](https://gitter.im/nestjs/nestjs?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=body_badge) | ||
## Description | ||
Nest is a powerful web framework for [Node.js](http://nodejs.org), which helps you effortlessly build efficient, scalable applications. It uses modern JavaScript, is built with [TypeScript](http://www.typescriptlang.org) and combines best concepts of both OOP (Object Oriented Progamming) and FP (Functional Programming). | ||
<p>Nest is a framework for building efficient, scalable <a href="http://nodejs.org" target="_blank">Node.js</a> web applications. It uses modern JavaScript, is built with <a href="http://www.typescriptlang.org" target="_blank">TypeScript</a> and combines elements of OOP (Object Oriented Progamming), FP (Functional Programming), and FRP (Functional Reactive Programming).</p> | ||
<p>Under the hood, Nest makes use of <a href="https://expressjs.com/" target="_blank">Express</a>, allowing for easy use of the myriad third-party plugins which are available.</p> | ||
It is not another framework. You don't have to wait for a large community, because Nest is built with awesome, popular well-known libraries - [Express](https://github.com/expressjs/express) and [socket.io](https://github.com/socketio/socket.io) (you can use any other library if you want to)! It means, that you could quickly start using framework without worrying about a third party plugins. | ||
## Philosophy | ||
<p>In recent years, thanks to Node.js, JavaScript has become the “lingua franca” of the web for both front and backend applications, giving rise to awesome projects like <a href="https://angular.io/" target="_blank">Angular</a>, <a href="https://github.com/facebook/react" target="_blank">React</a> and <a href="https://github.com/vuejs/vue" target="_blank">Vue</a> which improve developer productivity and enable the construction of fast, testable, extensible frontend applications. However, on the server-side, while there are a lot of superb libraries, helpers and tools for Node, none of them effectively solve the main problem - the architecture.</p> | ||
<p>Nest aims to provide an application architecture out of the box which allows for effortless creation of highly testable, scalable, loosely coupled and easily maintainable applications.</p> | ||
## Features | ||
<ul> | ||
<li>Built with <a href="http://www.typescriptlang.org" target="_blank">TypeScript</a></li> | ||
<li><strong>Easy</strong> to learn - syntax similar to <a href="https://angular.io/" target="_blank">Angular</a></li> | ||
<li><strong>Familiar</strong> - based on well-known libraries (<a href="https://github.com/expressjs/express" target="_blank">Express</a> / <a href="https://github.com/socketio/socket.io" target="_blank">socket.io</a>)</li> | ||
<li><strong>Dependency Injection</strong> - built-in asynchronous <strong>IoC</strong> container with a <strong>hierarchical injector</strong></li> | ||
<li><strong>WebSockets</strong> module (based on <a href="https://github.com/socketio/socket.io" target="_blank">socket.io</a>, but you can bring your own library, by making use of <code>WsAdapter</code>)</li> | ||
<li><strong>Modular</strong> - defines an easy to follow module definition pattern so you can split your system into reusable modules</li> | ||
<li><strong>Reactive microservice</strong> support with message patterns (built-in transport via TCP / <a href="https://redis.io/" target="_blank">Redis</a>, but other communication schemes can be implemented with <code>CustomTransportStrategy</code>)</li> | ||
<li><strong>Exception layer</strong> - throwable web exceptions with status codes, exception filters</li> | ||
<li><strong>Pipes</strong> - synchronous & asynchronous (e.g. validation purposes)</li> | ||
<li><strong>Guards</strong> - attach additional logic in a declarative manner (e.g. role-based access control)</li> | ||
<li><strong>Interceptors</strong> - built on top of <a href="https://github.com/reactivex/rxjs" target="blank">RxJS</a></li> | ||
<li>Testing utilities (both <strong>e2e & unit</strong> tests)</li> | ||
</ul> | ||
## Installation | ||
**Git:** | ||
**Install the TypeScript Starter Project with Git:** | ||
```bash | ||
@@ -30,3 +63,3 @@ $ git clone https://github.com/kamilmysliwiec/nest-typescript-starter.git project | ||
**NPM:** | ||
**Start a New Project from Scratch with NPM:** | ||
```bash | ||
@@ -36,23 +69,2 @@ $ npm i --save @nestjs/core @nestjs/common @nestjs/microservices @nestjs/websockets @nestjs/testing reflect-metadata rxjs | ||
## Philosophy | ||
JavaScript is awesome. This language is no longer just a trash to create simple animations in the browser. Now, the front end world is rich in variety of tools. We have a lot of amazing frameworks / libraries such as [Angular](https://angular.io/), [React](https://github.com/facebook/react) or [Vue](https://github.com/vuejs/vue), which improves our development process and makes our applications fast and flexible. | ||
[Node.js](http://nodejs.org) gave us a possibility to use this language also on the server side. There are a lot of superb libraries, helpers and tools for node, but non of them do not solve the main problem - the architecture. | ||
We want to create scalable, loosely coupled and easy to maintain applications. Let's show the entire world node.js potential together! | ||
## Features | ||
- Easy to learn - syntax is similar to [Angular](https://angular.io/) | ||
- Built on top of TypeScript, but also compatible with plain ES6 (I strongly recommend to use [TypeScript](http://www.typescriptlang.org)) | ||
- Based on well-known libraries ([Express](https://github.com/expressjs/express) / [socket.io](https://github.com/socketio/socket.io)) so you could share your experience | ||
- Supremely useful Dependency Injection, built-in **Inversion of Control** container | ||
- **Hierarchical injector** - increase abstraction in your application by creating reusable, loosely coupled modules with type injection | ||
- **WebSockets** module (based on [socket.io](https://github.com/socketio/socket.io), although you can use any other library using adapter) | ||
- Own modularity system (split your system into reusable modules) | ||
- Reactive **microservices** support with messages patterns (built-in transport via TCP / [Redis](https://redis.io/), but you can use any other type of communication using `CustomTransportStrategy`) | ||
- Exceptions handler layer, exception filters, **sync & async pipes** layer | ||
- Testing utilities | ||
## Documentation & Quick Start | ||
@@ -62,13 +74,2 @@ | ||
## Starter repos | ||
- [TypeScript](https://github.com/kamilmysliwiec/nest-typescript-starter) | ||
- [Babel](https://github.com/kamilmysliwiec/nest-babel-starter/) | ||
## Useful references | ||
- [Modules](http://docs.nestjs.com/modules.html) | ||
- [Examples](http://docs.nestjs.com/examples.html) | ||
- [API Reference](http://docs.nestjs.com/api-reference.html) | ||
## People | ||
@@ -117,10 +118,1 @@ | ||
[MIT](LICENSE) | ||
[npm-image]: https://badge.fury.io/js/%40nestjs%2Fcore.svg | ||
[npm-url]: https://www.npmjs.com/~nestjscore | ||
[downloads-image]: https://img.shields.io/npm/dm/@nestjs/core.svg | ||
[downloads-url]: https://www.npmjs.com/~nestjscore | ||
[travis-image]: https://api.travis-ci.org/kamilmysliwiec/nest.svg?branch=master | ||
[travis-url]: https://travis-ci.org/kamilmysliwiec/nest | ||
[linux-image]: https://img.shields.io/travis/kamilmysliwiec/nest/master.svg?label=linux | ||
[linux-url]: https://travis-ci.org/kamilmysliwiec/nest |
@@ -33,3 +33,3 @@ "use strict"; | ||
printStackTrace(trace) { | ||
if (Logger.mode === nest_environment_enum_1.NestEnvironment.TEST) | ||
if (Logger.mode === nest_environment_enum_1.NestEnvironment.TEST || !trace) | ||
return; | ||
@@ -36,0 +36,0 @@ process.stdout.write(trace); |
import 'reflect-metadata'; | ||
/** | ||
* Defines the Exceptions Filter. Takes set of exception types as an argument, which has to be catched by this Filter. | ||
* The class should implements the `ExceptionFilter` interface. | ||
*/ | ||
export declare const Catch: (...exceptions: any[]) => ClassDecorator; |
@@ -5,2 +5,6 @@ "use strict"; | ||
const constants_1 = require("../../constants"); | ||
/** | ||
* Defines the Exceptions Filter. Takes set of exception types as an argument, which has to be catched by this Filter. | ||
* The class should implements the `ExceptionFilter` interface. | ||
*/ | ||
exports.Catch = (...exceptions) => { | ||
@@ -7,0 +11,0 @@ return (target) => { |
@@ -0,1 +1,17 @@ | ||
/** | ||
* Defines the Component. The component can inject dependencies through constructor. | ||
* Those dependencies should belongs to the same module. | ||
*/ | ||
export declare const Component: () => ClassDecorator; | ||
/** | ||
* Defines the Pipe. The Pipe should implements the `PipeTransform` interface. | ||
*/ | ||
export declare const Pipe: () => ClassDecorator; | ||
/** | ||
* Defines the Guard. The Guard should implement the `CanActivate` interface. | ||
*/ | ||
export declare const Guard: () => ClassDecorator; | ||
/** | ||
* Defines the Middleware. The Middleware should implement the `NestMiddleware` interface. | ||
*/ | ||
export declare const Middleware: () => ClassDecorator; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
/** | ||
* Defines the Component. The component can inject dependencies through constructor. | ||
* Those dependencies should belongs to the same module. | ||
*/ | ||
exports.Component = () => { | ||
return (target) => { }; | ||
}; | ||
/** | ||
* Defines the Pipe. The Pipe should implements the `PipeTransform` interface. | ||
*/ | ||
exports.Pipe = () => { | ||
return (target) => { }; | ||
}; | ||
/** | ||
* Defines the Guard. The Guard should implement the `CanActivate` interface. | ||
*/ | ||
exports.Guard = () => { | ||
return (target) => { }; | ||
}; | ||
/** | ||
* Defines the Middleware. The Middleware should implement the `NestMiddleware` interface. | ||
*/ | ||
exports.Middleware = () => { | ||
return (target) => { }; | ||
}; |
import 'reflect-metadata'; | ||
import { ControllerMetadata } from '../../interfaces/controllers/controller-metadata.interface'; | ||
export declare const Controller: (metadata?: string | ControllerMetadata) => ClassDecorator; | ||
/** | ||
* Defines the Controller. The controller can inject dependencies through constructor. | ||
* Those dependencies should belongs to the same module. | ||
*/ | ||
export declare const Controller: (prefix?: string) => ClassDecorator; |
@@ -6,5 +6,8 @@ "use strict"; | ||
const constants_1 = require("../../constants"); | ||
exports.Controller = (metadata) => { | ||
let path = shared_utils_1.isObject(metadata) ? metadata[constants_1.PATH_METADATA] : metadata; | ||
path = shared_utils_1.isUndefined(path) ? '/' : path; | ||
/** | ||
* Defines the Controller. The controller can inject dependencies through constructor. | ||
* Those dependencies should belongs to the same module. | ||
*/ | ||
exports.Controller = (prefix) => { | ||
const path = shared_utils_1.isUndefined(prefix) ? '/' : prefix; | ||
return (target) => { | ||
@@ -11,0 +14,0 @@ Reflect.defineMetadata(constants_1.PATH_METADATA, path, target); |
@@ -8,2 +8,12 @@ import 'reflect-metadata'; | ||
export declare const ExceptionFilters: (...filters: ExceptionFilter[]) => (target: object, key?: any, descriptor?: any) => any; | ||
/** | ||
* Setups exception filters to the chosen context. | ||
* When the `@UseFilters()` is used on the controller level: | ||
* - Exception Filter will be setuped to the every handler (every method) | ||
* | ||
* When the `@UseFilters()` is used on the handle level: | ||
* - Exception Filter will be setuped only to specified method | ||
* | ||
* @param {ExceptionFilter[]} ...filters (instances) | ||
*/ | ||
export declare const UseFilters: (...filters: ExceptionFilter[]) => (target: object, key?: any, descriptor?: any) => any; |
@@ -25,2 +25,12 @@ "use strict"; | ||
}; | ||
/** | ||
* Setups exception filters to the chosen context. | ||
* When the `@UseFilters()` is used on the controller level: | ||
* - Exception Filter will be setuped to the every handler (every method) | ||
* | ||
* When the `@UseFilters()` is used on the handle level: | ||
* - Exception Filter will be setuped only to specified method | ||
* | ||
* @param {ExceptionFilter[]} ...filters (instances) | ||
*/ | ||
exports.UseFilters = (...filters) => defineFiltersMetadata(...filters); |
import 'reflect-metadata'; | ||
export declare const Inject: (param: any) => ParameterDecorator; | ||
/** | ||
* Injects component, which has to be available in the current injector (module) scope. | ||
* Components are recognized by types / or tokens. | ||
*/ | ||
export declare const Inject: (token: any) => ParameterDecorator; |
@@ -6,6 +6,10 @@ "use strict"; | ||
const shared_utils_1 = require("../shared.utils"); | ||
exports.Inject = (param) => { | ||
/** | ||
* Injects component, which has to be available in the current injector (module) scope. | ||
* Components are recognized by types / or tokens. | ||
*/ | ||
exports.Inject = (token) => { | ||
return (target, key, index) => { | ||
const args = Reflect.getMetadata(constants_1.SELF_DECLARED_DEPS_METADATA, target) || []; | ||
const type = shared_utils_1.isFunction(param) ? param.name : param; | ||
const type = shared_utils_1.isFunction(token) ? token.name : token; | ||
args.push({ index, param: type }); | ||
@@ -12,0 +16,0 @@ Reflect.defineMetadata(constants_1.SELF_DECLARED_DEPS_METADATA, args, target); |
import 'reflect-metadata'; | ||
import { ModuleMetadata } from '../../interfaces/modules/module-metadata.interface'; | ||
export declare const Module: (props: ModuleMetadata) => ClassDecorator; | ||
/** | ||
* Defines the module | ||
* - `modules` - the set of the 'imported' modules | ||
* - `controllers` - the list of controllers (e.g. HTTP controllers) | ||
* - `components` - the list of components that belong to this module. They can be injected between themselves. | ||
* - `exports` - the set of components, which should be available for modules, which imports this module | ||
* @param obj {ModuleMetadata} Module metadata | ||
*/ | ||
export declare const Module: (obj: { | ||
modules?: any[]; | ||
controllers?: any[]; | ||
components?: any[]; | ||
exports?: any[]; | ||
}) => ClassDecorator; |
@@ -21,9 +21,17 @@ "use strict"; | ||
}; | ||
exports.Module = (props) => { | ||
const propsKeys = Object.keys(props); | ||
/** | ||
* Defines the module | ||
* - `modules` - the set of the 'imported' modules | ||
* - `controllers` - the list of controllers (e.g. HTTP controllers) | ||
* - `components` - the list of components that belong to this module. They can be injected between themselves. | ||
* - `exports` - the set of components, which should be available for modules, which imports this module | ||
* @param obj {ModuleMetadata} Module metadata | ||
*/ | ||
exports.Module = (obj) => { | ||
const propsKeys = Object.keys(obj); | ||
validateKeys(propsKeys); | ||
return (target) => { | ||
for (const property in props) { | ||
if (props.hasOwnProperty(property)) { | ||
Reflect.defineMetadata(property, props[property], target); | ||
for (const property in obj) { | ||
if (obj.hasOwnProperty(property)) { | ||
Reflect.defineMetadata(property, obj[property], target); | ||
} | ||
@@ -30,0 +38,0 @@ } |
import 'reflect-metadata'; | ||
import { RequestMappingMetadata } from '../../interfaces/request-mapping-metadata.interface'; | ||
export declare const RequestMapping: (metadata?: RequestMappingMetadata) => MethodDecorator; | ||
/** | ||
* Routes HTTP POST requests to the specified path. | ||
*/ | ||
export declare const Post: (path?: string) => MethodDecorator; | ||
/** | ||
* Routes HTTP GET requests to the specified path. | ||
*/ | ||
export declare const Get: (path?: string) => MethodDecorator; | ||
/** | ||
* Routes HTTP DELETE requests to the specified path. | ||
*/ | ||
export declare const Delete: (path?: string) => MethodDecorator; | ||
/** | ||
* Routes HTTP PUT requests to the specified path. | ||
*/ | ||
export declare const Put: (path?: string) => MethodDecorator; | ||
/** | ||
* Routes HTTP PATCH requests to the specified path. | ||
*/ | ||
export declare const Patch: (path?: string) => MethodDecorator; | ||
/** | ||
* Routes all HTTP requests to the specified path. | ||
*/ | ||
export declare const All: (path?: string) => MethodDecorator; |
@@ -25,7 +25,25 @@ "use strict"; | ||
}; | ||
/** | ||
* Routes HTTP POST requests to the specified path. | ||
*/ | ||
exports.Post = createMappingDecorator(request_method_enum_1.RequestMethod.POST); | ||
/** | ||
* Routes HTTP GET requests to the specified path. | ||
*/ | ||
exports.Get = createMappingDecorator(request_method_enum_1.RequestMethod.GET); | ||
/** | ||
* Routes HTTP DELETE requests to the specified path. | ||
*/ | ||
exports.Delete = createMappingDecorator(request_method_enum_1.RequestMethod.DELETE); | ||
/** | ||
* Routes HTTP PUT requests to the specified path. | ||
*/ | ||
exports.Put = createMappingDecorator(request_method_enum_1.RequestMethod.PUT); | ||
/** | ||
* Routes HTTP PATCH requests to the specified path. | ||
*/ | ||
exports.Patch = createMappingDecorator(request_method_enum_1.RequestMethod.PATCH); | ||
/** | ||
* Routes all HTTP requests to the specified path. | ||
*/ | ||
exports.All = createMappingDecorator(request_method_enum_1.RequestMethod.ALL); |
@@ -15,6 +15,6 @@ import 'reflect-metadata'; | ||
export declare const Headers: (property?: string) => ParameterDecorator; | ||
export declare const Query: (property?: string, ...pipes: PipeTransform[]) => ParameterDecorator; | ||
export declare const Body: (property?: string, ...pipes: PipeTransform[]) => ParameterDecorator; | ||
export declare const Param: (property?: string, ...pipes: PipeTransform[]) => ParameterDecorator; | ||
export declare const Query: (property?: string, ...pipes: PipeTransform<any>[]) => ParameterDecorator; | ||
export declare const Body: (property?: string, ...pipes: PipeTransform<any>[]) => ParameterDecorator; | ||
export declare const Param: (property?: string, ...pipes: PipeTransform<any>[]) => ParameterDecorator; | ||
export declare const Req: () => ParameterDecorator; | ||
export declare const Res: () => ParameterDecorator; |
import 'reflect-metadata'; | ||
export declare const Shared: (token?: string) => (target: any) => any; | ||
/** | ||
* Specifies scope of this module. When module is `@Shared()`, Nest will create only one instance of this | ||
* module and share them between all of the modules. | ||
* @deprecated | ||
*/ | ||
export declare const Shared: (scope?: string) => (target: any) => any; |
@@ -5,3 +5,11 @@ "use strict"; | ||
const constants_1 = require("../../constants"); | ||
exports.Shared = (token = 'global') => { | ||
const index_1 = require("../../index"); | ||
/** | ||
* Specifies scope of this module. When module is `@Shared()`, Nest will create only one instance of this | ||
* module and share them between all of the modules. | ||
* @deprecated | ||
*/ | ||
exports.Shared = (scope = 'global') => { | ||
const logger = new index_1.Logger('Shared'); | ||
logger.warn('DEPRECATED! Since version 4.0.0 `@Shared()` decorator is deprecated. All modules are singletons now.'); | ||
return (target) => { | ||
@@ -14,3 +22,3 @@ const Metatype = target; | ||
}; | ||
Reflect.defineMetadata(constants_1.SHARED_MODULE_METADATA, token, Type); | ||
Reflect.defineMetadata(constants_1.SHARED_MODULE_METADATA, scope, Type); | ||
Object.defineProperty(Type, 'name', { value: target.name }); | ||
@@ -17,0 +25,0 @@ return Type; |
@@ -0,1 +1,11 @@ | ||
/** | ||
* Setups guards to the chosen context. | ||
* When the `@UseGuards()` is used on the controller level: | ||
* - Guard will be setuped to the every handler (every method) | ||
* | ||
* When the `@UseGuards()` is used on the handle level: | ||
* - Guard will be setuped only to specified method | ||
* | ||
* @param {} ...guards (types) | ||
*/ | ||
export declare const UseGuards: (...guards: any[]) => (target: object, key?: any, descriptor?: any) => any; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
const constants_1 = require("../../constants"); | ||
/** | ||
* Setups guards to the chosen context. | ||
* When the `@UseGuards()` is used on the controller level: | ||
* - Guard will be setuped to the every handler (every method) | ||
* | ||
* When the `@UseGuards()` is used on the handle level: | ||
* - Guard will be setuped only to specified method | ||
* | ||
* @param {} ...guards (types) | ||
*/ | ||
exports.UseGuards = (...guards) => { | ||
@@ -5,0 +15,0 @@ return (target, key, descriptor) => { |
import { PipeTransform } from '../../interfaces/index'; | ||
export declare const UsePipes: (...pipes: PipeTransform[]) => (target: object, key?: any, descriptor?: any) => any; | ||
/** | ||
* Setups pipes to the chosen context. | ||
* When the `@UsePipes()` is used on the controller level: | ||
* - Pipe will be setuped to the every handler (every method) | ||
* | ||
* When the `@UsePipes()` is used on the handle level: | ||
* - Pipe will be setuped only to specified method | ||
* | ||
* @param {PipeTransform[]} ...pipes (instances) | ||
*/ | ||
export declare const UsePipes: (...pipes: PipeTransform<any>[]) => (target: object, key?: any, descriptor?: any) => any; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
const constants_1 = require("../../constants"); | ||
/** | ||
* Setups pipes to the chosen context. | ||
* When the `@UsePipes()` is used on the controller level: | ||
* - Pipe will be setuped to the every handler (every method) | ||
* | ||
* When the `@UsePipes()` is used on the handle level: | ||
* - Pipe will be setuped only to specified method | ||
* | ||
* @param {PipeTransform[]} ...pipes (instances) | ||
*/ | ||
exports.UsePipes = (...pipes) => { | ||
@@ -5,0 +15,0 @@ return (target, key, descriptor) => { |
@@ -11,6 +11,10 @@ export * from './decorators/request-mapping.decorator'; | ||
export * from './decorators/shared.decorator'; | ||
export * from './decorators/single-scope.decorator'; | ||
export * from './decorators/use-pipes.decorator'; | ||
export * from './decorators/use-guards.decorator'; | ||
export { Component as Middleware } from './decorators/component.decorator'; | ||
export { Component as Pipe } from './decorators/component.decorator'; | ||
export { Component as Guard } from './decorators/component.decorator'; | ||
export * from './decorators/component.decorator'; | ||
export * from './decorators/component.decorator'; | ||
export * from './decorators/component.decorator'; | ||
export * from './decorators/reflect-metadata.decorator'; | ||
export * from './decorators/interceptor.decorator'; | ||
export * from './decorators/use-interceptors.decorator'; |
@@ -16,9 +16,10 @@ "use strict"; | ||
__export(require("./decorators/shared.decorator")); | ||
__export(require("./decorators/single-scope.decorator")); | ||
__export(require("./decorators/use-pipes.decorator")); | ||
__export(require("./decorators/use-guards.decorator")); | ||
var component_decorator_1 = require("./decorators/component.decorator"); | ||
exports.Middleware = component_decorator_1.Component; | ||
var component_decorator_2 = require("./decorators/component.decorator"); | ||
exports.Pipe = component_decorator_2.Component; | ||
var component_decorator_3 = require("./decorators/component.decorator"); | ||
exports.Guard = component_decorator_3.Component; | ||
__export(require("./decorators/component.decorator")); | ||
__export(require("./decorators/component.decorator")); | ||
__export(require("./decorators/component.decorator")); | ||
__export(require("./decorators/reflect-metadata.decorator")); | ||
__export(require("./decorators/interceptor.decorator")); | ||
__export(require("./decorators/use-interceptors.decorator")); |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
66825
147
1364
115