@nextgis/dom
Advanced tools
Comparing version 1.19.0 to 2.0.0-alpha.0
@@ -1,77 +0,62 @@ | ||
/** Bundle of @nextgis/dom; version: 1.19.0; author: NextGIS */ | ||
/** Bundle of @nextgis/dom; version: 2.0.0-alpha.0; author: NextGIS */ | ||
function create(tagName, className, container) { | ||
const el = window.document.createElement(tagName); | ||
if (className !== undefined && className !== null) { | ||
el.className = className; | ||
} | ||
if (container) { | ||
container.appendChild(el); | ||
} | ||
return el; | ||
const el = window.document.createElement(tagName); | ||
if (className !== void 0 && className !== null) { | ||
el.className = className; | ||
} | ||
if (container) { | ||
container.appendChild(el); | ||
} | ||
return el; | ||
} | ||
/** | ||
* Helper function for determining the HTML element through the transmitted parameters | ||
* | ||
* @example | ||
* ```javascript | ||
* let el = getElement(HTMLElement || 'element-id'); | ||
* el = getElement('#element-id .sub-class'); | ||
* el = getElement('.element-class'); | ||
* ``` | ||
*/ | ||
function getElement(el) { | ||
if (typeof el === 'string') { | ||
let el_ = document.getElementById(el); | ||
if (!el_) { | ||
try { | ||
el_ = document.querySelector(el); | ||
} | ||
catch { | ||
// ignore | ||
} | ||
} | ||
return el_ || undefined; | ||
if (typeof el === "string") { | ||
let el_ = document.getElementById(el); | ||
if (!el_) { | ||
try { | ||
el_ = document.querySelector(el); | ||
} catch (e) { | ||
} | ||
} | ||
return el; | ||
return el_ || void 0; | ||
} | ||
return el; | ||
} | ||
function remove(element) { | ||
const el = getElement(element); | ||
if (el) { | ||
const parent = el.parentElement; | ||
if (parent) { | ||
parent.removeChild(el); | ||
} | ||
const el = getElement(element); | ||
if (el) { | ||
const parent = el.parentElement; | ||
if (parent) { | ||
parent.removeChild(el); | ||
} | ||
} | ||
} | ||
function loadScript(src, options = {}) { | ||
return new Promise((resolve, reject) => { | ||
const s = document.createElement('script'); | ||
s.src = src; | ||
s.onload = resolve; | ||
s.onerror = reject; | ||
s.type = 'text/javascript'; | ||
if (options.crossOrigin) { | ||
s.setAttribute('crossorigin', options.crossOrigin); | ||
// s.crossOrigin = options.crossOrigin; | ||
} | ||
// s.setAttribute('SameSite', 'None'); | ||
// s.referrerPolicy = 'unsafe-url'; // 'same-origin'; | ||
if (options.async) { | ||
s.async = true; | ||
} | ||
if (options.defer) { | ||
s.defer = true; | ||
} | ||
if (options.id) { | ||
s.setAttribute('id', options.id); | ||
} | ||
if (options.data) { | ||
for (const k in options.data) { | ||
const dataKey = k.replace(/^data-/, '').replace(/_/g, '-'); | ||
s.setAttribute('data-' + dataKey, String(options.data[k])); | ||
} | ||
} | ||
document.head.appendChild(s); | ||
}); | ||
return new Promise((resolve, reject) => { | ||
const s = document.createElement("script"); | ||
s.src = src; | ||
s.onload = resolve; | ||
s.onerror = reject; | ||
s.type = "text/javascript"; | ||
if (options.crossOrigin) { | ||
s.setAttribute("crossorigin", options.crossOrigin); | ||
} | ||
if (options.async) { | ||
s.async = true; | ||
} | ||
if (options.defer) { | ||
s.defer = true; | ||
} | ||
if (options.id) { | ||
s.setAttribute("id", options.id); | ||
} | ||
if (options.data) { | ||
for (const k in options.data) { | ||
const dataKey = k.replace(/^data-/, "").replace(/_/g, "-"); | ||
s.setAttribute("data-" + dataKey, String(options.data[k])); | ||
} | ||
} | ||
document.head.appendChild(s); | ||
}); | ||
} | ||
@@ -78,0 +63,0 @@ |
@@ -1,2 +0,2 @@ | ||
function t(t,e,n){const r=window.document.createElement(t);return null!=e&&(r.className=e),n&&n.appendChild(r),r}function e(t){if("string"==typeof t){let e=document.getElementById(t);if(!e)try{e=document.querySelector(t)}catch{}return e||void 0}return t}function n(t){const n=e(t);if(n){const t=n.parentElement;t&&t.removeChild(n)}}function r(t,e={}){return new Promise(((n,r)=>{const c=document.createElement("script");if(c.src=t,c.onload=n,c.onerror=r,c.type="text/javascript",e.crossOrigin&&c.setAttribute("crossorigin",e.crossOrigin),e.async&&(c.async=!0),e.defer&&(c.defer=!0),e.id&&c.setAttribute("id",e.id),e.data)for(const t in e.data){const n=t.replace(/^data-/,"").replace(/_/g,"-");c.setAttribute("data-"+n,String(e.data[t]))}document.head.appendChild(c)}))}export{t as create,e as getElement,r as loadScript,n as remove}; | ||
function t(t,e,n){const r=window.document.createElement(t);return null!=e&&(r.className=e),n&&n.appendChild(r),r}function e(t){if("string"==typeof t){let n=document.getElementById(t);if(!n)try{n=document.querySelector(t)}catch(e){}return n||void 0}return t}function n(t){const n=e(t);if(n){const t=n.parentElement;t&&t.removeChild(n)}}function r(t,e={}){return new Promise(((n,r)=>{const c=document.createElement("script");if(c.src=t,c.onload=n,c.onerror=r,c.type="text/javascript",e.crossOrigin&&c.setAttribute("crossorigin",e.crossOrigin),e.async&&(c.async=!0),e.defer&&(c.defer=!0),e.id&&c.setAttribute("id",e.id),e.data)for(const t in e.data){const n=t.replace(/^data-/,"").replace(/_/g,"-");c.setAttribute("data-"+n,String(e.data[t]))}document.head.appendChild(c)}))}export{t as create,e as getElement,r as loadScript,n as remove}; | ||
//# sourceMappingURL=dom.esm-browser.prod.js.map |
@@ -1,77 +0,62 @@ | ||
/** Bundle of @nextgis/dom; version: 1.19.0; author: NextGIS */ | ||
/** Bundle of @nextgis/dom; version: 2.0.0-alpha.0; author: NextGIS */ | ||
function create(tagName, className, container) { | ||
const el = window.document.createElement(tagName); | ||
if (className !== undefined && className !== null) { | ||
el.className = className; | ||
} | ||
if (container) { | ||
container.appendChild(el); | ||
} | ||
return el; | ||
const el = window.document.createElement(tagName); | ||
if (className !== void 0 && className !== null) { | ||
el.className = className; | ||
} | ||
if (container) { | ||
container.appendChild(el); | ||
} | ||
return el; | ||
} | ||
/** | ||
* Helper function for determining the HTML element through the transmitted parameters | ||
* | ||
* @example | ||
* ```javascript | ||
* let el = getElement(HTMLElement || 'element-id'); | ||
* el = getElement('#element-id .sub-class'); | ||
* el = getElement('.element-class'); | ||
* ``` | ||
*/ | ||
function getElement(el) { | ||
if (typeof el === 'string') { | ||
let el_ = document.getElementById(el); | ||
if (!el_) { | ||
try { | ||
el_ = document.querySelector(el); | ||
} | ||
catch { | ||
// ignore | ||
} | ||
} | ||
return el_ || undefined; | ||
if (typeof el === "string") { | ||
let el_ = document.getElementById(el); | ||
if (!el_) { | ||
try { | ||
el_ = document.querySelector(el); | ||
} catch (e) { | ||
} | ||
} | ||
return el; | ||
return el_ || void 0; | ||
} | ||
return el; | ||
} | ||
function remove(element) { | ||
const el = getElement(element); | ||
if (el) { | ||
const parent = el.parentElement; | ||
if (parent) { | ||
parent.removeChild(el); | ||
} | ||
const el = getElement(element); | ||
if (el) { | ||
const parent = el.parentElement; | ||
if (parent) { | ||
parent.removeChild(el); | ||
} | ||
} | ||
} | ||
function loadScript(src, options = {}) { | ||
return new Promise((resolve, reject) => { | ||
const s = document.createElement('script'); | ||
s.src = src; | ||
s.onload = resolve; | ||
s.onerror = reject; | ||
s.type = 'text/javascript'; | ||
if (options.crossOrigin) { | ||
s.setAttribute('crossorigin', options.crossOrigin); | ||
// s.crossOrigin = options.crossOrigin; | ||
} | ||
// s.setAttribute('SameSite', 'None'); | ||
// s.referrerPolicy = 'unsafe-url'; // 'same-origin'; | ||
if (options.async) { | ||
s.async = true; | ||
} | ||
if (options.defer) { | ||
s.defer = true; | ||
} | ||
if (options.id) { | ||
s.setAttribute('id', options.id); | ||
} | ||
if (options.data) { | ||
for (const k in options.data) { | ||
const dataKey = k.replace(/^data-/, '').replace(/_/g, '-'); | ||
s.setAttribute('data-' + dataKey, String(options.data[k])); | ||
} | ||
} | ||
document.head.appendChild(s); | ||
}); | ||
return new Promise((resolve, reject) => { | ||
const s = document.createElement("script"); | ||
s.src = src; | ||
s.onload = resolve; | ||
s.onerror = reject; | ||
s.type = "text/javascript"; | ||
if (options.crossOrigin) { | ||
s.setAttribute("crossorigin", options.crossOrigin); | ||
} | ||
if (options.async) { | ||
s.async = true; | ||
} | ||
if (options.defer) { | ||
s.defer = true; | ||
} | ||
if (options.id) { | ||
s.setAttribute("id", options.id); | ||
} | ||
if (options.data) { | ||
for (const k in options.data) { | ||
const dataKey = k.replace(/^data-/, "").replace(/_/g, "-"); | ||
s.setAttribute("data-" + dataKey, String(options.data[k])); | ||
} | ||
} | ||
document.head.appendChild(s); | ||
}); | ||
} | ||
@@ -78,0 +63,0 @@ |
@@ -1,2 +0,2 @@ | ||
function t(t,e,n){const r=window.document.createElement(t);return null!=e&&(r.className=e),n&&n.appendChild(r),r}function e(t){if("string"==typeof t){let e=document.getElementById(t);if(!e)try{e=document.querySelector(t)}catch{}return e||void 0}return t}function n(t){const n=e(t);if(n){const t=n.parentElement;t&&t.removeChild(n)}}function r(t,e={}){return new Promise(((n,r)=>{const c=document.createElement("script");if(c.src=t,c.onload=n,c.onerror=r,c.type="text/javascript",e.crossOrigin&&c.setAttribute("crossorigin",e.crossOrigin),e.async&&(c.async=!0),e.defer&&(c.defer=!0),e.id&&c.setAttribute("id",e.id),e.data)for(const t in e.data){const n=t.replace(/^data-/,"").replace(/_/g,"-");c.setAttribute("data-"+n,String(e.data[t]))}document.head.appendChild(c)}))}export{t as create,e as getElement,r as loadScript,n as remove}; | ||
function t(t,e,n){const r=window.document.createElement(t);return null!=e&&(r.className=e),n&&n.appendChild(r),r}function e(t){if("string"==typeof t){let n=document.getElementById(t);if(!n)try{n=document.querySelector(t)}catch(e){}return n||void 0}return t}function n(t){const n=e(t);if(n){const t=n.parentElement;t&&t.removeChild(n)}}function r(t,e={}){return new Promise(((n,r)=>{const c=document.createElement("script");if(c.src=t,c.onload=n,c.onerror=r,c.type="text/javascript",e.crossOrigin&&c.setAttribute("crossorigin",e.crossOrigin),e.async&&(c.async=!0),e.defer&&(c.defer=!0),e.id&&c.setAttribute("id",e.id),e.data)for(const t in e.data){const n=t.replace(/^data-/,"").replace(/_/g,"-");c.setAttribute("data-"+n,String(e.data[t]))}document.head.appendChild(c)}))}export{t as create,e as getElement,r as loadScript,n as remove}; | ||
//# sourceMappingURL=dom.esm-bundler.prod.js.map |
@@ -1,2 +0,2 @@ | ||
/** Bundle of @nextgis/dom; version: 1.19.0; author: NextGIS */ | ||
/** Bundle of @nextgis/dom; version: 2.0.0-alpha.0; author: NextGIS */ | ||
var Dom = (function (exports) { | ||
@@ -6,77 +6,61 @@ 'use strict'; | ||
function create(tagName, className, container) { | ||
var el = window.document.createElement(tagName); | ||
if (className !== undefined && className !== null) { | ||
el.className = className; | ||
} | ||
if (container) { | ||
container.appendChild(el); | ||
} | ||
return el; | ||
const el = window.document.createElement(tagName); | ||
if (className !== void 0 && className !== null) { | ||
el.className = className; | ||
} | ||
if (container) { | ||
container.appendChild(el); | ||
} | ||
return el; | ||
} | ||
/** | ||
* Helper function for determining the HTML element through the transmitted parameters | ||
* | ||
* @example | ||
* ```javascript | ||
* let el = getElement(HTMLElement || 'element-id'); | ||
* el = getElement('#element-id .sub-class'); | ||
* el = getElement('.element-class'); | ||
* ``` | ||
*/ | ||
function getElement(el) { | ||
if (typeof el === 'string') { | ||
var el_ = document.getElementById(el); | ||
if (!el_) { | ||
try { | ||
el_ = document.querySelector(el); | ||
} | ||
catch (_a) { | ||
// ignore | ||
} | ||
} | ||
return el_ || undefined; | ||
if (typeof el === "string") { | ||
let el_ = document.getElementById(el); | ||
if (!el_) { | ||
try { | ||
el_ = document.querySelector(el); | ||
} catch (e) { | ||
} | ||
} | ||
return el; | ||
return el_ || void 0; | ||
} | ||
return el; | ||
} | ||
function remove(element) { | ||
var el = getElement(element); | ||
if (el) { | ||
var parent_1 = el.parentElement; | ||
if (parent_1) { | ||
parent_1.removeChild(el); | ||
} | ||
const el = getElement(element); | ||
if (el) { | ||
const parent = el.parentElement; | ||
if (parent) { | ||
parent.removeChild(el); | ||
} | ||
} | ||
} | ||
function loadScript(src, options) { | ||
if (options === void 0) { options = {}; } | ||
return new Promise(function (resolve, reject) { | ||
var s = document.createElement('script'); | ||
s.src = src; | ||
s.onload = resolve; | ||
s.onerror = reject; | ||
s.type = 'text/javascript'; | ||
if (options.crossOrigin) { | ||
s.setAttribute('crossorigin', options.crossOrigin); | ||
// s.crossOrigin = options.crossOrigin; | ||
} | ||
// s.setAttribute('SameSite', 'None'); | ||
// s.referrerPolicy = 'unsafe-url'; // 'same-origin'; | ||
if (options.async) { | ||
s.async = true; | ||
} | ||
if (options.defer) { | ||
s.defer = true; | ||
} | ||
if (options.id) { | ||
s.setAttribute('id', options.id); | ||
} | ||
if (options.data) { | ||
for (var k in options.data) { | ||
var dataKey = k.replace(/^data-/, '').replace(/_/g, '-'); | ||
s.setAttribute('data-' + dataKey, String(options.data[k])); | ||
} | ||
} | ||
document.head.appendChild(s); | ||
}); | ||
function loadScript(src, options = {}) { | ||
return new Promise((resolve, reject) => { | ||
const s = document.createElement("script"); | ||
s.src = src; | ||
s.onload = resolve; | ||
s.onerror = reject; | ||
s.type = "text/javascript"; | ||
if (options.crossOrigin) { | ||
s.setAttribute("crossorigin", options.crossOrigin); | ||
} | ||
if (options.async) { | ||
s.async = true; | ||
} | ||
if (options.defer) { | ||
s.defer = true; | ||
} | ||
if (options.id) { | ||
s.setAttribute("id", options.id); | ||
} | ||
if (options.data) { | ||
for (const k in options.data) { | ||
const dataKey = k.replace(/^data-/, "").replace(/_/g, "-"); | ||
s.setAttribute("data-" + dataKey, String(options.data[k])); | ||
} | ||
} | ||
document.head.appendChild(s); | ||
}); | ||
} | ||
@@ -89,4 +73,2 @@ | ||
Object.defineProperty(exports, '__esModule', { value: true }); | ||
return exports; | ||
@@ -93,0 +75,0 @@ |
@@ -1,2 +0,2 @@ | ||
var Dom=function(e){"use strict";function t(e){if("string"==typeof e){var t=document.getElementById(e);if(!t)try{t=document.querySelector(e)}catch(r){}return t||void 0}return e}return e.create=function(e,t,r){var n=window.document.createElement(e);return null!=t&&(n.className=t),r&&r.appendChild(n),n},e.getElement=t,e.loadScript=function(e,t){return void 0===t&&(t={}),new Promise((function(r,n){var a=document.createElement("script");if(a.src=e,a.onload=r,a.onerror=n,a.type="text/javascript",t.crossOrigin&&a.setAttribute("crossorigin",t.crossOrigin),t.async&&(a.async=!0),t.defer&&(a.defer=!0),t.id&&a.setAttribute("id",t.id),t.data)for(var i in t.data){var o=i.replace(/^data-/,"").replace(/_/g,"-");a.setAttribute("data-"+o,String(t.data[i]))}document.head.appendChild(a)}))},e.remove=function(e){var r=t(e);if(r){var n=r.parentElement;n&&n.removeChild(r)}},Object.defineProperty(e,"__esModule",{value:!0}),e}({}); | ||
var Dom=function(t){"use strict";function e(t){if("string"==typeof t){let n=document.getElementById(t);if(!n)try{n=document.querySelector(t)}catch(e){}return n||void 0}return t}return t.create=function(t,e,n){const r=window.document.createElement(t);return null!=e&&(r.className=e),n&&n.appendChild(r),r},t.getElement=e,t.loadScript=function(t,e={}){return new Promise(((n,r)=>{const c=document.createElement("script");if(c.src=t,c.onload=n,c.onerror=r,c.type="text/javascript",e.crossOrigin&&c.setAttribute("crossorigin",e.crossOrigin),e.async&&(c.async=!0),e.defer&&(c.defer=!0),e.id&&c.setAttribute("id",e.id),e.data)for(const t in e.data){const n=t.replace(/^data-/,"").replace(/_/g,"-");c.setAttribute("data-"+n,String(e.data[t]))}document.head.appendChild(c)}))},t.remove=function(t){const n=e(t);if(n){const t=n.parentElement;t&&t.removeChild(n)}},t}({}); | ||
//# sourceMappingURL=dom.global.prod.js.map |
@@ -1,5 +0,3 @@ | ||
export declare function create<K extends keyof HTMLElementTagNameMap>(tagName: K, className?: string | null, container?: HTMLElement): HTMLElementTagNameMap[K]; | ||
export declare type ElementDef = HTMLElement | string; | ||
declare function create<K extends keyof HTMLElementTagNameMap>(tagName: K, className?: string | null, container?: HTMLElement): HTMLElementTagNameMap[K]; | ||
type ElementDef = HTMLElement | string; | ||
/** | ||
@@ -15,7 +13,6 @@ * Helper function for determining the HTML element through the transmitted parameters | ||
*/ | ||
export declare function getElement(el: HTMLElement | string): HTMLElement | undefined; | ||
declare function getElement(el: HTMLElement | string): HTMLElement | undefined; | ||
declare function remove(element: ElementDef): void; | ||
export declare function loadScript(src: string, options?: LoadScriptOptions): Promise<unknown>; | ||
export declare interface LoadScriptOptions { | ||
interface LoadScriptOptions { | ||
crossOrigin?: 'anonymous' | string; | ||
@@ -27,5 +24,4 @@ id?: string; | ||
} | ||
declare function loadScript(src: string, options?: LoadScriptOptions): Promise<unknown>; | ||
export declare function remove(element: ElementDef): void; | ||
export { } | ||
export { type ElementDef, type LoadScriptOptions, create, getElement, loadScript, remove }; |
{ | ||
"name": "@nextgis/dom", | ||
"version": "1.19.0", | ||
"version": "2.0.0-alpha.0", | ||
"description": "Collection of libraries for working with the DOM", | ||
@@ -11,3 +11,3 @@ "main": "lib/dom.global.prod.js", | ||
"devDependencies": { | ||
"@nextgis/build-tools": "^1.19.0" | ||
"@nextgis/build-tools": "^2.0.0-alpha.0" | ||
}, | ||
@@ -47,3 +47,3 @@ "buildOptions": { | ||
}, | ||
"gitHead": "d634208dde1cf94e340f2cf1e3254c3c599fec0d" | ||
"gitHead": "6a4a43d17a08e468d2362f1ae9590094e39aa6cc" | ||
} |
@@ -27,2 +27,2 @@ # Dom | ||
[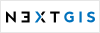](http://nextgis.com) | ||
[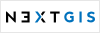](http://nextgis.com) |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
37108
234
1