@nextgis/properties-filter
Advanced tools
Comparing version 1.19.0 to 2.0.0-alpha.0
@@ -1,10 +0,3 @@ | ||
import type { Feature } from 'geojson'; | ||
import { Feature } from 'geojson'; | ||
/** @deprecated use {@link isPropertyFilter} instead */ | ||
export declare function checkIfPropertyFilter(filter: PropertyFilter | PropertiesFilter | string): filter is PropertyFilter; | ||
export declare function featureFilter(feature: Feature, filters: PropertiesFilter): boolean; | ||
export declare function isPropertyFilter(filter: PropertyFilter | PropertiesFilter | string): filter is PropertyFilter; | ||
/** | ||
@@ -20,15 +13,8 @@ * gt - greater (\>) | ||
*/ | ||
export declare type Operation = 'gt' | 'lt' | 'ge' | 'le' | 'eq' | 'ne' | 'in' | 'notin' | 'like' | 'ilike'; | ||
type Operation = 'gt' | 'lt' | 'ge' | 'le' | 'eq' | 'ne' | 'in' | 'notin' | 'like' | 'ilike'; | ||
/** @deprecated use {@link Operation} instead */ | ||
export declare type Operations = Operation; | ||
export declare type Properties = { | ||
type Operations = Operation; | ||
type Properties = { | ||
[name: string]: any; | ||
}; | ||
export declare type PropertiesFilter<T extends Properties = Properties> = ('all' | 'any' | PropertyFilter<T> | PropertiesFilter<T>)[]; | ||
export declare function propertiesFilter<T extends Properties = Properties>(properties: T, filters: PropertiesFilter<T>): boolean; | ||
/** | ||
@@ -39,8 +25,15 @@ * field, operation, value | ||
*/ | ||
export declare type PropertyFilter<T extends Properties = Properties> = [ | ||
T extends null ? string : keyof T | `%${string & keyof T}` | `%${string & keyof T}%` | `${string & keyof T}%`, | ||
Operation, | ||
any | ||
type PropertyFilter<T extends Properties = Properties> = [ | ||
T extends null ? string : keyof T | `%${string & keyof T}` | `%${string & keyof T}%` | `${string & keyof T}%`, | ||
Operation, | ||
any | ||
]; | ||
type PropertiesFilter<T extends Properties = Properties> = ('all' | 'any' | PropertyFilter<T> | PropertiesFilter<T>)[]; | ||
export { } | ||
declare function isPropertyFilter(filter: PropertyFilter | PropertiesFilter | string): filter is PropertyFilter; | ||
/** @deprecated use {@link isPropertyFilter} instead */ | ||
declare function checkIfPropertyFilter(filter: PropertyFilter | PropertiesFilter | string): filter is PropertyFilter; | ||
declare function featureFilter(feature: Feature, filters: PropertiesFilter): boolean; | ||
declare function propertiesFilter<T extends Properties = Properties>(properties: T, filters: PropertiesFilter<T>): boolean; | ||
export { type Operation, type Operations, type Properties, type PropertiesFilter, type PropertyFilter, checkIfPropertyFilter, featureFilter, isPropertyFilter, propertiesFilter }; |
@@ -1,2 +0,2 @@ | ||
/** Bundle of @nextgis/properties-filter; version: 1.19.0; author: NextGIS */ | ||
/** Bundle of @nextgis/properties-filter; version: 2.0.0-alpha.0; author: NextGIS */ | ||
'use strict'; | ||
@@ -7,89 +7,82 @@ | ||
function reEscape(s) { | ||
return s.replace(/[-/\\^$*+?.()|[\]{}]/g, '\\$&'); | ||
return s.replace(/[-/\\^$*+?.()|[\]{}]/g, "\\$&"); | ||
} | ||
function like(b, a, iLike) { | ||
a = String(a); | ||
b = String(b); | ||
if (a === b) | ||
return true; | ||
if (iLike && a.toUpperCase() === b.toUpperCase()) | ||
return true; | ||
const re = `^${reEscape(a)}$`.replace(/%/g, '.*').replace('_', '.'); | ||
return new RegExp(re, iLike ? 'i' : '').exec(b) !== null; | ||
a = String(a); | ||
b = String(b); | ||
if (a === b) | ||
return true; | ||
if (iLike && a.toUpperCase() === b.toUpperCase()) | ||
return true; | ||
const re = `^${reEscape(a)}$`.replace(/%/g, ".*").replace("_", "."); | ||
return new RegExp(re, iLike ? "i" : "").exec(b) !== null; | ||
} | ||
const operationsAliases = { | ||
// greater(>) | ||
gt: (a, b) => a > b, | ||
// lower(<) | ||
lt: (a, b) => a < b, | ||
// greater or equal(>=) | ||
ge: (a, b) => a >= b, | ||
// lower or equal(<=) | ||
le: (a, b) => a <= b, | ||
// equal(=) | ||
eq: (a, b) => a === b, | ||
// not equal(!=) | ||
ne: (a, b) => a !== b, | ||
in: (a, b) => b.indexOf(a) !== -1, | ||
notin: (a, b) => b.indexOf(a) === -1, | ||
// LIKE SQL statement(for strings compare) | ||
like: (a, b) => { | ||
return like(a, b); | ||
}, | ||
// ILIKE SQL statement(for strings compare) | ||
ilike: (a, b) => { | ||
return like(a, b, true); | ||
}, | ||
// greater(>) | ||
gt: (a, b) => a > b, | ||
// lower(<) | ||
lt: (a, b) => a < b, | ||
// greater or equal(>=) | ||
ge: (a, b) => a >= b, | ||
// lower or equal(<=) | ||
le: (a, b) => a <= b, | ||
// equal(=) | ||
eq: (a, b) => a === b, | ||
// not equal(!=) | ||
ne: (a, b) => a !== b, | ||
in: (a, b) => b.indexOf(a) !== -1, | ||
notin: (a, b) => b.indexOf(a) === -1, | ||
// LIKE SQL statement(for strings compare) | ||
like: (a, b) => { | ||
return like(a, b); | ||
}, | ||
// ILIKE SQL statement(for strings compare) | ||
ilike: (a, b) => { | ||
return like(a, b, true); | ||
} | ||
}; | ||
function isPropertyFilter(filter) { | ||
const pf = filter; | ||
if (pf.length === 3 && | ||
typeof pf[0] === 'string' && | ||
typeof pf[1] === 'string') { | ||
return true; | ||
} | ||
return false; | ||
const pf = filter; | ||
if (pf.length === 3 && typeof pf[0] === "string" && typeof pf[1] === "string") { | ||
return true; | ||
} | ||
return false; | ||
} | ||
/** @deprecated use {@link isPropertyFilter} instead */ | ||
function checkIfPropertyFilter(filter) { | ||
return isPropertyFilter(filter); | ||
return isPropertyFilter(filter); | ||
} | ||
function featureFilter(feature, filters) { | ||
const properties = { ...feature.properties }; | ||
if (properties) { | ||
// workaround to filter by feature id | ||
properties.$id = feature.id; | ||
return propertiesFilter(properties, filters); | ||
} | ||
return false; | ||
const properties = { ...feature.properties }; | ||
if (properties) { | ||
properties.$id = feature.id; | ||
return propertiesFilter(properties, filters); | ||
} | ||
return false; | ||
} | ||
function propertiesFilter(properties, filters) { | ||
const logic = typeof filters[0] === 'string' ? filters[0] : 'all'; | ||
const filterFunction = (p) => { | ||
if (isPropertyFilter(p)) { | ||
const [field, operation, value] = p; | ||
const operationExec = operationsAliases[operation]; | ||
if (operationExec) { | ||
if (operation === 'like' || operation === 'ilike') { | ||
if (typeof field === 'string') { | ||
let prop = ''; | ||
const value_ = field.replace(/^%?(\w+)%?$/, (match, cleanField) => { | ||
prop = properties[cleanField]; | ||
return field.replace(cleanField, value); | ||
}); | ||
return operationExec(prop, value_); | ||
} | ||
} | ||
return operationExec(properties[field], value); | ||
} | ||
return false; | ||
const logic = typeof filters[0] === "string" ? filters[0] : "all"; | ||
const filterFunction = (p) => { | ||
if (isPropertyFilter(p)) { | ||
const [field, operation, value] = p; | ||
const operationExec = operationsAliases[operation]; | ||
if (operationExec) { | ||
if (operation === "like" || operation === "ilike") { | ||
if (typeof field === "string") { | ||
let prop = ""; | ||
const value_ = field.replace(/^%?(\w+)%?$/, (match, cleanField) => { | ||
prop = properties[cleanField]; | ||
return field.replace(cleanField, value); | ||
}); | ||
return operationExec(prop, value_); | ||
} | ||
} | ||
else { | ||
return propertiesFilter(properties, p); | ||
} | ||
}; | ||
const filters_ = filters.filter((x) => Array.isArray(x)); | ||
return logic === 'any' | ||
? filters_.some(filterFunction) | ||
: filters_.every(filterFunction); | ||
return operationExec(properties[field], value); | ||
} | ||
return false; | ||
} else { | ||
return propertiesFilter(properties, p); | ||
} | ||
}; | ||
const filters_ = filters.filter((x) => Array.isArray(x)); | ||
return logic === "any" ? filters_.some(filterFunction) : filters_.every(filterFunction); | ||
} | ||
@@ -96,0 +89,0 @@ |
@@ -1,90 +0,99 @@ | ||
/** Bundle of @nextgis/properties-filter; version: 1.19.0; author: NextGIS */ | ||
/** Bundle of @nextgis/properties-filter; version: 2.0.0-alpha.0; author: NextGIS */ | ||
var __defProp = Object.defineProperty; | ||
var __getOwnPropSymbols = Object.getOwnPropertySymbols; | ||
var __hasOwnProp = Object.prototype.hasOwnProperty; | ||
var __propIsEnum = Object.prototype.propertyIsEnumerable; | ||
var __defNormalProp = (obj, key, value) => key in obj ? __defProp(obj, key, { enumerable: true, configurable: true, writable: true, value }) : obj[key] = value; | ||
var __spreadValues = (a, b) => { | ||
for (var prop in b || (b = {})) | ||
if (__hasOwnProp.call(b, prop)) | ||
__defNormalProp(a, prop, b[prop]); | ||
if (__getOwnPropSymbols) | ||
for (var prop of __getOwnPropSymbols(b)) { | ||
if (__propIsEnum.call(b, prop)) | ||
__defNormalProp(a, prop, b[prop]); | ||
} | ||
return a; | ||
}; | ||
function reEscape(s) { | ||
return s.replace(/[-/\\^$*+?.()|[\]{}]/g, '\\$&'); | ||
return s.replace(/[-/\\^$*+?.()|[\]{}]/g, "\\$&"); | ||
} | ||
function like(b, a, iLike) { | ||
a = String(a); | ||
b = String(b); | ||
if (a === b) | ||
return true; | ||
if (iLike && a.toUpperCase() === b.toUpperCase()) | ||
return true; | ||
const re = `^${reEscape(a)}$`.replace(/%/g, '.*').replace('_', '.'); | ||
return new RegExp(re, iLike ? 'i' : '').exec(b) !== null; | ||
a = String(a); | ||
b = String(b); | ||
if (a === b) | ||
return true; | ||
if (iLike && a.toUpperCase() === b.toUpperCase()) | ||
return true; | ||
const re = `^${reEscape(a)}$`.replace(/%/g, ".*").replace("_", "."); | ||
return new RegExp(re, iLike ? "i" : "").exec(b) !== null; | ||
} | ||
const operationsAliases = { | ||
// greater(>) | ||
gt: (a, b) => a > b, | ||
// lower(<) | ||
lt: (a, b) => a < b, | ||
// greater or equal(>=) | ||
ge: (a, b) => a >= b, | ||
// lower or equal(<=) | ||
le: (a, b) => a <= b, | ||
// equal(=) | ||
eq: (a, b) => a === b, | ||
// not equal(!=) | ||
ne: (a, b) => a !== b, | ||
in: (a, b) => b.indexOf(a) !== -1, | ||
notin: (a, b) => b.indexOf(a) === -1, | ||
// LIKE SQL statement(for strings compare) | ||
like: (a, b) => { | ||
return like(a, b); | ||
}, | ||
// ILIKE SQL statement(for strings compare) | ||
ilike: (a, b) => { | ||
return like(a, b, true); | ||
}, | ||
// greater(>) | ||
gt: (a, b) => a > b, | ||
// lower(<) | ||
lt: (a, b) => a < b, | ||
// greater or equal(>=) | ||
ge: (a, b) => a >= b, | ||
// lower or equal(<=) | ||
le: (a, b) => a <= b, | ||
// equal(=) | ||
eq: (a, b) => a === b, | ||
// not equal(!=) | ||
ne: (a, b) => a !== b, | ||
in: (a, b) => b.indexOf(a) !== -1, | ||
notin: (a, b) => b.indexOf(a) === -1, | ||
// LIKE SQL statement(for strings compare) | ||
like: (a, b) => { | ||
return like(a, b); | ||
}, | ||
// ILIKE SQL statement(for strings compare) | ||
ilike: (a, b) => { | ||
return like(a, b, true); | ||
} | ||
}; | ||
function isPropertyFilter(filter) { | ||
const pf = filter; | ||
if (pf.length === 3 && | ||
typeof pf[0] === 'string' && | ||
typeof pf[1] === 'string') { | ||
return true; | ||
} | ||
return false; | ||
const pf = filter; | ||
if (pf.length === 3 && typeof pf[0] === "string" && typeof pf[1] === "string") { | ||
return true; | ||
} | ||
return false; | ||
} | ||
/** @deprecated use {@link isPropertyFilter} instead */ | ||
function checkIfPropertyFilter(filter) { | ||
return isPropertyFilter(filter); | ||
return isPropertyFilter(filter); | ||
} | ||
function featureFilter(feature, filters) { | ||
const properties = { ...feature.properties }; | ||
if (properties) { | ||
// workaround to filter by feature id | ||
properties.$id = feature.id; | ||
return propertiesFilter(properties, filters); | ||
} | ||
return false; | ||
const properties = __spreadValues({}, feature.properties); | ||
if (properties) { | ||
properties.$id = feature.id; | ||
return propertiesFilter(properties, filters); | ||
} | ||
return false; | ||
} | ||
function propertiesFilter(properties, filters) { | ||
const logic = typeof filters[0] === 'string' ? filters[0] : 'all'; | ||
const filterFunction = (p) => { | ||
if (isPropertyFilter(p)) { | ||
const [field, operation, value] = p; | ||
const operationExec = operationsAliases[operation]; | ||
if (operationExec) { | ||
if (operation === 'like' || operation === 'ilike') { | ||
if (typeof field === 'string') { | ||
let prop = ''; | ||
const value_ = field.replace(/^%?(\w+)%?$/, (match, cleanField) => { | ||
prop = properties[cleanField]; | ||
return field.replace(cleanField, value); | ||
}); | ||
return operationExec(prop, value_); | ||
} | ||
} | ||
return operationExec(properties[field], value); | ||
} | ||
return false; | ||
const logic = typeof filters[0] === "string" ? filters[0] : "all"; | ||
const filterFunction = (p) => { | ||
if (isPropertyFilter(p)) { | ||
const [field, operation, value] = p; | ||
const operationExec = operationsAliases[operation]; | ||
if (operationExec) { | ||
if (operation === "like" || operation === "ilike") { | ||
if (typeof field === "string") { | ||
let prop = ""; | ||
const value_ = field.replace(/^%?(\w+)%?$/, (match, cleanField) => { | ||
prop = properties[cleanField]; | ||
return field.replace(cleanField, value); | ||
}); | ||
return operationExec(prop, value_); | ||
} | ||
} | ||
else { | ||
return propertiesFilter(properties, p); | ||
} | ||
}; | ||
const filters_ = filters.filter((x) => Array.isArray(x)); | ||
return logic === 'any' | ||
? filters_.some(filterFunction) | ||
: filters_.every(filterFunction); | ||
return operationExec(properties[field], value); | ||
} | ||
return false; | ||
} else { | ||
return propertiesFilter(properties, p); | ||
} | ||
}; | ||
const filters_ = filters.filter((x) => Array.isArray(x)); | ||
return logic === "any" ? filters_.some(filterFunction) : filters_.every(filterFunction); | ||
} | ||
@@ -91,0 +100,0 @@ |
@@ -1,2 +0,2 @@ | ||
function e(e,r,n){if((r=String(r))===(e=String(e)))return!0;if(n&&r.toUpperCase()===e.toUpperCase())return!0;const t=`^${i=r,i.replace(/[-/\\^$*+?.()|[\]{}]/g,"\\$&")}$`.replace(/%/g,".*").replace("_",".");var i;return null!==new RegExp(t,n?"i":"").exec(e)}const r={gt:(e,r)=>e>r,lt:(e,r)=>e<r,ge:(e,r)=>e>=r,le:(e,r)=>e<=r,eq:(e,r)=>e===r,ne:(e,r)=>e!==r,in:(e,r)=>-1!==r.indexOf(e),notin:(e,r)=>-1===r.indexOf(e),like:(r,n)=>e(r,n),ilike:(r,n)=>e(r,n,!0)};function n(e){return 3===e.length&&"string"==typeof e[0]&&"string"==typeof e[1]}function t(e){return n(e)}function i(e,r){const n={...e.properties};return!!n&&(n.$id=e.id,o(n,r))}function o(e,t){const i="string"==typeof t[0]?t[0]:"all",l=t=>{if(n(t)){const[n,i,o]=t,l=r[i];if(l){if(("like"===i||"ilike"===i)&&"string"==typeof n){let r="";const t=n.replace(/^%?(\w+)%?$/,((t,i)=>(r=e[i],n.replace(i,o))));return l(r,t)}return l(e[n],o)}return!1}return o(e,t)},c=t.filter((e=>Array.isArray(e)));return"any"===i?c.some(l):c.every(l)}export{t as checkIfPropertyFilter,i as featureFilter,n as isPropertyFilter,o as propertiesFilter}; | ||
var e=Object.defineProperty,r=Object.getOwnPropertySymbols,t=Object.prototype.hasOwnProperty,n=Object.prototype.propertyIsEnumerable,i=(r,t,n)=>t in r?e(r,t,{enumerable:!0,configurable:!0,writable:!0,value:n}):r[t]=n;function o(e,r,t){if((r=String(r))===(e=String(e)))return!0;if(t&&r.toUpperCase()===e.toUpperCase())return!0;const n=`^${i=r,i.replace(/[-/\\^$*+?.()|[\]{}]/g,"\\$&")}$`.replace(/%/g,".*").replace("_",".");var i;return null!==new RegExp(n,t?"i":"").exec(e)}const l={gt:(e,r)=>e>r,lt:(e,r)=>e<r,ge:(e,r)=>e>=r,le:(e,r)=>e<=r,eq:(e,r)=>e===r,ne:(e,r)=>e!==r,in:(e,r)=>-1!==r.indexOf(e),notin:(e,r)=>-1===r.indexOf(e),like:(e,r)=>o(e,r),ilike:(e,r)=>o(e,r,!0)};function p(e){return 3===e.length&&"string"==typeof e[0]&&"string"==typeof e[1]}function c(e){return p(e)}function a(e,o){const l=((e,o)=>{for(var l in o||(o={}))t.call(o,l)&&i(e,l,o[l]);if(r)for(var l of r(o))n.call(o,l)&&i(e,l,o[l]);return e})({},e.properties);return!!l&&(l.$id=e.id,f(l,o))}function f(e,r){const t="string"==typeof r[0]?r[0]:"all",n=r=>{if(p(r)){const[t,n,i]=r,o=l[n];if(o){if(("like"===n||"ilike"===n)&&"string"==typeof t){let r="";const n=t.replace(/^%?(\w+)%?$/,((n,o)=>(r=e[o],t.replace(o,i))));return o(r,n)}return o(e[t],i)}return!1}return f(e,r)},i=r.filter((e=>Array.isArray(e)));return"any"===t?i.some(n):i.every(n)}export{c as checkIfPropertyFilter,a as featureFilter,p as isPropertyFilter,f as propertiesFilter}; | ||
//# sourceMappingURL=properties-filter.esm-browser.prod.js.map |
@@ -1,90 +0,99 @@ | ||
/** Bundle of @nextgis/properties-filter; version: 1.19.0; author: NextGIS */ | ||
/** Bundle of @nextgis/properties-filter; version: 2.0.0-alpha.0; author: NextGIS */ | ||
var __defProp = Object.defineProperty; | ||
var __getOwnPropSymbols = Object.getOwnPropertySymbols; | ||
var __hasOwnProp = Object.prototype.hasOwnProperty; | ||
var __propIsEnum = Object.prototype.propertyIsEnumerable; | ||
var __defNormalProp = (obj, key, value) => key in obj ? __defProp(obj, key, { enumerable: true, configurable: true, writable: true, value }) : obj[key] = value; | ||
var __spreadValues = (a, b) => { | ||
for (var prop in b || (b = {})) | ||
if (__hasOwnProp.call(b, prop)) | ||
__defNormalProp(a, prop, b[prop]); | ||
if (__getOwnPropSymbols) | ||
for (var prop of __getOwnPropSymbols(b)) { | ||
if (__propIsEnum.call(b, prop)) | ||
__defNormalProp(a, prop, b[prop]); | ||
} | ||
return a; | ||
}; | ||
function reEscape(s) { | ||
return s.replace(/[-/\\^$*+?.()|[\]{}]/g, '\\$&'); | ||
return s.replace(/[-/\\^$*+?.()|[\]{}]/g, "\\$&"); | ||
} | ||
function like(b, a, iLike) { | ||
a = String(a); | ||
b = String(b); | ||
if (a === b) | ||
return true; | ||
if (iLike && a.toUpperCase() === b.toUpperCase()) | ||
return true; | ||
const re = `^${reEscape(a)}$`.replace(/%/g, '.*').replace('_', '.'); | ||
return new RegExp(re, iLike ? 'i' : '').exec(b) !== null; | ||
a = String(a); | ||
b = String(b); | ||
if (a === b) | ||
return true; | ||
if (iLike && a.toUpperCase() === b.toUpperCase()) | ||
return true; | ||
const re = `^${reEscape(a)}$`.replace(/%/g, ".*").replace("_", "."); | ||
return new RegExp(re, iLike ? "i" : "").exec(b) !== null; | ||
} | ||
const operationsAliases = { | ||
// greater(>) | ||
gt: (a, b) => a > b, | ||
// lower(<) | ||
lt: (a, b) => a < b, | ||
// greater or equal(>=) | ||
ge: (a, b) => a >= b, | ||
// lower or equal(<=) | ||
le: (a, b) => a <= b, | ||
// equal(=) | ||
eq: (a, b) => a === b, | ||
// not equal(!=) | ||
ne: (a, b) => a !== b, | ||
in: (a, b) => b.indexOf(a) !== -1, | ||
notin: (a, b) => b.indexOf(a) === -1, | ||
// LIKE SQL statement(for strings compare) | ||
like: (a, b) => { | ||
return like(a, b); | ||
}, | ||
// ILIKE SQL statement(for strings compare) | ||
ilike: (a, b) => { | ||
return like(a, b, true); | ||
}, | ||
// greater(>) | ||
gt: (a, b) => a > b, | ||
// lower(<) | ||
lt: (a, b) => a < b, | ||
// greater or equal(>=) | ||
ge: (a, b) => a >= b, | ||
// lower or equal(<=) | ||
le: (a, b) => a <= b, | ||
// equal(=) | ||
eq: (a, b) => a === b, | ||
// not equal(!=) | ||
ne: (a, b) => a !== b, | ||
in: (a, b) => b.indexOf(a) !== -1, | ||
notin: (a, b) => b.indexOf(a) === -1, | ||
// LIKE SQL statement(for strings compare) | ||
like: (a, b) => { | ||
return like(a, b); | ||
}, | ||
// ILIKE SQL statement(for strings compare) | ||
ilike: (a, b) => { | ||
return like(a, b, true); | ||
} | ||
}; | ||
function isPropertyFilter(filter) { | ||
const pf = filter; | ||
if (pf.length === 3 && | ||
typeof pf[0] === 'string' && | ||
typeof pf[1] === 'string') { | ||
return true; | ||
} | ||
return false; | ||
const pf = filter; | ||
if (pf.length === 3 && typeof pf[0] === "string" && typeof pf[1] === "string") { | ||
return true; | ||
} | ||
return false; | ||
} | ||
/** @deprecated use {@link isPropertyFilter} instead */ | ||
function checkIfPropertyFilter(filter) { | ||
return isPropertyFilter(filter); | ||
return isPropertyFilter(filter); | ||
} | ||
function featureFilter(feature, filters) { | ||
const properties = { ...feature.properties }; | ||
if (properties) { | ||
// workaround to filter by feature id | ||
properties.$id = feature.id; | ||
return propertiesFilter(properties, filters); | ||
} | ||
return false; | ||
const properties = __spreadValues({}, feature.properties); | ||
if (properties) { | ||
properties.$id = feature.id; | ||
return propertiesFilter(properties, filters); | ||
} | ||
return false; | ||
} | ||
function propertiesFilter(properties, filters) { | ||
const logic = typeof filters[0] === 'string' ? filters[0] : 'all'; | ||
const filterFunction = (p) => { | ||
if (isPropertyFilter(p)) { | ||
const [field, operation, value] = p; | ||
const operationExec = operationsAliases[operation]; | ||
if (operationExec) { | ||
if (operation === 'like' || operation === 'ilike') { | ||
if (typeof field === 'string') { | ||
let prop = ''; | ||
const value_ = field.replace(/^%?(\w+)%?$/, (match, cleanField) => { | ||
prop = properties[cleanField]; | ||
return field.replace(cleanField, value); | ||
}); | ||
return operationExec(prop, value_); | ||
} | ||
} | ||
return operationExec(properties[field], value); | ||
} | ||
return false; | ||
const logic = typeof filters[0] === "string" ? filters[0] : "all"; | ||
const filterFunction = (p) => { | ||
if (isPropertyFilter(p)) { | ||
const [field, operation, value] = p; | ||
const operationExec = operationsAliases[operation]; | ||
if (operationExec) { | ||
if (operation === "like" || operation === "ilike") { | ||
if (typeof field === "string") { | ||
let prop = ""; | ||
const value_ = field.replace(/^%?(\w+)%?$/, (match, cleanField) => { | ||
prop = properties[cleanField]; | ||
return field.replace(cleanField, value); | ||
}); | ||
return operationExec(prop, value_); | ||
} | ||
} | ||
else { | ||
return propertiesFilter(properties, p); | ||
} | ||
}; | ||
const filters_ = filters.filter((x) => Array.isArray(x)); | ||
return logic === 'any' | ||
? filters_.some(filterFunction) | ||
: filters_.every(filterFunction); | ||
return operationExec(properties[field], value); | ||
} | ||
return false; | ||
} else { | ||
return propertiesFilter(properties, p); | ||
} | ||
}; | ||
const filters_ = filters.filter((x) => Array.isArray(x)); | ||
return logic === "any" ? filters_.some(filterFunction) : filters_.every(filterFunction); | ||
} | ||
@@ -91,0 +100,0 @@ |
@@ -1,2 +0,2 @@ | ||
function e(e,r,n){if((r=String(r))===(e=String(e)))return!0;if(n&&r.toUpperCase()===e.toUpperCase())return!0;const t=`^${i=r,i.replace(/[-/\\^$*+?.()|[\]{}]/g,"\\$&")}$`.replace(/%/g,".*").replace("_",".");var i;return null!==new RegExp(t,n?"i":"").exec(e)}const r={gt:(e,r)=>e>r,lt:(e,r)=>e<r,ge:(e,r)=>e>=r,le:(e,r)=>e<=r,eq:(e,r)=>e===r,ne:(e,r)=>e!==r,in:(e,r)=>-1!==r.indexOf(e),notin:(e,r)=>-1===r.indexOf(e),like:(r,n)=>e(r,n),ilike:(r,n)=>e(r,n,!0)};function n(e){return 3===e.length&&"string"==typeof e[0]&&"string"==typeof e[1]}function t(e){return n(e)}function i(e,r){const n={...e.properties};return!!n&&(n.$id=e.id,o(n,r))}function o(e,t){const i="string"==typeof t[0]?t[0]:"all",l=t=>{if(n(t)){const[n,i,o]=t,l=r[i];if(l){if(("like"===i||"ilike"===i)&&"string"==typeof n){let r="";const t=n.replace(/^%?(\w+)%?$/,((t,i)=>(r=e[i],n.replace(i,o))));return l(r,t)}return l(e[n],o)}return!1}return o(e,t)},c=t.filter((e=>Array.isArray(e)));return"any"===i?c.some(l):c.every(l)}export{t as checkIfPropertyFilter,i as featureFilter,n as isPropertyFilter,o as propertiesFilter}; | ||
var e=Object.defineProperty,r=Object.getOwnPropertySymbols,t=Object.prototype.hasOwnProperty,n=Object.prototype.propertyIsEnumerable,i=(r,t,n)=>t in r?e(r,t,{enumerable:!0,configurable:!0,writable:!0,value:n}):r[t]=n;function o(e,r,t){if((r=String(r))===(e=String(e)))return!0;if(t&&r.toUpperCase()===e.toUpperCase())return!0;const n=`^${i=r,i.replace(/[-/\\^$*+?.()|[\]{}]/g,"\\$&")}$`.replace(/%/g,".*").replace("_",".");var i;return null!==new RegExp(n,t?"i":"").exec(e)}const l={gt:(e,r)=>e>r,lt:(e,r)=>e<r,ge:(e,r)=>e>=r,le:(e,r)=>e<=r,eq:(e,r)=>e===r,ne:(e,r)=>e!==r,in:(e,r)=>-1!==r.indexOf(e),notin:(e,r)=>-1===r.indexOf(e),like:(e,r)=>o(e,r),ilike:(e,r)=>o(e,r,!0)};function p(e){return 3===e.length&&"string"==typeof e[0]&&"string"==typeof e[1]}function c(e){return p(e)}function a(e,o){const l=((e,o)=>{for(var l in o||(o={}))t.call(o,l)&&i(e,l,o[l]);if(r)for(var l of r(o))n.call(o,l)&&i(e,l,o[l]);return e})({},e.properties);return!!l&&(l.$id=e.id,f(l,o))}function f(e,r){const t="string"==typeof r[0]?r[0]:"all",n=r=>{if(p(r)){const[t,n,i]=r,o=l[n];if(o){if(("like"===n||"ilike"===n)&&"string"==typeof t){let r="";const n=t.replace(/^%?(\w+)%?$/,((n,o)=>(r=e[o],t.replace(o,i))));return o(r,n)}return o(e[t],i)}return!1}return f(e,r)},i=r.filter((e=>Array.isArray(e)));return"any"===t?i.some(n):i.every(n)}export{c as checkIfPropertyFilter,a as featureFilter,p as isPropertyFilter,f as propertiesFilter}; | ||
//# sourceMappingURL=properties-filter.esm-bundler.prod.js.map |
@@ -1,136 +0,112 @@ | ||
/** Bundle of @nextgis/properties-filter; version: 1.19.0; author: NextGIS */ | ||
/** Bundle of @nextgis/properties-filter; version: 2.0.0-alpha.0; author: NextGIS */ | ||
var PropertiesFilter = (function (exports) { | ||
'use strict'; | ||
'use strict'; | ||
/****************************************************************************** | ||
Copyright (c) Microsoft Corporation. | ||
Permission to use, copy, modify, and/or distribute this software for any | ||
purpose with or without fee is hereby granted. | ||
THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH | ||
REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY | ||
AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT, | ||
INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM | ||
LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR | ||
OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR | ||
PERFORMANCE OF THIS SOFTWARE. | ||
***************************************************************************** */ | ||
var __assign = function() { | ||
__assign = Object.assign || function __assign(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
typeof SuppressedError === "function" ? SuppressedError : function (error, suppressed, message) { | ||
var e = new Error(message); | ||
return e.name = "SuppressedError", e.error = error, e.suppressed = suppressed, e; | ||
}; | ||
function reEscape(s) { | ||
return s.replace(/[-/\\^$*+?.()|[\]{}]/g, '\\$&'); | ||
var __defProp = Object.defineProperty; | ||
var __getOwnPropSymbols = Object.getOwnPropertySymbols; | ||
var __hasOwnProp = Object.prototype.hasOwnProperty; | ||
var __propIsEnum = Object.prototype.propertyIsEnumerable; | ||
var __defNormalProp = (obj, key, value) => key in obj ? __defProp(obj, key, { enumerable: true, configurable: true, writable: true, value }) : obj[key] = value; | ||
var __spreadValues = (a, b) => { | ||
for (var prop in b || (b = {})) | ||
if (__hasOwnProp.call(b, prop)) | ||
__defNormalProp(a, prop, b[prop]); | ||
if (__getOwnPropSymbols) | ||
for (var prop of __getOwnPropSymbols(b)) { | ||
if (__propIsEnum.call(b, prop)) | ||
__defNormalProp(a, prop, b[prop]); | ||
} | ||
return a; | ||
}; | ||
function reEscape(s) { | ||
return s.replace(/[-/\\^$*+?.()|[\]{}]/g, "\\$&"); | ||
} | ||
function like(b, a, iLike) { | ||
a = String(a); | ||
b = String(b); | ||
if (a === b) | ||
return true; | ||
if (iLike && a.toUpperCase() === b.toUpperCase()) | ||
return true; | ||
const re = `^${reEscape(a)}$`.replace(/%/g, ".*").replace("_", "."); | ||
return new RegExp(re, iLike ? "i" : "").exec(b) !== null; | ||
} | ||
const operationsAliases = { | ||
// greater(>) | ||
gt: (a, b) => a > b, | ||
// lower(<) | ||
lt: (a, b) => a < b, | ||
// greater or equal(>=) | ||
ge: (a, b) => a >= b, | ||
// lower or equal(<=) | ||
le: (a, b) => a <= b, | ||
// equal(=) | ||
eq: (a, b) => a === b, | ||
// not equal(!=) | ||
ne: (a, b) => a !== b, | ||
in: (a, b) => b.indexOf(a) !== -1, | ||
notin: (a, b) => b.indexOf(a) === -1, | ||
// LIKE SQL statement(for strings compare) | ||
like: (a, b) => { | ||
return like(a, b); | ||
}, | ||
// ILIKE SQL statement(for strings compare) | ||
ilike: (a, b) => { | ||
return like(a, b, true); | ||
} | ||
function like(b, a, iLike) { | ||
a = String(a); | ||
b = String(b); | ||
if (a === b) | ||
return true; | ||
if (iLike && a.toUpperCase() === b.toUpperCase()) | ||
return true; | ||
var re = "^".concat(reEscape(a), "$").replace(/%/g, '.*').replace('_', '.'); | ||
return new RegExp(re, iLike ? 'i' : '').exec(b) !== null; | ||
}; | ||
function isPropertyFilter(filter) { | ||
const pf = filter; | ||
if (pf.length === 3 && typeof pf[0] === "string" && typeof pf[1] === "string") { | ||
return true; | ||
} | ||
var operationsAliases = { | ||
// greater(>) | ||
gt: function (a, b) { return a > b; }, | ||
// lower(<) | ||
lt: function (a, b) { return a < b; }, | ||
// greater or equal(>=) | ||
ge: function (a, b) { return a >= b; }, | ||
// lower or equal(<=) | ||
le: function (a, b) { return a <= b; }, | ||
// equal(=) | ||
eq: function (a, b) { return a === b; }, | ||
// not equal(!=) | ||
ne: function (a, b) { return a !== b; }, | ||
in: function (a, b) { return b.indexOf(a) !== -1; }, | ||
notin: function (a, b) { return b.indexOf(a) === -1; }, | ||
// LIKE SQL statement(for strings compare) | ||
like: function (a, b) { | ||
return like(a, b); | ||
}, | ||
// ILIKE SQL statement(for strings compare) | ||
ilike: function (a, b) { | ||
return like(a, b, true); | ||
}, | ||
}; | ||
function isPropertyFilter(filter) { | ||
var pf = filter; | ||
if (pf.length === 3 && | ||
typeof pf[0] === 'string' && | ||
typeof pf[1] === 'string') { | ||
return true; | ||
} | ||
return false; | ||
return false; | ||
} | ||
function checkIfPropertyFilter(filter) { | ||
return isPropertyFilter(filter); | ||
} | ||
function featureFilter(feature, filters) { | ||
const properties = __spreadValues({}, feature.properties); | ||
if (properties) { | ||
properties.$id = feature.id; | ||
return propertiesFilter(properties, filters); | ||
} | ||
/** @deprecated use {@link isPropertyFilter} instead */ | ||
function checkIfPropertyFilter(filter) { | ||
return isPropertyFilter(filter); | ||
} | ||
function featureFilter(feature, filters) { | ||
var properties = __assign({}, feature.properties); | ||
if (properties) { | ||
// workaround to filter by feature id | ||
properties.$id = feature.id; | ||
return propertiesFilter(properties, filters); | ||
return false; | ||
} | ||
function propertiesFilter(properties, filters) { | ||
const logic = typeof filters[0] === "string" ? filters[0] : "all"; | ||
const filterFunction = (p) => { | ||
if (isPropertyFilter(p)) { | ||
const [field, operation, value] = p; | ||
const operationExec = operationsAliases[operation]; | ||
if (operationExec) { | ||
if (operation === "like" || operation === "ilike") { | ||
if (typeof field === "string") { | ||
let prop = ""; | ||
const value_ = field.replace(/^%?(\w+)%?$/, (match, cleanField) => { | ||
prop = properties[cleanField]; | ||
return field.replace(cleanField, value); | ||
}); | ||
return operationExec(prop, value_); | ||
} | ||
} | ||
return operationExec(properties[field], value); | ||
} | ||
return false; | ||
} | ||
function propertiesFilter(properties, filters) { | ||
var logic = typeof filters[0] === 'string' ? filters[0] : 'all'; | ||
var filterFunction = function (p) { | ||
if (isPropertyFilter(p)) { | ||
var field_1 = p[0], operation = p[1], value_1 = p[2]; | ||
var operationExec = operationsAliases[operation]; | ||
if (operationExec) { | ||
if (operation === 'like' || operation === 'ilike') { | ||
if (typeof field_1 === 'string') { | ||
var prop_1 = ''; | ||
var value_ = field_1.replace(/^%?(\w+)%?$/, function (match, cleanField) { | ||
prop_1 = properties[cleanField]; | ||
return field_1.replace(cleanField, value_1); | ||
}); | ||
return operationExec(prop_1, value_); | ||
} | ||
} | ||
return operationExec(properties[field_1], value_1); | ||
} | ||
return false; | ||
} | ||
else { | ||
return propertiesFilter(properties, p); | ||
} | ||
}; | ||
var filters_ = filters.filter(function (x) { return Array.isArray(x); }); | ||
return logic === 'any' | ||
? filters_.some(filterFunction) | ||
: filters_.every(filterFunction); | ||
} | ||
} else { | ||
return propertiesFilter(properties, p); | ||
} | ||
}; | ||
const filters_ = filters.filter((x) => Array.isArray(x)); | ||
return logic === "any" ? filters_.some(filterFunction) : filters_.every(filterFunction); | ||
} | ||
exports.checkIfPropertyFilter = checkIfPropertyFilter; | ||
exports.featureFilter = featureFilter; | ||
exports.isPropertyFilter = isPropertyFilter; | ||
exports.propertiesFilter = propertiesFilter; | ||
exports.checkIfPropertyFilter = checkIfPropertyFilter; | ||
exports.featureFilter = featureFilter; | ||
exports.isPropertyFilter = isPropertyFilter; | ||
exports.propertiesFilter = propertiesFilter; | ||
Object.defineProperty(exports, '__esModule', { value: true }); | ||
return exports; | ||
return exports; | ||
})({}); | ||
//# sourceMappingURL=properties-filter.global.js.map |
@@ -1,2 +0,2 @@ | ||
var PropertiesFilter=function(r){"use strict";var e=function(){return e=Object.assign||function(r){for(var e,n=1,t=arguments.length;n<t;n++)for(var i in e=arguments[n])Object.prototype.hasOwnProperty.call(e,i)&&(r[i]=e[i]);return r},e.apply(this,arguments)};function n(r,e,n){if((e=String(e))===(r=String(r)))return!0;if(n&&e.toUpperCase()===r.toUpperCase())return!0;var t,i="^".concat((t=e,t.replace(/[-/\\^$*+?.()|[\]{}]/g,"\\$&")),"$").replace(/%/g,".*").replace("_",".");return null!==new RegExp(i,n?"i":"").exec(r)}"function"==typeof SuppressedError&&SuppressedError;var t={gt:function(r,e){return r>e},lt:function(r,e){return r<e},ge:function(r,e){return r>=e},le:function(r,e){return r<=e},eq:function(r,e){return r===e},ne:function(r,e){return r!==e},in:function(r,e){return-1!==e.indexOf(r)},notin:function(r,e){return-1===e.indexOf(r)},like:function(r,e){return n(r,e)},ilike:function(r,e){return n(r,e,!0)}};function i(r){return 3===r.length&&"string"==typeof r[0]&&"string"==typeof r[1]}function u(r,e){var n="string"==typeof e[0]?e[0]:"all",o=function(e){if(i(e)){var n=e[0],o=e[1],f=e[2],c=t[o];if(c){if(("like"===o||"ilike"===o)&&"string"==typeof n){var p="",a=n.replace(/^%?(\w+)%?$/,(function(e,t){return p=r[t],n.replace(t,f)}));return c(p,a)}return c(r[n],f)}return!1}return u(r,e)},f=e.filter((function(r){return Array.isArray(r)}));return"any"===n?f.some(o):f.every(o)}return r.checkIfPropertyFilter=function(r){return i(r)},r.featureFilter=function(r,n){var t=e({},r.properties);return!!t&&(t.$id=r.id,u(t,n))},r.isPropertyFilter=i,r.propertiesFilter=u,Object.defineProperty(r,"__esModule",{value:!0}),r}({}); | ||
var PropertiesFilter=function(e){"use strict";var r=Object.defineProperty,t=Object.getOwnPropertySymbols,n=Object.prototype.hasOwnProperty,i=Object.prototype.propertyIsEnumerable,o=(e,t,n)=>t in e?r(e,t,{enumerable:!0,configurable:!0,writable:!0,value:n}):e[t]=n;function l(e,r,t){if((r=String(r))===(e=String(e)))return!0;if(t&&r.toUpperCase()===e.toUpperCase())return!0;const n=`^${i=r,i.replace(/[-/\\^$*+?.()|[\]{}]/g,"\\$&")}$`.replace(/%/g,".*").replace("_",".");var i;return null!==new RegExp(n,t?"i":"").exec(e)}const p={gt:(e,r)=>e>r,lt:(e,r)=>e<r,ge:(e,r)=>e>=r,le:(e,r)=>e<=r,eq:(e,r)=>e===r,ne:(e,r)=>e!==r,in:(e,r)=>-1!==r.indexOf(e),notin:(e,r)=>-1===r.indexOf(e),like:(e,r)=>l(e,r),ilike:(e,r)=>l(e,r,!0)};function c(e){return 3===e.length&&"string"==typeof e[0]&&"string"==typeof e[1]}function f(e,r){const t="string"==typeof r[0]?r[0]:"all",n=r=>{if(c(r)){const[t,n,i]=r,o=p[n];if(o){if(("like"===n||"ilike"===n)&&"string"==typeof t){let r="";const n=t.replace(/^%?(\w+)%?$/,((n,o)=>(r=e[o],t.replace(o,i))));return o(r,n)}return o(e[t],i)}return!1}return f(e,r)},i=r.filter((e=>Array.isArray(e)));return"any"===t?i.some(n):i.every(n)}return e.checkIfPropertyFilter=function(e){return c(e)},e.featureFilter=function(e,r){const l=((e,r)=>{for(var l in r||(r={}))n.call(r,l)&&o(e,l,r[l]);if(t)for(var l of t(r))i.call(r,l)&&o(e,l,r[l]);return e})({},e.properties);return!!l&&(l.$id=e.id,f(l,r))},e.isPropertyFilter=c,e.propertiesFilter=f,e}({}); | ||
//# sourceMappingURL=properties-filter.global.prod.js.map |
{ | ||
"name": "@nextgis/properties-filter", | ||
"version": "1.19.0", | ||
"version": "2.0.0-alpha.0", | ||
"description": "Filtering objects by its properties using expressions", | ||
@@ -11,7 +11,7 @@ "main": "index.js", | ||
"dependencies": { | ||
"@nextgis/utils": "^1.19.0", | ||
"@nextgis/utils": "^2.0.0-alpha.0", | ||
"@types/geojson": "^7946.0.8" | ||
}, | ||
"devDependencies": { | ||
"@nextgis/build-tools": "^1.19.0" | ||
"@nextgis/build-tools": "^2.0.0-alpha.0" | ||
}, | ||
@@ -52,3 +52,3 @@ "buildOptions": { | ||
}, | ||
"gitHead": "d634208dde1cf94e340f2cf1e3254c3c599fec0d" | ||
"gitHead": "6a4a43d17a08e468d2362f1ae9590094e39aa6cc" | ||
} |
@@ -77,2 +77,2 @@ # Properties Filter | ||
[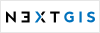](http://nextgis.com) | ||
[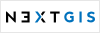](http://nextgis.com) |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
74652
467
1
+ Added@nextgis/utils@2.5.0(transitive)
- Removed@nextgis/utils@1.19.0(transitive)