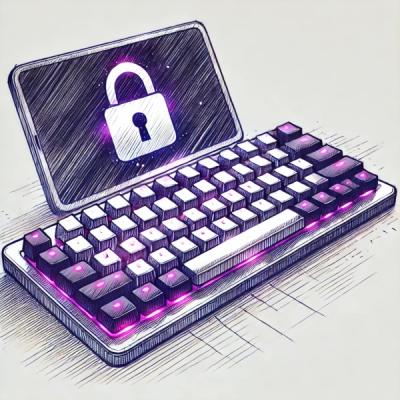
Research
Security News
Malicious npm Package Typosquats react-login-page to Deploy Keylogger
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
@nuxtjs/axios
Advanced tools
Package description
@nuxtjs/axios is a Nuxt.js module that integrates the Axios HTTP client with Nuxt.js applications. It simplifies making HTTP requests in a Nuxt.js project by providing a consistent API and additional features tailored for Nuxt.js.
Making GET Requests
This feature allows you to make GET requests to fetch data from an API endpoint. The example demonstrates how to use the $axios.$get method within the asyncData lifecycle hook to fetch data before rendering the page.
async asyncData({ $axios }) {
const data = await $axios.$get('https://api.example.com/data');
return { data };
}
Making POST Requests
This feature allows you to make POST requests to send data to an API endpoint. The example shows how to use the $axios.$post method to submit form data to a server.
async submitForm({ $axios }, formData) {
const response = await $axios.$post('https://api.example.com/submit', formData);
return response;
}
Setting Base URL
This feature allows you to set a base URL for all Axios requests. The example demonstrates how to configure the base URL in the Nuxt.js configuration file.
export default {
axios: {
baseURL: 'https://api.example.com'
}
}
Handling Errors
This feature allows you to handle errors that occur during HTTP requests. The example shows how to use a try-catch block to catch errors and handle them appropriately within the asyncData lifecycle hook.
async asyncData({ $axios, error }) {
try {
const data = await $axios.$get('https://api.example.com/data');
return { data };
} catch (e) {
error({ statusCode: 500, message: 'Internal Server Error' });
}
}
Axios is a promise-based HTTP client for the browser and Node.js. It provides a simple API for making HTTP requests and is the core library that @nuxtjs/axios is built upon. While @nuxtjs/axios is tailored for Nuxt.js, axios can be used in any JavaScript environment.
Vue-resource is an HTTP client for Vue.js applications. It provides services for making web requests and handling responses using a simple and easy-to-understand API. Compared to @nuxtjs/axios, vue-resource is more tightly integrated with Vue.js but lacks some of the advanced features and flexibility of axios.
Fetch is a native JavaScript API for making HTTP requests. It is built into modern browsers and provides a more low-level approach compared to axios. While fetch is more lightweight and does not require additional dependencies, it lacks some of the convenience features provided by axios, such as request and response interceptors.
Changelog
5.0.0-alpha.1 (2018-01-28)
<a name="5.0.0-alpha.0"></a>
Readme
Secure and Easy Axios integration with Nuxt.js.
If you are coming from an older release please be sure to read Migration Guide
✓ Automatically set base URL for client & server side
✓ Exposes setToken
function to $axios
so we can easily and globally set authentication tokens
✓ Throws nuxt-friendly errors and optionally redirect on specific error codes
✓ Automatically enables withCredentials
when requesting to base URL
✓ Proxy request headers in SSR (Useful for auth)
✓ Fetch Style requests
✓ Automatically integrate with Nuxt.js progress bar
Install with yarn:
yarn add @nuxtjs/axios
Install with npm:
npm install @nuxtjs/axios
nuxt.config.js
{
modules: [
'@nuxtjs/axios',
],
axios: {
// proxyHeaders: false
}
}
asyncData
async asyncData({ app }) {
const ip = await app.$axios.$get('http://icanhazip.com')
return { ip }
}
methods
/created
/mounted
/etc
methods: {
async fetchSomething() {
const ip = await this.$axios.$get('http://icanhazip.com')
this.ip = ip
}
}
nuxtServerInit
async nuxtServerInit ({ commit }, { app }) {
const ip = await app.$axios.$get('http://icanhazip.com')
commit('SET_IP', ip)
}
// In store
{
actions: {
async getIP ({ commit }) {
const ip = await this.$axios.$get('http://icanhazip.com')
commit('SET_IP', ip)
}
}
}
If you need to customize axios by registering interceptors and changing global config, you have to create a nuxt plugin.
nuxt.config.js
modules: [
'@nuxtjs/axios',
],
plugins: [
'~/plugins/axios'
]
plugins/axios.js
export default function ({ $axios, redirect }) {
$axios.onRequest(config => {
console.log('Making request to ' + config.url)
})
$axios.onError(error => {
if (error.code === 400) {
redirect('/400')
}
})
}
Axios plugin provides helpers to register axios interceptors easier and faster.
onRequest(config)
onResponse(response)
onError(err)
onRequestError(err)
onResponseError(err)
This functions don't have to return anything by default.
Example: (plugins/axios.js
)
export default function ({ $axios, redirect }) {
$axios.onError(error => {
if(error.code === 500) {
redirect('/sorry')
}
})
}
Axios plugin also supports fetch style requests with $
prefixed methods:
// Normal usage with axios
let data = (await $axios.get('...')).data
// Fetch Style
let data = await $axios.$get('...')
setHeader(name, value, scopes='common')
Axios instance has a helper to easily set any header.
Parameters:
common
meaning all types of requestsget
, post
, delete
, ...// Adds header: `Authorization: 123` to all requests
this.$axios.setHeader('Authorization', '123')
// Overrides `Authorization` header with new value
this.$axios.setHeader('Authorization', '456')
// Adds header: `Content-Type: application/x-www-form-urlencoded` to only post requests
this.$axios.setHeader('Content-Type', 'application/x-www-form-urlencoded', [
'post'
])
// Removes default Content-Type header from `post` scope
this.$axios.setHeader('Content-Type', false, ['post'])
setToken(token, type, scopes='common')
Axios instance has an additional helper to easily set global authentication header.
Parameters:
Bearer
).common
meaning all types of requestsget
, post
, delete
, ...// Adds header: `Authorization: 123` to all requests
this.$axios.setToken('123')
// Overrides `Authorization` header with new value
this.$axios.setToken('456')
// Adds header: `Authorization: Bearer 123` to all requests
this.$axios.setToken('123', 'Bearer')
// Adds header: `Authorization: Bearer 123` to only post and delete requests
this.$axios.setToken('123', 'Bearer', ['post', 'delete'])
// Removes default Authorization header from `common` scope (all requests)
this.$axios.setToken(false)
You can pass options using module options or axios
section in nuxt.config.js
prefix
, host
and port
This options are used for default values of baseURL
and browserBaseURL
.
Can be customized with API_PREFIX
, API_HOST
(or HOST
) and API_PORT
(or PORT
) environment variables.
Default value of prefix
is /
.
baseURL
http://[HOST]:[PORT][PREFIX]
Base URL which is used and prepended to make requests in server side.
Environment variable API_URL
can be used to override baseURL
.
browserBaseURL
baseURL
(or prefix
when options.proxyMode
is true
)Base URL which is used and prepended to make requests in client side.
Environment variable API_URL_BROWSER
can be used to override browserBaseURL
.
progress
true
Integrate with Nuxt.js progress bar to show a loading bar while making requests. (Only on browser, when loading bar is available.)
credentials
false
Adds an interceptor to automatically set withCredentials
config of axios when requesting to baseUrl
which allows passing authentication headers to backend.
debug
false
Adds interceptors to log request and responses.
proxyHeaders
true
In SSR context, sets client request header as axios default request headers. This is useful for making requests which need cookie based auth on server side. Also helps making consistent requests in both SSR and Client Side code.
NOTE: If directing requests at a url protected by CloudFlare's CDN you should set this to false to prevent CloudFlare from mistakenly detecting a reverse proxy loop and returning a 403 error.
proxyHeadersIgnore
['host', 'accept']
Only efficient when proxyHeaders
is set to true. Removes unwanted request headers to the API backend in SSR.
MIT License - Copyright (c) 2017 Nuxt Community
FAQs
Unknown package
The npm package @nuxtjs/axios receives a total of 103,873 weekly downloads. As such, @nuxtjs/axios popularity was classified as popular.
We found that @nuxtjs/axios demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers unpack a typosquatting package with malicious code that logs keystrokes and exfiltrates sensitive data to a remote server.
Security News
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.