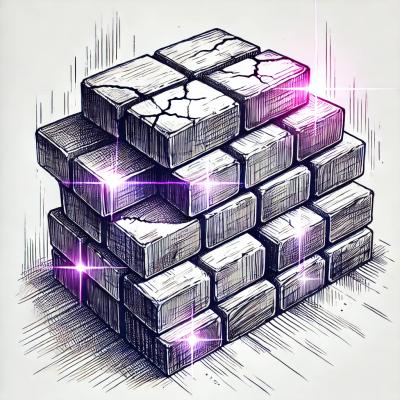
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@openbook-dex/pool
Advanced tools
JavaScript client library for interacting with Project Serum Pools.
Using npm:
npm install @solana/web3.js @project-serum/pool
Using yarn:
yarn add @solana/web3.js @project-serum/pool
Fetch and decode pool state:
import { Connection, PublicKey } from '@solana/web3.js';
import { loadPoolInfo, PoolTransactions } from '@openbook-dex/pool';
let connection = new Connection('...');
let poolAddress = new PublicKey('...'); // Address of the pool.
let poolInfo = await loadPoolInfo(connection, poolAddress);
console.log(poolInfo.state);
See loadPoolInfo()
and PoolState for details.
If you already have the pool state data and just need to decode it, you can
call isPoolState()
and decodePoolState()
directly.
import { decodePoolState } from '@openbook-dex/pool';
// Pool state account data, e.g. from Connection.getAccountInfo or Connection.onAccountChange
let data = new Buffer('...');
let poolState = decodePoolState(data);
console.log(poolState);
See PoolState
for details on what the pool state contains.
Use getPoolBasket()
to fetch the current pool basket (the quantity of each token needed to create N pool tokens
or the quantity of each token received for redeeming N pool tokens).
import { Connection, PublicKey } from '@solana/web3.js';
import { loadPoolInfo, getPoolBasket } from '@openbook-dex/pool';
import BN from 'bn.js';
let connection = new Connection('...');
let poolAddress = new PublicKey('...'); // Address of the pool.
let poolInfo = await loadPoolInfo(connection, poolAddress);
let basket = await getPoolBasket(
connection,
poolInfo,
{ create: new BN(100) },
// Arbitrary SOL address, can be anything as long as it has nonzero SOL
// and is not a program-owned address.
new PublicKey('...'),
);
console.log(basket);
Send a transaction to create pool tokens:
import { Account, Connection, PublicKey } from '@solana/web3.js';
import { loadPoolInfo, PoolTransactions } from '@openbook-dex/pool';
import BN from 'bn.js';
let connection = new Connection('...');
let poolAddress = new PublicKey('...'); // Address of the pool.
let payer = new Account('...'); // Account to pay for solana fees.
let poolInfo = await loadPoolInfo(connection, poolAddress);
let { transaction, signers } = PoolTransactions.execute(
poolInfo,
{
// Number of tokens to create.
create: new BN(100),
},
{
// Spl-token account to send the created tokens.
poolTokenAccount: new PublicKey('...'),
// Spl-token accounts to pull funds from.
assetAccounts: [new PublicKey('...'), new Public('...')],
// Owner of poolTokenAccount and assetAccounts.
owner: payer.publicKey,
},
// Expected creation cost.
[new BN(10), new BN(10)],
);
await connection.sendTransaction(transaction, [payer, ...signers]);
See PoolTransactions.execute
for details.
Send a transaction to redeem pool tokens:
import { Account, Connection, PublicKey } from '@solana/web3.js';
import { loadPoolInfo, PoolTransactions } from '@openbook-dex/pool';
import BN from 'bn.js';
let connection = new Connection('...');
let poolAddress = new PublicKey('...'); // Address of the pool.
let payer = new Account('...'); // Account to pay for solana fees.
let poolInfo = await loadPoolInfo(connection, poolAddress);
let { transaction, signers } = PoolTransactions.execute(
poolInfo,
{
// Number of tokens to redeem.
redeem: new BN(100),
},
{
// Spl-token account to pull the pool tokens to redeem from.
poolTokenAccount: new PublicKey('...'),
// Spl-token accounts to send the redemption proceeds.
assetAccounts: [new PublicKey('...'), new Public('...')],
// Owner of poolTokenAccount and assetAccounts.
owner: payer.publicKey,
},
// Expected redemption proceeds.
[new BN(10), new BN(10)],
);
await connection.sendTransaction(transaction, [payer, ...signers]);
See PoolTransactions.execute
for details.
FAQs
Library for interacting with the openbook pools
The npm package @openbook-dex/pool receives a total of 0 weekly downloads. As such, @openbook-dex/pool popularity was classified as not popular.
We found that @openbook-dex/pool demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.