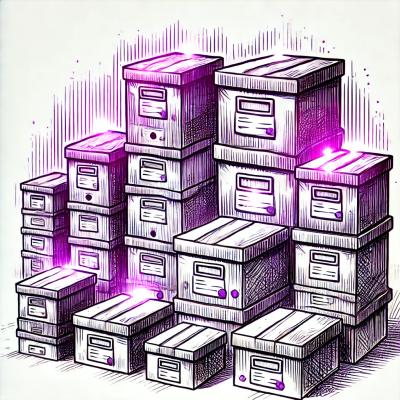
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
@phosphor-icons/react
Advanced tools
@phosphor-icons/react is a React component library for Phosphor Icons, a flexible icon family for interfaces, diagrams, presentations, and more. It allows you to easily integrate Phosphor Icons into your React applications.
Basic Icon Usage
This feature allows you to use Phosphor Icons in your React application by importing the desired icon and using it as a component.
import { PhosphorIcon } from '@phosphor-icons/react';
function App() {
return (
<div>
<PhosphorIcon name="smiley" size={32} />
</div>
);
}
export default App;
Customizing Icon Size
You can customize the size of the icons by passing the `size` prop to the icon component.
import { PhosphorIcon } from '@phosphor-icons/react';
function App() {
return (
<div>
<PhosphorIcon name="smiley" size={64} />
</div>
);
}
export default App;
Changing Icon Color
This feature allows you to change the color of the icons by passing the `color` prop to the icon component.
import { PhosphorIcon } from '@phosphor-icons/react';
function App() {
return (
<div>
<PhosphorIcon name="smiley" color="red" />
</div>
);
}
export default App;
Using Different Icon Weights
You can use different icon weights (e.g., thin, light, regular, bold) by passing the `weight` prop to the icon component.
import { PhosphorIcon } from '@phosphor-icons/react';
function App() {
return (
<div>
<PhosphorIcon name="smiley" weight="bold" />
</div>
);
}
export default App;
react-icons is a popular library that provides a wide range of icons from various icon libraries (e.g., Font Awesome, Material Design Icons) as React components. It offers a similar functionality to @phosphor-icons/react but with a broader selection of icon sets.
fontawesome-svg-core is a package that allows you to use Font Awesome icons as SVGs in your React applications. It provides a wide range of icons and offers extensive customization options. It is similar to @phosphor-icons/react but focuses on Font Awesome icons.
[!IMPORTANT] As part of a major update, we will be replacing the existing
phosphor-react
package with@phosphor-icons/react
. We recommend using this new version, as it has improved performance and a significantly smaller bundle size. No APIs have been changed, so drop-in replacement should be straightforward. The legacy package will continue to receive maintenance, but will not be updated with new icons upstream. Take me to the legacy version ➜
Phosphor is a flexible icon family for interfaces, diagrams, presentations — whatever, really. Explore all our icons at phosphoricons.com.
npm i @phosphor-icons/react
Simply import the icons you need, and add them anywhere in your render method. Phosphor supports tree-shaking, so your bundle only includes code for the icons you use.
import { Horse, Heart, Cube } from "@phosphor-icons/react";
const App = () => {
return (
<main>
<Horse />
<Heart color="#AE2983" weight="fill" size={32} />
<Cube color="teal" weight="duotone" />
</main>
);
};
When using Phosphor Icons in an SSR environment, within a React Server Component, or in any environment that does not permit the use of the Context API (Next.js Server Component, for example), import icons from the /dist/ssr
submodule:
import { Fish } from "@phosphor-icons/react/dist/ssr";
const MyServerComponent = () => {
return <Fish weight="duotone" />;
};
[!NOTE] These variants do not use React Context, and thus cannot inherit styles from an ancestor
IconContext
.
Icon components accept all props that you can pass to a normal SVG element, including inline style
objects, onClick
handlers, and more. The main way of styling them will usually be with the following props:
string
– Icon stroke/fill color. Can be any CSS color string, including hex
, rgb
, rgba
, hsl
, hsla
, named colors, or the special currentColor
variable.number | string
– Icon height & width. As with standard React elements, this can be a number, or a string with units in px
, %
, em
, rem
, pt
, cm
, mm
, in
."thin" | "light" | "regular" | "bold" | "fill" | "duotone"
– Icon weight/style. Can also be used, for example, to "toggle" an icon's state: a rating component could use Stars with weight="regular"
to denote an empty star, and weight="fill"
to denote a filled star.boolean
– Flip the icon horizontally. Can be useful in RTL languages where normal icon orientation is not appropriate.string
– Add accessible alt text to an icon.Phosphor takes advantage of React Context to make applying a default style to all icons simple. Create an IconContext.Provider
at the root of the app (or anywhere above the icons in the tree) and pass in a configuration object with props to be applied by default to all icons:
import { IconContext, Horse, Heart, Cube } from "@phosphor-icons/react";
const App = () => {
return (
<IconContext.Provider
value={{
color: "limegreen",
size: 32,
weight: "bold",
mirrored: false,
}}
>
<div>
<Horse /> {/* I'm lime-green, 32px, and bold! */}
<Heart /> {/* Me too! */}
<Cube /> {/* Me three :) */}
</div>
</IconContext.Provider>
);
};
You may create multiple Contexts for styling icons differently in separate regions of an application; icons use the nearest Context above them to determine their style.
[!NOTE] The context will also pass any provided SVG props down to icon instances, which can be useful E.G. in adding accessible
aria-label
s,classNames
, etc.
[!NOTE] React Context is not available in some environments. See React Server Components and SSR for details.
Components can accept arbitrary SVG elements as children, so long as they are valid children of the <svg>
element. This can be used to modify an icon with background layers or shapes, filters, animations, and more. The children will be placed below the normal icon contents.
The following will cause the Cube icon to rotate and pulse:
const RotatingCube = () => {
return (
<Cube color="darkorchid" weight="duotone">
<animate
attributeName="opacity"
values="0;1;0"
dur="4s"
repeatCount="indefinite"
></animate>
<animateTransform
attributeName="transform"
attributeType="XML"
type="rotate"
dur="5s"
from="0 0 0"
to="360 0 0"
repeatCount="indefinite"
></animateTransform>
</Cube>
);
};
[!NOTE] The coordinate space of slotted elements is relative to the contents of the icon
viewBox
, which is 256x256 square. Only valid SVG elements will be rendered.
You may wish to import all icons at once for use in your project, though depending on your bundler this could prevent tree-shaking and make your app's bundle larger.
import * as Icon from "@phosphor-icons/react";
<Icon.Smiley />
<Icon.Folder weight="thin" />
<Icon.BatteryHalf size="24px" />
For information on using Phosphor Icons in Server Components, see See React Server Components and SSR.
It is possible to extend Phosphor with your custom icons, taking advantage of the styling and context abstractions used in our library. To create a custom icon, first design your icons on a 256x256 pixel grid, and export them as SVG. For best results, flatten the icon so that you only export assets with path
elements. Strip any fill
or stroke
attributes, as these will be inherited from the wrapper.
Next, create a new React forwardRef
component, importing the IconBase
component, as well as the Icon
and IconWeight
types from this library. Define a Map<IconWeight, ReactElement>
that maps each icon weight to the contents of each SVG asset, effectively removing the wrapping <svg>
element from each. Name your component, and render an <IconBase />
, passing all props and the ref, as well as the weights
you defined earlier, as JSX props:
import { forwardRef, ReactElement } from "react";
import { Icon, IconBase, IconWeight } from "@phosphor-icons/react";
const weights = new Map<IconWeight, ReactElement>([
["thin", <path d="..." />],
["light", <path d="..." />],
["regular", <path d="..." />],
["bold", <path d="..." />],
["fill", <path d="..." />],
[
"duotone",
<>
<path d="..." opacity="0.2" />
<path d="..." />
</>,
],
]);
const CustomIcon: Icon = forwardRef((props, ref) => (
<IconBase ref={ref} {...props} weights={weights} />
));
CustomIcon.displayName = "CustomIcon";
export default CustomIcon;
[!NOTE] If you have multiple child elements, wrap them in a
Fragment
. Typically ourduotone
icons have multiple elements, with the background layer at 20% opacity.
If Custom Icons are intended to be used in React Server Components, use <SSRBase />
instead of <IconBase />
as the render component.
If you've made a port of Phosphor and you want to see it here, just open a PR here!
MIT © Phosphor Icons
FAQs
A clean and friendly icon family for React
The npm package @phosphor-icons/react receives a total of 216,531 weekly downloads. As such, @phosphor-icons/react popularity was classified as popular.
We found that @phosphor-icons/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.