@poppinss/cliui
Advanced tools
Comparing version 1.1.0 to 2.0.0
@@ -68,6 +68,5 @@ import { Logger } from '../Logger'; | ||
colors: boolean; | ||
iconColors: boolean; | ||
icons: boolean; | ||
labelColors: boolean; | ||
dim: boolean; | ||
dimIcons: boolean; | ||
dimLabels: boolean; | ||
interactive: boolean; | ||
@@ -74,0 +73,0 @@ }; |
@@ -82,3 +82,3 @@ "use strict"; | ||
dim(text) { | ||
return this.colors.gray(text); | ||
return this.colors.dim(text); | ||
} | ||
@@ -85,0 +85,0 @@ /** |
@@ -18,2 +18,3 @@ import { Logger } from '../index'; | ||
private getLabel; | ||
private formatMessage; | ||
/** | ||
@@ -20,0 +21,0 @@ * Define a custom renderer. Logs to "stdout" and "stderr" |
@@ -29,6 +29,12 @@ "use strict"; | ||
if (!this.logger.options.colors) { | ||
return `[${label}]`; | ||
return `${label.toUpperCase()}:`; | ||
} | ||
return this.logger.colors.underline()[color](label); | ||
return `${this.logger.colors[color](`${label.toUpperCase()}:`)}`; | ||
} | ||
formatMessage(message) { | ||
if (this.logger.options.dim) { | ||
return this.logger.colors.dim(message); | ||
} | ||
return message; | ||
} | ||
/** | ||
@@ -47,3 +53,3 @@ * Define a custom renderer. Logs to "stdout" and "stderr" | ||
const label = this.getLabel(this.label, 'green'); | ||
this.logger.success(`${label} ${message}`); | ||
this.logger.log(this.formatMessage(`${label} ${message}`)); | ||
} | ||
@@ -54,7 +60,7 @@ /** | ||
skipped(message, skipReason) { | ||
let logMessage = `${this.getLabel('skip', 'cyan')} ${message}`; | ||
let logMessage = this.formatMessage(`${this.getLabel('skip', 'cyan')} ${message}`); | ||
if (skipReason) { | ||
logMessage = `${logMessage} ${this.logger.colors.dim(`(${skipReason})`)}`; | ||
} | ||
this.logger.debug(logMessage); | ||
this.logger.log(logMessage); | ||
} | ||
@@ -65,6 +71,6 @@ /** | ||
failed(message, errorMessage) { | ||
const label = this.getLabel(this.label, 'red'); | ||
this.logger.error(`${label} ${message} ${this.logger.colors.dim(`(${errorMessage})`)}`); | ||
let logMessage = this.formatMessage(`${this.getLabel('error', 'red')} ${message}`); | ||
this.logger.logError(`${logMessage} ${this.logger.colors.dim(`(${errorMessage})`)}`); | ||
} | ||
} | ||
exports.Action = Action; |
@@ -20,5 +20,5 @@ import { Action } from './Action'; | ||
/** | ||
* The icon colors reference | ||
* The label colors reference | ||
*/ | ||
private iconColors; | ||
private labelColors; | ||
/** | ||
@@ -30,9 +30,9 @@ * The renderer to use to output logs | ||
/** | ||
* Colors the icon | ||
* Colors the logger label | ||
*/ | ||
private colorizeIcon; | ||
private colorizeLabel; | ||
/** | ||
* Returns the icon for a given logging type | ||
* Returns the label for a given logging type | ||
*/ | ||
private getIcon; | ||
private getLabel; | ||
/** | ||
@@ -50,3 +50,3 @@ * Appends the suffix to the message | ||
*/ | ||
private prefixIcon; | ||
private prefixLabel; | ||
/** | ||
@@ -53,0 +53,0 @@ * Decorate message string |
@@ -12,3 +12,2 @@ "use strict"; | ||
exports.Logger = void 0; | ||
const Icons_1 = require("../Icons"); | ||
const Action_1 = require("./Action"); | ||
@@ -22,7 +21,6 @@ const Colors_1 = require("../Colors"); | ||
const DEFAULTS = { | ||
icons: true, | ||
dim: false, | ||
dimIcons: false, | ||
dimLabels: false, | ||
colors: true, | ||
iconColors: true, | ||
labelColors: true, | ||
interactive: true, | ||
@@ -39,31 +37,31 @@ }; | ||
this.colors = Colors_1.getBest(this.testing, this.options.colors); | ||
this.iconColors = Colors_1.getBest(this.testing, this.options.iconColors && this.options.colors); | ||
this.labelColors = Colors_1.getBest(this.testing, this.options.labelColors && this.options.colors); | ||
} | ||
/** | ||
* Colors the icon | ||
* Colors the logger label | ||
*/ | ||
colorizeIcon(color, figure) { | ||
if (this.options.dim || this.options.dimIcons) { | ||
return this.iconColors.dim()[color](figure); | ||
colorizeLabel(color, text) { | ||
if (this.options.dim || this.options.dimLabels) { | ||
return `[ ${this.labelColors.dim()[color](text)} ]`; | ||
} | ||
return this.iconColors[color](figure); | ||
return `[ ${this.labelColors[color](text)} ]`; | ||
} | ||
/** | ||
* Returns the icon for a given logging type | ||
* Returns the label for a given logging type | ||
*/ | ||
getIcon(type) { | ||
getLabel(type) { | ||
switch (type) { | ||
case 'success': | ||
return this.colorizeIcon('green', Icons_1.icons.tick); | ||
return this.colorizeLabel('green', 'success'); | ||
case 'error': | ||
case 'fatal': | ||
return this.colorizeIcon('red', Icons_1.icons.cross); | ||
return this.colorizeLabel('red', type); | ||
case 'warning': | ||
return this.colorizeIcon('yellow', Icons_1.icons.warning); | ||
return this.colorizeLabel('yellow', 'warn'); | ||
case 'info': | ||
return this.colorizeIcon('blue', Icons_1.icons.info); | ||
return this.colorizeLabel('blue', 'info'); | ||
case 'debug': | ||
return this.colorizeIcon('cyan', Icons_1.icons.bullet); | ||
return this.colorizeLabel('cyan', 'debug'); | ||
case 'await': | ||
return this.colorizeIcon('yellow', Icons_1.icons.squareSmallFilled); | ||
return this.colorizeLabel('cyan', 'wait'); | ||
} | ||
@@ -89,3 +87,3 @@ } | ||
prefix = prefix.replace(/%time%/, new Date().toISOString()); | ||
return `${this.colors.gray(`[${prefix}]`)} ${message}`; | ||
return `${this.colors.dim(`[${prefix}]`)} ${message}`; | ||
} | ||
@@ -95,7 +93,4 @@ /** | ||
*/ | ||
prefixIcon(message, icon) { | ||
if (!this.options.icons) { | ||
return message; | ||
} | ||
return `${icon} ${message}`; | ||
prefixLabel(message, label) { | ||
return `${label} ${message}`; | ||
} | ||
@@ -174,3 +169,3 @@ /** | ||
message = this.decorateMessage(message); | ||
message = this.prefixIcon(message, this.getIcon('success')); | ||
message = this.prefixLabel(message, this.getLabel('success')); | ||
message = this.addPrefix(message, prefix); | ||
@@ -186,3 +181,3 @@ message = this.addSuffix(message, suffix); | ||
message = this.decorateMessage(message); | ||
message = this.prefixIcon(message, this.getIcon('error')); | ||
message = this.prefixLabel(message, this.getLabel('error')); | ||
message = this.addPrefix(message, prefix); | ||
@@ -199,3 +194,3 @@ message = this.addSuffix(message, suffix); | ||
message = this.decorateMessage(message); | ||
message = this.prefixIcon(message, this.getIcon('error')); | ||
message = this.prefixLabel(message, this.getLabel('error')); | ||
message = this.addPrefix(message, prefix); | ||
@@ -210,3 +205,3 @@ message = this.addSuffix(message, suffix); | ||
message = this.decorateMessage(message); | ||
message = this.prefixIcon(message, this.getIcon('warning')); | ||
message = this.prefixLabel(message, this.getLabel('warning')); | ||
message = this.addPrefix(message, prefix); | ||
@@ -221,3 +216,3 @@ message = this.addSuffix(message, suffix); | ||
message = this.decorateMessage(message); | ||
message = this.prefixIcon(message, this.getIcon('info')); | ||
message = this.prefixLabel(message, this.getLabel('info')); | ||
message = this.addPrefix(message, prefix); | ||
@@ -232,3 +227,3 @@ message = this.addSuffix(message, suffix); | ||
message = this.decorateMessage(message); | ||
message = this.prefixIcon(message, this.getIcon('debug')); | ||
message = this.prefixLabel(message, this.getLabel('debug')); | ||
message = this.addPrefix(message, prefix); | ||
@@ -248,3 +243,3 @@ message = this.addSuffix(message, suffix); | ||
text = this.logger.decorateMessage(text); | ||
text = this.logger.prefixIcon(text, this.logger.getIcon('await')); | ||
text = this.logger.prefixLabel(text, this.logger.getLabel('await')); | ||
text = this.logger.addPrefix(text, this.prefix); | ||
@@ -251,0 +246,0 @@ text = this.logger.addSuffix(text, this.suffix); |
@@ -86,3 +86,3 @@ "use strict"; | ||
head: this.state.head, | ||
style: { head: [] }, | ||
style: { head: [], border: ['dim'] }, | ||
...(this.state.colWidths ? { colWidths: this.state.colWidths } : {}), | ||
@@ -89,0 +89,0 @@ }); |
{ | ||
"name": "@poppinss/cliui", | ||
"version": "1.1.0", | ||
"version": "2.0.0", | ||
"description": "Highly opinionated command line UI KIT", | ||
@@ -37,3 +37,3 @@ "main": "build/index.js", | ||
"@adonisjs/mrm-preset": "^2.4.0", | ||
"@adonisjs/require-ts": "^1.0.0", | ||
"@adonisjs/require-ts": "^1.0.3", | ||
"@types/node": "^14.11.2", | ||
@@ -40,0 +40,0 @@ "commitizen": "^4.2.1", |
@@ -1,2 +0,2 @@ | ||
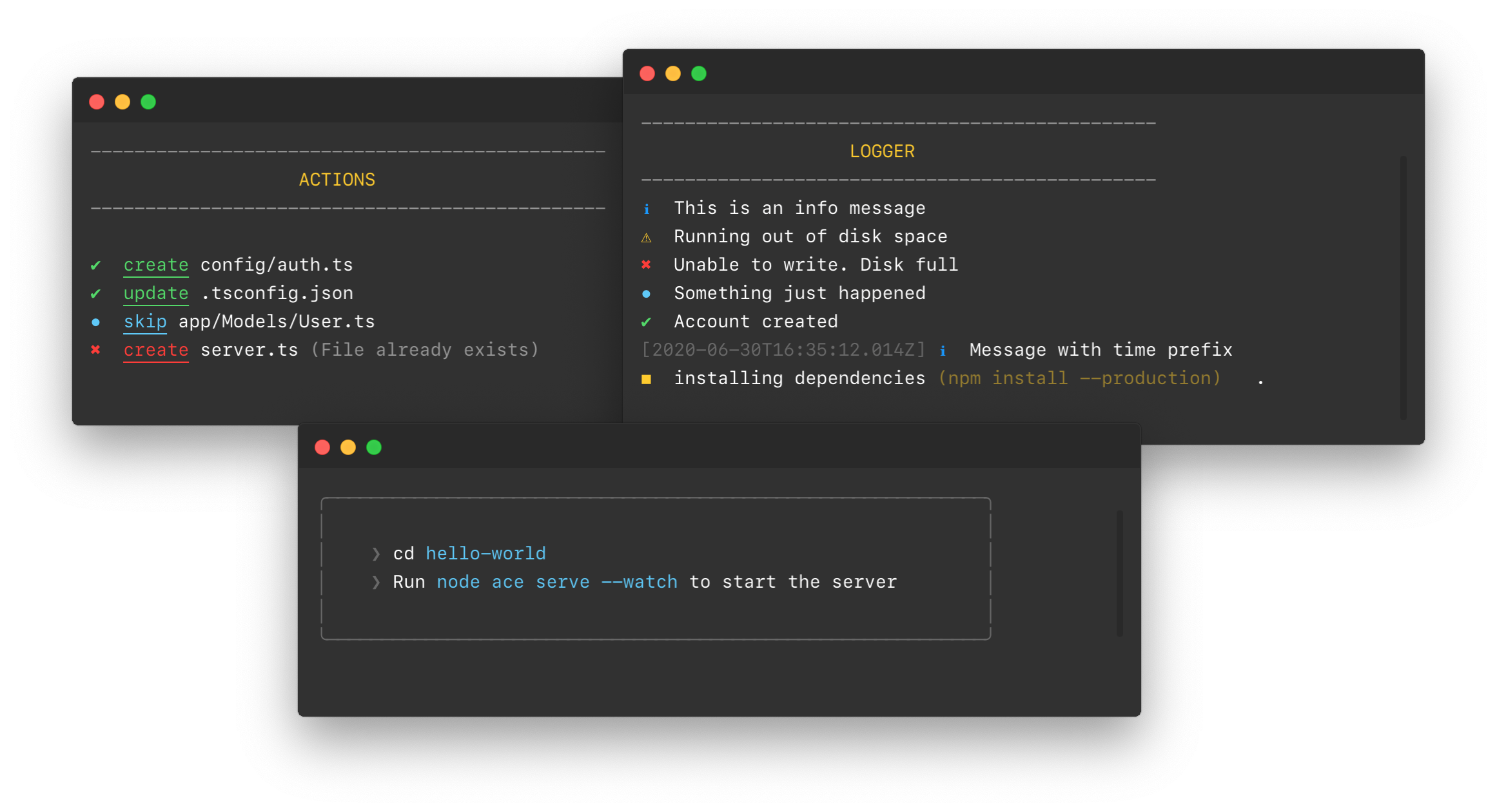 | ||
 | ||
@@ -17,3 +17,2 @@ # CLI UI | ||
- [Why AdonisJS needs a CLI UI Kit?](#why-adonisjs-needs-a-cli-ui-kit) | ||
- [Installation](#installation) | ||
@@ -44,6 +43,2 @@ - [Usage](#usage) | ||
## Why AdonisJS needs a CLI UI Kit? | ||
Read this official blog post to learn more about it. | ||
## Installation | ||
@@ -79,3 +74,3 @@ | ||
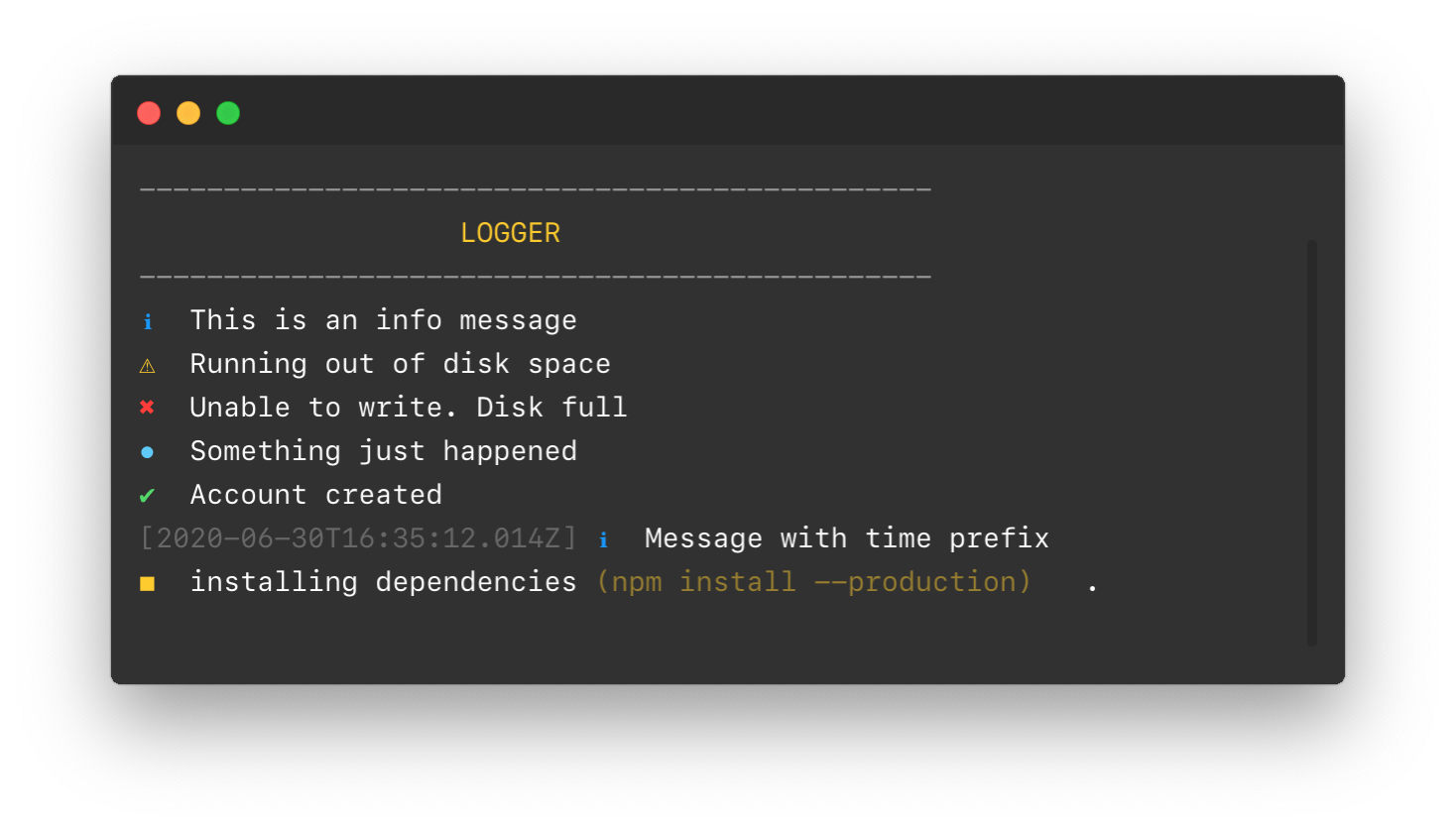 | ||
 | ||
@@ -91,3 +86,3 @@ The logger exposes the following methods. | ||
// ✔ Account created | ||
// [ success ] Account created | ||
``` | ||
@@ -100,3 +95,3 @@ | ||
// [ap-south-1] ✔ Account created | ||
// [ap-south-1] [ success ] Account created | ||
``` | ||
@@ -109,3 +104,3 @@ | ||
// ✔ Account created (ap-south-1) | ||
// [ success ] Account created (ap-south-1) | ||
``` | ||
@@ -125,3 +120,3 @@ | ||
// ✖ Unable to write. Disk full | ||
// [ error ] Unable to write. Disk full | ||
``` | ||
@@ -137,3 +132,3 @@ | ||
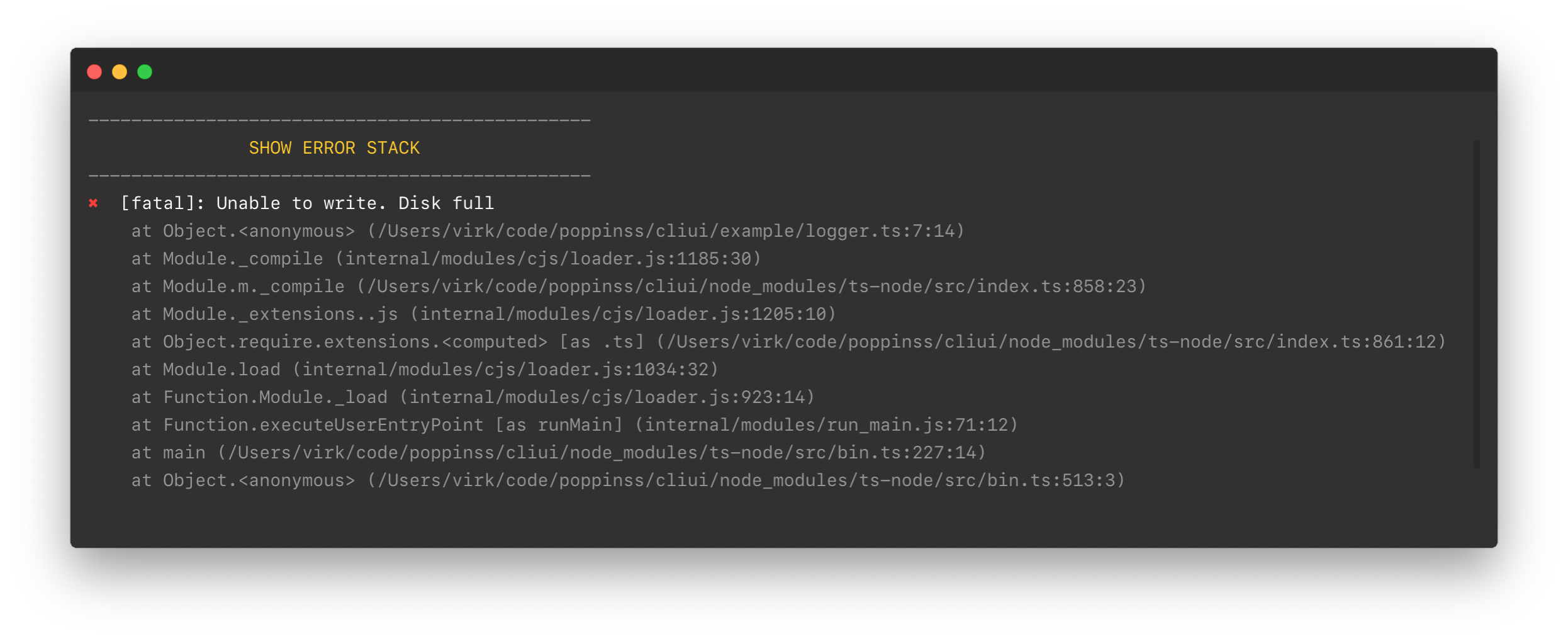 | ||
 | ||
@@ -147,3 +142,3 @@ ### `warning(message, prefix?, suffix?)` | ||
// ⚠ Running out of disk space | ||
// [ warn ] Running out of disk space | ||
``` | ||
@@ -158,3 +153,3 @@ | ||
// ℹ Your account is has been updated | ||
// [ info ] Your account is has been updated | ||
``` | ||
@@ -169,3 +164,3 @@ | ||
// ● Something just happened | ||
// [ debug ] Something just happened | ||
``` | ||
@@ -219,7 +214,7 @@ | ||
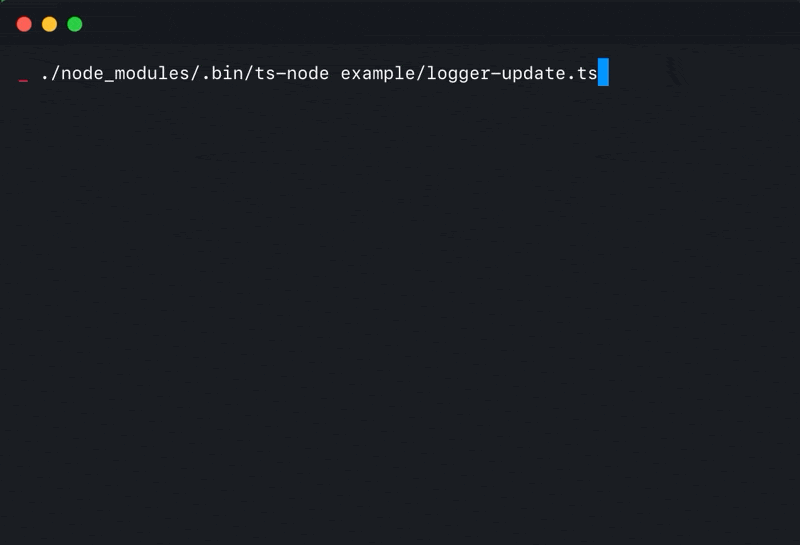 | ||
 | ||
## Action | ||
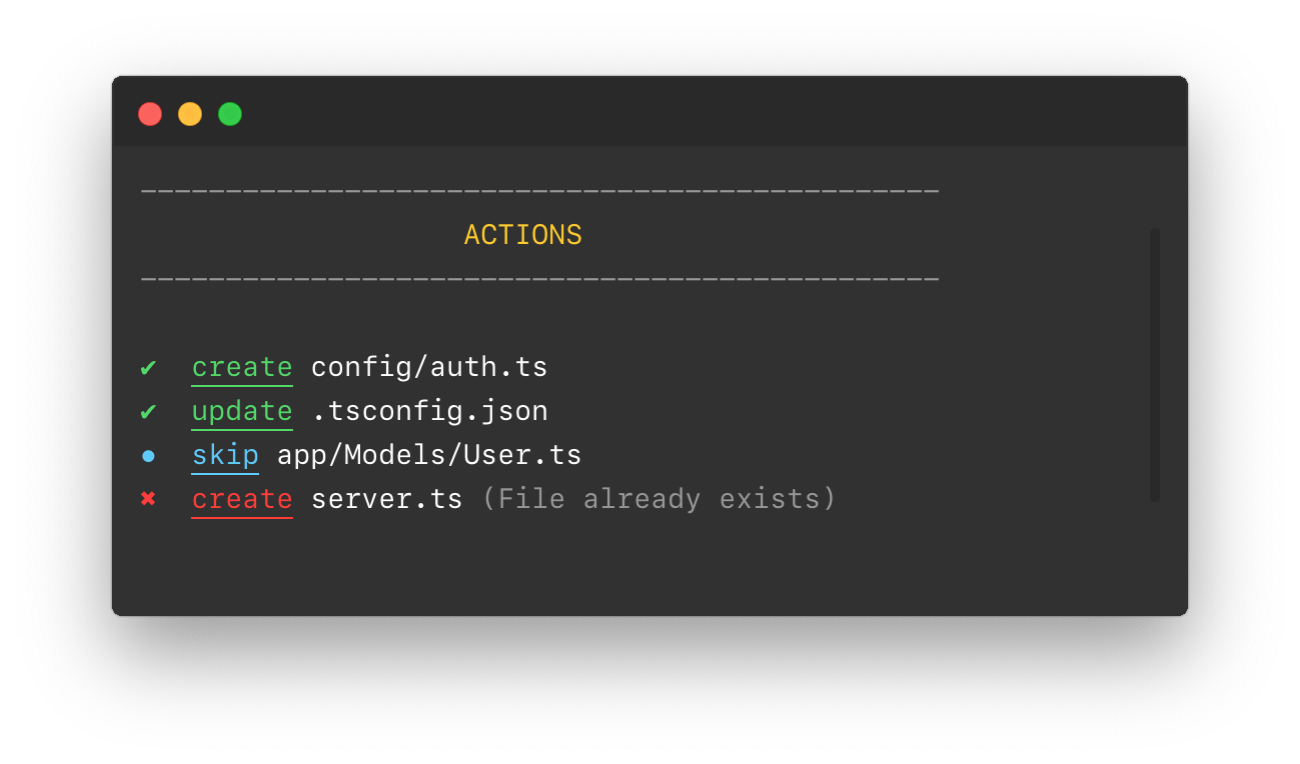 | ||
 | ||
@@ -264,3 +259,3 @@ In order to log results of an action/task, we make use of the `action` method. | ||
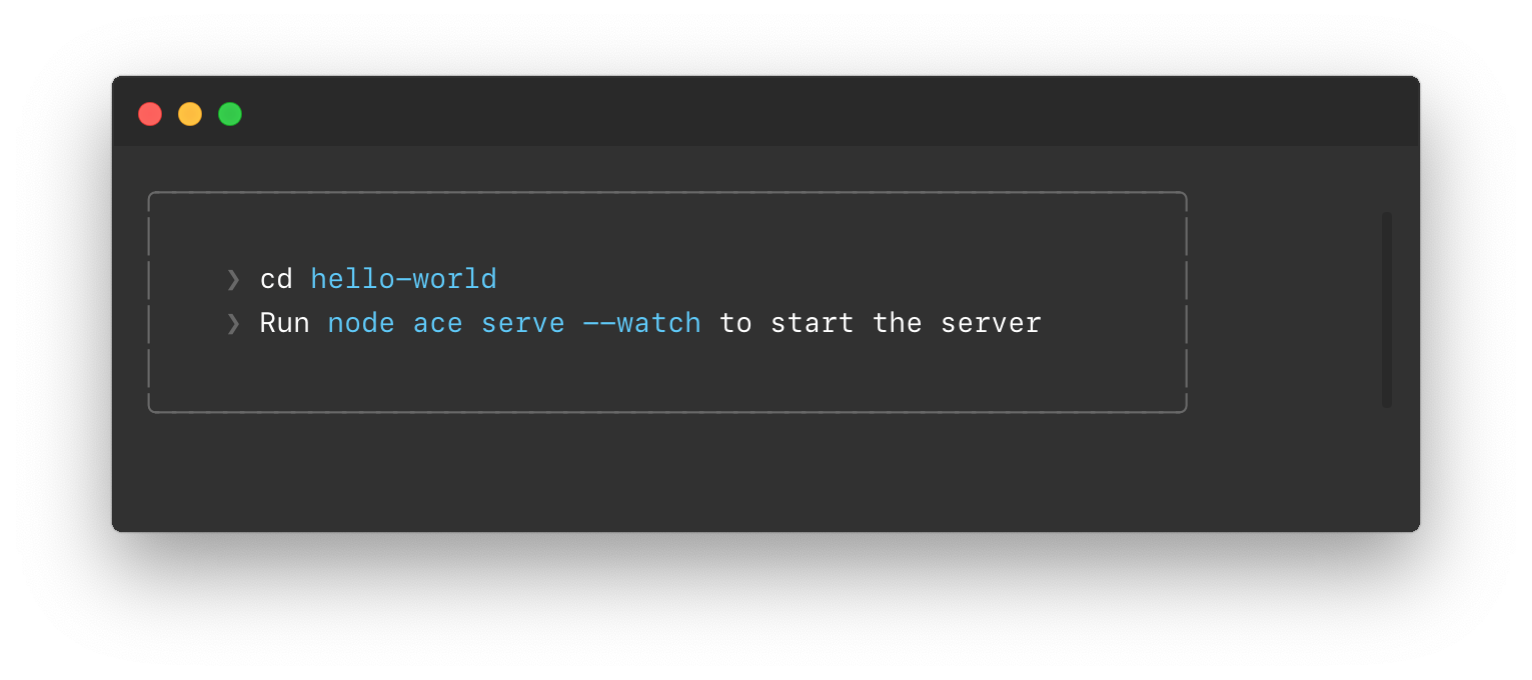 | ||
 | ||
@@ -287,3 +282,3 @@ Instructions are mainly the steps we want someone to perform in order to achieve something. For example: | ||
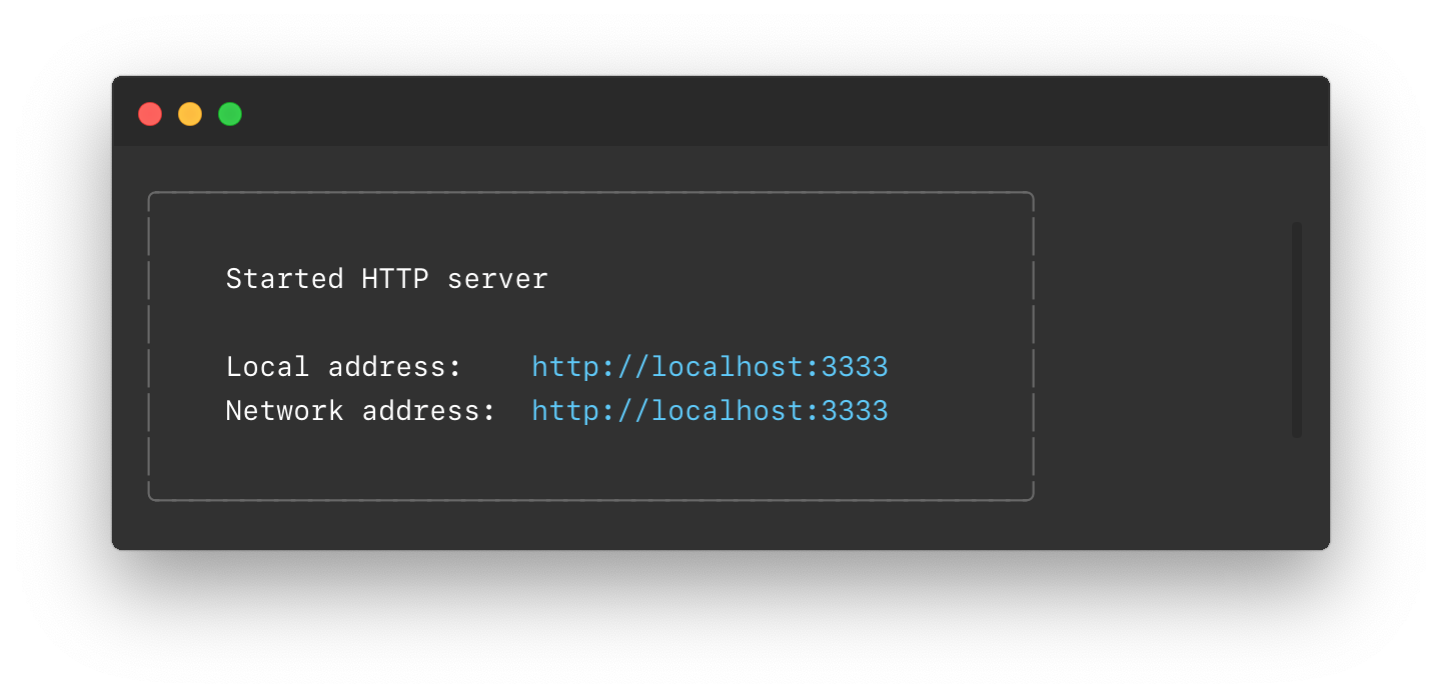 | ||
 | ||
@@ -356,3 +351,3 @@ Similar to the **instructions**, but a sticker does not prefix the lines with a pointer `>` arrow. Rest is all same. | ||
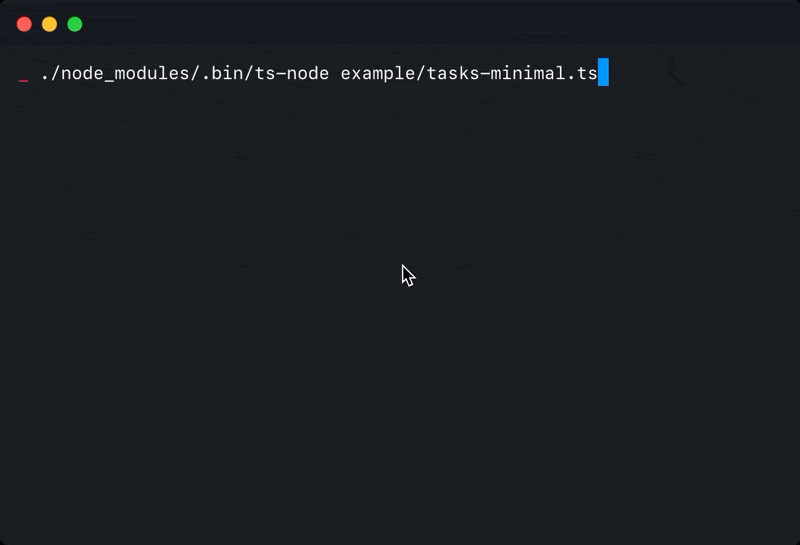 | ||
 | ||
@@ -367,3 +362,3 @@ ### Verbose renderer | ||
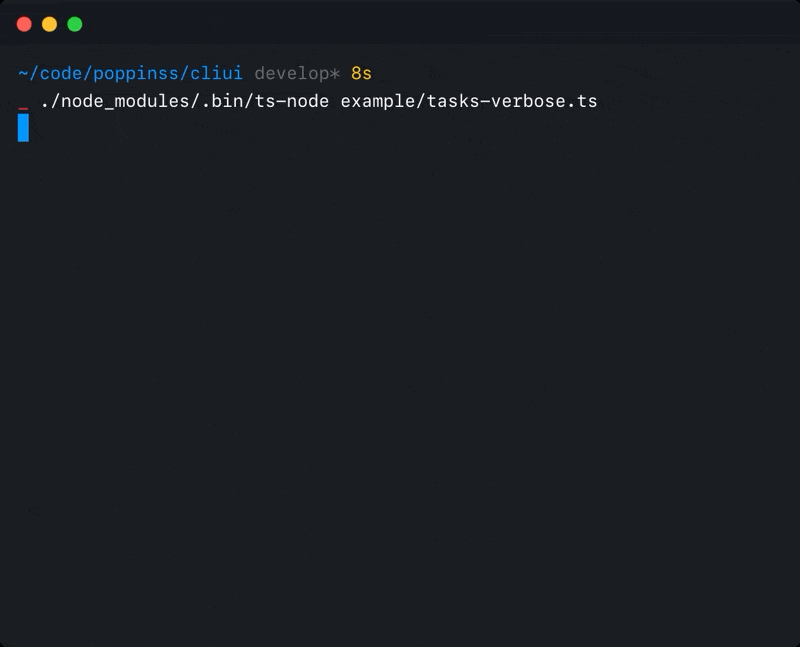 | ||
 | ||
@@ -370,0 +365,0 @@ [circleci-image]: https://img.shields.io/circleci/project/github/poppinss/cliui/master.svg?style=for-the-badge&logo=circleci |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
2301
78670
359