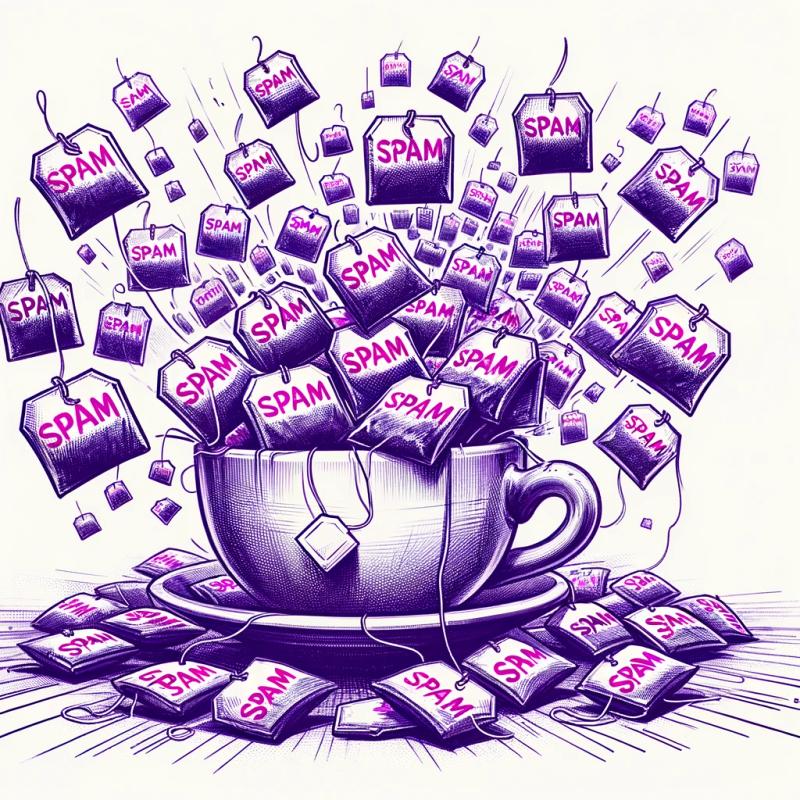
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@prince-obrymec/swipe-events-manager
Advanced tools
Readme
This Node Package Manager (NPM) is a front-end library for managing touch screen events on mobile devices. It provides a support for Javascript and Typescript.
An example of use case of the package is already hosted on web and
can be accessible through one of these links below :
The package sources can be found via one these links below:
This is the final result of the project :
We can install the module via npm
, pnpm
or yarn
. For npm
,
use the command line below :
npm install @prince-obrymec/swipe-events-manager --save-dev
For pnpm
, use the command line below :
pnpm install @prince-obrymec/swipe-events-manager --save-dev
For yarn
, use the command line below :
yarn add @prince-obrymec/swipe-events-manager --save-dev
We can also get the CDN link there :
<!--CDN links for ES6 support-->
<script
src = "https://CodiTheck.github.io/swipe_events_manager/build/swipe.min.js"
type = "text/javascript"
></script>
<script
src = "https://CodiTheck.github.io/swipe_events_manager/build/swipe.js"
type = "text/javascript"
></script>
<!--CDN links for CJS support-->
<script
src = "https://CodiTheck.github.io/swipe_events_manager/build/cjs/swipe.min.js"
type = "text/javascript"
></script>
<script
src = "https://CodiTheck.github.io/swipe_events_manager/build/cjs/swipe.js"
type = "text/javascript"
></script>
Before listen any swipe event, we must create an object instance of that. Of course, we must also import the module before any usage.
// Package importation.
import {SwipeEventsManager} from "@prince-obrymec/swipe-events-manager";
// We can create a swipe manager with a tag
// reference.
const swipeManager1 = new SwipeEventsManager (myTagRef);
// We can also create a swipe manager with
// a tag id, class etc...
const swipeManager2 = new SwipeEventsManager ("#my-tag");
Notice that when we creating a swipe manager, we can pass two arguments to that object. The first is the tag where swipe events will be listened and the second is a callback method that is triggered when any swipe event is detected on the selected markup. The callback fetch the swipe direction for you. Only first argument is required when we creating an object instance of a swipe events manager.
Now we can rewrite the code above like this :
// Package importation.
import {SwipeEventsManager} from "@prince-obrymec/swipe-events-manager";
// We can create a swipe manager with a tag
// id, class, etc... and a callback method
// to listen upcoming swipe events on the
// selected tag.
const swipeManager = new SwipeEventsManager (
"#my-tag", function (direction) {
/** ... */
}
);
We can know the swipe's direction thank the fetched direction from our callback. The direction is a constant with the following values :
Finally the full source code will be :
// Package importation.
import {
SwipeEventsManager,
SwipeEventType
} from "@prince-obrymec/swipe-events-manager";
// We can create a swipe manager with a tag
// id, class, etc... and a callback method
// to listen upcoming swipe events on the
// selected tag.
const swipeManager = new SwipeEventsManager (
"#my-tag", function (direction) {
// The selected tag's name.
const tagName = swipeManager.getTag ().tagName;
// Whether the direction is right.
if (direction === SwipeEventType.SWIPE_RIGHT) {
// Makes a warn on the browser.
window.alert (`SWIPE RIGHT DETECTED: ${tagName}`);
}
// Whether the direction is bottom.
else if (direction === SwipeEventType.SWIPE_DOWN) {
// Makes a warn on the browser.
window.alert (`SWIPE DOWN DETECTED: ${tagName}`);
}
// Whether the direction is left.
else if (direction === SwipeEventType.SWIPE_LEFT) {
// Makes a warn on the browser.
window.alert (`SWIPE LEFT DETECTED: ${tagName}`);
}
// Whether the direction is top.
else if (direction === SwipeEventType.SWIPE_UP) {
// Makes a warn on the browser.
window.alert (`SWIPE UP DETECTED: ${tagName}`);
}
}
);
Another way to do that is to use listen
method provided
by the swipe events manager directly. But use this method
if and only if you don't provide a callback when you
creating a swipe events manager. The code below show
you a use case :
// Package importation.
import {
SwipeEventsManager,
SwipeEventType
} from "@prince-obrymec/swipe-events-manager";
// We can create a swipe manager with a tag
// reference.
const swipeManager = new SwipeEventsManager (
myTagRef
);
// Listens swipe directions.
swipeManager.listen (function (direction) {
// Whether the direction is right.
if (direction === SwipeEventType.SWIPE_RIGHT) {
// Makes a warn on the browser.
window.alert ("SWIPE RIGHT DETECTED !");
}
// Whether the direction is bottom.
else if (direction === SwipeEventType.SWIPE_DOWN) {
// Makes a warn on the browser.
window.alert ("SWIPE DOWN DETECTED !");
}
// Whether the direction is left.
else if (direction === SwipeEventType.SWIPE_LEFT) {
// Makes a warn on the browser.
window.alert ("SWIPE LEFT DETECTED !");
}
// Whether the direction is top.
else if (direction === SwipeEventType.SWIPE_UP) {
// Makes a warn on the browser.
window.alert ("SWIPE UP DETECTED !");
}
});
The package offers also some additional that are :
// Package importation.
import {SwipeEventsManager} from "@prince-obrymec/swipe-events-manager";
// The old tag reference.
const oldTag = document.querySelector ("#old-tag");
// The new tag reference.
const newTag = document.querySelector ("#new-tag");
// Creating a new object instance of a swipe events manager.
const swipeManager = new SwipeEventsManager (oldTag);
// Returns the current selected tag reference on the manager.
console.log (swipeManager.getTag ());
// Overrides the current selected tag to another. Notice
// that old tag events are destroyed before go to the
// new tag.
swipeManager.setTag (newTag);
// We could also set the active tag by giving the id,
// class, etc... of the new tag directly.
swipeManager.setTag ("#new-tag");
// We can destroy all listened swipe events on the
// active markup.
swipeManager.free ();
If you want to get package sources code, make sure to have NodeJs already installed in your machine. If it isn't the case, you can install NodeJs through the command lines below :
cd ~;\
sudo apt install curl;\
curl https://raw.githubusercontent.com/creationix/nvm/master/install.sh | bash;\
source ~/.bashrc;\
nvm --version;\
nvm install --lts;\
node --version;\
npm install yarn --global;\
yarn --version
git clone git@github.com:CodiTheck/swipe_events_manager.git swipe_events_manager/
Go to the root folder of the project sources and run :
yarn install
Go to the root folder of the project and run :
yarn start
Then, open your favorite browser and tap on the search bar, the following link :
http://localhost:1234/
Enjoy :)
FAQs
A front-end library for managing touch screen events on mobile devices.
The npm package @prince-obrymec/swipe-events-manager receives a total of 3 weekly downloads. As such, @prince-obrymec/swipe-events-manager popularity was classified as not popular.
We found that @prince-obrymec/swipe-events-manager demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.