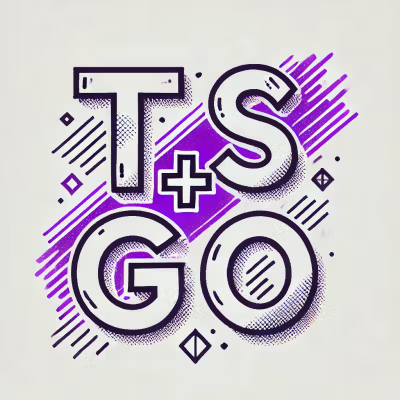
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@pryv/boiler
Advanced tools
Logging and config boilerplate library for Node.js apps and services at Pryv
The "boiler" must be initialized with the application name and configuration files settings, before invoking getConfig()
or getLogger()
:
require('@pryv/boiler').init({
appName: 'my-app', // This will will be prefixed to any log messages
baseFilesDir: path.resolve(__dirname, '..'), // use for file:// relative path if not give cwd() will be used
baseConfigDir: path.resolve(__dirname, '../config'),
extraConfigs: [{
scope: 'extra-config',
file: path.resolve(__dirname, '../config/extras.js')
}]
});
We use nconf.
During initialization default-config.yml
and ${NODE_ENV}-config.yml
will be loaded if present in baseConfigDir
.
The order configuration sources are loaded is important. Each source is loaded with a "scope" name, which can be used to replace the source's contents at any point of time.
The configuration sources are loaded in the following order, the first taking precedence:
${NODE_ENV}-config.yml
(if present) or specified by the --config
command line argumentextraConfigs
option (see below)${baseConfigDir}/default-config.yml
Additional configuration sources can be set or reserved at initialization with the extraConfigs
option, which expects an array of objects with one of the following structures:
{scope: <name>, file: <path to file> }
. Accepts .yml
, .json
and .js
files. Note .js
content is loaded with require(<path to file>)
.{scope: <name>, key: <optional key>, data: <object> }
. If key
is provided, the content of data
will be accessible by this key, otherwise it is loaded at the root of the configuration.{scope: <name>, key: <optional key>, url: <URL to json content> }
. The JSON contents of this URL will be loaded asynchronously.{scope: <name>, key: <optional key>, urlFromKey: <key> }
. Similar to remove URL, with the URL obtained from an existing configuration key.Retrieving the configuration object:
// synchronous loading
const { getConfigUnsafe } = require('@pryv/boiler'); // Until all asynchronous sources such as URL are loaded, items might not be available
const config = await getConfigUnsafe();
// asynchronous loading
const { getConfig } = require('@pryv/boiler');
const config = await getConfig(); // Here we can be sure all items are fully loaded
Retrieving settings:
// configuration content is {foo: { bar: 'hello'}};
const foo = config.get('foo'); // {bar: 'hello'}
const bar = config.get('foo:bar'); // 'hello'
const barExists = config.has('bar'); // true
Assigning settings:
// configuration content is {foo: { bar: 'hello'}};
config.set('foo', 'bye bye'); // {bar: 'hello'}
const foo = config.get('foo'); // 'bye bye'
Changing a scope's contents:
// configuration content is {foo: { bar: 'hello'}};
config.get('foo'); // {bar: 'hello'}
// replace 'test' existing content (test is always present as the topmost configuration source)
config.replaceScopeConfig('test', {foo: 'test'});
config.get('foo'); // 'test'
// reset content of 'test' scope
config.replaceScopeConfig('test', {});
config.get('foo'); // {bar: 'hello'}
// Note: for 'test' scope there is a "sugar" function with config.injectTestConfig(object)
Finding out from which scope a key applies: As nconf is hierachical sometimes you migth want to search from which scope the value of a key is issued.
config.getScopeAndValue('foo');
// returns {value: 'bar', scope: 'scopeName'; info: 'From <file> or Type <env, '}
To help detect unused configuration settings, a "learn" mode can be activated to track all calls to config.get()
in files.
Example when running tests:
export CONFIG_LEARN_DIR="{absolute path}/service-core/learn-config"
yarn test
Note, if CONFIG_LEARN_DIR is not given {process.cwd()}/learn-config
will be used
All messages are prefixed by the appName
value provided at initialization (see above)). appName
can be postfixed with a string by setting the environment variable PRYV_BOILER_SUFFIX
, which is useful when spawning several concurrent processes of the same application.
const {getLogger} = require('@pryv/boiler');
logger.info('Message', item); // standard log
logger.warn('Message', item); // warning
logger.error('Message', item); // warning
logger.debug('Message', item); // debug
logger.getLogger('sub'); // new logger name spaced with parent, here '{appName}:sub'
logger.info()
, logger.warn()
and logger.error()
use Winston logger.debug()
is based on debug.
Set the DEBUG
environment variable. For example: DEBUG="*" node app.js
will output all debug messages.
As "debug" is a widely used package, you might get way more debug lines than expected, so you can use the appName
property to only output messages from your application code: DEBUG="<appName>*" node app.js
A custom logger can be used by providing logs:custom
information to the configuration. A working sample of custom Logger is provided in ./examples/customLogger
.
The module must implement async init(settings)
and log(level, key, message, meta)
logs: {
console: {
active: true,
level: 'info',
format: {
color: true,
time: true,
aligned: true
}
},
file: {
active: true,
path: 'application.log'
},
custom: {
active: true,
path 'path/to/node/package',
settings: { /* settings passed to the custom logger */}
}
}
npm run lint
lints the code with Semi-Standard.
npm run license
updates license information.
FAQs
Logging and config boilerplate library for Node.js apps and services at Pryv
The npm package @pryv/boiler receives a total of 2 weekly downloads. As such, @pryv/boiler popularity was classified as not popular.
We found that @pryv/boiler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.