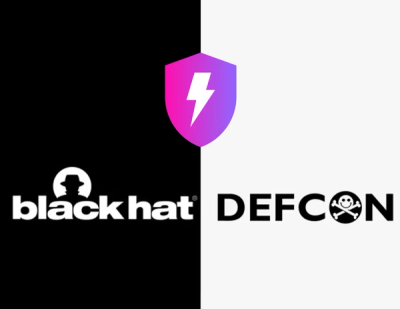
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
@react-pdf/textkit
Advanced tools
@react-pdf/textkit is a low-level text layout engine for React PDF. It provides tools for text shaping, line breaking, and text rendering, making it easier to handle complex text layouts in PDF documents.
Text Shaping
Text shaping involves converting a string of text into a series of positioned glyphs. This is essential for rendering text accurately in PDFs.
const Textkit = require('@react-pdf/textkit');
const font = new Textkit.Font('path/to/font.ttf');
const glyphs = font.layout('Hello, world!');
console.log(glyphs);
Line Breaking
Line breaking is the process of dividing text into lines that fit within a given width. This is crucial for creating readable and well-formatted text blocks in PDFs.
const Textkit = require('@react-pdf/textkit');
const lineBreaker = new Textkit.LineBreaker();
const breaks = lineBreaker.break('This is a long text that needs to be broken into lines.', 100);
console.log(breaks);
Text Rendering
Text rendering involves drawing the shaped and positioned glyphs onto a canvas or PDF. This is the final step in displaying text in a PDF document.
const Textkit = require('@react-pdf/textkit');
const renderer = new Textkit.Renderer();
const text = 'Hello, world!';
const renderedText = renderer.render(text, { x: 0, y: 0 });
console.log(renderedText);
PDFKit is a JavaScript library for generating PDFs. It provides high-level APIs for text rendering, image embedding, and vector graphics. Compared to @react-pdf/textkit, PDFKit is more comprehensive but less specialized in text layout.
pdf-lib is a library for creating and modifying PDF documents in JavaScript. It offers functionalities for text rendering, form creation, and document manipulation. While it provides text rendering capabilities, it lacks the low-level text shaping and line breaking features of @react-pdf/textkit.
This project is a fork of textkit by @devongovett and continued under the scope of this project since it has react-pdf specific features. Any recongnition should go to him and the original project mantainers.
FAQs
An advanced text layout framework
The npm package @react-pdf/textkit receives a total of 855,033 weekly downloads. As such, @react-pdf/textkit popularity was classified as popular.
We found that @react-pdf/textkit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.