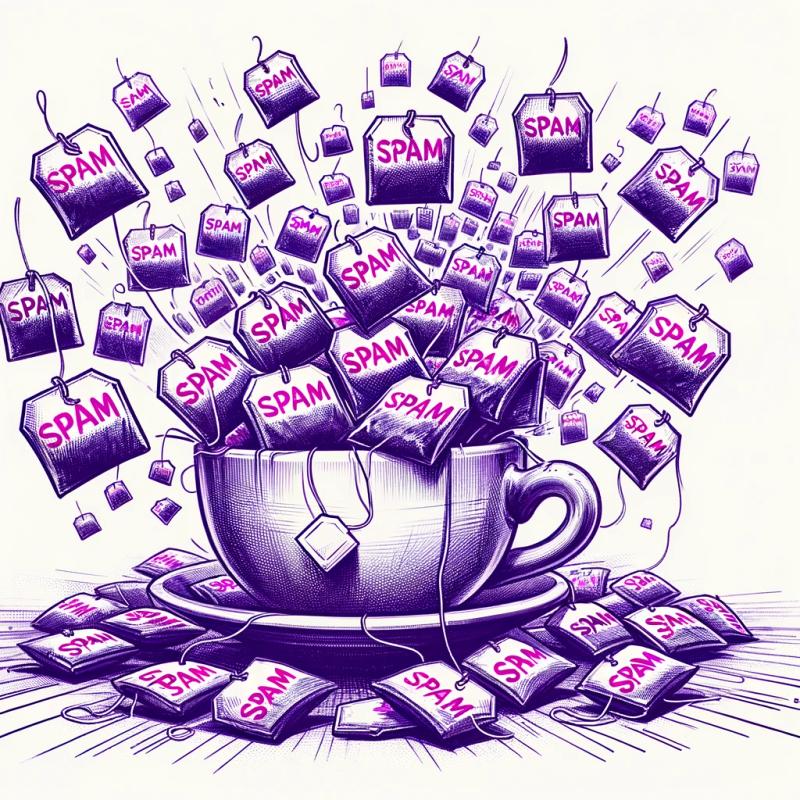
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@restyjs/core
Advanced tools
Readme
Fast, opinionated, minimalist and testable web framework built on top of express.js and typescript with decorators.
Install from the command line:
npm install @restyjs/core
enable following settings in tsconfig.json
:
"emitDecoratorMetadata": true,
"experimentalDecorators": true,
import resty, { Controller, Get, Context } from "@restyjs/core";
@Controller("/hello")
class HelloController {
@Get("/")
index() {
return "Hello World";
}
}
const app = resty({
controllers: [HelloController],
});
app.listen(8080);
// returns express application instance
const app = resty({
controllers: [],
});
resty always returns express application instance.
const app = resty({
routePrefix: "/api",
controllers: [],
});
inside resty you can pass your existing express app instance inside app
parameter.
const express_app = express()
const app = resty({
app: express_app,
controllers: [],
});
inside resty you can pass your existing express router inside router
parameter.
const router = express.Router()
const app = resty({
router: router,
controllers: [],
});
@Controller("/hello")
class HelloController {
@Get("/register")
async register(ctx: Context, @Body() input: UserDTO) {
// create record
const user = await createUser(input);
// return user record
return ctx.res.json({ user }).status(200);
}
}
Create a file HelloController.ts
.
@Controller("/hello")
class HelloController {
@Get("/")
index() {
return "Hello World";
}
@Get("/health")
health(ctx: Context) {
return ctx.res.json({ status: "ok" }).status(200);
}
}
@Controller("/")
export class UserController {
@Get("/users")
getAll() {
return "This action returns all users";
}
@Get("/users/:id")
getOne(@Param("id") id: number) {
return "This action returns user #" + id;
}
@Post("/users")
post(@Body() user: any) {
return "Saving user...";
}
@Put("/users/:id")
put(@Param("id") id: number, @Body() user: any) {
return "Updating a user...";
}
@Delete("/users/:id")
remove(@Param("id") id: number) {
return "Removing user...";
}
}
@Get("/health")
health(ctx: Context) {
return ctx.res.json({ status: "ok" }).status(200);
}
Application lavel middlewares like helmet
, cors
or body-parser
import cors from 'cors';
import helmet from 'helmet';
const app = resty({
routePrefix: "/api",
controllers: [HelloController],
middlewares: [cors(), helmet()]
});
const isAdmin = async (req: any, res: any, next: any) => {
if (!req.currentUser) {
return next(new HTTPError("Error in checking current user", 500));
}
if (req.currentUser.role != Role.Admin) {
return next(new HTTPError("Insufficient permission", 403));
}
return next();
};
@Controller("/admin", [isAdmin])
export class AdminController {
@Get("/")
async allUsers() {
const users = await getAllUsers();
return { users };
}
}
const isAdmin = async (req: any, res: any, next: any) => {
return next();
};
@Controller("/post")
export class AdminController {
@Get("/", [isAdmin])
async allUsers() {
const users = await getAllUsers();
return { users };
}
}
const app = resty({
routePrefix: "/api",
controllers: [HelloController],
postMiddlewares: []
});
// User postMiddlewares or use same old app.use form express.
app.use((req, res, next) => {
next();
});
resty provides inbuilt 404
and HTTP
errors and UnauthorizedError
Handling. if you want to implement your own error handling pass handleErrors: false
to app config.
install typeorm module
npm install @restyjs/typeorm
resty will create database connection directly when provided Database(options: ConnectionOptions)
inside providers: []
.
import resty from "@restyjs/core";
import { Database } from "@restyjs/typeorm";
const app = resty({
controllers: [],
providers: [
Database({
type: "sqlite",
database: "example.db",
entities: [],
}),
],
});
For more info please refer to typeorm docs regarding database connections parameters and other orm features.
Satish Babariya, satish.babariya@gmail.com
resty.js is available under the MIT license. See the LICENSE file for more info.
FAQs
A Node.js framework
The npm package @restyjs/core receives a total of 24 weekly downloads. As such, @restyjs/core popularity was classified as not popular.
We found that @restyjs/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.