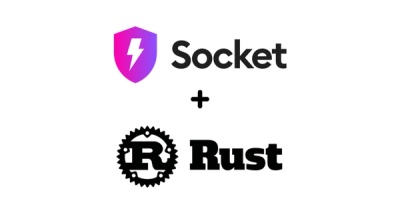
Product
Introducing Rust Support in Socket
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
@robinbobin/react-native-google-drive-api-wrapper
Advanced tools
This wrapper facilitates the use of the Google Drive API in React Native projects.
This wrapper facilitates the use of the Google Drive API v3.
It doesn't provide any authorization mechanism, so another package has to be used. I use @react-native-google-signin/google-signin (thanks for the great work, vonovak!).
If something doesn't work as expected, please do have a look at an example project before opening an issue.
npm i @robinbobin/react-native-google-drive-api-wrapper
// General setup
import { GoogleSignin } from '@react-native-google-signin/google-signin'
import { INFINITE_TIMEOUT, GDrive } from '@robinbobin/react-native-google-drive-api-wrapper'
...
// Somewhere in your code
GoogleSignin.configure(...)
await GoogleSignin.signIn()
const gdrive = new GDrive()
gdrive.accessToken = (await GoogleSignin.getTokens()).accessToken
// fetch() invocations wait infinitely
// gdrive.fetchTimeout = INFINITE_TIMEOUT
// List files
await gdrive.files.list(...)
// List files in `appDataFolder`
import { APP_DATA_FOLDER_ID } from '@robinbobin/react-native-google-drive-api-wrapper'
...
await gdrive.files.list({ ..., spaces: APP_DATA_FOLDER_ID })
// Create a binary file and read it
import { MIME_TYPES } from '@robinbobin/react-native-google-drive-api-wrapper'
...
const file = await gdrive.files.newMultipartUploader()
.setData([1, 2, 3, 4, 5])
.setDataMimeType(MIME_TYPES.application.octetStream)
.setRequestBody({ name: "multipart_bin" })
.execute()
console.log(await gdrive.files.getBinary(file.id))
Notes:
STANDARD_PARAMETERS_FIELDS_ALL
can be used instead of *
as a value for fields
in queryParameters
of all methods:
import { STANDARD_PARAMETERS_FIELDS_ALL } from '@robinbobin/react-native-google-drive-api-wrapper'
...
await gdrive.about.get(STANDARD_PARAMETERS_FIELDS_ALL)
Query parameters of certain methods have string properties containing a comma-separated list of some values (e.g. spaces
in list
). This wrapper accepts string
and string[]
values for these properties. string
values are passed as-is, and string[]
values are converted to comma-separated lists.
This class gives information about the user, the user's Drive, and system capabilities.
Name | Description |
---|---|
get() | Gets information about the user, the user's Drive, and system capabilities. Parameters:
|
This class is used to manage files in a google drive.
Notes:
The parameter range
for the methods that accept it is specified as here with one exception:
<unit> is always bytes
and mustn't be set. E.g.:
await gdrive.files.getBinary('bin_file_id', { range: '1-1' })
will return the byte at index one.
ROOT_FOLDER_ID
can be used instead of 'root'
:
import { ROOT_FOLDER_ID } from '@robinbobin/react-native-google-drive-api-wrapper'
Name | Description |
---|---|
copy() | Creates a copy of a file. Parameters:
|
createIfNotExists() | Conditionally creates a file resource. Parameters:
|
delete() | Deletes a file without moving it to the trash. Parameters:
|
emptyTrash() | Permanently deletes all of the user's trashed files. Returns:
|
export() | Exports a Google Doc to the requested MIME type. Parameters:
|
generateIds() | Generates file IDs. This info might seem interesting. Parameters:
|
get() | Gets the file metadata or content. Parameters:
|
getBinary() | Gets the file content as binary data. Parameters:
|
getContent() | Gets the file content. Parameters:
|
getJson<T = JsonObject>() | Gets the file content as JSON. A template parameter can be supplied to type the return value. If omitted, a JSON object will be returned. Parameters:
|
getMetadata() | Gets the file metadata. Parameters:
|
getText() | Gets the file content as text. Parameters:
|
list() | Lists files. Parameters:
queryParameters.q can be a query string or a ListQueryBuilder instance.Returns:
|
newMetadataOnlyUploader() | Creates a class instance to perform a metadata-only upload. Returns: |
newMultipartUploader() | Creates a class instance to perform a multipart upload. Returns: |
newResumableUploader() | Creates a class instance to perform a resumable upload. Returns: |
newSimpleUploader() | Creates a class instance to perform a simple upload. Returns: |
A GDrive
instance stores various api access parameters and the instances of the classes that wrap individual parts of the google drive api.
Name | Description |
---|---|
about | This read-only property stores the About instance. |
accessToken | This read/write property stores an access token to be used in subsequent calls to the apis. |
fetchTimeout | This read/write property stores a timeout in milliseconds for fetch() invocations. The default value is 1500 . If the value is INFINITE_TIMEOUT , fetch() will wait infinitely. |
files | This read-only property stores the Files instance. |
permissions | This read-only property stores the Permissions instance. |
This class handles file permissions.
Name | Description |
---|---|
create() | Creates a permission. Parameters:
|
delete() | Deletes a permission. Parameters:
|
An Uploader
descendant, this class handles metadata-only uploads. It doesn't have own methods or properties. ExecuteResultType
is set to file resource.
An UploaderWithSimpleData
descendant, this class handles multipart uploads. ExecuteResultType
is set to file resource.
Name | Description |
---|---|
setIsBase64() | Conditionally adds header Content-Transfer-Encoding: base64 to the request.Parameters:
|
setMultipartBoundary() | Sets the boundary string to be used for this upload. The default is foo_bar_baz .Parameters:
|
An UploaderWithDataMimeType
descendant, this class handles resumable uploads. ExecuteResultType
is set to ResumableUploadRequest
.
An UploaderWithSimpleData
descendant, this class handles simple uploads. It doesn't have own methods or properties. ExecuteResultType
is set to file resource.
Descendants of this abstract class handle create and update requests.
Uploader
has one template parameter, ExecuteResultType
, set by descendants.
Name | Description |
---|---|
execute() | Executes the request. Returns:
|
setIdOfFileToUpdate() | Sets the id of a file resource to be updated. Parameters:
|
setQueryParameters() | Sets query parameters. Parameters:Returns:
|
setRequestBody() | Sets the request body. Parameters:Returns:
|
This abstract descendant of Uploader
makes it possible to set the data mime type.
Name | Description |
---|---|
setDataMimeType() | Sets the data mime type. Parameters:
MIME_TYPES can be used as an easy to use source of MIME constants:import { MIME_TYPES } from '@robinbobin/react-native-google-drive-api-wrapper' |
This abstract descendant of UploaderWithDataMimeType
makes it possible to set the data to be uploaded.
Name | Description |
---|---|
setData() | Sets the data to be uploaded. Parameters:
|
An instance of this class is thrown when a response to an api call is received, but its ok
property is false
.
Name | Description |
---|---|
json | This read-only property will contain a JSON object describing the error. It can be null . |
response | This read-only property will contain the result of fetch() . |
This interface describes the result type of Files.createIfNotExists()
.
Name | Description |
---|---|
alreadyExisted | Will be true if the file already existed before the method invocation, false otherwise. |
result | Will contain a file resource, describing the existing file, if alreadyExisted is true . It will contain the result of invoking Uploader.execute() if alreadyExisted is false . |
This interface describes the parameters
type for Files.copy()
.
Name | Description |
---|---|
queryParameters? | query parameters |
requestBody? | file resource |
This interface describes the parameters
type for Files.get()
and the like.
Name | Description |
---|---|
queryParameters? | query parameters |
range? | Data range to get |
This interface describes the result type of ResumableUploadRequest.requestUploadStatus().
Name | Description |
---|---|
isComplete | Will be true if the upload is completed, false otherwise. |
transferredByteCount | Will hold the number of bytes currently transferred. |
Extending IRequestUploadStatusResultType, this interface describes the result type of ResumableUploadRequest.uploadChunk().
Name | Description |
---|---|
json? | Will contain a file resource, describing the file, if isComplete is true . |
A helper class for building Files.list()
queries. It uses the following type aliases:
type TKey = string
type TValue = JsonValue
type TValueQuotationFlag = boolean
type TKeyValueOperator = 'contains' | '=' | '>' | '<'
type TValueKeyOperator = 'in'
type TClause =
| [TKey, TKeyValueOperator, TValue, TValueQuotationFlag?]
| [TValue, TValueKeyOperator, TKey, TValueQuotationFlag?]
JsonValue
matches any valid JSON value.TValueQuotationFlag
determines whether string values will be quoted. The default is true
, meaning they will be quoted.Name | Description |
---|---|
constructor() | Parameters:
|
and() | Joins two subqueries with and .Parameters:
|
or() | Joins two subqueries with or .Parameters:
|
pop() | Adds ) .Returns:
|
push() | Adds ( .Parameters:
|
toString() | Stringifies the query. Returns:
|
This class serves as ExecuteResultType
for ResumableUploader
.
Name | Description |
---|---|
requestUploadStatus() | Requests the current upload status. Returns:
|
setContentLength() | Must be invoked when the content length is determined, if ResumableUploader.setContentLength() wasn't invoked.Parameters:
|
transferredByteCount | This read-only property will contain the current transferred byte count. |
uploadChunk() | Uploads a chunk of data. Parameters:
|
This type alias describes the queryParameters
type for About.get()
:
type TAboutGetQueryParameters = IStandardParameters | string | string[]
, where IStandardParameters
are defined as here and string
or string[]
determines the value of fields
.
This type alias describes the type of binary data returned from different api methods.
type TBlobToByteArrayResultType = Uint8Array | null
This type alias describes the type of data for uploaders
:
Uint8Array | string | number[]
An instance of this class is thrown by Files.createIfNotExists()
if the number of matching files is not zero or one.
Name | Description |
---|---|
realCount | Will contain the number of matching files. |
FAQs
This wrapper facilitates the use of the Google Drive API in React Native projects.
The npm package @robinbobin/react-native-google-drive-api-wrapper receives a total of 2,246 weekly downloads. As such, @robinbobin/react-native-google-drive-api-wrapper popularity was classified as popular.
We found that @robinbobin/react-native-google-drive-api-wrapper demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
Product
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.