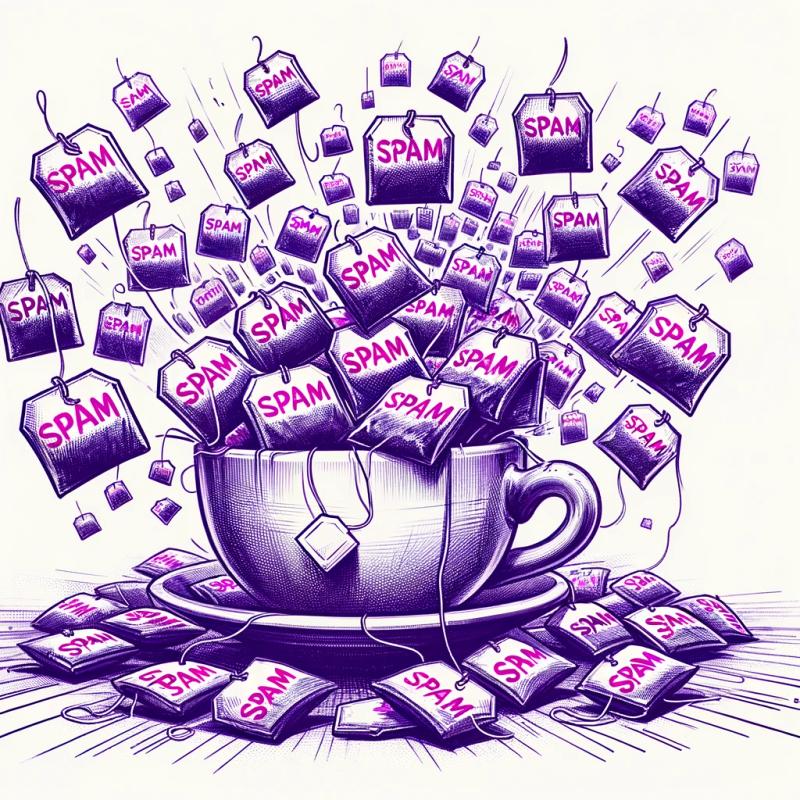
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@saipulanuar/scraper
Advanced tools
Readme
Install package
npm i @saipulanuar/scraper
Install latest version from github (not recommended)
npm i github:saipulanuar/scraper
didyoumean
to search for similar words.snapsave
zippyshare
sfilemobi
// ESM
import * as scraper from '@saipulanuar/scraper'
// CJS
const scraper = require('@saipulanuar/scraper')
// Instagram Downloader
import {
instagramdl,
instagramdlv2,
instagramStory,
instagramStoryv2
} from '@saipulanuar/scraper'
const url = 'https://www.instagram.com/reel/CXK49yFLtJ_/?utm_source=ig_web_copy_link'
instagramdl(url).then(console.log).catch(console.error)
instagramdlv2(url).then(console.log).catch(console.error)
// use both to handle error
instagramdl(url).catch(_ => instagramdlv2(url)).then(console.log)
// Use async/await or top level await
console.log(await instagramdl(url).catch(console.error))
console.log(await instagramdlv2(url).catch(console.error))
// Instagram Story downloader
const username = 'freefirebgid'
const story = await instagramStory(username).catch(async _ => await instagramStoryv2(username))
console.log(story)
instagramdl
use website https://snapinsta.app,instagramdlv2
use website https://downloadgram.orginstagramdlv3
use website https://downvideo.quora-wiki.cominstagramdlv4
use website https://instadownloader.coinstagramStory
use website https://storydownloader.appinstagramStoryv2
use website https://www.instadp.com [not working]// Youtube downloader
import {
youtubedl,
youtubedlv2
} from '@saipulanuar/scraper'
const url = 'https://youtu.be/iik25wqIuFo'
youtubedl(url).catch(_ => youtubedlv2(url)).then(({ video }) => {
video['240p'].download().then(console.log).catch(console.error)
})
// Use async/await
const yt = await youtubedl(url).catch(async () => await youtubedlv2(url))
const dl_url = await yt.video['240p'].download()
console.log(dl_url)
youtubedl
use website https://www.y2mate.comyoutubedlv2
use website https://yt5s.comyoutubedlv3
use website https://onlinevideoconverter.proyoutubeSearch
use website https://www.youtube.com// Tiktok downloader
import {
tiktokdl,
tiktokdlv2
} from '@saipulanuar/scraper'
// Tiktok downloader v1
const url = 'https://www.tiktok.com/@tiktok/video/6844446901010982300'
tiktokdl(url).then(console.log).catch(console.error)
// tiktokdl v2
tiktokdlv2(url).then(console.log).catch(console.error)
// async / await
console.log(await tiktokdl(url).catch(console.error))
console.log(await tiktokdlv2(url).catch(console.error))
tiktokdl
use website https://snaptik.apptiktokdlv2
use website https://api.tikmate.apptiktokdlv3
use website 'https://ssstik.iotiktokfyp
use website https://t.tiktok.com [Not Working]import {
aiovideodl,
savefrom,
snapsave
} from '@saipulanuar/scraper'
// Facebook video downloader
console.log(await aiovideodl('https://fb.watch/9WktuN9j-z/'))
// Twitter video downloader
console.log(await aiovideodl('https://twitter.com/jen_degen/status/1458167531869458440?s=20'))
// Tiktok downloader
console.log(await savefrom('https://www.tiktok.com/@omagadsus/video/7025456384175017243?is_from_webapp=1&sender_device=pc&web_id6982004129280116226'))
// Instagram downloader
console.log(await savefrom('https://www.instagram.com/reel/CXK49yFLtJ_/?utm_source=ig_web_copy_link'))
// Instagram downloader
console.log(await snapsave('https://www.instagram.com/reel/CXK49yFLtJ_/?utm_source=ig_web_copy_link'))
// Facebook video downloader
console.log(await snapsave('https://fb.watch/9WktuN9j-z/'))
aiovideodl
use website https://aiovideodl.ml [Not Working]savefrom
use website https://id.savefrom.netsnapsave
use website https://snapsave.app// Aksara jawa
import {
latinToAksara,
aksaraToLatin
} from '@saipulanuar/scraper'
// Latin to aksara jawa
console.log(latinToAksara('hallo rek'))
// Aksara jawa to latin
console.log(aksaraToLatin('ꦲꦭ꧀ꦭꦺꦴꦫꦺꦏ꧀', { HVokal: false })) // Hvokal: false mean ꦲ will return 'ha' not vokal
Source: https://bennylin.github.io/transliterasijawa/
// Primbons
import {
getZodiac,
nomorhoki
} from '@saipulanuar/scraper'
// Get zodiac
console.log(getZodiac(1, 1))
// Get nomor hoki
console.log(await nomorhoki(6213353))
artimimpi
use website https://www.primbon.comartiname
use website https://www.primbon.comnomorhoki
use website https://www.primbon.comgetZodiac
source: https://github.com/Nurutomo/wabot-aq/blob/master/plugins/zodiac.js// Images
import {
googleImage,
pinterest,
wallpaper,
stickerTelegram,
} from '@saipulanuar/scraper'
const keyword = 'minecraft'
// Google image
console.log(await googleImage(keyword))
// Pinterest image
console.log(await pinterest(keyword))
// Wallpaper
console.log(await wallpaper(keyword))
// Sticker telegram
console.log(await stickerTelegram(keyword))
googleImage
use website https://www.google.compinterest
use website https://www.pinterest.comstickerTelegram
use website https://combot.orgstickerLine
use website https://store.line.mewallpaper
use website https://www.shutterstock.comwallpaperv2
use website https://wall.alphacoders.comwallpaperv3
use website https://www.hdwallpapers.in// Religions
import {
asmaulhusna, asmaulhusnajson,
alquran,
jadwalsholat, listJadwalSholat
} from '@saipulanuar/scraper'
// Asmaul Husna
console.log(await asmaulhusna())
// Asmaul Husna Json
console.log(asmaulhusnajson) // the json will empty if you never use `asmaulhusna()`
// alquran
console.log(await alquran())
// Jadwal Sholat
console.log(await jadwalsholat('semarang'))
alquran
source: https://raw.githubusercontent.com/rzkytmgr/quran-api/master/data/quran.jsonasmaulhusna
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/religi/asmaulhusna.jsonjadwalsholat
use website https://www.jadwalsholat.org// Games
import {
tebakgambar, tebakgambarjson,
asahotak, asahotakjson
} from '@saipulanuar/scraper'
// Tebak gambar
console.log(await tebakgambar())
// Tebak gambar json
console.log(tebakgambarjson) // the json will empty if you never use `tebakgambar()`
// Asahotak
console.log(await asahotak())
// Asahotak json
console.log(asahotakjson) // the json will empty if you never use `asahotak()`
asahotak
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/asahotak.jsoncaklontong
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/caklontong.jsonfamily100
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/family100.jsonsiapakahaku
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/siapakahaku.jsonsusunkata
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/susunkata.jsontebakbendera
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/tebakbendera.jsontebakgambar
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/tebakgambar.jsontebakkabupaten
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/tebakkabupaten.jsontebakkata
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/tebakkata.jsontebakkimia
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/tebakkimia.jsontebaklirik
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/tebaklirik.jsontebaktebakan
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/tebaktebakan.jsontekateki
source: https://raw.githubusercontent.com/SaipulAnuar/database/master/games/tekateki.json// News
import {
cnbindonesia,
antaranews,
kompas
} from '@saipulanuar/scraper'
// Cnbindonesia
console.log(await cnbindonesia())
// Antaranews
console.log(await antaranews())
// Kompas
console.log(await kompas())
antaranews
use website https://www.antaranews.comcnbindonesia
use website https://www.cnbcindonesia.comkompas
use website https://www.kompas.comliputan6
use website https://www.liputan6.commerdeka
use website https://www.merdeka.comsuaracom
use website https://www.suara.com// Encryption
import {
toBase64,
fromBase64ToString,
randomUUID,
randomBytes,
createHash
} from '@saipulanuar/scraper'
// To base64
const base64 = toBase64('Hello World!!')
console.log(base64)
// From base64 to string
console.log(fromBase64ToString(base64)) // 'Hello World!!'
// Random UUID
console.log(randomUUID())
// Random Bytes
console.log(randomBytes(16))
// Hash
console.log(createHash('sha256', 'Hello World!!'))
randomUUID
source: https://github.com/uuidjs/uuid/blob/main/src/v4.js and https://github.com/nodejs/node/blob/master/lib/internal/crypto/random.jsrandomBytes
use crypto
modulecreateHash
use crypto
module// Bioskop
import {
bioskopNow,
bioskop
} from '@saipulanuar/scraper'
// Bioskop
console.log(await bioskop())
// Bioskop Now
console.log(await bioskopNow())
bioskop
use website https://jadwalnonton.combioskopNow
use website https://jadwalnonton.comimport {
mediafiredl,
sfilemobi,
sfilemobiSearch,
zippyshare
} from '@saipulanuar/scraper'
// Mediafire
console.log(await mediafiredl('https://www.mediafire.com/file/gpeiucmm1xo6ln0/hello_world.mp4/file'))
// Sfilemobi
console.log(await sfilemobi('https://sfile.mobi/oGm8kAIQCs7'))
// Sfilemobi Search
console.log(await sfilemobiSearch('minecraft'))
// Zippyshare
console.log(await zippyshare('https://www53.zippyshare.com/v/Gajlfjd4/file.html'))
mediafiredl
use website https://www.mediafire.comsfilemobi
use website https://www.sfilemobi.comsfilemobiSearch
use website https://www.sfilemobi.comzippyshare
use website https://www.zippyshare.comimport {
chord
} from '@saipulanuar/scraper'
// Chord
console.log(await chord('Until i found you'))
chord
use website https://www.gitagram.comsavefrom
now output array of object instead of objectasmaulhusna
optionally can take index
parameter to get the asmaulhusna of that index (default is random 1-99)FAQs
scraper module
The npm package @saipulanuar/scraper receives a total of 875 weekly downloads. As such, @saipulanuar/scraper popularity was classified as not popular.
We found that @saipulanuar/scraper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.