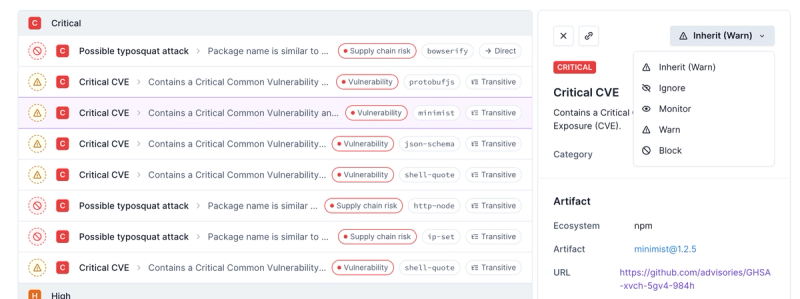
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@sentry/webpack-plugin
Advanced tools
Package description
The @sentry/webpack-plugin is a plugin for Webpack that enables you to automatically upload source maps to Sentry, facilitating better debugging of JavaScript errors by linking errors to the original source code, rather than the minified or compiled code served to clients. It integrates seamlessly with Sentry's error tracking and monitoring system, making it easier to track down and fix issues in production environments.
Source Maps Upload
Automatically uploads source maps to Sentry for better error tracking and debugging. This feature is crucial for understanding and resolving issues in production by linking errors back to the original source code.
{
plugins: [
new SentryWebpackPlugin({
include: ".", // The directory to include in source maps upload
ignore: ["node_modules", "webpack.config.js"], // Files and directories to ignore
urlPrefix: "~/static/js", // Prefix that will be added to each of the files in the `include` option
release: process.env.RELEASE_VERSION // The release version to associate the source maps with
})
]
}
Release Health Tracking
Configures release and deploy information, enabling tracking of release health within Sentry. This helps in monitoring the impact of each release on your application's stability and performance.
{
plugins: [
new SentryWebpackPlugin({
release: process.env.RELEASE_VERSION, // Define the release version
setCommits: {
repo: 'your-repo-name',
auto: true
},
deploy: {
env: 'production'
}
})
]
}
The source-map-loader uses source maps to allow you to debug your original source code instead of the transformed code. While it doesn't upload source maps to Sentry, it serves a similar purpose in improving the debugging experience by handling source maps in Webpack builds.
This package provides a set of Webpack plugins for integrating with Bugsnag, similar to how @sentry/webpack-plugin integrates with Sentry. It includes features for uploading source maps to Bugsnag and automatically reporting build information, offering a comparable functionality for error tracking and monitoring in applications.
Readme
A Webpack plugin that provides release management features for Sentry:
Using npm:
$ npm install @sentry/webpack-plugin --save-dev
Using yarn:
$ yarn add @sentry/webpack-plugin --dev
// webpack.config.js
const { sentryWebpackPlugin } = require("@sentry/webpack-plugin");
module.exports = {
plugins: [
sentryWebpackPlugin({
include: ".",
ignore: ["node_modules", "webpack.config.js"],
org: process.env.SENTRY_ORG,
project: process.env.SENTRY_PROJECT,
authToken: process.env.SENTRY_AUTH_TOKEN,
}),
],
};
As an alternative to passing options explicitly, you can also use a .sentryclirc
file or environment variables as described in https://docs.sentry.io/product/cli/configuration/.
The Sentry Webpack Plugin takes an options argument with the following properties:
Option | Type | Required | Description |
---|---|---|---|
org | string | optional | The slug of the Sentry organization associated with the app. Can also be specified via the SENTRY_ORG environment variable. |
project | string | optional | The slug of the Sentry project associated with the app. Can also be specified via the SENTRY_PROJECT environment variable. |
authToken | string | optional | The authentication token to use for all communication with Sentry. Can be obtained from https://sentry.io/settings/account/api/auth-tokens/. Required scopes: project:releases (and org:read if setCommits option is used). Can also be specified via the SENTRY_AUTH_TOKEN env variable. |
sourcemaps.assets | string | string[] | optional | A glob or an array of globs that specify the build artifacts that should be uploaded to Sentry. Leave this option undefined if you do not want to upload source maps to Sentry. The globbing patterns follow the implementation of the glob package. Use the debug option to print information about which files end up being uploaded. |
sourcemaps.ignore | string | string[] | optional | A glob or an array of globs that specify which build artifacts should not be uploaded to Sentry. The globbing patterns follow the implementation of the glob package. Use the debug option to print information about which files end up being uploaded. Default: [] |
url | string | optional | The base URL of your Sentry instance. Use this if you are using a self-hosted or Sentry instance other than sentry.io. This value can also be set via the SENTRY_URL environment variable. Defaults to https://sentry.io/, which is the correct value for SaaS customers. |
headers | Record<string, string> | optional | Headers added to every outgoing network request. |
vcsRemote | string | optional | Version control system remote name. This value can also be specified via the SENTRY_VSC_REMOTE environment variable. Defaults to 'origin' . |
release | string | optional | Unique identifier for the release. This value can also be specified via the SENTRY_RELEASE environment variable. Defaults to the output of the sentry-cli releases propose-version command, which automatically detects values for Cordova, Heroku, AWS CodeBuild, CircleCI, Xcode, and Gradle, and otherwise uses the git HEAD 's commit SHA. (the latter requires access to git CLI and for the root directory to be a valid repository). |
dist | string | optional | Unique identifier for the distribution, used to further segment your release. Usually your build number. |
releaseInjectionTargets | array /RegExp /(string | RegExp)[] /function(filePath: string): bool | optional | Filter for modules that the release should be injected in. This option takes a string, a regular expression, or an array containing strings, regular expressions, or both. It's also possible to provide a filter function that takes the absolute path of a processed module. It should return true if the release should be injected into the module and false otherwise. String values of this option require a full match with the absolute path of the module. By default, the release will be injected into all modules - however, bundlers will include the injected release code only once per entrypoint. If release injection should be disabled, provide an empty array here. |
ignoreFile | string | optional | Path to a file containing list of files/directories to ignore. Can point to .gitignore or anything with the same format. |
ignore | string /array | optional | One or more paths to ignore during upload. Overrides entries in ignoreFile file. Defaults to ['node_modules'] if neither ignoreFile nor ignore is set. |
configFile | string | optional | Path to Sentry CLI config properties, as described in https://docs.sentry.io/product/cli/configuration/#configuration-file. By default, the config file is looked for upwards from the current path, and defaults from ~/.sentryclirc are always loaded. |
ext | array | optional | Array of file extensions of files to be collected for the file upload. By default the following file extensions are processed: js, map, jsbundle and bundle. |
urlPrefix | string | optional | URL prefix to add to the beginning of all filenames. Defaults to '~/' but you might want to set this to the full URL. This is also useful if your files are stored in a sub folder. eg: url-prefix '~/static/js' . |
urlSuffix | string | optional | URL suffix to add to the end of all filenames. Useful for appending query parameters. |
validate | boolean | optional | When true , attempts source map validation before upload if rewriting is not enabled. It will spot a variety of issues with source maps and cancel the upload if any are found. Defaults to false as this can cause false positives. |
stripPrefix | array | optional | When paired with the rewrite option, this will remove a prefix from filename references inside of sourcemaps. For instance you can use this to remove a path that is build machine specific. Note that this will NOT change the names of uploaded files. |
stripCommonPrefix | boolean | optional | When paired with the rewrite option, this will add ~ to the stripPrefix array. |
sourceMapReference | boolean | optional | Determines whether sentry-cli should attempt to link minified files with their corresponding maps. By default, it will match files and maps based on name, and add a Sourcemap header to each minified file for which it finds a map. Can be disabled if all minified files contain a sourceMappingURL . Defaults to true . |
rewrite | boolean | optional | Enables rewriting of matching source maps so that indexed maps are flattened and missing sources are inlined if possible. Defaults to true |
finalize | boolean | optional | Determines if the Sentry release record should be automatically finalized (meaning a date_released timestamp is added) after artifact upload. Defaults to true . |
dryRun | boolean | optional | Attempts a dry run (useful for dev environments), making release creation a no-op. Defaults to false . |
debug | boolean | optional | Print useful debug information. Defaults to false . |
silent | boolean | optional | Suppresses all logs. Defaults to false . |
cleanArtifacts | boolean | optional | Remove all the artifacts in the release before the upload. Defaults to false . |
errorHandler | function(err: Error): void | optional | When an error occurs during release creation or sourcemaps upload, the plugin will call this function. By default, the plugin will simply throw an error, thereby stopping the bundling process. If an errorHandler callback is provided, compilation will continue, unless an error is thrown in the provided callback. |
setCommits | Object | optional | Associates the release with its commits in Sentry. See table below for details. |
deploy | Object | optional | Adds deployment information to the release in Sentry. See table below for details. |
injectRelease | boolean | optional | Whether the plugin should inject release information into the build. Defaults to true . |
uploadSourceMaps | boolean | optional | Whether the plugin should upload source maps to Sentry. Defaults to true . |
telemetry | boolean | optional | If set to true, internal plugin errors and performance data will be sent to Sentry. At Sentry we like to use Sentry ourselves to deliver faster and more stable products. We're very careful of what we're sending. We won't collect anything other than error and high-level performance data. We will never collect your code or any details of the projects in which you're using this plugin. Defaults to true . |
include | string /array /object | required | One or more paths that the plugin should scan recursively for sources. It will upload all .map files and match associated .js files. Other file types can be uploaded by using the ext option. Each path can be given as a string or an object with path-specific options. See table below for details. (Note: This option is deprecated. Please use the sourcemap option instead) |
_experiments.injectBuildInformation | boolean | optional | If set to true, the plugin will inject an additional SENTRY_BUILD_INFO variable that contains information about the build that can be used to improve SDK functionality. Defaults to false . |
Option | Type | Required | Description |
---|---|---|---|
paths | string[] | required | One or more paths to scan for files to upload. |
ignoreFile | string | optional | See above. |
ignore | string /string[] | optional | See above. |
ext | array | optional | See above. |
urlPrefix | string | optional | See above. |
urlSuffix | string | optional | See above. |
stripPrefix | array | optional | See above. |
stripCommonPrefix | boolean | optional | See above. |
sourceMapReference | boolean | optional | See above. |
rewrite | boolean | optional | See above. |
Example:
// webpack.config.js
const sentryWebpackPlugin = require("@sentry/webpack-plugin");
module.exports = {
plugins: [
sentryWebpackPlugin({
// ...
include: [
{
paths: ["./packages"],
urlPrefix: "~/path/to/packages",
},
{
paths: ["./client"],
urlPrefix: "~/path/to/client",
},
],
}),
],
};
Option | Type | Required | Description |
---|---|---|---|
repo | string | see notes | The full git repo name as defined in Sentry. Required if the auto option is not true , otherwise optional. |
commit | string | see notes | The current (most recent) commit in the release. Required if the auto option is not true , otherwise optional. |
previousCommit | string | optional | The commit before the beginning of this release (in other words, the last commit of the previous release). Defaults to the last commit of the previous release in Sentry. If there was no previous release, the last 10 commits will be used. |
auto | boolean | optional | Automatically sets commit and previousCommit . Sets commit to HEAD and previousCommit as described in the option's documentation. If you set this to true , manually specified commit and previousCommit options will be overridden. It is best to not specify them at all if you set this option to true . |
ignoreMissing | boolean | optional | If the flag is to true and the previous release commit was not found in the repository, the plugin creates a release with the default commits count instead of failing the command. Defaults to false . |
Option | Type | Required | Description |
---|---|---|---|
env | string | required | Environment value for the release, for example production or staging . |
started | number | optional | Deployment start time in Unix timestamp (in seconds) or ISO 8601 format. |
finished | number | optional | Deployment finish time in Unix timestamp (in seconds) or ISO 8601 format. |
time | number | optional | Deployment duration in seconds. Can be used instead of started and finished . |
name | string | optional | Human-readable name for this deployment. |
url | string | optional | URL that points to the deployment. |
You can find more information about these options in our official docs: https://docs.sentry.io/product/cli/releases/#sentry-cli-sourcemaps.
FAQs
Official Sentry Webpack plugin
The npm package @sentry/webpack-plugin receives a total of 1,725,718 weekly downloads. As such, @sentry/webpack-plugin popularity was classified as popular.
We found that @sentry/webpack-plugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.