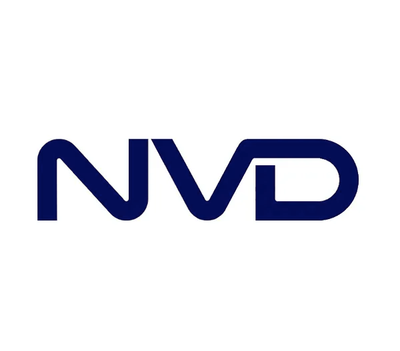
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
@solana-mobile/mobile-wallet-adapter-walletlib
Advanced tools
A React Native wrapper of the Solana Mobile, Mobile Wallet Adapter Wallet Library. Wallet apps can use this to handle dapp requests for signing and sending.
@solana-mobile/mobile-wallet-adapter-walletlib
This is a package that provides React Native bridge for the native mobile-wallet-adapter-walletlib
library and it is designed for Wallet apps built in React Native. It provides an API to implement the wallet endpoint of the mobile wallet adapter protocol.
Deep dive and read the full Mobile Wallet Adapter protocol specification.
This package is still in alpha and is not production ready. However, the API is stable and will not change drastically, so you can begin integration with your wallet.
Use the following API to start listening for MWA requests and events, and register request handlers.
import {
initializeMWAEventListener,
MWARequest,
MWASessionEvent,
} from '@solana-mobile/mobile-wallet-adapter-walletlib';
const listener: EmitterSubscription = initializeMWAEventListener(
(request: MWARequest) => { /* ... */ },
(sessionEvent: MWASessionEvent) => { /* ... */ },
);
/* ... */
// Clean up the listener when it is out of scope
listener.remove()
You should ensure the listener is cleaned up with listener.remove()
when it goes out of scope (e.g listener.remove()
on component lifecycle unmount).
Define your wallet config and use initializeMWASession
to establish a session with the dApp endpoint and begin emission of MWA requests/events.
Note: This should be called after
initializeMWAEventListener
is called, to ensure no events are missed.
const config: MobileWalletAdapterConfig = {
supportsSignAndSendTransactions: true,
maxTransactionsPerSigningRequest: 10,
maxMessagesPerSigningRequest: 10,
supportedTransactionVersions: [0, 'legacy'],
noConnectionWarningTimeoutMs: 3000,
optionalFeatures: ['solana:signInWithSolana']
};
try {
const sessionId = await initializeMobileWalletAdapterSession(
'Wallet Name',
config,
);
console.log('sessionId: ' + sessionId);
} catch (e: any) {
if (e instanceof SolanaMWAWalletLibError) {
console.error(e.name, e.code, e.message);
} else {
console.error(e);
}
}
Note: Although, the
initializeMobileWalletAdapterSession
method returns asessionId
, this library only supports one active session for now.
// When your MWA entrypoint is loaded, call a `useEffect` to kick off the listener and session.
useEffect(() => {
async function initializeMWASession() {
const config: MobileWalletAdapterConfig = {
supportsSignAndSendTransactions: true,
maxTransactionsPerSigningRequest: 10,
maxMessagesPerSigningRequest: 10,
supportedTransactionVersions: [0, 'legacy'],
noConnectionWarningTimeoutMs: 3000,
};
try {
const sessionId = await initializeMobileWalletAdapterSession(
'Wallet Name',
config,
);
console.log('sessionId: ' + sessionId);
} catch (e: any) {
if (e instanceof SolanaMWAWalletLibError) {
console.error(e.name, e.code, e.message);
} else {
console.error(e);
}
}
}
const listener = initializeMWAEventListener(
(request: MWARequest) => { /* ... */ },
(sessionEvent: MWASessionEvent) => { /* ... */ },
);
initializeMWASession();
// When the component is unmounted, clean up the listener.
return () => listener.remove();
}, []);
A MWARequest
is handled by calling resolve(request, response)
and each request have their appropriate response types.
An example of handling an AuthorizationRequest
:
import {
AuthorizeDappResponse
} from '@solana-mobile/mobile-wallet-adapter-walletlib';
const response = {
publicKey: Keypair.generate().publicKey.toBytes(),
label: 'Wallet Label',
} as AuthorizeDappResponse;
resolve(authorizationRequest, response)
There are a a selection of "fail" responses that you can return to the dApp. These are for cases where the user declines, or an error occurs during signing, etc.
import {
UserDeclinedResponse
} from '@solana-mobile/mobile-wallet-adapter-walletlib';
const response = {
failReason: MWARequestFailReason.UserDeclined,
} as UserDeclinedResponse;
// Tells the dApp user has declined the authorization request
resolve(authorizationRequest, response)
Each MWA Request is defined in resolve.ts
.
Each come with their own properties and completion response structures.
If you want to understand the dApp perspective and how a dApp would send these requests, see MWA API Documentation for dAppstypescript/mobile-wallet-adapter.
IMWARequest
This is the base interface that all MWARequsts inherit from. The fields defined here are used in the package's internal implementation and the package consumer will generally not use them.
Fields:
__type
: An enum defining the type of MWA Request it is.requestId
: A unique identifier of this specific MWA RequestsessionId
: A unique identifier of the MWA Session this request belongs to.IVerifiableIdentityRequest
This an interface that describes MWA Requests that come with a verifiable identity and the following 3 fields.
Fields:
authorizationScope
: A byte representation of the authorization token granted to the dApp.cluster
: The Solana RPC cluster that the dApp intends to use.appIdentity
: An object containing 3 optional identity fields about the dApp:
iconRelativeUri
is a relative path, relative to identityUri
.{
identityName: 'dApp Name',
identityUri: 'https://yourdapp.com'
iconRelativeUri: "favicon.ico", // Full path resolves to https://yourdapp.com/favicon.ico
}
AuthorizeDappRequest
IMWARequest
ReauthorizeDappRequest
IMWARequest
, IVerifiableIdentityRequest
DeauthorizeDappRequest
IMWARequest
, IVerifiableIdentityRequest
SignMessagesRequest
IMWARequest
, IVerifiableIdentityRequest
SignTransactionsRequest
IMWARequest
, IVerifiableIdentityRequest
SignAndSendTransactionsRequest
IMWARequest
, IVerifiableIdentityRequest
FAQs
A React Native wrapper of the Solana Mobile, Mobile Wallet Adapter Wallet Library. Wallet apps can use this to handle dapp requests for signing and sending.
The npm package @solana-mobile/mobile-wallet-adapter-walletlib receives a total of 28 weekly downloads. As such, @solana-mobile/mobile-wallet-adapter-walletlib popularity was classified as not popular.
We found that @solana-mobile/mobile-wallet-adapter-walletlib demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.