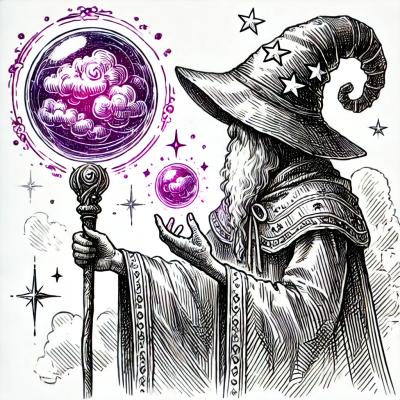
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@solana/solidity
Advanced tools
@solana/solidity
The Solang Compiler compiles Solidity contracts to native Solana BPF programs.
This TypeScript library, inspired by Ethers.js, can deploy and interact with Solidity contracts on Solana.
This is a short guide to deploying and interacting with the standard ERC20 Solidity contract on Solana.
git clone https://github.com/solana-labs/solana-solidity.js.git
cd solana-solidity.js
yarn docker
yarn validator
mkdir -p project/contracts project/build
cd project
curl -o contracts/ERC20.sol \
https://raw.githubusercontent.com/solana-labs/solana-solidity.js/master/test/examples/erc20/contracts/ERC20.sol
docker run --rm -it -v $PWD:/project \
ghcr.io/hyperledger/solang \
-o /project/build --target solana -v /project/contracts/ERC20.sol
This outputs ERC20.abi
and bundle.so
files to the build
directory.
yarn add @solana/solidity
# OR
npm install @solana/solidity
touch erc20.js
const { Connection, LAMPORTS_PER_SOL, Keypair } = require('@solana/web3.js');
const { Contract } = require('@solana/solidity');
const { readFileSync } = require('fs');
const ERC20_ABI = JSON.parse(readFileSync('./build/ERC20.abi', 'utf8'));
const BUNDLE_SO = readFileSync('./build/bundle.so');
(async function () {
console.log('Connecting to your local Solana node ...');
const connection = new Connection('http://localhost:8899', 'confirmed');
const payer = Keypair.generate();
console.log('Airdropping SOL to a new wallet ...');
const signature = await connection.requestAirdrop(payer.publicKey, 10 * LAMPORTS_PER_SOL);
await connection.confirmTransaction(signature, 'confirmed');
const address = publicKeyToHex(payer.publicKey);
const program = Keypair.generate();
const storage = Keypair.generate();
const contract = new Contract(
connection,
program.publicKey,
storage.publicKey,
ERC20_ABI,
payer
);
console.log('Deploying the Solang-compiled ERC20 program ...');
await contract.load(program, BUNDLE_SO);
console.log('Program deployment finished, deploying the ERC20 contract ...');
await contract.deploy(
'ERC20',
['Solana', 'SOL', '1000000000000000000'],
storage,
4096 * 8
);
console.log('Contract deployment finished, invoking some contract functions ...');
const symbol = await contract.symbol();
const balance = await contract.balanceOf(address);
console.log(`ERC20 contract for ${symbol} deployed!`);
console.log(`Your wallet at ${address} has a balance of ${balance} tokens.`);
contract.addEventListener(function (event) {
console.log(`${event.name} event emitted!`);
console.log(`${event.args[0]} sent ${event.args[2]} tokens to ${event.args[1]}`);
});
console.log('Sending tokens will emit a "Transfer" event ...');
const recipient = Keypair.generate();
await contract.transfer(recipient.publicKey.toBytes(), 1000000000000000000);
process.exit(0);
})();
node erc20.js
git clone https://github.com/solana-labs/solana-solidity.js.git
cd solana-solidity.js
yarn install
yarn build
yarn docker
yarn validator
yarn build:test
yarn test
FAQs
Solana Solidity Contracts JavaScript Client
The npm package @solana/solidity receives a total of 0 weekly downloads. As such, @solana/solidity popularity was classified as not popular.
We found that @solana/solidity demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 13 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.