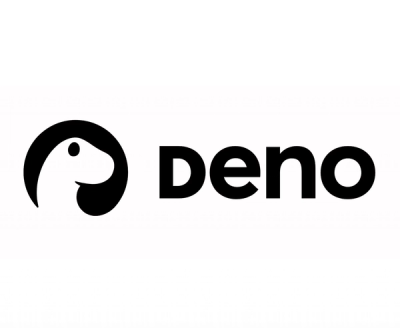
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
@stdlib/assert-is-function
Advanced tools
@stdlib/assert-is-function is a utility package that provides functionality to check if a value is a function. This can be useful in various scenarios where type checking is necessary to ensure that a value can be invoked as a function.
Basic Function Check
This feature allows you to check if a given value is a function. It returns `true` if the value is a function and `false` otherwise.
const isFunction = require('@stdlib/assert-is-function');
console.log(isFunction(function() {})); // true
console.log(isFunction(null)); // false
Arrow Function Check
This feature supports checking if an arrow function is a function. It returns `true` for arrow functions.
const isFunction = require('@stdlib/assert-is-function');
console.log(isFunction(() => {})); // true
Class Method Check
This feature allows you to check if a class method is a function. It returns `true` for class methods.
const isFunction = require('@stdlib/assert-is-function');
class MyClass {
myMethod() {}
}
console.log(isFunction(MyClass.prototype.myMethod)); // true
lodash.isfunction is a utility function from the Lodash library that checks if a value is classified as a Function object. It is similar to @stdlib/assert-is-function but is part of the larger Lodash utility library, which provides a wide range of utility functions for common programming tasks.
is-function is a lightweight npm package that checks if a given value is a function. It is similar to @stdlib/assert-is-function in its core functionality but is a standalone package without additional utilities.
type-detect is a library that provides type detection for JavaScript values. It can be used to check if a value is a function among other types. It offers more comprehensive type checking compared to @stdlib/assert-is-function, which focuses solely on function detection.
We believe in a future in which the web is a preferred environment for numerical computation. To help realize this future, we've built stdlib. stdlib is a standard library, with an emphasis on numerical and scientific computation, written in JavaScript (and C) for execution in browsers and in Node.js.
The library is fully decomposable, being architected in such a way that you can swap out and mix and match APIs and functionality to cater to your exact preferences and use cases.
When you use stdlib, you can be absolutely certain that you are using the most thorough, rigorous, well-written, studied, documented, tested, measured, and high-quality code out there.
To join us in bringing numerical computing to the web, get started by checking us out on GitHub, and please consider financially supporting stdlib. We greatly appreciate your continued support!
Test if a value is a function.
npm install @stdlib/assert-is-function
var isFunction = require( '@stdlib/assert-is-function' );
Tests if a value
is a function
.
function beep() {
console.log( 'beep' );
}
var bool = isFunction( beep );
// returns true
var isFunction = require( '@stdlib/assert-is-function' );
var bool = isFunction( function foo() {} );
// returns true
bool = isFunction( 'beep' );
// returns false
bool = isFunction( 5 );
// returns false
bool = isFunction( true );
// returns false
bool = isFunction( null );
// returns false
bool = isFunction( [] );
// returns false
bool = isFunction( {} );
// returns false
This package is part of stdlib, a standard library for JavaScript and Node.js, with an emphasis on numerical and scientific computing. The library provides a collection of robust, high performance libraries for mathematics, statistics, streams, utilities, and more.
For more information on the project, filing bug reports and feature requests, and guidance on how to develop stdlib, see the main project repository.
See LICENSE.
Copyright © 2016-2024. The Stdlib Authors.
0.2.0 (2024-02-14)
No changes reported for this release.
</section> <!-- /.release --> <section class="release" id="v0.1.1">FAQs
Test if a value is a function.
The npm package @stdlib/assert-is-function receives a total of 295,466 weekly downloads. As such, @stdlib/assert-is-function popularity was classified as popular.
We found that @stdlib/assert-is-function demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.