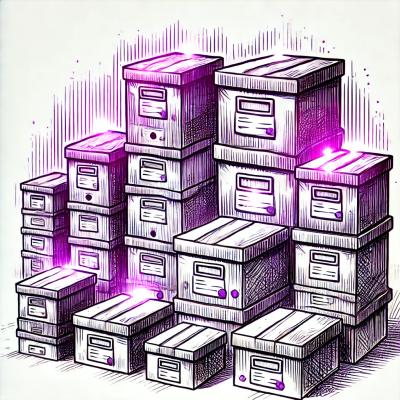
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
@sumup/design-tokens
Advanced tools
Visual primitives such as typography, color, and spacing that are shared across platforms.
Depending on your preference, run one of the following in your terminal:
# With npm
npm install @sumup/design-tokens
# With yarn v1
yarn add @sumup/design-tokens
The design tokens are exported as CSS custom properties. Choose a color scheme, then import the corresponding CSS file globally in your application, such as in Next.js' app/layout.tsx
file:
// app/layout.tsx
import '@sumup/design-tokens/light.css';
function App({ Component, pageProps }) {
return <Component {...pageProps} />;
}
The application code must be processed by a bundler that can handle CSS files. Next.js, Create React App, Vite, Parcel, and others support importing CSS files out of the box.
Refer to the theme documentation for a complete reference of the available tokens.
For applications that support a single color scheme, import the @sumup/design-tokens/light.css
or @sumup/design-tokens/dark.css
themes. They contain the complete set of design tokens, in light and dark mode respectively. The tokens are defined globally on the :root
element. The themes can be used independently or with a scoped theme.
To apply a different color scheme to a subset of an application, import the @sumup/design-tokens/light-scoped.css
or @sumup/design-tokens/dark-scoped.css
themes. They contain only color tokens which are scoped to the data-color-scheme="light"
and data-color-scheme="dark"
selectors respectively. The themes must be used alongside a full theme to ensure that all design tokens are defined.
For applications that support switching between light and dark color schemes, import the @sumup/design-tokens/dynamic.css
theme. It contains all tokens defined globally on the :root
element, the dark color tokens scoped to the prefers-color-scheme: dark
media query, and the scoped themes. By default, the color scheme follows the system settings. Add the data-color-scheme
attribute on the document root to set a specific color scheme.
Ensure that users have full control over their preferred color mode: dark, light, or match system. Their choice should be persisted between devices and sessions. There should be no Flash of inAccurate coloR Theme (FART), regardless of whether the app is client-side or server-side rendered.
[!WARNING] The JavaScript theme is being replaced by CSS custom properties (aka CSS variables) to improve performance and compatibility with other frameworks. We recommend installing the
@sumup/eslint-plugin-circuit-ui
ESLint plugin and turning on theprefer-custom-properties
andno-invalid-custom-properties
rules to help with the migration.
The light
theme is meant to be used with Emotion.js' ThemeProvider
:
import { light } from '@sumup/design-tokens';
import { ThemeProvider } from '@emotion/react';
import styled from '@emotion/styled';
const Bold = styled.strong`
font-weight: ${(p) => p.theme.fontWeight.bold};
`;
function App() {
return (
<ThemeProvider theme={light}>
<Bold>This styled component has access to the theme.</Bold>
</ThemeProvider>
);
}
The theme is a plain JavaScript object, so you can use it in other ways, too.
The package exports a themePropType
which can be used to check the theme
prop:
import PropTypes from 'prop-types';
import { withTheme } from '@emotion/react';
import { themePropType } from '@sumup/design-tokens';
function ComponentWithInlineStyles({ theme, label }) {
return <div style={{ borderRadius: theme.borderRadius.kilo }}>{label}</div>;
}
ComponentWithInlineStyles.propTypes = {
theme: themePropType.isRequired,
label: PropTypes.string,
};
export default function withTheme(ComponentWithInlineStyles);
The package exports a Theme
interface that can be used to augment Emotion.js' types as described in the Emotion.js docs:
import '@emotion/react';
import type { Theme as CircuitUITheme } from '@sumup/design-tokens';
declare module '@emotion/react' {
export interface Theme extends CircuitUITheme {}
}
FAQs
Visual primitives such as typography, color, and spacing that are shared across platforms.
The npm package @sumup/design-tokens receives a total of 660 weekly downloads. As such, @sumup/design-tokens popularity was classified as not popular.
We found that @sumup/design-tokens demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.