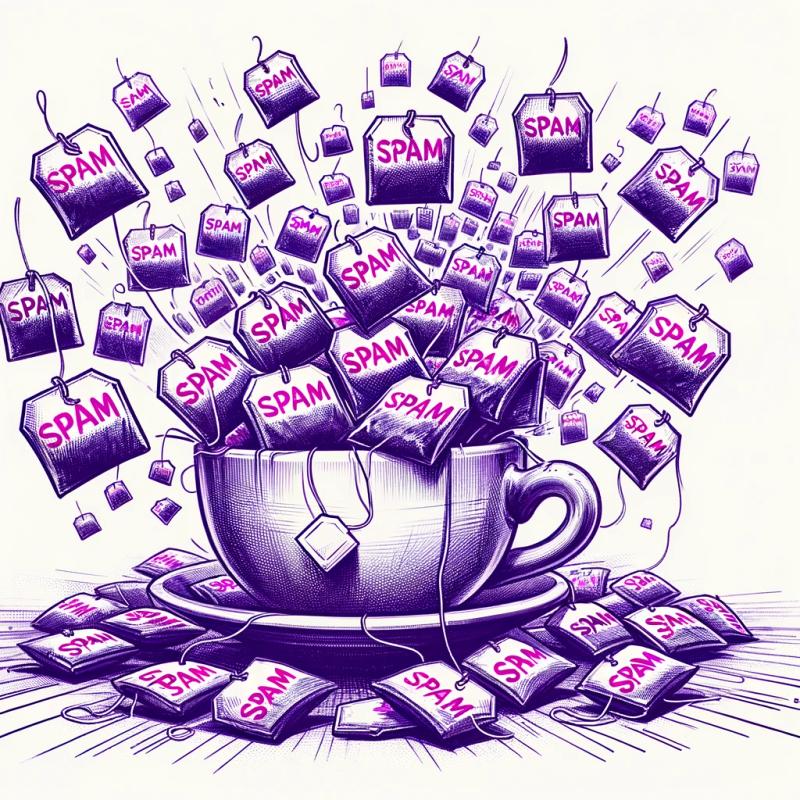
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@tybys/wasm-util
Advanced tools
Readme
WebAssembly related utils for browser environment
The output code is ES2019
All example code below need to be bundled by ES module bundlers like webpack
/ rollup
, or specify import map in browser native ES module runtime.
The API is similar to the require('wasi').WASI
in Node.js.
You can use memfs-browser
to provide filesystem capability.
import { load, WASI } from '@tybys/wasm-util'
import { Volume, createFsFromVolume } from 'memfs-browser'
const fs = createFsFromVolume(Volume.fromJSON({
'/home/wasi': null
}))
const wasi = new WASI({
args: ['chrome', 'file.wasm'],
env: {
NODE_ENV: 'development',
WASI_SDK_PATH: '/opt/wasi-sdk'
},
preopens: {
'/': '/'
},
fs,
// redirect stdout / stderr
// print (text) { console.log(text) },
// printErr (text) { console.error(text) }
})
const imports = {
wasi_snapshot_preview1: wasi.wasiImport
}
const { module, instance } = await load('/path/to/file.wasm', imports)
wasi.start(instance)
// wasi.initialize(instance)
Implemented syscalls: wasi_snapshot_preview1
load
/ loadSync
loadSync
has 4KB wasm size limit in browser.
// bundler
import { load, loadSync } from '@tybys/wasm-util'
const imports = { /* ... */ }
// using path
const { module, instance } = await load('/path/to/file.wasm', imports)
const { module, instance } = loadSync('/path/to/file.wasm', imports)
// using URL
const { module, instance } = await load(new URL('./file.wasm', import.meta.url), imports)
const { module, instance } = loadSync(new URL('./file.wasm', import.meta.url), imports)
// using Uint8Array
const buffer = new Uint8Array([
0x00, 0x61, 0x73, 0x6d,
0x01, 0x00, 0x00, 0x00
])
const { module, instance } = await load(buffer, imports)
const { module, instance } = loadSync(buffer, imports)
// auto asyncify
const {
module,
instance: asyncifiedInstance
} = await load(buffer, imports, { /* asyncify options */})
asyncifiedInstance.exports.fn() // => return Promise
import { Memory, extendMemory } from '@tybys/wasm-util'
const memory = new WebAssembly.Memory({ initial: 256 })
// const memory = instance.exports.memory
extendMemory(memory)
console.log(memory instanceof Memory)
console.log(memory instanceof WebAssembly.Memory)
// expose memory view getters like Emscripten
const { HEAPU8, HEAPU32, view } = memory
Build the C code using clang
, wasm-ld
and wasm-opt
void async_sleep(int ms);
int main() {
async_sleep(200);
return 0;
}
import { Asyncify } from '@tybys/wasm-util'
const asyncify = new Asyncify()
const imports = {
env: {
async_sleep: asyncify.wrapImportFunction(function (ms) {
return new Promise((resolve) => {
setTimeout(resolve, ms)
})
})
}
}
// async_sleep(200)
const bytes = await (await fetch('/asyncfied_by_wasm-opt.wasm')).arrayBuffer()
const { instance } = await WebAssembly.instantiate(bytes, imports)
const asyncifiedInstance = asyncify.init(instance.exports.memory, instance, {
wrapExports: ['_start']
})
const p = asyncifedInstance._start()
console.log(typeof p.then === 'function')
const now = Date.now()
await p
console.log(Date.now() - now >= 200)
FAQs
WASI polyfill for browser and some wasm util
The npm package @tybys/wasm-util receives a total of 64,516 weekly downloads. As such, @tybys/wasm-util popularity was classified as popular.
We found that @tybys/wasm-util demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.