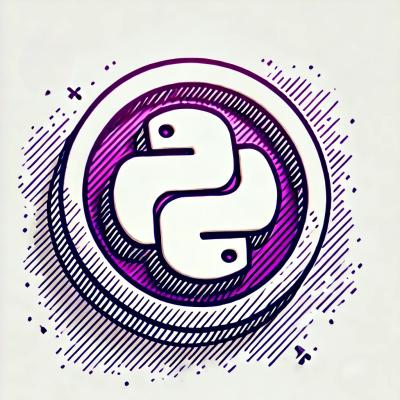
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@types/caseless
Advanced tools
TypeScript definitions for caseless
@types/caseless provides TypeScript type definitions for the 'caseless' npm package, which allows for case-insensitive operations on HTTP headers.
Set a header
This feature allows you to set a header in a case-insensitive manner. The 'set' method ensures that the header name is stored in a consistent case.
const caseless = require('caseless');
const headers = caseless();
headers.set('Content-Type', 'application/json');
Get a header
This feature allows you to retrieve a header value in a case-insensitive manner. The 'get' method will return the value regardless of the case of the input header name.
const caseless = require('caseless');
const headers = caseless();
headers.set('Content-Type', 'application/json');
const contentType = headers.get('content-type');
Check if a header exists
This feature allows you to check if a header exists in a case-insensitive manner. The 'has' method returns a boolean indicating the presence of the header.
const caseless = require('caseless');
const headers = caseless();
headers.set('Content-Type', 'application/json');
const hasContentType = headers.has('content-type');
Delete a header
This feature allows you to delete a header in a case-insensitive manner. The 'del' method removes the header regardless of the case of the input header name.
const caseless = require('caseless');
const headers = caseless();
headers.set('Content-Type', 'application/json');
headers.del('content-type');
The 'http-headers' package provides a similar functionality for managing HTTP headers in a case-insensitive manner. It offers methods to get, set, and delete headers, but it is more focused on parsing and formatting HTTP headers.
The 'header-case-normalizer' package focuses on normalizing the case of HTTP headers. It ensures that headers are stored in a consistent case format, similar to 'caseless', but it does not provide as many utility methods for managing headers.
While 'node-fetch' is primarily a library for making HTTP requests, it includes built-in support for case-insensitive headers. It provides methods to set, get, and delete headers in a case-insensitive manner, similar to 'caseless'.
npm install --save @types/caseless
This package contains type definitions for caseless (https://github.com/mikeal/caseless).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/caseless.
type KeyType = string;
type ValueType = any;
type RawDict = object;
declare function caseless(dict?: RawDict): caseless.Caseless;
declare namespace caseless {
function httpify(resp: object, headers: RawDict): Caseless;
interface Caseless {
set(name: KeyType, value: ValueType, clobber?: boolean): KeyType | false;
set(dict: RawDict): void;
has(name: KeyType): KeyType | false;
get(name: KeyType): ValueType | undefined;
swap(name: KeyType): void;
del(name: KeyType): boolean;
}
interface Httpified {
headers: RawDict;
setHeader(name: KeyType, value: ValueType, clobber?: boolean): KeyType | false;
setHeader(dict: RawDict): void;
hasHeader(name: KeyType): KeyType | false;
getHeader(name: KeyType): ValueType | undefined;
removeHeader(name: KeyType): boolean;
}
}
export = caseless;
These definitions were written by downace, Matt R. Wilson, and Emily Klassen.
FAQs
TypeScript definitions for caseless
The npm package @types/caseless receives a total of 2,229,119 weekly downloads. As such, @types/caseless popularity was classified as popular.
We found that @types/caseless demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.