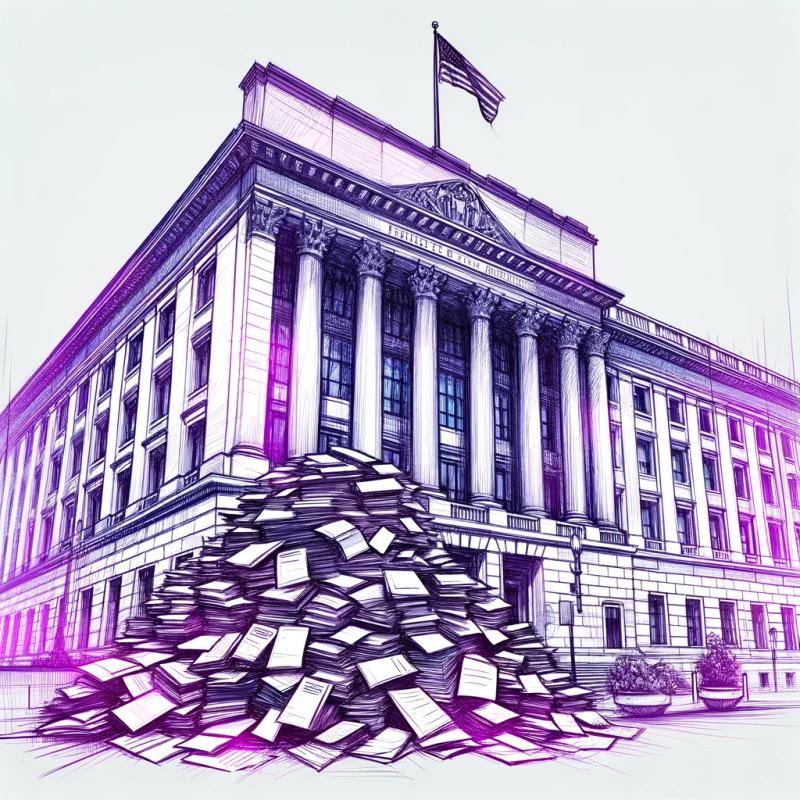
Security News
White House to Tackle Cybersecurity Regulation Fragmentation: CISOs Spend up to 50% of Their Time on Compliance
The White House is addressing fragmented cybersecurity regulations as CISOs report spending up to 50% of their time on compliance, aiming to harmonize requirements and improve cybersecurity outcomes.