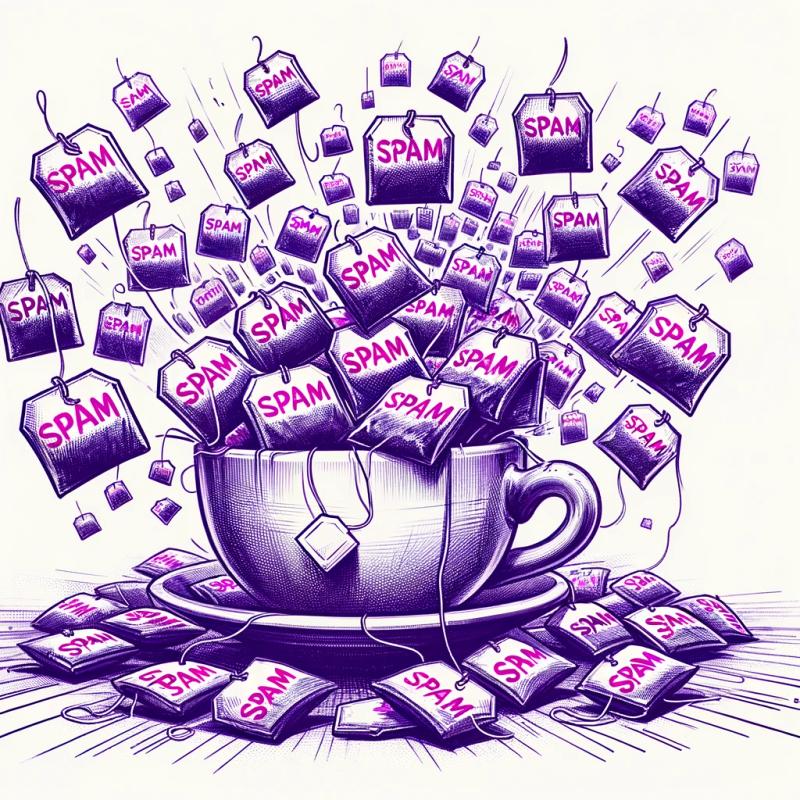
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@types/http-errors
Advanced tools
Readme
npm install --save @types/http-errors
This package contains type definitions for http-errors (https://github.com/jshttp/http-errors).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/http-errors.
These definitions were written by Tanguy Krotoff, and BendingBender.
FAQs
TypeScript definitions for http-errors
The npm package @types/http-errors receives a total of 11,661,386 weekly downloads. As such, @types/http-errors popularity was classified as popular.
We found that @types/http-errors demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.