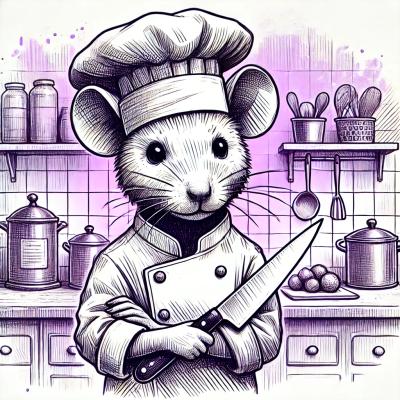
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@types/passport-jwt
Advanced tools
TypeScript definitions for passport-jwt
@types/passport-jwt provides TypeScript type definitions for the passport-jwt library, which is a Passport strategy for authenticating with a JSON Web Token (JWT). This package allows developers to use TypeScript's type-checking features when working with passport-jwt, ensuring better code quality and reducing runtime errors.
JWT Strategy Configuration
This feature allows you to configure the JWT strategy for Passport. The code sample demonstrates how to set up the strategy with options for extracting the JWT from the authorization header and specifying the secret key.
const JwtStrategy = require('passport-jwt').Strategy;
const ExtractJwt = require('passport-jwt').ExtractJwt;
const opts = {
jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(),
secretOrKey: 'your_jwt_secret'
};
passport.use(new JwtStrategy(opts, (jwt_payload, done) => {
User.findOne({id: jwt_payload.sub}, (err, user) => {
if (err) {
return done(err, false);
}
if (user) {
return done(null, user);
} else {
return done(null, false);
}
});
}));
JWT Authentication Middleware
This feature allows you to protect routes using JWT authentication. The code sample shows how to use the configured JWT strategy as middleware in an Express route to protect it from unauthorized access.
const express = require('express');
const passport = require('passport');
const app = express();
app.use(passport.initialize());
app.get('/protected', passport.authenticate('jwt', { session: false }), (req, res) => {
res.json({ message: 'You have accessed a protected route!' });
});
@types/passport provides TypeScript type definitions for the core Passport library. While @types/passport-jwt focuses on JWT authentication, @types/passport provides types for the core Passport functionalities, including session management and other authentication strategies.
@types/jsonwebtoken provides TypeScript type definitions for the jsonwebtoken library, which is used to sign and verify JWTs. While @types/passport-jwt is used for integrating JWTs with Passport, @types/jsonwebtoken focuses on the creation and validation of JWTs themselves.
@types/express provides TypeScript type definitions for the Express framework. While it does not directly relate to JWT authentication, it is often used in conjunction with @types/passport-jwt to build web applications that require authentication.
npm install --save @types/passport-jwt
This package contains type definitions for passport-jwt (https://github.com/themikenicholson/passport-jwt).
Files were exported from https://github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/passport-jwt.
These definitions were written by TANAKA Koichi, Alex Young, Carlos Eduardo Scheffer, Byungjin Kim, and Svyatoslav Bychkov.
FAQs
TypeScript definitions for passport-jwt
The npm package @types/passport-jwt receives a total of 257,153 weekly downloads. As such, @types/passport-jwt popularity was classified as popular.
We found that @types/passport-jwt demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.