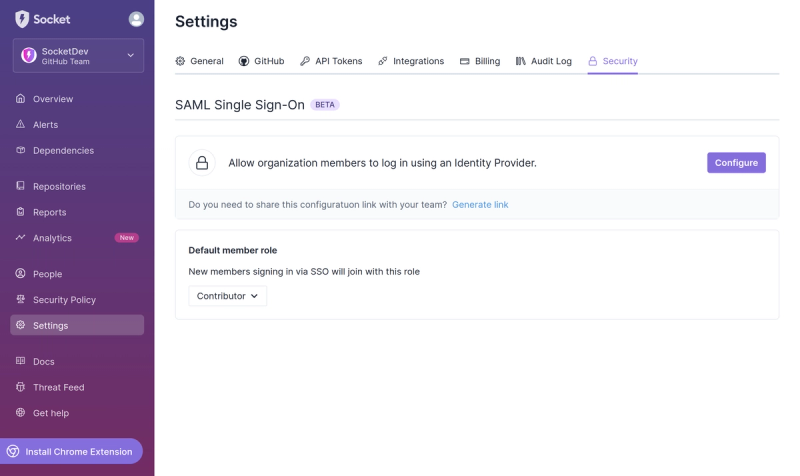
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@uiw/react-date-input
Advanced tools
Readme
显示一个月的日历,并允许用户选择单个日期。
import { DateInput, DateInputRange } from 'uiw';
// or
import DateInput,{ DateInputRange } from '@uiw/react-date-input';
// 在 `v4.13.0` 版本中增加 DateInputRange 组件
import React from 'react';
import { DateInput, DateInputRange, Row, Col } from 'uiw';
export default function Demo () {
const [dataRange,dataRangeSet] =React.useState(['2022/02/25 15:06:24','2022/02/27 14:47:32'])
function onChange(selectedDate,dataRange) {
console.log('selectedDate',selectedDate,dataRange)
}
return (
<div>
<Row style={{ marginBottom:10 }}>
<Col style={{ width: 200 }} fixed>
<DateInput
value={new Date()}
datePickerProps={{ todayButton: '今天' }}
onChange={onChange}
/>
</Col>
<Col style={{ width: 200, marginLeft: 10 }} fixed>
<DateInput
value={new Date()}
disabled
onChange={onChange}
/>
</Col>
<Col style={{ width: 400, marginLeft: 10 }} fixed>
<DateInputRange
bodyStyle={{ width: 400 }}
format="YYYY/MM/DD HH:mm:ss"
value={dataRange}
datePickerProps={{ todayButton: '今天',showTime:true }}
onChange={onChange}
/>
</Col>
</Row>
</div>
)
}
在 <Form />
表单中应用 <DateInput />
组件。
import React from 'react';
import { DateInput,DateInputRange, Notify, Button, Form, Row, Col } from 'uiw';
export default function Demo() {
const form = React.useRef(null)
const resetDateRange = () => {
form.current.resetForm()
}
const setDateRange = () => {
form.current.setFields({dateRange:[new Date(),new Date()]})
}
return (
<Form
ref={form}
onSubmit={({ initial, current }) => {
if (current.date) {
Notify.success({
title: '提交成功!',
description: `表单提交时间成功,时间为:${current.date} range:${current.dateRange}`,
});
} else {
Notify.error({
title: '提交失败!',
description: `表单提交时间成功,时间为:${current.date} range:${current.dateRange},将自动填充初始化值!`,
});
}
}}
fields={{
date: {
initialValue: '2019/02/17',
labelClassName: 'fieldLabel',
labelFor: 'date-inline',
children: <DateInput datePickerProps={{ todayButton: '今天' }} id="date-inline" />
},
dateRange: {
initialValue: ['2019/02/17', '2019/02/20'],
labelClassName: 'fieldLabel',
labelFor: 'date-inline',
children: <DateInputRange datePickerProps={{ todayButton: '今天' }} id="date-inline" />
},
}}
>
{({ fields, state, canSubmit }) => {
return (
<div>
<Row>
<Col style={{ width: 200 }} fixed>{fields.date}</Col>
<Col fixed>{fields.dateRange}</Col>
</Row>
<Row>
<Col style={{ overflow: 'auto', height: 130 }}>
<pre>
{JSON.stringify(state, null, 2)}
</pre>
</Col>
</Row>
<Row gutter={10}>
<Col>
<Button disabled={!canSubmit()} type="primary" htmlType="submit">提交</Button>
<Button onClick={resetDateRange} >重置</Button>
<Button onClick={setDateRange}>setValue</Button>
</Col>
</Row>
</div>
)
}}
</Form>
)
}
import React from 'react';
import { DateInput } from 'uiw';
class Demo extends React.Component {
onChange(selectedDate) {
console.log('selectedDate:', selectedDate);
}
render() {
return (
<div style={{ width: 200 }}>
<DateInput
format="YYYY # MM # DD"
datePickerProps={{ todayButton: '今天' }}
onChange={this.onChange.bind(this)}
/>
</div>
)
}
}
export default Demo;
import React from 'react';
import { DateInput } from 'uiw';
class Demo extends React.Component {
onChange(selectedDate) {
console.log('selectedDate:', selectedDate);
}
render() {
return (
<div style={{ width: 200 }}>
<DateInput
format="YYYY/MM/DD HH:mm:ss"
datePickerProps={{ showTime: true, todayButton: '今天' }}
onChange={this.onChange.bind(this)}
/>
</div>
)
}
}
export default Demo;
import React from 'react';
import { DateInput } from 'uiw';
class Demo extends React.Component {
onChange(selectedDate) {
console.log('selectedDate:', selectedDate);
}
render() {
return (
<div style={{ maxWidth: 200 }}>
<DateInput
autoClose={true}
format="YYYY/MM/DD HH:mm:ss"
datePickerProps={{ showTime: true, todayButton: '今天' }}
onChange={this.onChange.bind(this)}
/>
</div>
)
}
}
export default Demo;
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
value | 初始时间值 | Date | - |
placeholder | 输入框提示文字 | String | - |
allowClear | 是否显示清除按钮 | Boolean | true |
format | 格式化时间,规则查看 <formatter> 文档 | String | YYYY/MM/DD |
onChange | 选择一天时调用。 | Function(selectedDate:Date) | - |
popoverProps | 将参数传递给 <Popover> 组件 | Object | - |
datePickerProps | 将参数传递给 <DatePicker> 组件 | Object | - |
disabled | 组件 <Input> 的属性,禁用日历 | Boolean | - |
autoClose | 是否自动关闭弹层 | Boolean | false |
组件 DateInput
继承 <Input>
组件,更多属性文档请参考 <Input>
。
在 v4.13.0
版本中增加 DateInputRange
组件。
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
value | 初始时间值 | Array<Date, string> | - |
placeholder | 输入框提示文字 | String | - |
allowClear | 是否显示清除按钮 | Boolean | true |
format | 格式化时间,规则查看 <formatter> 文档 | String | YYYY/MM/DD |
onChange | 选择一天时调用。 | Function(selectedDate:Date, dateRange: Array<Date>) | - |
popoverProps | 将参数传递给 <Popover> 组件 | Object | - |
datePickerProps | 将参数传递给 <DatePicker> 组件 | Object | - |
disabled | 组件 <Input> 的属性,禁用日历 | Boolean | - |
bodyStyle | 选择框样式 | Object | { width: 300 } |
组件 DateInputRange
继承 <Input>
组件,更多属性文档请参考 <Input>
。
FAQs
DateInput component
The npm package @uiw/react-date-input receives a total of 250 weekly downloads. As such, @uiw/react-date-input popularity was classified as not popular.
We found that @uiw/react-date-input demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.