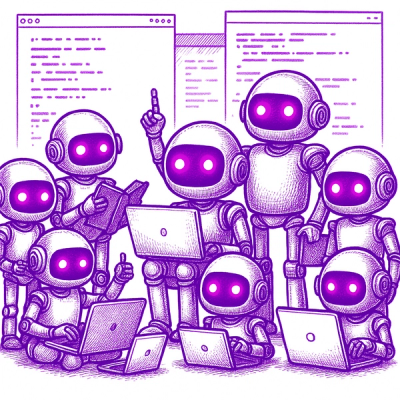
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
@uiw/react-time-picker
Advanced tools
当用户需要输入一个时间,可以点击标准输入框,弹出时间面板进行选择。
import { TimePicker } from 'uiw';
// or
import TimePicker from '@uiw/react-time-picker';
import React from 'react';
import { TimePicker, Row, Col } from 'uiw';
const Demo = () => (
<Row gutter={10}>
<Col style={{ width: 200 }} fixed>
<TimePicker
onChange={(formatDate, date) => {
console.log('--->', formatDate, date);
}}
/>
</Col>
<Col style={{ width: 200 }} fixed>
<TimePicker format="HH:mm" precision="minute" />
</Col>
<Col style={{ width: 200 }} fixed>
<TimePicker format="HH" precision="hour" />
</Col>
</Row>
)
export default Demo;
import React from 'react';
import { TimePicker, Row, Col } from 'uiw';
const Demo = () => {
const value = new Date(2018, 1, 24, 4,5,35);
return (
<Row gutter={10}>
<Col style={{ width: 200 }} fixed>
<TimePicker value={value} />
</Col>
<Col style={{ width: 200 }} fixed>
<TimePicker value={value} format="HH:mm" precision="minute" />
</Col>
<Col style={{ width: 200 }} fixed>
<TimePicker value={value} format="HH" precision="hour" />
</Col>
</Row>
)
}
export default Demo;
import React from 'react';
import { TimePicker, Row, Col } from 'uiw';
const Demo = () => {
const value = new Date(2018, 1, 24, 4,5,35);
return (
<Row gutter={10}>
<Col style={{ width: 200 }} fixed>
<TimePicker size="small" value={value} />
</Col>
<Col style={{ width: 200 }} fixed>
<TimePicker value={value} format="HH" precision="hour" />
</Col>
<Col style={{ width: 200 }} fixed>
<TimePicker size="large" value={value} format="HH:mm" precision="minute" />
</Col>
</Row>
)
}
export default Demo;
在表单返回的数据,并没有将 format
格式化后的数据返回给你,而是返回的一个 Date
,你可以通过 formatter
重新格式化。
import React from 'react';
import { TimePicker, Notify, Row, Col, Form, Button } from 'uiw';
const Demo = () => (
<div>
<Form
onSubmit={({initial, current}) => {
console.log('-->>', initial, current);
if(current.date) {
Notify.success({
title: '提交成功!',
description: `表单提交时间成功,时间为:${formatter('HH:mm:ss', current.date)}`,
});
} else {
Notify.error({
title: '提交失败!',
description: <span>表单提交时间成功,时间为:<b>空</b>,将自动填充初始化值!</span>,
});
}
}}
fields={{
date: {
labelClassName: 'fieldLabel',
labelFor: 'date-inline',
children: <TimePicker />
},
}}
>
{({ fields, state, canSubmit }) => {
return (
<Row gutter={10}>
<Col style={{ width: 200 }} fixed>{fields.date}</Col>
<Col>
<Button disabled={!canSubmit()} type="primary" htmlType="submit">提交</Button>
</Col>
</Row>
)
}}
</Form>
</div>
)
export default Demo;
可以使用 disabledHours
disabledMinutes
disabledSeconds
禁用部分时间选择。
import React from 'react';
import { TimePicker, Row, Col } from 'uiw';
const Demo = () => (
<Row gutter={10} style={{ maxWidth: 360 }}>
<Col style={{ width: 200 }} fixed>
<TimePicker
disabledMinutes={(minute, type) => {
if (minute % 15 !== 0) {
return true;
}
}}
disabledHours={(hour, type, date) => {
// console.log('~~:', hour, type, date);
if (hour < 3) {
return true;
}
}}
/>
</Col>
<Col style={{ width: 200 }} fixed>
<TimePicker disabled value={new Date(2018, 1, 24, 4,5,35)} />
</Col>
</Row>
)
export default Demo;
可以使用 hideDisabled
将禁用的部分时间隐藏。
import React from 'react';
import { TimePicker, Row, Col } from 'uiw';
const Demo = () => (
<Row gutter={10} style={{ maxWidth: 360 }}>
<Col style={{ width: 200 }} fixed>
<TimePicker
hideDisabled
disabledMinutes={(minute, type) => {
if (minute % 15 !== 0) {
return true;
}
}}
disabledHours={(hour, type, date) => {
// console.log('~~:', hour, type, date);
if (hour < 3) {
return true;
}
}}
/>
</Col>
</Row>
)
export default Demo;
可以使用 hideDisabled
将禁用的部分时间隐藏。
import React from 'react';
import { TimePicker, Row, Col } from 'uiw';
const Demo = () => (
<Row gutter={10} style={{ maxWidth: 360 }}>
<Col style={{ width: 200 }} fixed>
<TimePicker
hideDisabled
disabledMinutes={(minute, date) => {
if (minute % 15 !== 0) {
return true;
}
}}
disabledSeconds={(second, date) => {
if (second % 15 !== 0) {
return true;
}
}}
/>
</Col>
</Row>
)
export default Demo;
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
value | 初始时间值 | Date | - |
placeholder | 输入框提示文字 | String | - |
format | 格式化时间,规则查看 formatter 文档 | Function | HH:mm:ss |
precision | 选择时间精确度 | Enum{hour , minute , second } | false |
disabled | 禁用全部操作 | Boolean | false |
disabledHours | 禁止选择部分小时选项 | Function(hour, type{ Hours , Minutes , Seconds }, selectedDate) | - |
disabledMinutes | 禁止选择部分分钟选项 | Function(minute, type{ Hours , Minutes , Seconds }, selectedDate) | - |
disabledSeconds | 禁止选择部分秒选项 | Function(second, type{ Hours , Minutes , Seconds }, selectedDate) | - |
hideDisabled | 不可选择的项隐藏 | Boolean | false |
onChange | 时间选择的回调函数 | Function(formatDate, Date, type{ Hours , Minutes , Seconds }, , num, disableds) | - |
uiw@3.3.0+
组件集成部分 Input,不再通过 inputProps 属性传值,更多属性请参考 Input
uiw@3.3.0+
将不支持此属性
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
<Input> 组件 | Object | - |
参数 | 说明 | 类型 | 默认值 |
---|---|---|---|
popoverProps | 将参数传递给 <Popover> 组件 | Object | - |
FAQs
TimePicker component
The npm package @uiw/react-time-picker receives a total of 246 weekly downloads. As such, @uiw/react-time-picker popularity was classified as not popular.
We found that @uiw/react-time-picker demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.