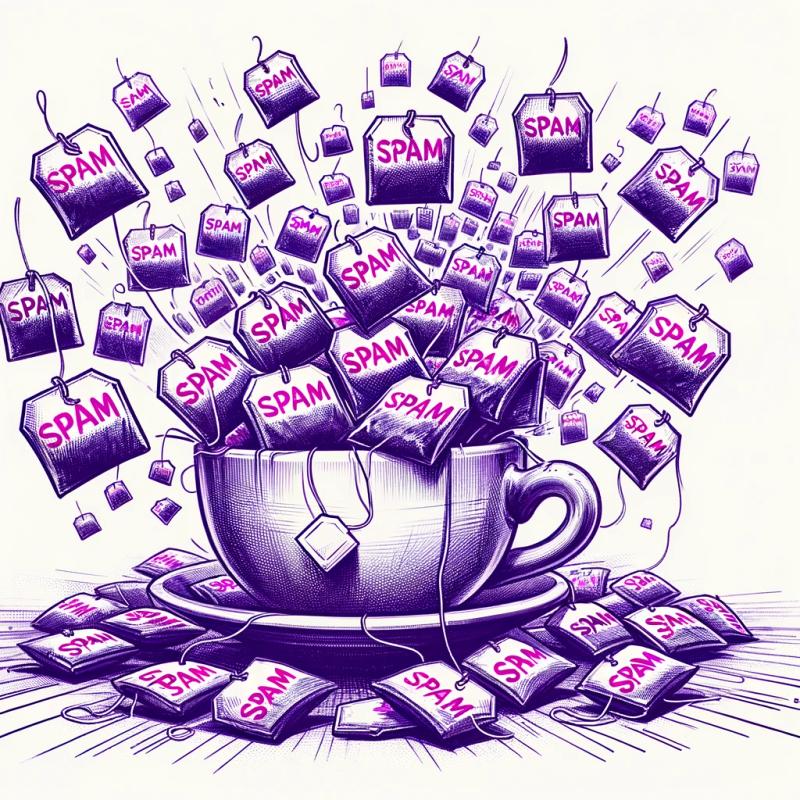
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@ukab/container
Advanced tools
Readme
Container is a IoC packge for NodeJS. This package supports both ESM and CommonJs modules.
npm i @ukab/container
Note: You should install and import reflect-metadata before using this package.
1. using default container
import 'reflect-metadata';
import { Container, Injectable } from '@ukab/container';
class Router {
test() {
console.log('router test called')
}
}
// register router
Container.register(Router);
@Injectable()
class Test {
constructor(public router: Router) { }
test() {
this.router.test()
}
}
// register and get resolved instance
const instance = Container.resolve<Test>(Test);
instance.test();
2. creating and using container
import 'reflect-metadata';
import { Container, Injectable } from '@ukab/container';
const myContainer = Container.of('myContainer');
class Router {
test() {
console.log('router test called')
}
}
// register router
myContainer.register(Router);
@Injectable()
class Test {
constructor(public router: Router) { }
test() {
this.router.test()
}
}
// register and get resolved instance
const instance = myContainer.resolve<Test>(Test);
instance.test();
3. using provider with custom identity
import 'reflect-metadata';
import { Inject, Injectable, Container } from '@ukab/container';
class Router {
test() {
console.log('router test called')
}
}
// register router with custom identity
Container.register(Router, 'IRouter');
interface IRouter {
test(): void
}
@Injectable()
class Test {
constructor(@Inject('IRouter') public router: IRouter) {
}
test() {
this.router.test();
}
}
// register and get resolved instance
const instance = Container.resolve<Test>(Test);
instance.test();
4. injecting custom dependency in method
import 'reflect-metadata';
import { Inject, Injectable, Container } from '@ukab/container';
class Router {
test() {
console.log('router test called')
}
}
// register router with custom identity
Container.register(Router, 'IRouter');
interface IRouter {
test(): void
}
class User {
test() {
console.log('user test called')
}
}
// register user service
Container.register(User);
@Injectable()
class Test {
@Inject()
user: User
constructor(@Inject('IRouter') public router: IRouter) {
}
test(@Inject('p1') p1: number) {
this.router.test();
this.user.test();
console.log(p1);
}
}
Container.value<number>('p1', 1);
// register and call method with param injection
Container.register<Test>(Test);
Container.call(Test, 'test');
5. using context container
import 'reflect-metadata';
import { Inject, Injectable, Container, IocContext, Scope } from '.';
const container = Container.of('test');
@Injectable({ scope: Scope.Contextual })
class Test {
constructor(@Inject('p1') public p1: number) {
}
test() {
console.log('p1 value', this.p1);
}
}
container.register<Test>(Test);
// this context will use the given container
const context1 = new IocContext('random context id', container);
context1.set<string, number>('p1', 2);
// this context will use the given container
const context2 = new IocContext('random context id', container);
context2.set<string, number>('p1', 3);
const testFromContext2 = context2.get<Test>(Test);
testFromContext2.test() // p1 value 3
const testFromContext1 = context1.get<Test>(Test);
testFromContext1.test() // p1 value 2
FAQs
Ukab Ecosystem ioc container library
The npm package @ukab/container receives a total of 0 weekly downloads. As such, @ukab/container popularity was classified as not popular.
We found that @ukab/container demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.