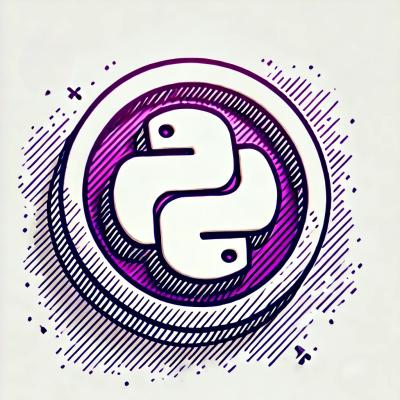
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
@unirep/crypto
Advanced tools
Client library for cryptography related functions which are used in unirep protocol.
Client library for cryptography related functions which are used in unirep protocol.
Install the @unirep/crypto
package with npm:
npm i @unirep/crypto
or yarn:
yarn add @unirep/crypto
Generate a random ZkIdentity
import { ZkIdentity } from '@unirep/crypto'
const identity = new ZkIdentity()
Generate identity commitment
const commitment = identity.genIdentityCommitment()
Get identity nullifier
const commitment = identity.identityNullifier
get identity trapdoor
const commitment = identity.trapdoor
Serialize/ unserialize identity
import { Strategy } from '@unirep/crypto'
// serialize identity
const serializedIdentity = identity.serializeIdentity()
// unserialize identity
const unserializedIdentity = new ZkIdentity(
Strategy.SERIALIZED,
serializedIdentity
)
Create a IncrementalMerkleTree
import { IncrementalMerkleTree } from '@unirep/crypto'
const depth = 4
// initialize incremental merkle tree with depth 4
const tree = new IncrementalMerkleTree(depth)
Get tree root
const root = tree.root
Insert leaf
const leaf = 1
tree.insert(leaf)
Generate merkle proof
const index = 0
const proof = tree.createProof(index)
Verify merkle proof
const isValid = tree.verifyProof(proof)
Create a SparseMerkleTree
import { SparseMerkleTree } from '@unirep/crypto'
const depth = 4
// initialize incremental merkle tree with depth 4
const zeroHash = 0
// initialize sparse merkle tree with depth 4 and zeroHash 0
const tree = new SparseMerkleTree(
depth,
zeroHash
)
Get tree root
const root = tree.root
Update leaf
const leafKey = BigInt(3)
const leafValue = BigInt(4)
tree.update(leafKey, leafValue)
Generate merkle proof
const leafKey = Bigint(1)
const proof = tree.createProof(leafKey)
Verify merkle proof
const isValid = tree.verifyProof(proof)
genRandomSalt
import { genRandomSalt } from '@unirep/crypto'
// generate random BigInt
const salt = genRandomSalt()
hash5
import { hash5 } from '@unirep/crypto'
// poseidon hash 5 BigInt elements
const values = [
genRandomSalt(),
genRandomSalt(),
genRandomSalt(),
genRandomSalt(),
genRandomSalt(),
]
const hash5Value = hash5(values)
hashOne
import { hashOne } from '@unirep/crypto'
// poseidon hash 1 BigInt elements
const value = genRandomSalt()
const hashOneValue = hashOne(value)
hashLeftRight
import { hashLeftRight } from '@unirep/crypto'
// poseidon hash 2 BigInt elements
const leftValue = genRandomSalt()
const rightValue = genRandomSalt()
const hash = hashLeftRight(leftValue, rightValue)
stringifyBigInts/unstringifyBigInts
const values = {
input1: genRandomSalt(),
input2: genRandomSalt(),
input3: genRandomSalt(),
}
// stringify BigInt elements with stringifyBigInts function
const stringifiedValues = stringifyBigInts(values)
// it can be recoverd by unstringifyBigInts function
const unstringifiedValues = unstringifyBigInts(stringifiedValues)
FAQs
Client library for cryptography related functions which are used in unirep protocol.
The npm package @unirep/crypto receives a total of 0 weekly downloads. As such, @unirep/crypto popularity was classified as not popular.
We found that @unirep/crypto demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.