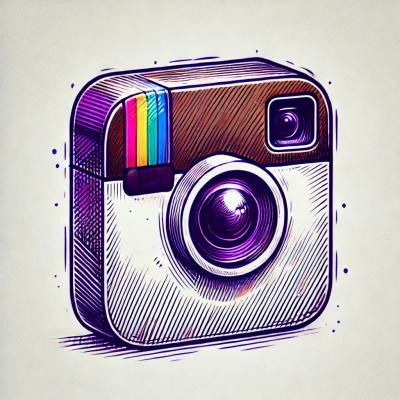
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
@vue/server-renderer
Advanced tools
The @vue/server-renderer package is designed for server-side rendering (SSR) of Vue.js applications. It allows developers to render Vue components on the server and send the rendered HTML to the client, improving the initial load time and SEO for Vue.js applications. This package is part of the Vue.js ecosystem and is specifically tailored for Vue 3.x applications.
Rendering a Vue Component to a String
This feature allows you to render a Vue component to an HTML string on the server. This is useful for generating the initial HTML for a page on the server, which can then be sent to the client for faster initial load times and better SEO.
const { createSSRApp } = require('vue');
const { renderToString } = require('@vue/server-renderer');
const app = createSSRApp({
data: () => ({ msg: 'Hello, server-side rendering!' }),
template: '<div>{{ msg }}</div>'
});
renderToString(app).then(html => {
console.log(html);
});
Streaming a Vue Component
This feature enables streaming rendering of a Vue component. Instead of waiting for the entire component to be rendered before sending it to the client, the rendered output is streamed to the client as it's generated. This can improve the perceived performance of the application.
const { createSSRApp } = require('vue');
const { renderToNodeStream } = require('@vue/server-renderer');
const express = require('express');
const app = express();
const vueApp = createSSRApp({
data: () => ({ msg: 'Hello, streaming SSR!' }),
template: '<div>{{ msg }}</div>'
});
app.get('/', (req, res) => {
res.setHeader('Content-Type', 'text/html');
const stream = renderToNodeStream(vueApp);
stream.pipe(res);
});
app.listen(3000);
Note: as of 3.2.13+, this package is included as a dependency of the main vue
package and can be accessed as vue/server-renderer
. This means you no longer need to explicitly install this package and ensure its version match that of vue
's. Just use the vue/server-renderer
deep import instead.
renderToString
Signature
function renderToString(
input: App | VNode,
context?: SSRContext,
): Promise<string>
Usage
const { createSSRApp } = require('vue')
const { renderToString } = require('@vue/server-renderer')
const app = createSSRApp({
data: () => ({ msg: 'hello' }),
template: `<div>{{ msg }}</div>`,
})
;(async () => {
const html = await renderToString(app)
console.log(html)
})()
If the rendered app contains teleports, the teleported content will not be part of the rendered string. Instead, they are exposed under the teleports
property of the ssr context object:
const ctx = {}
const html = await renderToString(app, ctx)
console.log(ctx.teleports) // { '#teleported': 'teleported content' }
renderToNodeStream
Renders input as a Node.js Readable stream.
Signature
function renderToNodeStream(input: App | VNode, context?: SSRContext): Readable
Usage
// inside a Node.js http handler
renderToNodeStream(app).pipe(res)
Note: This method is not supported in the ESM build of @vue/server-renderer
, which is decoupled from Node.js environments. Use pipeToNodeWritable
instead.
pipeToNodeWritable
Render and pipe to an existing Node.js Writable stream instance.
Signature
function pipeToNodeWritable(
input: App | VNode,
context: SSRContext = {},
writable: Writable,
): void
Usage
// inside a Node.js http handler
pipeToNodeWritable(app, {}, res)
renderToWebStream
Renders input as a Web ReadableStream.
Signature
function renderToWebStream(
input: App | VNode,
context?: SSRContext,
): ReadableStream
Usage
// inside an environment with ReadableStream support
return new Response(renderToWebStream(app))
Note: in environments that do not expose ReadableStream
constructor in the global scope, pipeToWebWritable
should be used instead.
pipeToWebWritable
Render and pipe to an existing Web WritableStream instance.
Signature
function pipeToWebWritable(
input: App | VNode,
context: SSRContext = {},
writable: WritableStream,
): void
Usage
This is typically used in combination with TransformStream
:
// TransformStream is available in environments such as CloudFlare workers.
// in Node.js, TransformStream needs to be explicitly imported from 'stream/web'
const { readable, writable } = new TransformStream()
pipeToWebWritable(app, {}, writable)
return new Response(readable)
renderToSimpleStream
Renders input in streaming mode using a simple readable interface.
Signature
function renderToSimpleStream(
input: App | VNode,
context: SSRContext,
options: SimpleReadable,
): SimpleReadable
interface SimpleReadable {
push(content: string | null): void
destroy(err: any): void
}
Usage
let res = ''
renderToSimpleStream(
app,
{},
{
push(chunk) {
if (chunk === null) {
// done
console(`render complete: ${res}`)
} else {
res += chunk
}
},
destroy(err) {
// error encountered
},
},
)
FAQs
@vue/server-renderer
The npm package @vue/server-renderer receives a total of 4,633,174 weekly downloads. As such, @vue/server-renderer popularity was classified as popular.
We found that @vue/server-renderer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.