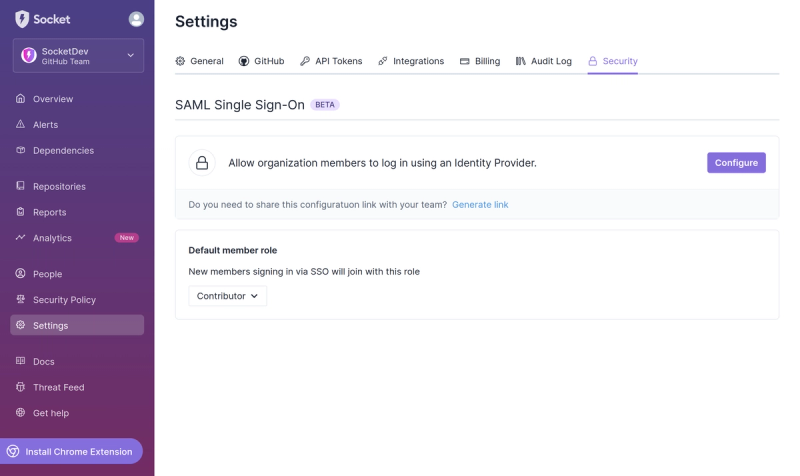
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
abex-react
Advanced tools
Readme
abex-react
is a React package that provides components and utilities for handling A/B testing experiments using the Abex platform. It allows you to define and render different content based on assigned variant keys. The package includes the following modules:
To install abex-react
, you can use npm or yarn:
npm install abex-react
or
yarn add abex-react
The AbexProvider
component is used to wrap your application and provide access to the variant keys for experiments. It serves as the context provider for the child components that rely on A/B testing experiments. The AbexProvider
component accepts the following props:
Prop | Type | Required | Description |
---|---|---|---|
product | string | Yes | The product or module within your application. |
screen | string | Yes | The screen or page within the specified product. |
component | string | Yes | The specific component within the given screen. |
experimentData | object | Yes | An object containing experiment data |
onInit | function | No | An optional callback function called after the AbexClient instance is created. |
children | ReactNode | Yes | The child components to be wrapped by the AbexProvider . |
import React from 'react';
import { AbexProvider } from 'abex-react';
const App = () => {
return (
<AbexProvider
product="product1"
screen="screen1"
component="component1"
experimentData={{
utm_source: 'scaler',
}}
>
{/* Your application components */}
</AbexProvider>
);
};
onInit
Callback:import React from 'react';
import { AbexProvider } from 'abex-react';
const onProviderInit = (client) => {
console.log('AbexClient instance created:', client);
// Perform initialization or other tasks with the client
};
const App = () => {
return (
<AbexProvider
product="product1"
screen="screen1"
component="component1"
experimentData={{
utm_source: 'scaler',
}}
onInit={onProviderInit}
>
{/* Your application components */}
</AbexProvider>
);
};
In the examples above, we demonstrate the usage of the AbexProvider
component. In the first example, we provide an array of experiments to the experiments
prop, and in the second example, we also specify an onInit
callback function that will be called after the AbexClient
instance is created. The AbexProvider
component wraps your application components, making the variant keys available through the AbexContext
for child components to access and render different content based on the assigned variant keys.
abexClient.identify
To identify the user and associate a user ID with the AbexClient
instance, you can use the abexClient.identify(userID)
method. This allows you to retrieve the user ID and use it as a token for A/B testing experiments.
Here's an example of how to use abexClient.identify
in conjunction with AbexProvider
:
import React from 'react';
import { AbexProvider } from 'abex-react';
import AbexClient from '../utils/abexClient';
const experimentsData = {
utmSource: 'scaler_event',
}
const onProviderInit = (client) => {
console.log('AbexClient instance created:', client);
// Perform initialization or other tasks with the client
client.identify('user123'); // Identify the user with a user ID
};
const App = () => {
return (
<AbexProvider
product="exampleProduct"
screen="exampleScreen"
component="exampleComponent"
experimentData={experimentsData}
onInit={onProviderInit}
>
{/* Your application components */}
</AbexProvider>
);
};
In this example, we define an onProviderInit
callback function, which is called after the AbexClient
instance is created. Inside this callback, we have access to the AbexClient
instance, and we use the identify
method to associate the user with the ID 'user123'
. By identifying the user, the AbexClient
instance will use the user ID as a token when retrieving variant keys for experiments.
Note: Make sure to adapt the example to your specific use case and replace 'user123'
with the appropriate user ID.
By combining the abexClient.identify
method with AbexProvider
, you can effectively identify the user and leverage the assigned variant keys in your A/B testing experiments.
The ExperimentsProvider
component is an integral part of the abex-react
package, used in conjunction with the AbexProvider
to efficiently cache and manage experiment data throughout your application. It forms the foundation for optimizing the distribution of experiment information, leading to improved performance and consistency in your A/B testing implementation.
The ExperimentsProvider
component is internally employed within the AbexProvider
, and its implementation is abstracted away from the direct usage of developers. It plays a crucial role in caching and distributing experiment data, ensuring that your application efficiently utilizes A/B testing experiments without causing unnecessary network requests.
The ExperimentsProvider
is used as a caching mechanism within the AbexProvider
component. Here's how it works:
Initialization: When the AbexProvider
is initialized with experiment data, it creates an instance of the ExperimentsProvider
.
Caching: The ExperimentsProvider
stores experiment data in its internal state, caching it for subsequent use. This prevents the need for repeated fetching of experiment data from the Abex platform.
Distribution: As child components of the AbexProvider
are rendered, they can access the cached experiment data from the ExperimentsProvider
context. This eliminates the need for each component to fetch experiment data individually.
Optimized Performance: By centralizing the storage and distribution of experiment data, the ExperimentsProvider
ensures that components within the AbexProvider
scope can access cached data efficiently, resulting in optimized rendering and responsiveness.
The ExperimentsProvider
is used within the AbexProvider
to manage experiment data. Here's an example of how the AbexProvider
integrates the ExperimentsProvider
:
import React from 'react';
import { AbexProvider, ExperimentsProvider } from 'abex-react'; // Import both components
const App = () => {
const experimentsData = {
utmSource: 'scaler_event',
}
return (
<ExperimentsProvider>
{' '}
{/* Use ExperimentsProvider at the root */}
<AbexProvider
product="exampleProduct"
screen="exampleScreen"
component="exampleComponent"
experimentData={experimentsData}
>
{/* Your application components */}
</AbexProvider>
</ExperimentsProvider>
);
};
export default App;
In this example, the ExperimentsProvider
is used internally within the AbexProvider
. The AbexProvider
wraps your application components and effectively utilizes the caching capabilities of the ExperimentsProvider
.
The ExperimentsProvider
brings several benefits to your abex-react
implementation:
Efficient Data Sharing: Experiment data is efficiently shared across components within the AbexProvider
scope, eliminating the need for redundant network requests.
Optimized Performance: Caching experiment data leads to faster rendering and better user experience, as components can access the data without delay.
Consistency: All components within the AbexProvider
receive the same consistent experiment data, ensuring uniformity.
Simplified Implementation: You don't need to manually manage experiment data distribution; the ExperimentsProvider
handles it for you.
In summary, the ExperimentsProvider
within the abex-react
package efficiently manages and caches experiment data, enhancing the performance and consistency of your A/B testing implementation. It collaborates seamlessly with the AbexProvider
to optimize the distribution of experiment information within your application.
The AbexCase
component is used within the AbexSwitch
component to define different cases or variants based on the assigned variant key. It allows rendering different content based on the variant assigned to the user.
<AbexCase variant={variantName}>
{/* Content for the specified variant */}
</AbexCase>
Prop | Type | Required | Description |
---|---|---|---|
variant | string | Yes | The variant key that defines which content to render. |
children | ReactNode | Yes | The content to be rendered when the variant matches. It can be any valid React element or JSX. |
import { AbexCase } from 'abex-react';
const MyComponent = () => {
const variant = 'variant1';
return (
<AbexCase variant={variant}>
<h1>Welcome to Variant 1</h1>
<p>This is the content for variant 1.</p>
</AbexCase>
);
};
In the example above, we define an AbexCase
component with the variant
prop set to 'variant1'
. When the assigned variant key matches 'variant1'
, the content within the AbexCase
component will be rendered. In this case, it renders a heading and a paragraph specific to variant 1.
Use the AbexCase
component within an AbexSwitch
component to handle different cases or variants based on the assigned variant key in your A/B testing implementation.
The AbexSwitch
component is a higher-level React component that conditionally renders content based on the assigned variant key of an A/B testing experiment. It leverages the AbexContext
to retrieve variant keys and render appropriate cases.
Prop | Type | Required | Description |
---|---|---|---|
experimentKey | string | Yes | The experiment key to identify the experiment. |
defaultVariant | string | Yes | The default variant to render while loading or when experiment key is undefined. |
children | ReactNode | Yes | The AbexCase components representing different variants. |
customLoader | ReactNode | No | Custom loader to render while the variant key is being fetched. |
loaderProps | object | No | Additional props to pass to the custom loader (customLoader ). |
import React from 'react';
import { AbexSwitch, AbexCase } from 'abex-react';
const MyComponent = () => {
const experimentKey = 'experiment1';
const defaultVariant = 'defaultVariant';
return (
<AbexSwitch experimentKey={experimentKey} defaultVariant={defaultVariant}>
<AbexCase variant="variant1">
<h1>Welcome to Variant 1</h1>
<p>This is the content for Variant 1.</p>
</AbexCase>
<AbexCase variant="variant2">
<h1>Welcome to Variant 2</h1>
<p>This is the content for Variant 2.</p>
</AbexCase>
<AbexCase variant="defaultVariant">
<h1>Loading Default Variant...</h1>
</AbexCase>
</AbexSwitch>
);
};
import React from 'react';
import { AbexSwitch, AbexCase } from 'abex-react';
const MyComponent = () => {
const experimentKey = 'experiment1';
const defaultVariant = 'defaultVariant';
const CustomLoader = () => (
<div className="custom-loader">
<h1>Loading...</h1>
{/* Add your custom loader UI here */}
</div>
);
return (
<AbexSwitch
experimentKey={experimentKey}
defaultVariant={defaultVariant}
customLoader={<CustomLoader />}
>
<AbexCase variant="variant1">
<h1>Welcome to Variant 1</h1>
<p>This is the content for Variant 1.</p>
</AbexCase>
{/* ... other AbexCase components ... */}
</AbexSwitch>
);
};
import React from 'react';
import { AbexSwitch, AbexCase } from 'abex-react';
const MyComponent = () => {
const experimentKey = 'experiment1';
const defaultVariant = 'defaultVariant';
const CustomLoader = ({ loaderText }) => (
<div className="custom-loader">
<h1>{loaderText}</h1>
{/* Add your custom loader UI here */}
</div>
);
return (
<AbexSwitch
experimentKey={experimentKey}
defaultVariant={defaultVariant}
customLoader={<CustomLoader loaderText="Loading Experiment..." />}
loaderProps={{ loaderText: 'Loading Content...' }}
>
<AbexCase variant="variant1">
<h1>Welcome to Variant 1</h1>
<p>This is the content for Variant 1.</p>
</AbexCase>
{/* ... other AbexCase components ... */}
</AbexSwitch>
);
};
In the example above, the loaderProps prop is used to pass additional props to the custom loader component. This can be useful for customizing the loader's behavior or appearance based on specific needs.
The abexClient
is a utility class provided by the abex-react
package that interacts with the Abex platform APIs and handles A/B testing experiments. It allows you to fetch variant keys for experiments and identify users with a user ID. Here is the documentation for the abexClient
class and its available methods:
The abexClient
constructor creates an instance of the AbexClient
class.
const abexClient = new AbexClient(experiments: { experimentKey: string; experimentData: object; }[], callback?: (client: AbexClient) => void)
Parameter | Type | Description |
---|---|---|
experiments | Array of objects: { experimentKey: string; experimentData: object; } | An array of experiments with their keys and data. |
callback | Function | An optional callback function called after the AbexClient instance is created. |
import AbexClient from 'abexClient';
const product = 'scaler';
const screen = 'events';
const component = 'reg_form';
const experimentsData = {
utmSource: 'scaler_event',
}
const onClientInit = (client) => {
console.log('AbexClient instance created:', client);
// Perform initialization or other tasks with the client
};
const abexClient = new AbexClient(
product,
screen,
component,
experimentsData,
onClientInit
);
In the example above, we create an instance of the AbexClient
class with an array of experiments and an onClientInit
callback function. The onClientInit
function will be called after the AbexClient
instance is created, allowing you to perform any necessary initialization or tasks with the client.
identify
The identify
method is used to associate a user with a user ID in the AbexClient
instance.
abexClient.identify(userID: string): void
Parameter | Type | Description |
---|---|---|
userID | string | The user ID to be associated. |
const userID = 'user123';
abexClient.identify(userID);
In the example above, we use the identify
method to associate the user with the ID 'user123'
. This allows the AbexClient
instance to use the user ID as a token when fetching variant keys for experiments.
getVariantKey
The getVariantKey
method retrieves the variant key for each experiment in the AbexClient
instance.
abexClient.getVariantKey(): Promise<Record<string, string | null>>
abexClient.getVariantKey().then((variantKeys) => {
console.log('Variant Keys:', variantKeys);
// Use the variant keys for rendering different content based on experiments
});
In the example above, we use the getVariantKey
method to fetch the variant keys for experiments. The method returns a promise that resolves to an object containing experiment keys and their corresponding variant keys. You can then use the variant keys to render different content based on the assigned variants.
getVariantsInBatch
The getVariantsInBatch
method retrieves the variant keys for experiments in batch.
abexClient.getVariantsInBatch(): Promise<Record<string, string | null>>
abexClient.getVariantsInBatch().then((variantKeys) => {
console.log('Variant Keys:', variantKeys);
// Use the variant keys for rendering different content based on experiments
});
In the example above, we use the getVariantsInBatch
method to fetch the variant keys for experiments in a batch. The method returns a promise that resolves to an object containing experiment keys and their corresponding variant keys. You can then use the variant keys to render different content based on the assigned variants.
The useAbex
hook provides a simple way to access the variant key for a particular experiment within a component. It takes an experiment key as an argument and returns the variant key associated with that experiment. Example usage:
import React from 'react';
import { useAbex } from 'abex-react';
const MyComponent = () => {
const variantKey = useAbex('experiment1');
return <div>{/* Use the variant key in your component */}</div>;
};
FAQs
`abex-react` is a React package that provides components and utilities for handling A/B testing experiments using the Abex platform. It allows you to define and render different content based on assigned variant keys. The package includes the following mo
The npm package abex-react receives a total of 0 weekly downloads. As such, abex-react popularity was classified as not popular.
We found that abex-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.