abstract-leveldown
Advanced tools
Comparing version 2.4.1 to 2.5.0
@@ -9,2 +9,10 @@ /* Copyright (c) 2013 Rod Vagg, MIT License */ | ||
AbstractChainedBatch.prototype._serializeKey = function (key) { | ||
return this._db._serializeKey(key) | ||
} | ||
AbstractChainedBatch.prototype._serializeValue = function (value) { | ||
return this._db._serializeValue(value) | ||
} | ||
AbstractChainedBatch.prototype._checkWritten = function () { | ||
@@ -22,4 +30,4 @@ if (this._written) | ||
if (!this._db._isBuffer(key)) key = String(key) | ||
if (!this._db._isBuffer(value)) value = String(value) | ||
key = this._serializeKey(key) | ||
value = this._serializeValue(value) | ||
@@ -40,3 +48,3 @@ if (typeof this._put == 'function' ) | ||
if (!this._db._isBuffer(key)) key = String(key) | ||
key = this._serializeKey(key) | ||
@@ -83,2 +91,2 @@ if (typeof this._del == 'function' ) | ||
module.exports = AbstractChainedBatch | ||
module.exports = AbstractChainedBatch |
@@ -82,7 +82,6 @@ /* Copyright (c) 2013 Rod Vagg, MIT License */ | ||
if (err = this._checkKey(key, 'key', this._isBuffer)) | ||
if (err = this._checkKey(key, 'key')) | ||
return callback(err) | ||
if (!this._isBuffer(key)) | ||
key = String(key) | ||
key = this._serializeKey(key) | ||
@@ -109,13 +108,8 @@ if (typeof options != 'object') | ||
if (err = this._checkKey(key, 'key', this._isBuffer)) | ||
if (err = this._checkKey(key, 'key')) | ||
return callback(err) | ||
if (!this._isBuffer(key)) | ||
key = String(key) | ||
key = this._serializeKey(key) | ||
value = this._serializeValue(value) | ||
// coerce value to string in node, don't touch it in browser | ||
// (indexeddb can store any JS type) | ||
if (value != null && !this._isBuffer(value) && !process.browser) | ||
value = String(value) | ||
if (typeof options != 'object') | ||
@@ -139,7 +133,6 @@ options = {} | ||
if (err = this._checkKey(key, 'key', this._isBuffer)) | ||
if (err = this._checkKey(key, 'key')) | ||
return callback(err) | ||
if (!this._isBuffer(key)) | ||
key = String(key) | ||
key = this._serializeKey(key) | ||
@@ -184,6 +177,6 @@ if (typeof options != 'object') | ||
if (err = this._checkKey(e.type, 'type', this._isBuffer)) | ||
if (err = this._checkKey(e.type, 'type')) | ||
return callback(err) | ||
if (err = this._checkKey(e.key, 'key', this._isBuffer)) | ||
if (err = this._checkKey(e.key, 'key')) | ||
return callback(err) | ||
@@ -210,8 +203,5 @@ } | ||
if (!this._isBuffer(start)) | ||
start = String(start) | ||
start = this._serializeKey(start) | ||
end = this._serializeKey(end) | ||
if (!this._isBuffer(end)) | ||
end = String(end) | ||
if (typeof this._approximateSize == 'function') | ||
@@ -265,11 +255,21 @@ return this._approximateSize(start, end, callback) | ||
AbstractLevelDOWN.prototype._serializeKey = function (key) { | ||
return this._isBuffer(key) | ||
? key | ||
: String(key) | ||
} | ||
AbstractLevelDOWN.prototype._serializeValue = function (value) { | ||
return this._isBuffer(value) || process.browser | ||
? value | ||
: String(value) | ||
} | ||
AbstractLevelDOWN.prototype._checkKey = function (obj, type) { | ||
if (obj === null || obj === undefined) | ||
return new Error(type + ' cannot be `null` or `undefined`') | ||
if (this._isBuffer(obj)) { | ||
if (obj.length === 0) | ||
return new Error(type + ' cannot be an empty Buffer') | ||
} else if (String(obj) === '') | ||
if (this._isBuffer(obj) && obj.length === 0) | ||
return new Error(type + ' cannot be an empty Buffer') | ||
else if (String(obj) === '') | ||
return new Error(type + ' cannot be an empty String') | ||
@@ -276,0 +276,0 @@ } |
var db | ||
, leveldown | ||
, testCommon | ||
module.exports.setUp = function (leveldown, test, testCommon) { | ||
test('setUp common', testCommon.setUp) | ||
module.exports.setUp = function (_leveldown, test, _testCommon) { | ||
test('setUp common', _testCommon.setUp) | ||
test('setUp db', function (t) { | ||
leveldown = _leveldown | ||
testCommon = _testCommon | ||
db = leveldown(testCommon.location()) | ||
@@ -65,2 +69,44 @@ db.open(t.end.bind(t)) | ||
}) | ||
test('test _serialize object', function (t) { | ||
t.plan(3) | ||
var db = leveldown(testCommon.location()) | ||
db._approximateSize = function (start, end, callback) { | ||
t.equal(start, '[object Object]') | ||
t.equal(end, '[object Object]') | ||
callback() | ||
} | ||
db.approximateSize({}, {}, function (err, val) { | ||
t.error(err) | ||
}) | ||
}) | ||
test('test _serialize buffer', function (t) { | ||
t.plan(3) | ||
var db = leveldown(testCommon.location()) | ||
db._approximateSize = function (start, end, callback) { | ||
t.same(start, Buffer('start')) | ||
t.same(end, Buffer('end')) | ||
callback() | ||
} | ||
db.approximateSize(Buffer('start'), Buffer('end'), function (err, val) { | ||
t.error(err) | ||
}) | ||
}) | ||
test('test custom _serialize*', function (t) { | ||
t.plan(3) | ||
var db = leveldown(testCommon.location()) | ||
db._serializeKey = function (data) { return data } | ||
db._approximateSize = function (start, end, callback) { | ||
t.deepEqual(start, { foo: 'bar' }) | ||
t.deepEqual(end, { beep: 'boop' }) | ||
callback() | ||
} | ||
db.open(function () { | ||
db.approximateSize({ foo: 'bar' }, { beep: 'boop' }, function (err) { | ||
t.error(err) | ||
}) | ||
}) | ||
}) | ||
} | ||
@@ -67,0 +113,0 @@ |
var db | ||
, leveldown | ||
, testCommon | ||
module.exports.setUp = function (leveldown, test, testCommon) { | ||
test('setUp common', testCommon.setUp) | ||
module.exports.setUp = function (_leveldown, test, _testCommon) { | ||
test('setUp common', _testCommon.setUp) | ||
test('setUp db', function (t) { | ||
leveldown = _leveldown | ||
testCommon = _testCommon | ||
db = leveldown(testCommon.location()) | ||
@@ -155,2 +159,38 @@ db.open(t.end.bind(t)) | ||
}) | ||
test('test serialize object', function (t) { | ||
var batch = db.batch() | ||
.put({ foo: 'bar' }, { beep: 'boop' }) | ||
.del({ bar: 'baz' }) | ||
t.deepEqual(batch._operations, [ | ||
{ type: 'put', key: '[object Object]', value: '[object Object]' } | ||
, { type: 'del', key: '[object Object]' } | ||
]) | ||
t.end() | ||
}) | ||
test('test serialize buffer', function (t) { | ||
var batch = db.batch() | ||
.put(Buffer('foo'), Buffer('bar')) | ||
.del(Buffer('baz')) | ||
t.equal(batch._operations[0].key.toString(), 'foo') | ||
t.equal(batch._operations[0].value.toString(), 'bar') | ||
t.equal(batch._operations[1].key.toString(), 'baz') | ||
t.end() | ||
}) | ||
test('test custom _serialize*', function (t) { | ||
var db = leveldown(testCommon.location()) | ||
db._serializeKey = db._serializeValue = function (data) { return data } | ||
db.open(function () { | ||
var batch = db.batch() | ||
.put({ foo: 'bar' }, { beep: 'boop' }) | ||
.del({ bar: 'baz' }) | ||
t.deepEqual(batch._operations, [ | ||
{ type: 'put', key: { foo: 'bar' }, value: { beep: 'boop' } } | ||
, { type: 'del', key: { bar: 'baz' } } | ||
]) | ||
db.close(t.end.bind(t)) | ||
}) | ||
}) | ||
} | ||
@@ -211,2 +251,2 @@ | ||
module.exports.tearDown(test, testCommon) | ||
} | ||
} |
var db | ||
, leveldown | ||
, testCommon | ||
, verifyNotFoundError = require('./util').verifyNotFoundError | ||
, isTypedArray = require('./util').isTypedArray | ||
module.exports.setUp = function (leveldown, test, testCommon) { | ||
test('setUp common', testCommon.setUp) | ||
module.exports.setUp = function (_leveldown, test, _testCommon) { | ||
test('setUp common', _testCommon.setUp) | ||
test('setUp db', function (t) { | ||
leveldown = _leveldown | ||
testCommon = _testCommon | ||
db = leveldown(testCommon.location()) | ||
@@ -40,2 +44,41 @@ db.open(t.end.bind(t)) | ||
}) | ||
test('test _serialize object', function (t) { | ||
t.plan(2) | ||
var db = leveldown(testCommon.location()) | ||
db._del = function (key, opts, callback) { | ||
t.equal(key, '[object Object]') | ||
callback() | ||
} | ||
db.del({}, function (err, val) { | ||
t.error(err) | ||
}) | ||
}) | ||
test('test _serialize buffer', function (t) { | ||
t.plan(2) | ||
var db = leveldown(testCommon.location()) | ||
db._del = function (key, opts, callback) { | ||
t.ok(Buffer.isBuffer(key)) | ||
callback() | ||
} | ||
db.del(Buffer('buf'), function (err, val) { | ||
t.error(err) | ||
}) | ||
}) | ||
test('test custom _serialize*', function (t) { | ||
t.plan(2) | ||
var db = leveldown(testCommon.location()) | ||
db._serializeKey = function (data) { return data } | ||
db._del = function (key, options, callback) { | ||
t.deepEqual(key, { foo: 'bar' }) | ||
callback() | ||
} | ||
db.open(function () { | ||
db.del({ foo: 'bar' }, function (err) { | ||
t.error(err) | ||
}) | ||
}) | ||
}) | ||
} | ||
@@ -42,0 +85,0 @@ |
var db | ||
, leveldown | ||
, testCommon | ||
, verifyNotFoundError = require('./util').verifyNotFoundError | ||
, isTypedArray = require('./util').isTypedArray | ||
module.exports.setUp = function (leveldown, test, testCommon) { | ||
test('setUp common', testCommon.setUp) | ||
module.exports.setUp = function (_leveldown, test, _testCommon) { | ||
test('setUp common', _testCommon.setUp) | ||
test('setUp db', function (t) { | ||
leveldown = _leveldown | ||
testCommon = _testCommon | ||
db = leveldown(testCommon.location()) | ||
@@ -40,2 +44,41 @@ db.open(t.end.bind(t)) | ||
}) | ||
test('test _serialize object', function (t) { | ||
t.plan(2) | ||
var db = leveldown(testCommon.location()) | ||
db._get = function (key, opts, callback) { | ||
t.equal(key, '[object Object]') | ||
callback() | ||
} | ||
db.get({}, function (err, val) { | ||
t.error(err) | ||
}) | ||
}) | ||
test('test _serialize buffer', function (t) { | ||
t.plan(2) | ||
var db = leveldown(testCommon.location()) | ||
db._get = function (key, opts, callback) { | ||
t.same(key, Buffer('key')) | ||
callback() | ||
} | ||
db.get(Buffer('key'), function (err, val) { | ||
t.error(err) | ||
}) | ||
}) | ||
test('test custom _serialize*', function (t) { | ||
t.plan(2) | ||
var db = leveldown(testCommon.location()) | ||
db._serializeKey = function (data) { return data } | ||
db._get = function (key, options, callback) { | ||
t.deepEqual(key, { foo: 'bar' }) | ||
callback() | ||
} | ||
db.open(function () { | ||
db.get({ foo: 'bar' }, function (err) { | ||
t.error(err) | ||
}) | ||
}) | ||
}) | ||
} | ||
@@ -134,2 +177,2 @@ | ||
module.exports.tearDown(test, testCommon) | ||
} | ||
} |
var db | ||
, leveldown | ||
, testCommon | ||
, verifyNotFoundError = require('./util').verifyNotFoundError | ||
, isTypedArray = require('./util').isTypedArray | ||
module.exports.setUp = function (leveldown, test, testCommon) { | ||
test('setUp common', testCommon.setUp) | ||
module.exports.setUp = function (_leveldown, test, _testCommon) { | ||
test('setUp common', _testCommon.setUp) | ||
test('setUp db', function (t) { | ||
leveldown = _leveldown | ||
testCommon = _testCommon | ||
db = leveldown(testCommon.location()) | ||
@@ -49,2 +53,44 @@ db.open(t.end.bind(t)) | ||
}) | ||
test('test _serialize object', function (t) { | ||
t.plan(3) | ||
var db = leveldown(testCommon.location()) | ||
db._put = function (key, value, opts, callback) { | ||
t.equal(key, '[object Object]') | ||
t.equal(value, '[object Object]') | ||
callback() | ||
} | ||
db.put({}, {}, function (err, val) { | ||
t.error(err) | ||
}) | ||
}) | ||
test('test _serialize buffer', function (t) { | ||
t.plan(3) | ||
var db = leveldown(testCommon.location()) | ||
db._put = function (key, value, opts, callback) { | ||
t.same(key, Buffer('key')) | ||
t.same(value, Buffer('value')) | ||
callback() | ||
} | ||
db.put(Buffer('key'), Buffer('value'), function (err, val) { | ||
t.error(err) | ||
}) | ||
}) | ||
test('test custom _serialize*', function (t) { | ||
t.plan(3) | ||
var db = leveldown(testCommon.location()) | ||
db._serializeKey = db._serializeValue = function (data) { return data } | ||
db._put = function (key, value, options, callback) { | ||
t.deepEqual(key, { foo: 'bar' }) | ||
t.deepEqual(value, { beep: 'boop' }) | ||
callback() | ||
} | ||
db.open(function () { | ||
db.put({ foo: 'bar' }, { beep: 'boop'}, function (err) { | ||
t.error(err) | ||
}) | ||
}) | ||
}) | ||
} | ||
@@ -51,0 +97,0 @@ |
{ | ||
"name": "abstract-leveldown", | ||
"description": "An abstract prototype matching the LevelDOWN API", | ||
"version": "2.4.1", | ||
"version": "2.5.0", | ||
"contributors": [ | ||
@@ -36,5 +36,5 @@ "Rod Vagg <r@va.gg> (https://github.com/rvagg)", | ||
"devDependencies": { | ||
"rimraf": "~2.3.2", | ||
"sinon": "~1.14.1", | ||
"tape": "~4.0.0" | ||
"rimraf": "~2.5.0", | ||
"sinon": "~1.17.2", | ||
"tape": "~4.4.0" | ||
}, | ||
@@ -41,0 +41,0 @@ "browser": { |
@@ -1,5 +0,8 @@ | ||
# Abstract LevelDOWN [](http://travis-ci.org/Level/abstract-leveldown) | ||
# Abstract LevelDOWN | ||
<img alt="LevelDB Logo" height="100" src="http://leveldb.org/img/logo.svg"> | ||
[](http://travis-ci.org/Level/abstract-leveldown) | ||
[](https://david-dm.org/level/abstract-leveldown) | ||
[](https://nodei.co/npm/abstract-leveldown/) | ||
@@ -83,3 +86,3 @@ [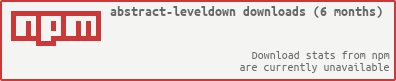](https://nodei.co/npm/abstract-leveldown/) | ||
See [MemDOWN](https://github.com/rvagg/memdown/) if you are looking for a complete in-memory replacement for LevelDOWN. | ||
See [MemDOWN](https://github.com/Level/memdown/) if you are looking for a complete in-memory replacement for LevelDOWN. | ||
@@ -98,9 +101,11 @@ ## Extensible API | ||
If `batch()` is called without argument or with only an options object then it should return a `Batch` object with chainable methods. Otherwise it will invoke a classic batch operation. | ||
If `batch()` is called without arguments or with only an options object then it should return a `Batch` object with chainable methods. Otherwise it will invoke a classic batch operation. | ||
### AbstractLevelDOWN#_chainedBatch() | ||
By default an `batch()` operation without argument returns a blank `AbstractChainedBatch` object. The prototype is available on the main exports for you to extend. If you want to implement chainable batch operations then you should extend the `AbstractChaindBatch` and return your object in the `_chainedBatch()` method. | ||
By default a `batch()` operation without arguments returns a blank `AbstractChainedBatch` object. The prototype is available on the main exports for you to extend. If you want to implement chainable batch operations then you should extend the `AbstractChaindBatch` and return your object in the `_chainedBatch()` method. | ||
### AbstractLevelDOWN#_approximateSize(start, end, callback) | ||
### AbstractLevelDOWN#_serializeKey(key) | ||
### AbstractLevelDOWN#_serializeValue(value) | ||
### AbstractLevelDOWN#_iterator(options) | ||
@@ -110,3 +115,3 @@ | ||
`AbstractIterator` implements the basic state management found in LevelDOWN. It keeps track of when a `next()` is in progress and when an `end()` has been called so it doesn't allow concurrent `next()` calls, it does it allow `end()` while a `next()` is in progress and it doesn't allow either `next()` or `end()` after `end()` has been called. | ||
`AbstractIterator` implements the basic state management found in LevelDOWN. It keeps track of when a `next()` is in progress and when an `end()` has been called so it doesn't allow concurrent `next()` calls, it does allow `end()` while a `next()` is in progress and it doesn't allow either `next()` or `end()` after `end()` has been called. | ||
@@ -127,2 +132,4 @@ ### AbstractIterator(db) | ||
### AbstractChainedBatch#_write(options, callback) | ||
### AbstractChainedBatch#_serializeKey(key) | ||
### AbstractChainedBatch#_serializeValue(value) | ||
@@ -129,0 +136,0 @@ ### isLevelDown(db) |
@@ -10,4 +10,4 @@ var test = require('tape') | ||
function factory (location) { | ||
return new AbstractLevelDOWN(location) | ||
function factory (location, opts) { | ||
return new AbstractLevelDOWN(location, opts) | ||
} | ||
@@ -716,1 +716,2 @@ | ||
}) | ||
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
125695
3034
153