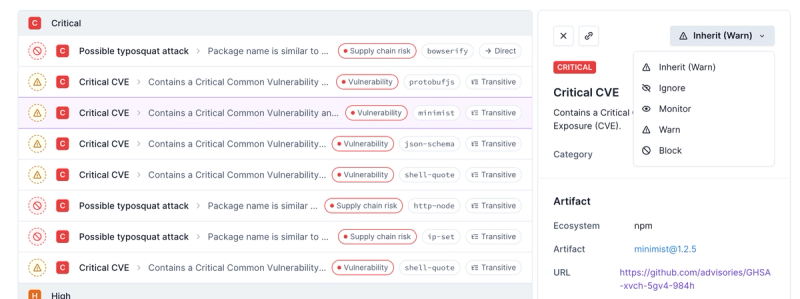
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
airwallex-payment-elements
Advanced tools
[](https://github.com/semantic-release/semantic-release)
Readme
This universal library is a lightweight javascript SDK, which allow merchant site to convenient integrate with airwallex checkout flow
From the merchant perspective, The Hosted Payment Page(HPP) / Drop-in / Elements integration happen after shopper decide to checkout an order on merchant's website, Airwallex javascript SDK help merchant accept payments on merchant website, the whole user experience include create an PaymentIntent entity to tacking the payment request, which link to merchant’s order entity, Airwallex using a paymentAttempt entity to collect payment methods details from a shopper, and link back to the PaymentIntent entity, which tracking the state change all the way through user interactions, you can checkout the PaymentIntent section for more details
With the API integration from merchant server, after PaymentIntent is successfully created, the response of the API call will return a unique ID for the PaymentIntent, and a client_secret which act as a single source of truth cross over the shopping experience, each time user's interaction trigger a service call Airwallex client API will validate against the client_secret to secure the whole payment flow
So long story short, the merchant website checkout start with PaymentIntent and client_secret
Below are the step by step guide to use this library
yarn add airwallex-payment-elements
OR with NPM:
npm install airwallex-payment-elements
import { loadAirwallex } from 'airwallex-payment-elements';
await loadAirwallex({
env: 'demo', // 'staging' | 'demo' | 'prod'
});
options
pass to loadAirwallex
is useful to customize looks and feel of the checkout form when you are adopt Drop-in / Elements integration
The HPP provide the most convenient way to integrate
import { redirectToCheckout } from 'airwallex-payment-elements';
redirectToCheckout({
env: 'demo', // 'staging' | 'demo' | 'prod'
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}
The primary integration path through this library is with Elements, which enables merchant to collect sensitive payment information(PCI) using customizable UI elements. this library also provides a single interface for Payment Request API
With this library, you can also tokenize sensitive information, and handle 3D Secure authentication
Airwallex element is a set of prebuilt UI components, like inputs and buttons, for building your checkout flow. It’s available as a feature of this library. this library handle checkout confirm within an Element without ever having it touch your server
Elements includes features like:
Formatting card information automatically as it’s entered
Customize placeholders to fit your UX design
Using responsive design to fit the width of your customer’s screen or mobile device
Customizing the styling to match the look and feel of your checkout flow
When you select dropIn element type you are actually adopt Drop-in integration
With Drop-in integration, those prebuilt UI components has been composed into a payment form with all available payment methods, you can put it directly into you html page, and integrate with the rest of your own components to render your checkout page.
With cardNumber | expiry | cvc | paymentRequestButton | wechat elements, they are just building blocks:
You can arrange with any order you want
Show and hide anyone of them at any moment you want
Customize the look and feels as you want
Listen on the events and interact with shoppers when they click or typing in those elements
<div id='dropIn'></div>
import { createElement } from 'airwallex-payment-elements';
const dropInElement = createElement('dropIn', {
intent: { // Required, dropIn use intent Id and client_secret to prepare checkout
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}
});
dropInElement.mount('dropIn');
window.addEventListener('onSuccess', (event: ElementEvent) => {
// Handle checkout success event
});
<div id='card'></div>
import { createElement } from 'airwallex-payment-elements';
const cardElement = createElement('card', {
intent: { // Required, Card use intent Id and client_secret to prepare checkout
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}
});
cardElement.mount('card');
try {
const res = await confirmPaymentIntent({
element: cardElement,
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret',
});
const { confirmResult } = res || {};
const { id, status } = confirmResult || {};
// Handle success
} catch (err) {
const { reason } = err;
const { error: { code, message } } = reason || {};
// Handle fail
}
What's different from card is that full featured card contains payment button so that you don't need to confirm payment intent yourself.
<div id='fullFeaturedCard'></div>
import { createElement } from 'airwallex-payment-elements';
const cardElement = createElement('fullFeaturedCard', {
intent: { // Required, Card use intent Id and client_secret to prepare checkout
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}
});
cardElement.mount('full-featured-card');
window.addEventListener('onSuccess', (event: ElementEvent) => {
// Handle checkout success event
});
<label>
Card number
<div id='cardNumber'></div>
</label>
<label>
Expiry
<div id='expiry'></div>
</label>
<label>
CVC
<div id='cvc'></div>
</label>
import { createElement, confirmPaymentIntent } from 'airwallex-payment-elements';
// Create and mount 'cardNumber' element
const cardNumber = createElement('cardNumber');
cardNumber.mount('cardNumber');
// Create and mount 'expiry' element
const expiry = Airwallex.createElement('expiry');
expiry.mount('expiry');
// Create and mount 'cvc' element
const cvc = Airwallex.createElement('cvc');
cvc.mount('cvc');
try {
const res = await confirmPaymentIntent({
element: cardNumber,
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret',
});
const { confirmResult } = res || {};
const { id, status } = confirmResult || {};
// Handle success
} catch (err) {
const { reason } = err;
const { error: { code, message } } = reason || {};
// Handle fail
}
<div id='wechat'></div>
import { createElement } from 'airwallex-payment-elements';
// Create and mount 'wechat' element
const wechat = createElement('wechat', {
intent: { // Required, dropIn use intent Id and client_secret to prepare checkout
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}
});
wechat.mount('wechat');
window.addEventListener('onSuccess', (event: ElementEvent) => {
// Handle checkout success event
});
Add customer_id
when create payment intent and we will get the saved payment methods for the customer and redner saved cards list in the element. Nothing need to change during the integration.
You could to get saved cards list when create intent or query intent. Then render them by your self to let shopper choose one of them.
Then create a cvc element to enter cvc code and call confirmPaymentIntentWithSavedCard
function to finish checkout.
<label>
CVC
<div id='cvc'></div>
</label>
import { createElement, confirmPaymentIntentWithSavedCard } from 'airwallex-payment-elements';
// Create and mount 'cvc' element
const cvc = Airwallex.createElement('cvc');
cvc.mount('cvc');
try {
const res = await confirmPaymentIntentWithSavedCard({
id: 'replace-with-your-intent-id',
methodId: 'replace-with-chosen-payment-method-id',
customerId: 'replace-with-your-customer-id',
client_secret: 'replace-with-your-client-secret',
element: cvcElement,
});
const { confirmResult } = res || {};
const { id, status } = confirmResult || {};
// Handle success
} catch (err) {
const { reason } = err;
const { error: { code, message } } = reason || {};
// Handle fail
}
For more detail please check on the interface in the package
Download and run those example from github airwallex-payment-demo
!!!Those examples only show integration with required parameters and steps, more details can be found in product doc Payment-Web integration section or in the types
folder of the package inside node_modules
after npm install airwallex-payment-elements
command
<!doctype html>
<html>
<head lang='en'>
<meta charset='utf-8'>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<title>Airwallex Checkout Playground</title>
<!-- Step #1: Load Checkout Universal Module Definition (UMD) bundle js-->
<script src="https://checkout.airwallex.com/assets/bundle.0.0.xx.min.js"></script>
</head>
<body>
<h1>Hosted payment page (HPP) integration</h1>
<button id='hpp'>Redirect to HPP</button>
<script>
document.getElementById('hpp').addEventListener('click', () => {
// Step #2: Call the HPP function to checkout
Airwallex.redirectToCheckout({
env: 'demo', // Which env('staging' | 'demo' | 'prod') you would like to integrate with
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
});
})
</script>
</body>
</html>
<!doctype html>
<html>
<head lang='en'>
<meta charset='utf-8'>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<title>Airwallex Checkout Playground</title>
<!-- Step #1: Load Checkout Universal Module Definition (UMD) bundle js-->
<script src="https://checkout.airwallex.com/assets/bundle.0.0.xx.min.js"></script>
</head>
<body>
<h1>DropIn integration</h1>
<div id='dropIn'></div>
<script>
// Step #2: Initialize the Airwallex global context for event communication
Airwallex.init({
env: 'demo', // Setup which env('staging' | 'demo' | 'prod') you would like to integrate with
origin: window.location.origin, // Setup your event target to receive the browser events message
});
// Step #3: Create 'dropIn' element
const dropIn = Airwallex.createElement('dropIn', {
intent: { // Required, dropIn use intent Id and client_secret to prepare checkout
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}
});
// Step #4: Mount 'dropIn' element
dropIn.mount('dropIn');
window.addEventListener('onSuccess', (event) => {
// Handle event
alert(JSON.stringify(event.detail));
})
</script>
</body>
</html>
<!doctype html>
<html>
<head lang='en'>
<meta charset='utf-8'>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<title>Airwallex Checkout Playground</title>
<!-- Step #1: Load Checkout Universal Module Definition (UMD) bundle js-->
<script src="https://checkout.airwallex.com/assets/bundle.xx.xx.xx.min.js"></script>
</head>
<body>
<h1>Card integration</h1>
<label>
Card Information
<div id='card'></div>
</label>
<br>
<button id='submit'>Submit</button>
<script>
// Step #2: Initialize the Airwallex global context for event communication
Airwallex.init({
env: 'staging', // Setup which env('staging' | 'demo' | 'prod') you would like to integrate with
origin: window.location.origin, // Setup your event target to receive the browser events message
});
// Step #3: Create 'card' element
const card = Airwallex.createElement('card');
// Step #4: Mount card element
card.mount('card');
document.getElementById('submit').addEventListener('click', () => {
// Step #5: Confirm payment intent with id and client_secret
Airwallex.confirmPaymentIntent({
element: card,
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}).then((response) => {
/* handle confirm response in your business flow */
alert(JSON.stringify(response));
});
});
window.addEventListener('onReady', (event) => {
/*
... Handle event
*/
alert(event.detail)
})
</script>
</body>
</html>
What's different from card is that full featured card contains payment button so that you don't need to confirm payment intent yourself.
<!doctype html>
<html>
<head lang='en'>
<meta charset='utf-8'>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<title>Airwallex Checkout Playground</title>
<!-- Step #1: Load Checkout Universal Module Definition (UMD) bundle js-->
<script src="https://checkout.airwallex.com/assets/bundle.0.0.xx.min.js"></script>
</head>
<body>
<h1>Card integration</h1>
<div id='full-featured-card'></div>
<script>
// Step #2: Initialize the Airwallex global context for event communication
Airwallex.init({
env: 'demo', // Setup which env('staging' | 'demo' | 'prod') you would like to integrate with
origin: window.location.origin, // Setup your event target to receive the browser events message
});
// Step #3: Create 'card' element
const card = Airwallex.createElement('fullFeaturedCard', {
intent: { // Required, use intent Id and client_secret to prepare checkout
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}
});
// Step #4: Mount 'card' element
card.mount('full-featured-card');
window.addEventListener('onSuccess', (event) => {
// Handle event
alert(JSON.stringify(event.detail));
})
</script>
</body>
</html>
<!doctype html>
<html>
<head lang='en'>
<meta charset='utf-8'>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<title>Airwallex Checkout Playground</title>
<!-- Step #1: Load Checkout Universal Module Definition (UMD) bundle js-->
<script src="https://checkout.airwallex.com/assets/bundle.0.0.xx.min.js"></script>
</head>
<body>
<h1>Card elements integration</h1>
<label>
Card number
<div id='cardNumber'></div>
</label>
<label>
Expiry
<div id='expiry'></div>
</label>
<label>
CVC
<div id='cvc'></div>
</label>
<br>
<button id='submit'>Submit</button>
<script>
// Step #2: Initialize the Airwallex global context for event communication
Airwallex.init({
env: 'demo', // Setup which env('staging' | 'demo' | 'prod') you would like to integrate with
origin: window.location.origin, // Setup your event target to receive the browser events message
});
// Step #3.1: Create 'cardNumber' element
const cardNumber = Airwallex.createElement('cardNumber');
// Step #3.2: Create 'expiry' element
const expiry = Airwallex.createElement('expiry');
// Step #3.3: Create 'cvc' element
const cvc = Airwallex.createElement('cvc');
// Step #4: Mount card elements
cardNumber.mount('cardNumber');
expiry.mount('expiry');
cvc.mount('cvc');
document.getElementById('submit').addEventListener('click', () => {
// Step #5: Confirm payment intent with id and client_secret
Airwallex.confirmPaymentIntent({
element: cardNumber,
id: 'replace-with-your-intent-id',
client_secret: 'replace-with-your-client-secret'
}).then((response) => {
// handle confirm response in your business flow
alert(JSON.stringify(response));
});
});
</script>
</body>
</html>
<!doctype html>
<html>
<head lang='en'>
<meta charset='utf-8'>
<meta name='viewport' content='width=device-width, initial-scale=1'>
<title>Airwallex Checkout Playground</title>
<!-- Step #1: Load Checkout Universal Module Definition (UMD) bundle js-->
<script src="https://checkout.airwallex.com/assets/bundle.0.0.xx.min.js"></script>
</head>
<body>
<script>
// Define the intent id and client_secret to checkout across different integration methods
const id = 'replace-with-your-intent-id';
const client_secret = 'replace-with-your-client-secret';
</script>
<h1>Airwallex checkout integration</h1>
<hr>
<!-- HPP -->
<h2>Option #1: Hosted payment page (HPP) integration</h2>
<button id='hpp'>Redirect to HPP</button>
<script>
document.getElementById('hpp').addEventListener('click', () => {
Airwallex.redirectToCheckout({
env: 'demo',
id,
client_secret
});
})
</script>
<hr>
<!-- DropIn integration-->
<h2>Option #2: DropIn integration</h2>
<div id='dropIn'></div>
<script>
Airwallex.init({
env: 'demo',
origin: window.location.origin,
});
const dropIn = Airwallex.createElement('dropIn', {
intent: {
id,
client_secret
}
});
dropIn.mount('dropIn');
</script>
<hr>
<!-- Card integration-->
<h2>Option #3: Card integration</h2>
<div id='card'></div>
<script>
Airwallex.init({
origin: window.location.origin,
env: 'demo'
});
const card = Airwallex.createElement('card', {
intent: {
id,
client_secret
}
});
card.mount('card');
</script>
<script>
window.addEventListener('onSuccess', (event) => {
/*
... Handle event
*/
alert(JSON.stringify(event.detail));
})
</script>
<hr>
<!-- Card elements integration-->
<h2>Option #4: Card elements integration</h2>
<label>
Card number
<div id='cardNumber'></div>
</label>
<label>
Expiry
<div id='expiry'></div>
</label>
<label>
CVC
<div id='cvc'></div>
</label>
<br>
<button id='submit'>Submit</button>
<script>
Airwallex.init({
env: 'demo',
origin: window.location.origin,
});
const cardNumber = Airwallex.createElement('cardNumber');
const expiry = Airwallex.createElement('expiry');
const cvc = Airwallex.createElement('cvc');
cardNumber.mount('cardNumber');
expiry.mount('expiry');
cvc.mount('cvc');
document.getElementById('submit').addEventListener('click', () => {
Airwallex.confirmPaymentIntent({
element: cardNumber,
id,
client_secret
}).then((response) => {
// handle confirm response
alert(JSON.stringify(response));
});
});
</script>
<hr>
</body>
</html>
FAQs
[](https://www.npmjs.org/package/airwallex-payment-elements) [](https://git
The npm package airwallex-payment-elements receives a total of 5,552 weekly downloads. As such, airwallex-payment-elements popularity was classified as popular.
We found that airwallex-payment-elements demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.