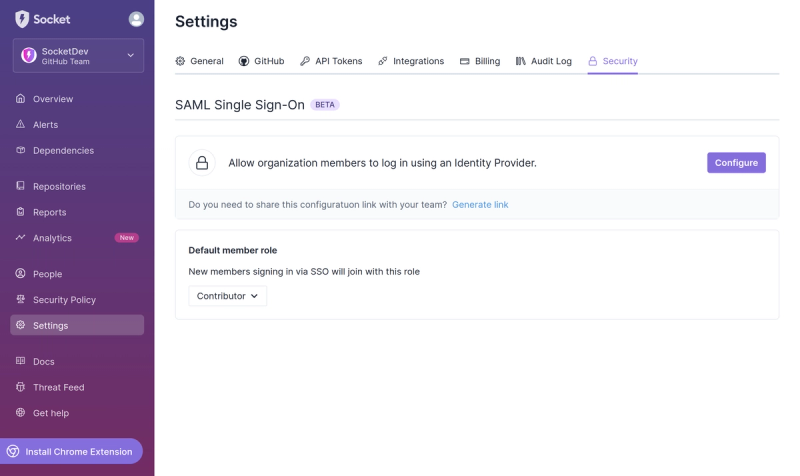
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
ajv
Advanced tools
Package description
The ajv npm package is a fast JSON Schema validator that allows you to validate JSON data against a JSON schema. It supports the latest JSON Schema draft-07 and has several extensions. It can be used for data validation, data sanitization, and to ensure that JSON documents comply with a predefined schema.
Validate data against a JSON Schema
This feature allows you to compile a JSON Schema and use it to validate JSON data. If the data does not conform to the schema, the errors can be logged or handled as needed.
{"const Ajv = require('ajv');
const ajv = new Ajv();
const schema = {
"type": "object",
"properties": {
"foo": {"type": "integer"},
"bar": {"type": "string"}
},
"required": ["foo"]
};
const validate = ajv.compile(schema);
const valid = validate({foo: 1, bar: 'abc'});
if (!valid) console.log(validate.errors);"}
Add custom keywords
Ajv allows you to define custom keywords for a JSON Schema, which can be used to create custom validation rules that are not defined in the JSON Schema specification.
{"const Ajv = require('ajv');
const ajv = new Ajv();
ajv.addKeyword('even', {
validate: function(schema, data) {
return data % 2 === 0;
}
});
const schema = {"even": true};
const validate = ajv.compile(schema);
const valid = validate(2); // true
const invalid = validate(3); // false"}
Asynchronous validation
Ajv supports asynchronous schema compilation, which is useful when your JSON Schema depends on other schemas that need to be fetched remotely.
{"const Ajv = require('ajv');
const ajv = new Ajv({loadSchema: loadExternalSchema});
// Assume loadExternalSchema is a function that loads a schema asynchronously
ajv.compileAsync(schema).then(function(validate) {
const valid = validate(data);
if (!valid) console.log(validate.errors);
}).catch(function(err) {
console.error('Failed to compile schema:', err);
});"}
Joi is a powerful schema description language and data validator for JavaScript. Unlike ajv, which focuses on JSON Schema, Joi allows you to create validation schemas using a fluent API. It is often used for validating data in REST APIs.
Tiny Validator (tv4) is a small and fast JSON Schema (v4) validator. It is less feature-rich compared to ajv and does not support the latest JSON Schema specifications, but it is suitable for simple validation tasks.
The jsonschema package is another validator for JSON Schema that supports draft-04/06/07. It is not as fast as ajv but provides a straightforward API for validating JSON data against schemas.
Readme
One of the fastest JSON Schema validators for node.js and browser.
It uses precompiled doT templates to generate super-fast validating functions.
ajv implements full JSON Schema draft 4 standard:
addSchema
or compiled to be available)ajv passes all the tests from JSON Schema Test Suite (apart from the one that requires that 1.0
is not an integer).
Benchmark of the test suite - json-schema-benchmark.
Same benchmark run on faster CPU with node 0.12.
Benchmark of schemas of different complexity by jsck.
Benchmark of individual test cases by z-schema.
npm install ajv
The fastest validation call:
var Ajv = require('ajv');
var ajv = Ajv(); // options can be passed
var validate = ajv.compile(schema);
var valid = validate(data);
if (!valid) console.log(validate.errors);
or with less code
// ...
var valid = ajv.validate(schema, data);
if (!valid) console.log(ajv.errors);
// ...
or
// ...
ajv.addSchema(schema, 'mySchema');
var valid = ajv.validate('mySchema', data);
if (!valid) console.log(ajv.errorsText());
// ...
ajv compiles schemas to functions and caches them in all cases (using stringified schema as a key - using json-stable-stringify), so that the next time the same schema is used (not necessarily the same object instance) it won't be compiled again.
The best performance is achieved when using compiled functions returned by compile
or getSchema
methods (there is no additional function call).
You can require ajv directly from the code you browserify - in this case ajv will be a part of your bundle.
If you need to use ajv in several bundles you can create a separate browserified bundle using bin/create-bundle
script (thanks to siddo420).
Then you need to load ajv in the browser:
<script src="ajv.bundle.js"></script>
Now you can use it as shown above - require
will be global and you can require('ajv')
.
Ajv was tested with these browsers:
The following formats are supported for string validation with "format" keyword:
date
and date-time
validate ranges in full
mode and only regexp in fast
mode (see options).There are two modes of format validation: fast
and full
that affect all formats but ipv4
and ipv6
. See Options for details.
You can add additional formats and replace any of the formats above using addFormat method.
You can find patterns used for format validation and the sources that were used in formats.js.
With option removeAdditional
(added by andyscott) you can filter data during the validation.
This option modifies original object.
Create ajv instance.
All the instance methods below are bound to the instance, so they can be used without the instance.
Generate validating function and cache the compiled schema for future use.
Validating function returns boolean and has properties errors
with the errors from the last validation (null
if there were no errors) and schema
with the reference to the original schema.
Unless the option validateSchema
is false, the schema will be validated against meta-schema and if schema is invalid the error will be thrown. See options.
Validate data using passed schema (it will be compiled and cached).
Instead of the schema you can use the key that was previously passed to addSchema
, the schema id if it was present in the schema or any previously resolved reference.
Validation errors will be available in the errors
property of ajv instance (null
if there were no errors).
Add schema(s) to validator instance. From version 1.0.0 this method does not compile schemas (but it still validates them). Because of that change, dependencies can be added in any order and circular dependencies are supported. It also prevents unnecessary compilation of schemas that are containers for other schemas but not used as a whole.
Array of schemas can be passed (schemas should have ids), the second parameter will be ignored.
Key can be passed that can be used to reference the schema and will be used as the schema id if there is no id inside the schema. If the key is not passed, the schema id will be used as the key.
Once the schema is added, it (and all the references inside it) can be referenced in other schemas and used to validate data.
Although addSchema
does not compile schemas, explicit compilation is not required - the schema will be compiled when it is used first time.
By default the schema is validated against meta-schema before it is added, and if the schema does not pass validation the exception is thrown. This behaviour is controlled by validateSchema
option.
Adds meta schema that can be used to validate other schemas. That function should be used instead of addSchema
because there may be instance options that would compile a meta schema incorrectly (at the moment it is removeAdditional
option).
There is no need to explicitely add draft 4 meta schema (http://json-schema.org/draft-04/schema) - it is added by default, unless option meta
is set to false
. You only need to use it if you have a changed meta-schema that you want to use to validate your schemas. See validateSchema
.
Validates schema. This method should be used to validate schemas rather than validate
due to the inconsistency of uri
format in JSON-Schema standart.
By default this method is called automatically when the schema is added, so you rarely need to use it directly.
If schema doesn't have $schema
property it is validated against draft 4 meta-schema (option meta
should not be false).
If schema has $schema
property then the schema with this id (should be previously added) is used to validate passed schema.
Errors will be available at ajv.errors
.
Retrieve compiled schema previously added with addSchema
by the key passed to addSchema
or by its full reference (id). Returned validating function has schema
property with the reference to the original schema.
Remove added/cached schema. Even if schema is referenced by other schemas it can be safely removed as dependent schemas have local references.
Schema can be removed using key passed to addSchema
, it's full reference (id) or using actual schema object that will be stable-stringified to remove schema from cache.
Add custom format to validate strings. It can also be used to replace pre-defined formats for ajv instance.
Strings are converted to RegExp.
Function should return validation result as true
or false
.
Custom formats can be also added via formats
option.
Returns the text with all errors in a String.
Options can have properties separator
(string used to separate errors, ", " by default) and dataVar
(the variable name that dataPaths are prefixed with, "data" by default).
additionalProperties
keyword in schema (and no validation is made for them). If the option is true
(or truthy), only additional properties with additionalProperties
keyword equal to false
are removed. If the option is 'failing', then additional properties that fail schema validation will be removed too (where additionalProperties
keyword is schema).false
not to validate formats at all. E.g., 25:00:00 and 2015/14/33 will be invalid time and date in 'full' mode but it will be valid in 'fast' mode.addFormat
method.addSchema(value, key)
will be called for each schema in this object.$schema
property in the schema can either be http://json-schema.org/draft-04/schema or absent (draft-4 meta-schema will be used) or can be a reference to the schema previously added with addMetaSchema
method. If the validation fails, the exception is thrown. Pass "log" in this option to log error instead of throwing exception. Pass false
to skip schema validation.uniqueItems
keyword (true by default).false
to use .length
of strings that is faster, but gives "incorrect" lengths of strings with unicode pairs - each unicode pair is counted as two characters.npm install js-beautify
to use this option. true
or js-beautify options can be passed.put(key, value)
, get(key)
and del(key)
.npm install
git submodule update --init
npm test
Browser:
bin/prepare-tests
karma start
All validation functions are generated using doT templates in dot folder. Templates are precompiled so doT is not a run-time dependency.
bin/compile-dots
- compiles templates to dotjs folder (please use node 0.10 to compile - 0.12 is fully supported but it inserts some empty comments in function parameters when Function constructor is called).
bin/watch-dots
- automatically compiles templates when files in dot folder change
bin/git-hook
- installs symbolic link to pre-commit hook that will compile templates and run tests.
Only compile schemas when they are used first time.
Order in which schemas are added is not important.
Circular references are supported.
addShema
no longer returns compiled schema(s).
Improved / fixed compilation of recursive schemas.
If cache instance is supplied it must have put
, get
and del
methods.
Improved/fixed data filtering with removeAdditional
option.
removeAdditional
option allowing to remove additional properties.
addSchema
no longer compiles schemas and its return value is undefined
Dependencies can be added in any order (all dependencies should be present when the schema is compiled though)
Circular dependencies support
Errors for "required" keyword validation include missing properties
Better references resolution in schemas without IDs
cache
option and removeSchema
method
doT is no longer a run-time dependency
ajv can be used in the browser (with browserify)
Schemas are validated against meta-schema before compilation
Custom formats support.
Errors are set to null
if there are no errors (previously empty array).
FAQs
Another JSON Schema Validator
The npm package ajv receives a total of 60,882,349 weekly downloads. As such, ajv popularity was classified as popular.
We found that ajv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.