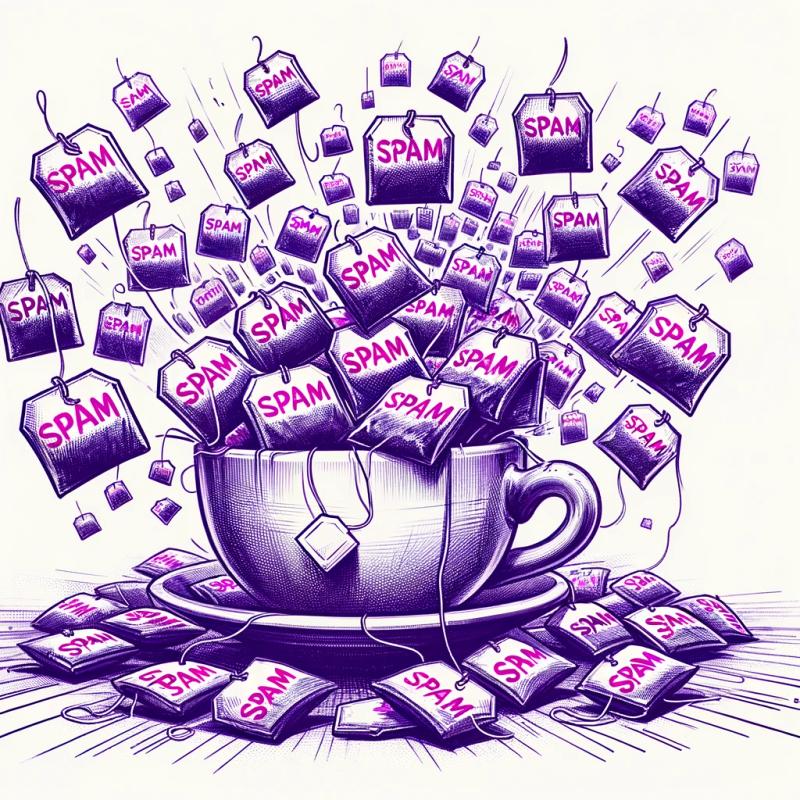
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
algebra-ring
Advanced tools
Readme
defines an algebra ring structure
Installation | Example | API | License
With npm do
npm install algebra-ring
All code in the examples below is intended to be contained into a single file.
Create a ring structure over real numbers.
const ring = require('algebra-ring')
// Define operators.
function contains (a) {
// NaN, Infinity and -Infinity are not allowed
return (typeof a === 'number' && isFinite(a))
}
function equality (a, b) { return a === b }
function addition (a, b) { return a + b }
function negation (a) { return -a }
function multiplication (a, b) { return a * b }
function inversion (a) { return 1 / a }
// Create a ring by defining its identities and operators.
const R = ring([0, 1], {
equality: equality,
contains: contains,
addition: addition,
negation: negation,
multiplication: multiplication,
inversion: inversion
})
You get a Ring that is a Group with multiplication operator. The multiplication operator must be closed respect the underlying set; its inverse operator is division.
This is the list of ring operators:
The neutral element for addition and multiplication are, as usual, called zero and one respectively.
R.contains(10) // true
R.contains(-1, 0.5, Math.PI, 5) // true
R.notContains(Infinity) // true
R.addition(1, 2) // 3
R.addition(2, 3, 5, 7) // 17
R.equality(R.negation(2), -2) // true
R.subtraction(2, 3) // -1
R.multiplication(2, 5) // 10
R.multiplication(2, 2, 2, 2) // 16
R.equality(R.inversion(10), 0.1) // true
R.division(1, 2) // 0.5
R.equality(R.addition(2, R.zero), 2) // true
R.equality(R.subtraction(2, 2), R.zero) // true
R.equality(R.multiplication(2, R.one), 2) // true
R.equality(R.division(2, 2), R.one) // true
R.division(1, 0) // will complain
R.inversion(R.zero) // will complain too
It is possible to create a ring over the booleans.
const Boole = ring([false, true], {
equality: (a, b) => (a === b),
contains: (a) => (typeof a === 'boolean'),
addition: (a, b) => (a || b),
negation: (a) => (a),
multiplication: (a, b) => (a && b),
inversion: (a) => (a)
})
There are only two elements, you know, true
and false
.
Boole.contains(true, false) // true
Check that false
is the neutral element of addition and true
is the
neutral element of multiplication.
Boole.addition(true, Boole.zero) // true
Boole.multiplication(true, Boole.one) // true
As usual, it is not allowed to divide by zero: the following code will throw.
Boole.division(true, false)
Boole.inversion(Bool.zero)
ring(identities, operator)
{Array}
identities{*}
identities[0] a.k.a zero{*}
identities1 a.k.a one{Object}
operator{Function}
operator.contains{Function}
operator.equality{Function}
operator.addition{Function}
operator.negation{Function}
operator.multiplication{Function}
operator.inversion{Object}
ringring.error
An object exposing the following error messages:
FAQs
defines an algebra ring structure
The npm package algebra-ring receives a total of 13 weekly downloads. As such, algebra-ring popularity was classified as not popular.
We found that algebra-ring demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.