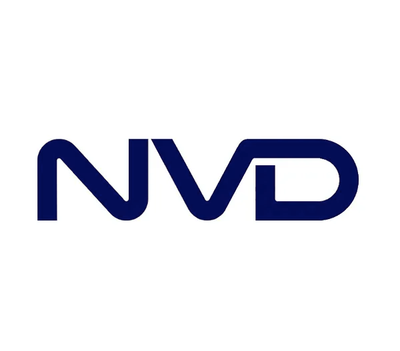
Security News
NVD Backlog Tops 20,000 CVEs Awaiting Analysis as NIST Prepares System Updates
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
amazon-ivs-chat-messaging
Advanced tools
The IVS Chat Messaging SDK makes it quick and easy to build excellent chat experience in your JavaScript or TypeScript app.
The IVS Chat Messaging SDK makes it quick and easy to build excellent chat experience in your JavaScript or TypeScript app.
Before starting, you should be familiar with Getting Started with Amazon IVS Chat.
See also SDK Documentation.
$ npm install --save amazon-ivs-chat-messaging
or
$ yarn add amazon-ivs-chat-messaging
This integration requires endpoints on your server that talk to the Amazon IVS Chat API. Use the official AWS libraries for access to the Amazon IVS API from your server. These are accessible within several languages from the public packages; e.g. node.js, java, go.
Next, create a server endpoint that talks to the AWS IVS Chat CreateChatToken endpoint in order to create a chat token for chat users. You can follow our sample backend implementation as a reference.
Create an asynchronous token provider function that fetches chat token from your backend.
type ChatTokenProvider = () => Promise<ChatToken>;
Function should accept no parameters and return a Promise containing chat token object.
Chat token object should have following shape:
type ChatToken = {
token: string;
sessionExpirationTime?: Date;
tokenExpirationTime?: Date;
}
This function is needed to instantiate ChatRoom
object in the next step.
// You will need to fetch a fresh token each time this method is called by
// the IVS Chat Messaging SDK, since each token is only accepted once.
function tokenProvider(): Promise<ChatToken> {
// Call you backend to fetch chat token from IVS Chat endpoint:
// e.g. const token = await appBackend.getChatToken()
return {
token: "<token>",
sessionExpirationTime: new Date("<date-time>"),
tokenExpirationTime: new Date("<date-time>")
}
}
Note: you need to fill in <token>
& <date-time>
fields with proper data received from you backend.
Create an instance of the ChatRoom
class. It requires passing regionOrUrl
, which is the AWS region in which your chat room is hosted, and tokenProvider
which is the token fetching method created in the previous step.
import { ChatRoom } from 'amazon-ivs-chat-messaging';
const room = new ChatRoom({
regionOrUrl: 'us-west-2',
tokenProvider,
});
Next, you should subscribe for chat room events in order to receive lifecycle events, as well as receive messages & events delivered in the chat room.
/**
* Called when room is establishing the initial connection or reestablishing
* connection after socket failure/token expiration/etc
*/
const unsubscribeOnConnecting = room.addListener('connecting', () => { });
/** Called when connection has been established. */
const unsubscribeOnConnected = room.addListener('connect', () => { });
/** Called when a room has been disconnected. */
const unsubscribeOnDisconnected = room.addListener('disconnect', () => { });
/** Called when chat message has been received. */
const unsubscribeOnMessageReceived = room.addListener('message', (message) => {
/* Example message:
* {
* id: "5OPsDdX18qcJ",
* sender: { userId: "user1" },
* content: "hello world",
* sendTime: new Date("2022-10-11T12:46:41.723Z"),
* requestId: "d1b511d8-d5ed-4346-b43f-49197c6e61de"
* }
*/
});
/** Called when chat event has been received. */
const unsubscribeOnEventReceived = room.addListener('event', (event) => {
/* Example event:
* {
* id: "5OPsDdX18qcJ",
* eventName: "customEvent,
* sendTime: new Date("2022-10-11T12:46:41.723Z"),
* requestId: "d1b511d8-d5ed-4346-b43f-49197c6e61de",
* attributes: { "Custom Attribute": "Custom Attribute Value" }
* }
*/
});
/** Called when `aws:DELETE_MESSAGE` system event has been received. */
const unsubscribeOnMessageDelete = room.addListener('messageDelete', (deleteMessageEvent) => {
/* Example delete message event:
* {
* id: "AYk6xKitV4On",
* messageId: "R1BLTDN84zEO",
* reason: "Spam",
* sendTime: new Date("2022-10-11T12:56:41.113Z"),
* requestId: "b379050a-2324-497b-9604-575cb5a9c5cd",
* attributes: { MessageID: "R1BLTDN84zEO", Reason: "Spam" }
* }
*/
});
/** Called when `aws:DISCONNECT_USER` system event has been received. */
const unsubscribeOnUserDisconnect = room.addListener('userDisconnect', (disconnectUserEvent) => {
/* Example event payload:
* {
* id: "AYk6xKitV4On",
* userId": "R1BLTDN84zEO",
* reason": "Spam",
* sendTime": new Date("2022-10-11T12:56:41.113Z"),
* requestId": "b379050a-2324-497b-9604-575cb5a9c5cd",
* attributes": { UserId: "R1BLTDN84zEO", Reason: "Spam" }
* }
*/
});
The last step of the basic initialization is connecting to the chat room by establishing the WebSocket connection. In order to do that simply call a connect()
method within the room instance.
room.connect();
The SDK will attempt to establish a connection to chat room encoded in chat token received from your server.
After calling connect()
the room will transition into connecting
state and will also emit a connecting
event. When room successfully connects, it will transition into connected
state and will emit a connect
event.
In case of connection failure, which might happen either due to issues when fetching token or issues when connecting to WebSocket, the room will try to reconnect automatically up to number of times indicated by maxReconnectAttempts
constructor parameter. During the reconnection attempts the room will be in connecting
state and will not emit additional events. After exhausting the reconnect attempts the room will transition to disconnected
state and will emit a disconnect
event with relevant disconnect reason. In disconnected
state room will no longer attempt to connect, you will need to call connect()
again to trigger the connection process.
IVS Chat Messaging SDK offers user actions dedicated for sending messages, deleting messages and disconnecting other users.
Those are available on the ChatRoom
instance, and return Promise
object that allows you to receive request confirmation or rejection.
Note: that for this particular request you need to have a
SEND_MESSAGE
capacity encoded in your chat token.
To trigger a send-message request:
const request = new SendMessageRequest('Test Echo');
room.sendMessage(request);
If you are interested in receiving the confirmation or rejection of the request, you can
await
returned promise or usethen()
method:
try {
const message = await room.sendMessage(request);
// Message has been successfully send to chat room
} catch (error) {
// Message request has been rejected, inspect `error` parameter for details.
}
Note: that for this particular request you need to have a
DELETE_MESSAGE
capacity encoded in your chat token.
To trigger a delete-message request:
const request = new DeleteMessageRequest(messageId, 'Reason for deletion');
room.deleteMessage(request);
If you're interested receiving confirmation or rejection of the request, you can
await
returned promise or usethen()
method:
try {
const deleteMessageEvent = await room.deleteMessage(request);
// Message has been successfully deleted from chat room
} catch (error) {
// Delete message request has been rejected, inspect `error` parameter for details.
}
In order to disconnect another user for moderation purposes call disconnectUser()
method.
Note: that for this particular request you need to have a
DISCONNECT_USER
capacity encoded in your chat token.
To disconnect another user for moderation purposes:
const request = new DisconnectUserRequest(userId, 'Reason for disconnecting user');
room.disconnectUser(request);
If you're interested receiving confirmation or rejection of the request, you can await
returned promise or use then()
method:
try {
const disconnectUserEvent = await room.disconnectUser(request);
// User has been successfully disconnected from the chat room
} catch (error) {
// Disconnect user request has been rejected, inspect `error` parameter for details.
}
In order to close your connection to the chat room, call the disconnect()
method on the room
instance:
room.disconnect();
Calling this method will cause room to closes the underlying Web Socket in an orderly manner. The room instance will transition to a disconnected state and will emit a disconnect event, with disconnect reason being set to "clientDisconnect"
.
Amazon Interactive Video Service (IVS) (https://docs.aws.amazon.com/ivs/) Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
Provided as AWS Content and subject to the AWS Customer Agreement (https://aws.amazon.com/agreement/) and any other agreement with AWS governing your use of AWS services.
See full license text for more information.
FAQs
The IVS Chat Messaging SDK makes it quick and easy to build excellent chat experience in your JavaScript or TypeScript app.
The npm package amazon-ivs-chat-messaging receives a total of 2,417 weekly downloads. As such, amazon-ivs-chat-messaging popularity was classified as popular.
We found that amazon-ivs-chat-messaging demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.