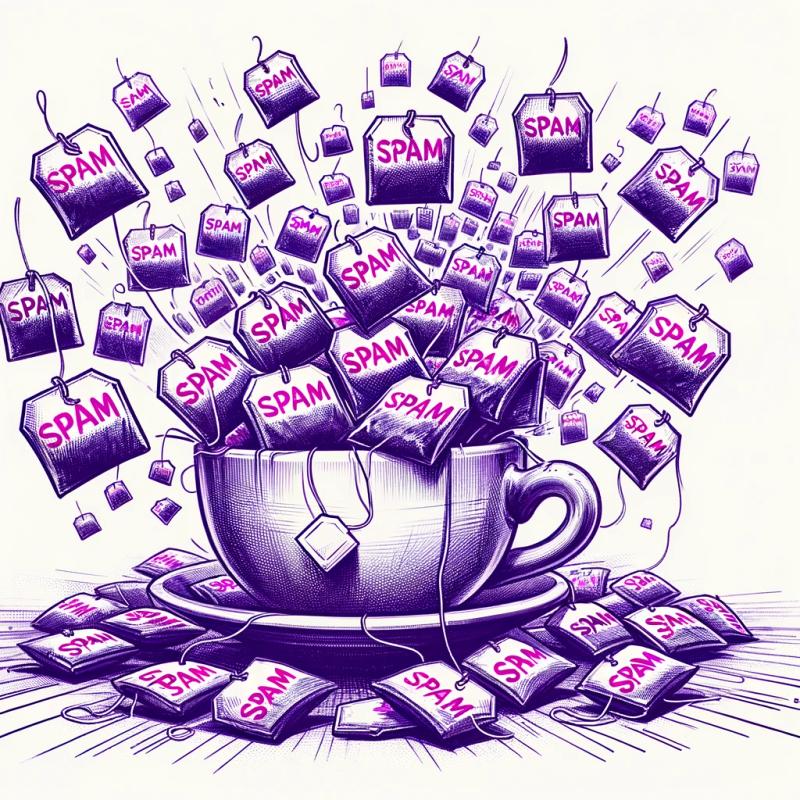
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
amqp-cacoon
Advanced tools
Readme
This is a basic library to provide amqp support. This library is a wrapper around amqplib and makes amqp easier to work with.
If using macos brew cask install docker
should install both docker and docker-compose
docker-compose up -d
npm install
npm run test
This allows you to connect to an amqp server
const config = {
messageBus: {
// Protocol should be "amqp" or "amqps"
protocol: 'amqp',
// Username + Password on the RabbitMQ host
username: 'valtech',
password: 'iscool',
// Host
host: 'localhost',
// Port
port: 5672,
// Queue setup
testQueue: 'test-queue',
},
};
let amqpCacoon = new AmqpCacoon({
protocol: config.messageBus.protocol,
username: config.messageBus.username,
password: config.messageBus.password,
host: config.messageBus.host,
port: config.messageBus.port,
amqp_opts: {},
providers: {
logger: logger,
},
});
This allows you to publish a message
let channel = await amqpCacoon.getPublishChannel(); // Connects and sets up a publish channel
// Create queue and setup publish channel
channel.assertQueue(config.messageBus.testQueue);
// Publish
await amqpCacoon.publish(
'', // Publish directly to queue
config.messageBus.testQueue,
Buffer.from('TestMessage')
);
This will allow use to consume a single message.
let channel = await amqpCacoon.getConsumerChannel(); // Connects and sets up a subscription channel
// Create queue
channel.assertQueue(config.messageBus.testQueue);
// Consume single message at a time
amqpCacoon.registerConsumer(
config.messageBus.testQueue,
async (channel: Channel, msg: ConsumeMessage) => {
try {
console.log('Messsage content', msg.content.toString());
// ... Do other processing here
channel.ack(msg) // To ack a messages
} catch (e) {
channel.nack(msg) // To ack a messages
}
}
);
This allows you to wait until either some time has elapsed or a specified message size has been exceeded before the messages are consumed
// Consume batch of message at a time. Configuration for time based or size based batching is provided
amqpCacoon.registerConsumerBatch(
config.messageBus.testQueue,
async (channel: Channel, msg: ConsumeBatchMessages) => {
try {
console.log('Messsage content', msg.content.toString());
// ... Do other processing here
msg.ackAll() // To ack all messages
} catch (e) {
msg.nackAll() // To nack all messages
}
},
{
batching: {
maxTimeMs: 60000, // Don't provide messages to the callback until at least 60000 ms have passed
maxSizeBytes: 1024 * 1024 * 2, // 1024 * 1024 * 2 = 2 MB -don't provide message to the callback until 2 MB of data have been received
}
}
);
This library expose amqplib channel when you call either getConsumerChannel
or getPublishChannel
. The channel is also exposed when registering a consumer. To learn more about that api see documentation for amqplib. Just a couple thing that you should remember to do.
registerConsumer
to not require an ack (noAck). The problem with this is that if your application is reset or errors out, you may loose the message or messages.FAQs
AmqpCacoon is an abstraction around amqplib that provides a simple interface with flow control included out of the box
The npm package amqp-cacoon receives a total of 91 weekly downloads. As such, amqp-cacoon popularity was classified as not popular.
We found that amqp-cacoon demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.