angular-star-rating
Advanced tools
Comparing version 2.0.0-rc.5 to 2.0.0
@@ -1,135 +0,26 @@ | ||
import { OnChanges, EventEmitter } from "@angular/core"; | ||
import { starRatingSizes, starRatingSpeed, starRatingColor, starRatingPosition, starRatingStarSpace, starRatingStarTypes, IStarRatingOnClickEvent, IStarRatingOnRatingChangeEven, starRatingDirection } from "./star-rating-struct"; | ||
export declare class StarRatingComponent implements OnChanges { | ||
/** | ||
* _getStarsArray | ||
* | ||
* returns an array of increasing numbers starting at 1 | ||
* | ||
* @param numOfStars | ||
* @returns {Array} | ||
*/ | ||
static _getStarsArray(numOfStars: number): Array<number>; | ||
/** | ||
* _getHalfStarVisible | ||
* | ||
* Returns true if there should be a half star visible, and false if not. | ||
* | ||
* @param rating | ||
* @returns {boolean} | ||
*/ | ||
static _getHalfStarVisible(rating: number): boolean; | ||
/** | ||
* _getColor | ||
* | ||
* The default function for color calculation | ||
* based on the current rating and the the number of stars possible. | ||
* If a staticColor is set the function will use it as return value. | ||
* | ||
* @param rating | ||
* @param numOfStars | ||
* @param staticColor | ||
* @returns {starRatingColor} | ||
*/ | ||
static _getColor(rating: number, numOfStars: number, staticColor?: starRatingColor): starRatingColor; | ||
protected config: any; | ||
/** | ||
* id property to identify the DOM element | ||
*/ | ||
protected _id: string; | ||
id: string; | ||
/** | ||
* labelText | ||
*/ | ||
protected _labelText: string; | ||
labelText: string; | ||
/** | ||
* labelPosition | ||
*/ | ||
protected _labelPosition: starRatingPosition; | ||
labelPosition: starRatingPosition; | ||
/** | ||
* labelVisible | ||
*/ | ||
protected _labelVisible: boolean; | ||
labelVisible: boolean; | ||
/** | ||
* staticColor | ||
*/ | ||
protected _staticColor: starRatingColor; | ||
staticColor: starRatingColor; | ||
/** | ||
* direction | ||
*/ | ||
protected _direction: starRatingDirection; | ||
direction: starRatingDirection; | ||
/** | ||
* numOfStars | ||
*/ | ||
protected _numOfStars: number; | ||
numOfStars: number; | ||
/** | ||
* speed | ||
*/ | ||
protected _speed: starRatingSpeed; | ||
speed: starRatingSpeed; | ||
/** | ||
* size | ||
*/ | ||
protected _size: starRatingSizes; | ||
size: starRatingSizes; | ||
/** | ||
* starType | ||
*/ | ||
protected _starType: starRatingStarTypes; | ||
starType: starRatingStarTypes; | ||
/** | ||
* space | ||
*/ | ||
protected _space: starRatingStarSpace; | ||
space: starRatingStarSpace; | ||
/** | ||
* readOnly | ||
*/ | ||
protected _readOnly: boolean; | ||
readOnly: boolean; | ||
/** | ||
* disabled | ||
*/ | ||
protected _disabled: boolean; | ||
disabled: boolean; | ||
/** | ||
* rating | ||
*/ | ||
protected _rating: number; | ||
rating: number; | ||
/** | ||
* showHalfStars | ||
*/ | ||
protected _showHalfStars: boolean; | ||
showHalfStars: boolean; | ||
/** | ||
* getColor | ||
*/ | ||
getColor: (rating: number, numOfStars: number, staticColor?: starRatingColor) => starRatingColor; | ||
/** | ||
* getHalfStarVisible | ||
*/ | ||
getHalfStarVisible: (rating: number) => boolean; | ||
import { OnChanges, EventEmitter, OnInit } from "@angular/core"; | ||
import { IStarRatingOnClickEvent, IStarRatingOnRatingChangeEven } from "./star-rating-struct"; | ||
import { StarRatingController } from "./star-rating.controller"; | ||
import { ControlValueAccessor } from "@angular/forms"; | ||
export declare class StarRatingComponent extends StarRatingController implements OnChanges, OnInit, ControlValueAccessor { | ||
onClick: EventEmitter<IStarRatingOnClickEvent>; | ||
saveOnClick($event: IStarRatingOnClickEvent): void; | ||
onRatingChange: EventEmitter<IStarRatingOnRatingChangeEven>; | ||
classEmpty: string; | ||
classHalf: string; | ||
classFilled: string; | ||
pathEmpty: string; | ||
pathHalf: string; | ||
pathFilled: string; | ||
color: starRatingColor; | ||
stars: Array<number>; | ||
ratingAsInteger: number; | ||
halfStarVisible: boolean; | ||
saveOnRatingChange($event: IStarRatingOnRatingChangeEven): void; | ||
onTouch: Function; | ||
onModelChange: Function; | ||
private onModelChangeRegistered; | ||
private onTouchRegistered; | ||
saveOnTouch(): void; | ||
saveOnModelChange(value: number): void; | ||
/**ACCESSEBILITY **/ | ||
onKeyDown(event: KeyboardEvent): void; | ||
onBlur(event: FocusEvent): void; | ||
onFocus(event: FocusEvent): void; | ||
/**Form Control - ControlValueAccessor implementation**/ | ||
writeValue(obj: any): void; | ||
registerOnChange(fn: any): void; | ||
registerOnTouched(fn: any): void; | ||
constructor(); | ||
svgVisible(): boolean; | ||
setColor(): void; | ||
setHalfStarVisible(): void; | ||
setRating(value: number): void; | ||
/** | ||
@@ -145,4 +36,9 @@ * onStarClicked | ||
*/ | ||
protected onStarClicked(rating: number): void; | ||
onStarClicked(rating: number): void; | ||
/** | ||
* ngOnChanges | ||
* @param changes | ||
*/ | ||
ngOnChanges(changes: any): void; | ||
ngOnInit(): void; | ||
} |
@@ -13,233 +13,111 @@ "use strict"; | ||
const core_1 = require("@angular/core"); | ||
const star_rating_config_1 = require("./star-rating-config"); | ||
let StarRatingComponent = StarRatingComponent_1 = class StarRatingComponent { | ||
const star_rating_controller_1 = require("./star-rating.controller"); | ||
const forms_1 = require("@angular/forms"); | ||
const star_rating_utils_1 = require("./star-rating.utils"); | ||
const STAR_RATING_CONTROL_ACCESSOR = { | ||
provide: forms_1.NG_VALUE_ACCESSOR, | ||
useExisting: core_1.forwardRef(() => StarRatingComponent), | ||
multi: true | ||
}; | ||
let StarRatingComponent = class StarRatingComponent extends star_rating_controller_1.StarRatingController { | ||
constructor() { | ||
///////////////////////////////////////////// | ||
//Output | ||
super(); | ||
//Outputs | ||
/////////////////////////////////////////////////////////////////////////////////////////// | ||
this.onClick = new core_1.EventEmitter(); | ||
this.onRatingChange = new core_1.EventEmitter(); | ||
let config = new star_rating_config_1.StarRatingConfig(); | ||
//set default ctrl props | ||
this.classEmpty = config.classEmpty; | ||
this.classHalf = config.classHalf; | ||
this.classFilled = config.classFilled; | ||
this.pathEmpty = config.svgPathEmpty; | ||
this.pathHalf = config.svgPathHalf; | ||
this.pathFilled = config.svgPathFilled; | ||
//set default Component Inputs | ||
if ('getColor' in config && typeof config.getColor === "function") { | ||
this.getColor = config.getColor; | ||
this.onModelChangeRegistered = false; | ||
this.onTouchRegistered = false; | ||
} | ||
saveOnClick($event) { | ||
if (this.onClick) { | ||
this.onClick.emit($event); | ||
} | ||
if ('getHalfStarVisible' in config && typeof config.getHalfStarVisible === "function") { | ||
this.getHalfStarVisible = config.getHalfStarVisible; | ||
} | ||
saveOnRatingChange($event) { | ||
if (this.onRatingChange) { | ||
this.onRatingChange.emit($event); | ||
} | ||
this.numOfStars = config.numOfStars; | ||
this.rating = 0; | ||
} | ||
//Static methods | ||
/////////////////////////////////////////////////////////////////////////////////////////// | ||
/** | ||
* _getStarsArray | ||
* | ||
* returns an array of increasing numbers starting at 1 | ||
* | ||
* @param numOfStars | ||
* @returns {Array} | ||
*/ | ||
static _getStarsArray(numOfStars) { | ||
let stars = []; | ||
for (let i = 0; i < numOfStars; i++) { | ||
stars.push(i + 1); | ||
saveOnTouch() { | ||
if (this.onTouchRegistered) { | ||
this.onTouch(); | ||
} | ||
return stars; | ||
} | ||
/** | ||
* _getHalfStarVisible | ||
* | ||
* Returns true if there should be a half star visible, and false if not. | ||
* | ||
* @param rating | ||
* @returns {boolean} | ||
*/ | ||
static _getHalfStarVisible(rating) { | ||
return Math.abs(rating % 1) > 0; | ||
saveOnModelChange(value) { | ||
if (this.onModelChangeRegistered) { | ||
this.onModelChange(value); | ||
} | ||
} | ||
/** | ||
* _getColor | ||
* | ||
* The default function for color calculation | ||
* based on the current rating and the the number of stars possible. | ||
* If a staticColor is set the function will use it as return value. | ||
* | ||
* @param rating | ||
* @param numOfStars | ||
* @param staticColor | ||
* @returns {starRatingColor} | ||
*/ | ||
static _getColor(rating, numOfStars, staticColor) { | ||
rating = rating || 0; | ||
//if a fix color is set use this one | ||
if (staticColor) { | ||
return staticColor; | ||
/**ACCESSEBILITY **/ | ||
//Keyboard events | ||
onKeyDown(event) { | ||
const handlers = { | ||
//Decrement | ||
Minus: () => this.decrement(), | ||
ArrowDown: () => this.decrement(), | ||
ArrowLeft: () => this.decrement(), | ||
//Increment | ||
Plus: () => this.increment(), | ||
ArrowRight: () => this.increment(), | ||
ArrowUp: () => this.increment(), | ||
//Reset | ||
Backspace: () => this.reset(), | ||
Delete: () => this.reset(), | ||
Digit0: () => this.reset() | ||
}; | ||
const handleDigits = (eventCode) => { | ||
let dStr = "Digit"; | ||
let digit = parseInt(eventCode.substr(dStr.length, eventCode.length - 1)); | ||
this.rating = digit; | ||
}; | ||
if (handlers[event['code']] || star_rating_utils_1.StarRatingUtils.isDigitKeyEventCode(event['code'])) { | ||
if (star_rating_utils_1.StarRatingUtils.isDigitKeyEventCode(event['code'])) { | ||
handleDigits(event['code']); | ||
} | ||
else { | ||
handlers[event['code']](); | ||
} | ||
event.preventDefault(); | ||
event.stopPropagation(); | ||
} | ||
//calculate size of smallest fraction | ||
let fractionSize = numOfStars / 3; | ||
//apply color by fraction | ||
let color = 'default'; | ||
if (rating > 0) { | ||
color = 'negative'; | ||
} | ||
if (rating > fractionSize) { | ||
color = 'ok'; | ||
} | ||
if (rating > fractionSize * 2) { | ||
color = 'positive'; | ||
} | ||
return color; | ||
this.saveOnTouch(); | ||
} | ||
get id() { | ||
return this._id; | ||
//Focus events | ||
onBlur(event) { | ||
this.focus = false; | ||
event.preventDefault(); | ||
event.stopPropagation(); | ||
this.saveOnTouch(); | ||
} | ||
set id(value) { | ||
this._id = value || ''; | ||
onFocus(event) { | ||
this.focus = true; | ||
event.preventDefault(); | ||
event.stopPropagation(); | ||
this.saveOnTouch(); | ||
} | ||
get labelText() { | ||
return this._labelText; | ||
/**Form Control - ControlValueAccessor implementation**/ | ||
writeValue(obj) { | ||
this.rating = obj; | ||
} | ||
set labelText(value) { | ||
this._labelText = value; | ||
registerOnChange(fn) { | ||
this.onModelChange = fn; | ||
this.onModelChangeRegistered = true; | ||
} | ||
get labelPosition() { | ||
return this._labelPosition; | ||
registerOnTouched(fn) { | ||
this.onTouch = fn; | ||
this.onTouchRegistered = true; | ||
} | ||
set labelPosition(value) { | ||
this._labelPosition = value || this.config.labelPosition; | ||
} | ||
get labelVisible() { | ||
return this._labelVisible; | ||
} | ||
set labelVisible(value) { | ||
this._labelVisible = !!value; | ||
} | ||
get staticColor() { | ||
return this._staticColor; | ||
} | ||
set staticColor(value) { | ||
this._staticColor = value || undefined; | ||
//update color. | ||
this.setColor(); | ||
} | ||
get direction() { | ||
return this._direction; | ||
} | ||
set direction(value) { | ||
this._direction = value || undefined; | ||
} | ||
get numOfStars() { | ||
return this._numOfStars; | ||
} | ||
set numOfStars(value) { | ||
this._numOfStars = (value > 0) ? value : this.config.numOfStars; | ||
//update stars array | ||
this.stars = StarRatingComponent_1._getStarsArray(this.numOfStars); | ||
//update color | ||
this.setColor(); | ||
} | ||
get speed() { | ||
return this._speed; | ||
} | ||
set speed(value) { | ||
this._speed = value || this.config.speed; | ||
} | ||
get size() { | ||
return this._size; | ||
} | ||
set size(value) { | ||
this._size = value || this.config.size; | ||
} | ||
get starType() { | ||
return this._starType; | ||
} | ||
set starType(value) { | ||
this._starType = value || this.config.starType; | ||
} | ||
get space() { | ||
return this._space; | ||
} | ||
set space(value) { | ||
this._space = value; | ||
} | ||
get readOnly() { | ||
return this._readOnly; | ||
} | ||
set readOnly(value) { | ||
this._readOnly = !!value; | ||
} | ||
get disabled() { | ||
return this._disabled; | ||
} | ||
set disabled(value) { | ||
this._disabled = !!value; | ||
} | ||
get rating() { | ||
return this._rating; | ||
} | ||
set rating(value) { | ||
//validate and apply newRating | ||
let newRating = 0; | ||
if (value >= 0 | ||
&& value <= this.numOfStars) { | ||
newRating = value; | ||
//Overrides | ||
setRating(value) { | ||
let initValue = this.rating; | ||
super.setRating(value); | ||
//if value changed trigger valueAccessor events and outputs | ||
if (initValue !== this.rating) { | ||
let $event = { rating: this.rating }; | ||
this.saveOnRatingChange($event); | ||
this.saveOnModelChange(this.rating); | ||
} | ||
//limit max value to max number of stars | ||
if (value > this.numOfStars) { | ||
newRating = this.numOfStars; | ||
} | ||
this._rating = newRating; | ||
//update ratingAsInteger. rating parsed to int for the value-[n] modifier | ||
this.ratingAsInteger = parseInt(this._rating.toString()); | ||
//update halfStarsVisible | ||
this.setHalfStarVisible(); | ||
//update calculated Color | ||
this.setColor(); | ||
//fire onRatingChange event | ||
let $event = { rating: this._rating }; | ||
this.onRatingChange.emit($event); | ||
} | ||
get showHalfStars() { | ||
return this._showHalfStars; | ||
} | ||
set showHalfStars(value) { | ||
this._showHalfStars = !!value; | ||
//update halfStarVisible | ||
this.setHalfStarVisible(); | ||
} | ||
svgVisible() { | ||
return this.starType === "svg"; | ||
} | ||
setColor() { | ||
//check if custom function is given | ||
if (typeof this.getColor === "function") { | ||
this.color = this.getColor(this.rating, this.numOfStars, this.staticColor); | ||
} | ||
else { | ||
this.color = StarRatingComponent_1._getColor(this.rating, this.numOfStars, this.staticColor); | ||
} | ||
} | ||
setHalfStarVisible() { | ||
//update halfStarVisible | ||
if (this.showHalfStars) { | ||
//check if custom function is given | ||
if (typeof this.getHalfStarVisible === "function") { | ||
this.halfStarVisible = this.getHalfStarVisible(this.rating); | ||
} | ||
else { | ||
this.halfStarVisible = StarRatingComponent_1._getHalfStarVisible(this.rating); | ||
} | ||
} | ||
else { | ||
this.halfStarVisible = false; | ||
} | ||
} | ||
; | ||
/** | ||
@@ -265,4 +143,8 @@ * onStarClicked | ||
}; | ||
this.onClick.emit(onClickEventObject); | ||
this.saveOnClick(onClickEventObject); | ||
} | ||
/** | ||
* ngOnChanges | ||
* @param changes | ||
*/ | ||
ngOnChanges(changes) { | ||
@@ -280,3 +162,2 @@ let valueChanged = function (key, changes) { | ||
//--------------------------------------- | ||
//functions | ||
//boolean | ||
@@ -324,103 +205,47 @@ if (valueChanged('showHalfStars', changes)) { | ||
} | ||
if (valueChanged('step', changes)) { | ||
this.step = changes['step'].currentValue; | ||
} | ||
} | ||
ngOnInit() { | ||
console.log('ngOnInit'); | ||
this.saveOnModelChange(this.rating); | ||
} | ||
}; | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "id", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "labelText", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "labelPosition", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", Boolean), | ||
__metadata("design:paramtypes", [Boolean]) | ||
], StarRatingComponent.prototype, "labelVisible", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "staticColor", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "direction", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", Number), | ||
__metadata("design:paramtypes", [Number]) | ||
], StarRatingComponent.prototype, "numOfStars", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "speed", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "size", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "starType", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", String), | ||
__metadata("design:paramtypes", [String]) | ||
], StarRatingComponent.prototype, "space", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", Boolean), | ||
__metadata("design:paramtypes", [Boolean]) | ||
], StarRatingComponent.prototype, "readOnly", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", Boolean), | ||
__metadata("design:paramtypes", [Boolean]) | ||
], StarRatingComponent.prototype, "disabled", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", Number), | ||
__metadata("design:paramtypes", [Number]) | ||
], StarRatingComponent.prototype, "rating", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", Boolean), | ||
__metadata("design:paramtypes", [Boolean]) | ||
], StarRatingComponent.prototype, "showHalfStars", null); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", Function) | ||
], StarRatingComponent.prototype, "getColor", void 0); | ||
__decorate([ | ||
core_1.Input(), | ||
__metadata("design:type", Function) | ||
], StarRatingComponent.prototype, "getHalfStarVisible", void 0); | ||
__decorate([ | ||
core_1.Output(), | ||
__metadata("design:type", core_1.EventEmitter) | ||
], StarRatingComponent.prototype, "onClick", void 0); | ||
__decorate([ | ||
core_1.Output(), | ||
__metadata("design:type", core_1.EventEmitter) | ||
], StarRatingComponent.prototype, "onRatingChange", void 0); | ||
StarRatingComponent = StarRatingComponent_1 = __decorate([ | ||
StarRatingComponent = __decorate([ | ||
core_1.Component({ | ||
selector: 'star-rating-comp', | ||
providers: [STAR_RATING_CONTROL_ACCESSOR], | ||
inputs: [ | ||
'getHalfStarVisible', | ||
'getColor', | ||
'showHalfStars', | ||
'rating', | ||
'step', | ||
'disabled', | ||
'readOnly', | ||
'space', | ||
'starType', | ||
'size', | ||
'speed', | ||
'numOfStars', | ||
'direction', | ||
'staticColor', | ||
'labelVisible', | ||
'labelPosition', | ||
'labelText', | ||
'id' | ||
], | ||
outputs: [ | ||
'onClick', | ||
'onRatingChange' | ||
], | ||
//templateUrl: 'star-rating.component.html', | ||
template: ` | ||
<div id="{{id}}" | ||
class="rating {{rating?'value-'+ratingAsInteger:'value-0'}} {{readOnly?'read-only':''}} {{disabled?'disabled':''}} {{halfStarVisible?'half':''}} {{space?'space-'+space:''}} {{labelVisible?'label-'+labelVisible:''}} {{labelPosition?'label-'+labelPosition:''}} {{color?'color-'+color:''}} {{starType?'star-'+starType:''}} {{speed}} {{size}} {{direction?'direction-'+direction:''}}" | ||
> | ||
<div id="{{id}}" | ||
class="rating {{getComponentClassNames()}}" | ||
tabindex="0" | ||
(keydown)="onKeyDown($event)" | ||
(blur)="onBlur($event)" | ||
(focus)="onFocus($event)"> | ||
<div *ngIf="labelVisible" class="label-value">{{labelText}}</div> | ||
@@ -454,3 +279,2 @@ <div class="star-container"> | ||
exports.StarRatingComponent = StarRatingComponent; | ||
var StarRatingComponent_1; | ||
//# sourceMappingURL=star-rating.component.js.map |
{ | ||
"name": "angular-star-rating", | ||
"description": "Angular Star Rating is a Angular2 component written in typescript.", | ||
"version": "2.0.0-rc.5", | ||
"version": "2.0.0", | ||
"scripts": { | ||
@@ -10,6 +10,6 @@ "build": "ngc -p tsconfig.json", | ||
"prepublish": "npm run tsc", | ||
"tsc": "npm run deleteDist && tsc && npm run copyAsstes", | ||
"deleteDist":"rimraf dist", | ||
"copyAsstes" : "copyfiles assets/**/* dist", | ||
"tsc": "npm run deleteDist && tsc && npm run copyAssets", | ||
"deleteDist": "rimraf dist", | ||
"copyAssets": "copyfiles assets/**/* dist", | ||
"updateExample": "npm run tsc && copyfiles dist/**/* examples/angular2/node_modules/angular-star-rating/dist", | ||
"coverage": "http-server -c-1 -o -p 9875 ./coverage", | ||
@@ -57,4 +57,3 @@ "changelog": "conventional-changelog -p angular -i CHANGELOG.md -s", | ||
"main": "./dist/index.js", | ||
"dependencies": { | ||
}, | ||
"dependencies": {}, | ||
"devDependencies": { | ||
@@ -65,2 +64,3 @@ "@angular/common": "^4.0.0", | ||
"@angular/core": "^4.0.0", | ||
"@angular/forms": "^4.0.0", | ||
"@angular/platform-browser": "^4.0.0", | ||
@@ -77,5 +77,5 @@ "@angular/platform-browser-dynamic": "^4.0.0", | ||
"karma-cli": "~1.0.1", | ||
"karma-coverage-istanbul-reporter": "^0.2.0", | ||
"karma-jasmine": "~1.1.0", | ||
"karma-jasmine-html-reporter": "^0.2.2", | ||
"karma-coverage-istanbul-reporter": "^0.2.0", | ||
"protractor": "~5.1.0", | ||
@@ -82,0 +82,0 @@ "rxjs": "^5.1.0", |
332
README.MD
# Angular Star Rating ⭐⭐⭐⭐⭐ | ||
#### ⭐ Angular Component written in typescript, based on css only techniques. ⭐ | ||
#### ⭐ Angular Star Rating is a >2 Angular made with ❤, based on css only techniques. ⭐ | ||
 | ||
 | ||
[](https://www.npmjs.com/package/angular-star-rating) | ||
[](https://github.com/BioPhoton/angular-star-rating) | ||
[](https://www.npmjs.com/package/angular-star-rating) | ||
[](https://npmjs.org/angular-star-rating) | ||
[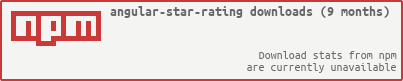](https://npmjs.org/angular-star-rating) | ||
Angular Star Rating is a >2 Angular made with ❤. | ||
It is based on best practice UX/UI methods, accessibility in mind and an eye for details. | ||
In love with reactive forms the easy to control over the keybord it is a perfect fit for all angular projects with ⭐'s. | ||
To keep it as flexible as possible a major part of the logic is based on [css only techniques](https://github.com/BioPhoton/css-star-rating). The component simple applies the state depending css modifiers. | ||
[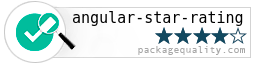](http://packagequality.com/#?package=angular-star-rating) | ||
Angular Star Rating is a >2 Angular component written in typescript. | ||
It is based on [css-sar-rating](https://github.com/BioPhoton/css-star-rating), a fully featured and customizable css only star rating component written in scss. | ||
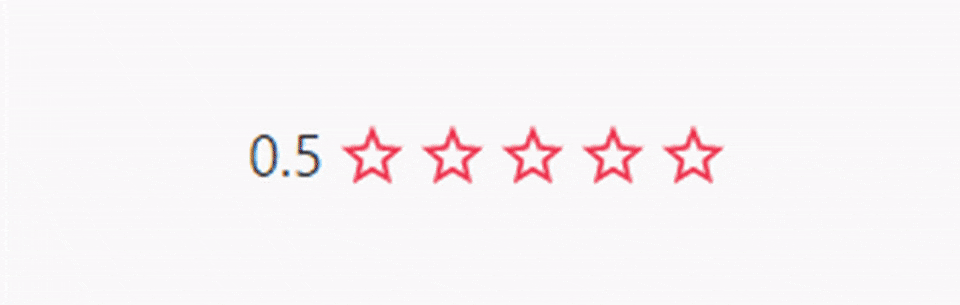 | ||
## DEMO | ||
- [x] [Example-App](https://github.com/BioPhoton/angular-star-rating/tree/master/examples/angular2) | ||
## Related Projects | ||
| Css | Angular1 (>=1.5)| Angular (>=2) | | ||
|--- |--- |--- | | ||
| <img src="https://raw.githubusercontent.com/BioPhoton/angular1-star-rating/dev/resources/family/css3.png" width="100"> | <img src="https://raw.githubusercontent.com/BioPhoton/angular1-star-rating/dev/resources/family/angular1.png" width="100"> | <img src="https://raw.githubusercontent.com/BioPhoton/angular1-star-rating/dev/resources/family/angular.png" width="80"> | | ||
| [Css Star Rating](https://github.com/BioPhoton/css-star-rating) | [Angular1 Star Rating](https://github.com/BioPhoton/angular1-star-rating) | [Angular Star Rating](https://github.com/BioPhoton/angular-star-rating) | | ||
## Features | ||
This module implements all Features from [CSS-STAR-RATING](https://github.com/BioPhoton/css-star-rating). | ||
It also provides callbacks for all calculation functions used in the component as well as all possible event emitters. | ||
- [x] **id** - The html id attribute of the star rating | ||
- [x] **rating** - The actual Star rating | ||
- [x] **showHalfStars** - To display half stars or not | ||
- [x] **numOfStars** - The max number of stars you can rate | ||
- [x] **size** - The different sizes of the component | ||
- [x] **space** - The space between the stars | ||
- [x] **staticColor** - A static color for the stars | ||
- [x] **disabled** - Component is in disabled mode | ||
- [x] **starType** - Stars can be displayed as svg, character or icon-font like fontawesome, glyphicons or ionicons | ||
- [x] **labelText** - The value of the label text | ||
- [x] **labelPosition** - The position of the label text | ||
- [x] **labelVisible** - The visibility of the label text | ||
- [x] **speed** - The duration of the animation | ||
- [x] **direction** - The direction of the component i.e. right to left | ||
- [x] **readOnly** - Click event is disabled | ||
- [x] **getColor** - Custom function to calculate the color for a rating | ||
- [x] **getHalfStarVisible** - Custom function to calculate value for displaying half stars or not | ||
- [x] **onClick** - Hook for Click action | ||
- [x] **onRatingChange** - Hook for onRatingChange event | ||
## Browser support | ||
@@ -65,2 +27,20 @@ | ||
## Features | ||
Fully featured this component is provided with: | ||
- [x] easy **configurable** and reasonable defaults | ||
- [x] all **12 css modifiers** of the awesome [css only star rating](https://github.com/BioPhoton/css-star-rating) library | ||
- [x] it integrates well with **reactive forms** and all it's states | ||
- [x] focus and blur events are handled for a **smooth keyboard navigation** | ||
- [x] it's **keyboard control** is even better than a native input once :-) | ||
- [x] importable as a **angular ngModule** it is a plug and play to use | ||
## Related Projects | ||
| Css | Angular 1 | Angular | Ionic 1 | | ||
|--- |--- |--- |--- | | ||
| <img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/family/css3.png" width="100"> | <img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/family/angular1.png" width="100"> | <img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/family/angular.png" width="80"> | <img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/family/ionic1.png" width="80"> | | ||
| [Css Star Rating](https://github.com/BioPhoton/css-star-rating) | [Angular1 Star Rating](https://github.com/BioPhoton/angular1-star-rating) | [Angular Star Rating](https://github.com/BioPhoton/angular-star-rating) |[Ionic1 Star Rating](https://github.com/BioPhoton/ionic1-star-rating) | | ||
## DEMO | ||
- [x] [Example-App](https://github.com/BioPhoton/angular-star-rating/tree/master/examples/angular2) | ||
## Installation | ||
@@ -131,3 +111,3 @@ | ||
<!-- USE COMPONENT HERE--> | ||
<star-rating-comp [rating]="2.63"></star-rating-comp> | ||
<star-rating-comp [startype]="'svg'" [rating]="2.63"></star-rating-comp> | ||
``` | ||
@@ -139,42 +119,110 @@ | ||
## Component Properties | ||
## Implement the output handler | ||
### Inputs | ||
```typescript | ||
import {Component} from "@angular/core"; | ||
import {FormGroup, FormControl} from "@angular/forms"; | ||
import {IStarRatingOnClickEvent, IStarRatingOnRatingChangeEven} from "angular-star-rating/src/star-rating-struct"; | ||
**id**: string (Optional) | ||
The html id attribute of the star rating | ||
Default: undefined | ||
@Component({ | ||
selector: 'my-event-component', | ||
template: ` | ||
<star-rating-comp [starType]="'svg'" | ||
(onClick)="onClick($event)" | ||
(onRatingChange)="onRatingChange($event)"> | ||
</star-rating-comp> | ||
<p>onClickResult: {{onClickResult | json}}</p> | ||
<p>onRatingChangeResult: {{onRatingChangeResult | json}}</p> | ||
` | ||
}) | ||
export class MyEventsComponent { | ||
onClickResult:IStarRatingOnClickEvent; | ||
onRatingChangeResult:IStarRatingOnRatingChangeEven; | ||
onClick = ($event:IStarRatingOnClickEvent) => { | ||
console.log('onClick $event: ', $event); | ||
this.onClickResult = $event; | ||
}; | ||
onRatingChange = ($event:IStarRatingOnRatingChangeEven) => { | ||
console.log('onRatingUpdated $event: ', $event); | ||
this.onRatingChangeResult = $event; | ||
}; | ||
} | ||
``` | ||
```html | ||
<star-rating-comp [id]="'my-id'"></star-rating-comp> | ||
<!-- app.component.html--> | ||
<my-event-component></my-event-component> | ||
``` | ||
**rating**: number (Optional) | ||
The actual star rating value | ||
Default: no | ||
## Use it with reactive forms | ||
```html | ||
<star-rating-comp [rating]="3"></star-rating-comp> | ||
As easy as it could be. Just apply the ```formControlName``` attribute to the star rating component. | ||
```typescript | ||
//my.component.ts | ||
import {Component} from "@angular/core"; | ||
import {FormGroup, FormControl} from "@angular/forms"; | ||
@Component({ | ||
selector: 'my-form-component', | ||
template: ` | ||
<form [formGroup]="form"> | ||
<star-rating-comp [starType]="'svg'" formControlName="myRatingControl" ></star-rating-comp> | ||
<pre>{{ form.value | json }}</pre> | ||
</form> | ||
` | ||
}) | ||
export class MyFormComponent { | ||
form = new FormGroup({ | ||
myRatingControl: new FormControl('') | ||
}); | ||
} | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-value.PNG" width="290"> | ||
**showHalfStars**: boolean (Optional) | ||
To show half stars or not | ||
Options: true, false | ||
Default: false | ||
```html | ||
<star-rating-comp [showHalfStars]="true"></star-rating-comp> | ||
<!-- app.component.html--> | ||
<my-form-component></my-form-component> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-show_half_stars-false.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-show_half_stars-true.PNG" width="290"> | ||
**numOfStars**: number (Optional) | ||
The possible number of stars to choose from | ||
Default: 5 | ||
## Component Bindings | ||
### Modifier list | ||
- Label modifier | ||
- Label visible | ||
- Label text | ||
- Label position | ||
- Style modifier | ||
- Star type | ||
- Color | ||
- Size | ||
- Space | ||
- Speed | ||
- Direction | ||
- Disabled | ||
- Control modifier | ||
- Rating | ||
- Step | ||
- Number of stars | ||
- Show half stars | ||
- Read only | ||
- Id | ||
- getColor | ||
- getHalfStarVisible | ||
#### Label Modifier | ||
**labelVisible**: string (Optional) | ||
The visibility of the label text. | ||
Default: true | ||
```html | ||
<star-rating-comp [numOfStars]="6"></star-rating-comp> | ||
<star-rating-comp [labelVisible]="false"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-num_of_stars.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-label-visible_false.PNG" width="290"> | ||
@@ -203,21 +251,26 @@ **labelText**: string (Optional) | ||
**labelVisible**: string (Optional) | ||
The visibility of the label text. | ||
Default: true | ||
#### Style Modifier | ||
**starType**: starRatingStarTypes (Optional) | ||
The type of start resource to use. | ||
Options: svg, icon | ||
Default: svg | ||
```html | ||
<star-rating-comp [labelVisible]="false"></star-rating-comp> | ||
<star-rating-comp [starType]="'icon'"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-label-visible_false.PNG" width="290"> | ||
**space**: starRatingStarSpace (Optional) | ||
If the start use the whole space or not. | ||
Options: no, between, around | ||
Default: no | ||
**staticColor**: starRatingColor (Optional) | ||
Possible color names for the stars. | ||
Options: default, negative, middle, positive | ||
Default: undefined | ||
```html | ||
<star-rating-comp [space]="'around'"></star-rating-comp> | ||
<star-rating-comp [staticColor]="'positive'"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-spread-false.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-spread-true.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-color-default.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-color-positive.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-color-middle.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-color-negative.PNG" width="290"> | ||
@@ -236,25 +289,28 @@ **size**: starRatingSizes (Optional) | ||
**staticColor**: starRatingColor (Optional) | ||
Possible color names for the stars. | ||
Options: default, negative, middle, positive | ||
Default: undefined | ||
**space**: starRatingStarSpace (Optional) | ||
If the start use the whole space or not. | ||
Options: no, between, around | ||
Default: no | ||
```html | ||
<star-rating-comp [staticColor]="'positive'"></star-rating-comp> | ||
<star-rating-comp [space]="'around'"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-color-default.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-color-positive.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-color-middle.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-color-negative.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-spread-false.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-spread-true.PNG" width="290"> | ||
**disabled**: boolean (Optional) | ||
The click callback is disabled, colors are transparent | ||
Default: false | ||
**speed**: starRatingSpeed (Optional) | ||
The duration of the animation in ms. | ||
Options: immediately, noticeable, slow | ||
Default: noticeable | ||
```html | ||
<star-rating-comp [disabled]="true"></star-rating-comp> | ||
<star-rating-comp [speed]="'slow'"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-disabled-false.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-disabled-true.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-animation_speed-immediately.gif" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-animation_speed-noticeable.gif" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-animation_speed-slow.gif" width="290"> | ||
**direction**: string (Optional) | ||
@@ -271,2 +327,53 @@ The direction of the stars and label. | ||
**disabled**: boolean (Optional) | ||
The click callback is disabled, colors are transparent | ||
Default: false | ||
```html | ||
<star-rating-comp [disabled]="true"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-disabled-false.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-disabled-true.PNG" width="290"> | ||
#### Cotrol modifier | ||
**rating**: number (Optional) | ||
The actual star rating value | ||
Default: no | ||
```html | ||
<star-rating-comp [rating]="3"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-value.PNG" width="290"> | ||
**step**: number (Optional) | ||
The step interval of the control | ||
Default: 1 | ||
```html | ||
<star-rating-comp [step]="0.5"></star-rating-comp> | ||
``` | ||
**numOfStars**: number (Optional) | ||
The possible number of stars to choose from | ||
Default: 5 | ||
```html | ||
<star-rating-comp [numOfStars]="6"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-num_of_stars.PNG" width="290"> | ||
**showHalfStars**: boolean (Optional) | ||
To show half stars or not | ||
Options: true, false | ||
Default: false | ||
```html | ||
<star-rating-comp [showHalfStars]="true"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-show_half_stars-false.PNG" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-show_half_stars-true.PNG" width="290"> | ||
**readOnly**: boolean (Optional) | ||
@@ -282,23 +389,11 @@ The click callback is disabled | ||
**speed**: starRatingSpeed (Optional) | ||
The duration of the animation in ms. | ||
Options: immediately, noticeable, slow | ||
Default: noticeable | ||
**id**: string (Optional) | ||
The html id attribute of the star rating | ||
Default: undefined | ||
```html | ||
<star-rating-comp [speed]="'slow'"></star-rating-comp> | ||
<star-rating-comp [id]="'my-id'"></star-rating-comp> | ||
``` | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-animation_speed-immediately.gif" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-animation_speed-noticeable.gif" width="290"> | ||
<img src="https://raw.githubusercontent.com/BioPhoton/angular-star-rating/master/resources/properties/prop-animation_speed-slow.gif" width="290"> | ||
**starType**: starRatingStarTypes (Optional) | ||
The type of start resource to use. | ||
Options: svg, icon | ||
Default: svg | ||
```html | ||
<star-rating-comp [starType]="'icon'"></star-rating-comp> | ||
``` | ||
**getColor**: Function (Optional) | ||
@@ -325,2 +420,3 @@ Calculation of the color by rating. | ||
### Outputs | ||
@@ -344,4 +440,12 @@ | ||
## Shields and other draft | ||
[](https://github.com/BioPhoton/angular-star-rating) | ||
[](https://www.npmjs.com/package/angular-star-rating) | ||
[](https://npmjs.org/angular-star-rating) | ||
[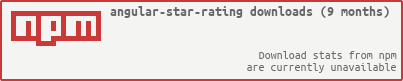](https://npmjs.org/angular-star-rating) | ||
[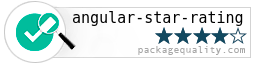](http://packagequality.com/#?package=angular-star-rating) | ||
## License | ||
MIT © [Michael Hladky](mailto:michael@hladky.at) |
@@ -0,0 +0,0 @@ export type starRatingSizes = "small" | "medium" | "large"; |
@@ -1,21 +0,50 @@ | ||
import {Component, Input, OnChanges, Output, EventEmitter} from "@angular/core"; | ||
import { | ||
starRatingSizes, | ||
starRatingSpeed, | ||
starRatingColor, | ||
starRatingPosition, | ||
starRatingStarSpace, | ||
starRatingStarTypes, | ||
IStarRatingOnClickEvent, | ||
IStarRatingOnRatingChangeEven, starRatingDirection | ||
} from "./star-rating-struct"; | ||
import {StarRatingConfig} from "./star-rating-config"; | ||
import {Component, OnChanges, EventEmitter, forwardRef, OnInit} from "@angular/core"; | ||
import {IStarRatingOnClickEvent, IStarRatingOnRatingChangeEven} from "./star-rating-struct"; | ||
import {StarRatingController} from "./star-rating.controller"; | ||
import {ControlValueAccessor, NG_VALUE_ACCESSOR} from "@angular/forms"; | ||
import {StarRatingUtils} from "./star-rating.utils"; | ||
const STAR_RATING_CONTROL_ACCESSOR = { | ||
provide: NG_VALUE_ACCESSOR, | ||
useExisting: forwardRef(() => StarRatingComponent), | ||
multi: true | ||
}; | ||
@Component({ | ||
selector: 'star-rating-comp', | ||
providers: [STAR_RATING_CONTROL_ACCESSOR], | ||
inputs: [ | ||
'getHalfStarVisible' | ||
, 'getColor' | ||
, 'showHalfStars' | ||
, 'rating' | ||
, 'step' | ||
, 'disabled' | ||
, 'readOnly' | ||
, 'space' | ||
, 'starType' | ||
, 'size' | ||
, 'speed' | ||
, 'numOfStars' | ||
, 'direction' | ||
, 'staticColor' | ||
, 'labelVisible' | ||
, 'labelPosition' | ||
, 'labelText' | ||
, 'id' | ||
], | ||
outputs: [ | ||
'onClick' | ||
, 'onRatingChange' | ||
], | ||
//templateUrl: 'star-rating.component.html', | ||
template: ` | ||
<div id="{{id}}" | ||
class="rating {{rating?'value-'+ratingAsInteger:'value-0'}} {{readOnly?'read-only':''}} {{disabled?'disabled':''}} {{halfStarVisible?'half':''}} {{space?'space-'+space:''}} {{labelVisible?'label-'+labelVisible:''}} {{labelPosition?'label-'+labelPosition:''}} {{color?'color-'+color:''}} {{starType?'star-'+starType:''}} {{speed}} {{size}} {{direction?'direction-'+direction:''}}" | ||
> | ||
<div id="{{id}}" | ||
class="rating {{getComponentClassNames()}}" | ||
tabindex="0" | ||
(keydown)="onKeyDown($event)" | ||
(blur)="onBlur($event)" | ||
(focus)="onFocus($event)"> | ||
<div *ngIf="labelVisible" class="label-value">{{labelText}}</div> | ||
@@ -46,444 +75,134 @@ <div class="star-container"> | ||
}) | ||
export class StarRatingComponent implements OnChanges { | ||
export class StarRatingComponent extends StarRatingController implements OnChanges, OnInit, ControlValueAccessor { | ||
//Static methods | ||
//Outputs | ||
/////////////////////////////////////////////////////////////////////////////////////////// | ||
/** | ||
* _getStarsArray | ||
* | ||
* returns an array of increasing numbers starting at 1 | ||
* | ||
* @param numOfStars | ||
* @returns {Array} | ||
*/ | ||
static _getStarsArray(numOfStars: number): Array<number> { | ||
let stars: Array<number> = []; | ||
for (let i = 0; i < numOfStars; i++) { | ||
stars.push(i + 1); | ||
onClick: EventEmitter<IStarRatingOnClickEvent> = new EventEmitter<IStarRatingOnClickEvent>(); | ||
saveOnClick($event:IStarRatingOnClickEvent) { | ||
if(this.onClick) { | ||
this.onClick.emit($event); | ||
} | ||
return stars; | ||
} | ||
/** | ||
* _getHalfStarVisible | ||
* | ||
* Returns true if there should be a half star visible, and false if not. | ||
* | ||
* @param rating | ||
* @returns {boolean} | ||
*/ | ||
static _getHalfStarVisible(rating: number): boolean { | ||
return Math.abs(rating % 1) > 0; | ||
} | ||
onRatingChange: EventEmitter<IStarRatingOnRatingChangeEven> = new EventEmitter<IStarRatingOnRatingChangeEven>(); | ||
/** | ||
* _getColor | ||
* | ||
* The default function for color calculation | ||
* based on the current rating and the the number of stars possible. | ||
* If a staticColor is set the function will use it as return value. | ||
* | ||
* @param rating | ||
* @param numOfStars | ||
* @param staticColor | ||
* @returns {starRatingColor} | ||
*/ | ||
static _getColor(rating: number, numOfStars: number, staticColor?: starRatingColor): starRatingColor { | ||
rating = rating || 0; | ||
//if a fix color is set use this one | ||
if (staticColor) { | ||
return staticColor; | ||
saveOnRatingChange($event:IStarRatingOnRatingChangeEven) { | ||
if(this.onRatingChange) { | ||
this.onRatingChange.emit($event); | ||
} | ||
//calculate size of smallest fraction | ||
let fractionSize = numOfStars / 3; | ||
//apply color by fraction | ||
let color: starRatingColor = 'default'; | ||
if (rating > 0) { | ||
color = 'negative'; | ||
} | ||
if (rating > fractionSize) { | ||
color = 'ok'; | ||
} | ||
if (rating > fractionSize * 2) { | ||
color = 'positive'; | ||
} | ||
return color; | ||
} | ||
protected config:any; | ||
//Inputs | ||
/////////////////////////////////////////////////////////////////////////////////////////// | ||
onTouch: Function; | ||
onModelChange: Function; | ||
private onModelChangeRegistered: boolean = false; | ||
private onTouchRegistered: boolean = false; | ||
/** | ||
* id property to identify the DOM element | ||
*/ | ||
protected _id: string; | ||
get id(): string { | ||
return this._id; | ||
saveOnTouch() { | ||
if (this.onTouchRegistered) { | ||
this.onTouch(); | ||
} | ||
} | ||
@Input() | ||
set id(value: string) { | ||
this._id = value || ''; | ||
saveOnModelChange(value:number) { | ||
if (this.onModelChangeRegistered) { | ||
this.onModelChange(value); | ||
} | ||
} | ||
///////////////////////////////////////////// | ||
/**ACCESSEBILITY **/ | ||
/** | ||
* labelText | ||
*/ | ||
protected _labelText: string; | ||
get labelText(): string { | ||
return this._labelText; | ||
} | ||
//Keyboard events | ||
onKeyDown(event: KeyboardEvent) { | ||
@Input() | ||
set labelText(value: string) { | ||
this._labelText = value; | ||
} | ||
const handlers: any = { | ||
//Decrement | ||
Minus: () => this.decrement(), | ||
ArrowDown: () => this.decrement(), | ||
ArrowLeft: () => this.decrement(), | ||
///////////////////////////////////////////// | ||
//Increment | ||
Plus: () => this.increment(), | ||
ArrowRight: () => this.increment(), | ||
ArrowUp: () => this.increment(), | ||
/** | ||
* labelPosition | ||
*/ | ||
protected _labelPosition: starRatingPosition; | ||
get labelPosition(): starRatingPosition { | ||
return this._labelPosition; | ||
} | ||
//Reset | ||
Backspace: () => this.reset(), | ||
Delete: () => this.reset(), | ||
Digit0: () => this.reset() | ||
}; | ||
@Input() | ||
set labelPosition(value: starRatingPosition) { | ||
this._labelPosition = value || this.config.labelPosition; | ||
} | ||
const handleDigits = (eventCode: string): void => { | ||
let dStr = "Digit"; | ||
let digit: number = parseInt(eventCode.substr(dStr.length, eventCode.length-1)); | ||
this.rating = digit; | ||
}; | ||
///////////////////////////////////////////// | ||
if (handlers[event['code']] || StarRatingUtils.isDigitKeyEventCode(event['code'])) { | ||
if (StarRatingUtils.isDigitKeyEventCode(event['code'])) { | ||
handleDigits(event['code']); | ||
} else { | ||
handlers[event['code']](); | ||
} | ||
event.preventDefault(); | ||
event.stopPropagation(); | ||
} | ||
/** | ||
* labelVisible | ||
*/ | ||
protected _labelVisible: boolean; | ||
get labelVisible(): boolean { | ||
return this._labelVisible; | ||
this.saveOnTouch(); | ||
} | ||
@Input() | ||
set labelVisible(value: boolean) { | ||
this._labelVisible = !!value; | ||
//Focus events | ||
onBlur(event: FocusEvent) { | ||
this.focus = false; | ||
event.preventDefault(); | ||
event.stopPropagation(); | ||
this.saveOnTouch(); | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* staticColor | ||
*/ | ||
protected _staticColor: starRatingColor; | ||
get staticColor(): starRatingColor { | ||
return this._staticColor; | ||
onFocus(event: FocusEvent) { | ||
this.focus = true; | ||
event.preventDefault(); | ||
event.stopPropagation(); | ||
this.saveOnTouch(); | ||
} | ||
@Input() | ||
set staticColor(value: starRatingColor) { | ||
this._staticColor = value || undefined; | ||
/**Form Control - ControlValueAccessor implementation**/ | ||
//update color. | ||
this.setColor(); | ||
writeValue(obj: any): void { | ||
this.rating = obj; | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* direction | ||
*/ | ||
protected _direction: starRatingDirection; | ||
get direction(): starRatingDirection { | ||
return this._direction; | ||
registerOnChange(fn: any): void { | ||
this.onModelChange = fn; | ||
this.onModelChangeRegistered = true; | ||
} | ||
@Input() | ||
set direction(value: starRatingDirection) { | ||
this._direction = value || undefined; | ||
registerOnTouched(fn: any): void { | ||
this.onTouch = fn; | ||
this.onTouchRegistered = true; | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* numOfStars | ||
*/ | ||
protected _numOfStars: number; | ||
get numOfStars(): number { | ||
return this._numOfStars; | ||
} | ||
@Input() | ||
set numOfStars(value: number) { | ||
this._numOfStars = (value > 0) ? value : this.config.numOfStars; | ||
//update stars array | ||
this.stars = StarRatingComponent._getStarsArray(this.numOfStars); | ||
//update color | ||
this.setColor(); | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* speed | ||
*/ | ||
protected _speed: starRatingSpeed; | ||
get speed(): starRatingSpeed { | ||
return this._speed; | ||
} | ||
@Input() | ||
set speed(value: starRatingSpeed) { | ||
this._speed = value || this.config.speed; | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* size | ||
*/ | ||
protected _size: starRatingSizes; | ||
get size(): starRatingSizes { | ||
return this._size; | ||
} | ||
@Input() | ||
set size(value: starRatingSizes) { | ||
this._size = value || this.config.size; | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* starType | ||
*/ | ||
protected _starType: starRatingStarTypes; | ||
get starType(): starRatingStarTypes { | ||
return this._starType; | ||
} | ||
@Input() | ||
set starType(value: starRatingStarTypes) { | ||
this._starType = value || this.config.starType; | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* space | ||
*/ | ||
protected _space: starRatingStarSpace; | ||
get space(): starRatingStarSpace { | ||
return this._space; | ||
} | ||
@Input() | ||
set space(value: starRatingStarSpace) { | ||
this._space = value; | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* readOnly | ||
*/ | ||
protected _readOnly: boolean; | ||
get readOnly(): boolean { | ||
return this._readOnly; | ||
} | ||
@Input() | ||
set readOnly(value: boolean) { | ||
this._readOnly = !!value; | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* disabled | ||
*/ | ||
protected _disabled: boolean; | ||
get disabled(): boolean { | ||
return this._disabled; | ||
} | ||
@Input() | ||
set disabled(value: boolean) { | ||
this._disabled = !!value; | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* rating | ||
*/ | ||
protected _rating: number; | ||
get rating(): number { | ||
return this._rating; | ||
} | ||
@Input() | ||
set rating(value: number) { | ||
//validate and apply newRating | ||
let newRating: number = 0; | ||
if (value >= 0 | ||
&& value <= this.numOfStars) { | ||
newRating = value; | ||
} | ||
//limit max value to max number of stars | ||
if (value > this.numOfStars) { | ||
newRating = this.numOfStars; | ||
} | ||
this._rating = newRating; | ||
//update ratingAsInteger. rating parsed to int for the value-[n] modifier | ||
this.ratingAsInteger = parseInt(this._rating.toString()); | ||
//update halfStarsVisible | ||
this.setHalfStarVisible(); | ||
//update calculated Color | ||
this.setColor(); | ||
//fire onRatingChange event | ||
let $event: IStarRatingOnRatingChangeEven = {rating: this._rating}; | ||
this.onRatingChange.emit($event); | ||
} | ||
/** | ||
* showHalfStars | ||
*/ | ||
protected _showHalfStars: boolean; | ||
get showHalfStars(): boolean { | ||
return this._showHalfStars; | ||
} | ||
@Input() | ||
set showHalfStars(value: boolean) { | ||
this._showHalfStars = !!value; | ||
//update halfStarVisible | ||
this.setHalfStarVisible(); | ||
} | ||
///////////////////////////////////////////// | ||
/** | ||
* getColor | ||
*/ | ||
@Input() | ||
getColor: (rating: number, numOfStars: number, staticColor?: starRatingColor) => starRatingColor; | ||
///////////////////////////////////////////// | ||
/** | ||
* getHalfStarVisible | ||
*/ | ||
@Input() | ||
getHalfStarVisible: (rating: number) => boolean; | ||
///////////////////////////////////////////// | ||
//Output | ||
/////////////////////////////////////////////////////////////////////////////////////////// | ||
@Output() | ||
onClick: EventEmitter<IStarRatingOnClickEvent> = new EventEmitter<IStarRatingOnClickEvent>(); | ||
@Output() | ||
onRatingChange: EventEmitter<IStarRatingOnRatingChangeEven> = new EventEmitter<IStarRatingOnRatingChangeEven>(); | ||
//CTRL ONLY | ||
/////////////////////////////////////////////////////////////////////////////////////////// | ||
classEmpty: string; | ||
classHalf: string; | ||
classFilled: string; | ||
pathEmpty: string; | ||
pathHalf: string; | ||
pathFilled: string; | ||
color: starRatingColor; | ||
stars: Array<number>; | ||
ratingAsInteger: number; | ||
halfStarVisible: boolean; | ||
constructor() { | ||
let config = new StarRatingConfig(); | ||
//set default ctrl props | ||
this.classEmpty = config.classEmpty; | ||
this.classHalf = config.classHalf; | ||
this.classFilled = config.classFilled; | ||
this.pathEmpty = config.svgPathEmpty; | ||
this.pathHalf = config.svgPathHalf; | ||
this.pathFilled = config.svgPathFilled; | ||
//set default Component Inputs | ||
if ('getColor' in config && typeof config.getColor === "function") { | ||
this.getColor = config.getColor; | ||
} | ||
if ('getHalfStarVisible' in config && typeof config.getHalfStarVisible === "function") { | ||
this.getHalfStarVisible = config.getHalfStarVisible; | ||
} | ||
this.numOfStars = config.numOfStars; | ||
this.rating = 0; | ||
super(); | ||
} | ||
svgVisible():boolean { | ||
return this.starType === "svg"; | ||
} | ||
//Overrides | ||
setRating(value: number): void { | ||
let initValue = this.rating; | ||
super.setRating(value); | ||
setColor(): void { | ||
//check if custom function is given | ||
if (typeof this.getColor === "function") { | ||
this.color = this.getColor(this.rating, this.numOfStars, this.staticColor); | ||
} | ||
else { | ||
this.color = StarRatingComponent._getColor(this.rating, this.numOfStars, this.staticColor); | ||
} | ||
} | ||
//if value changed trigger valueAccessor events and outputs | ||
if (initValue !== this.rating) { | ||
let $event: IStarRatingOnRatingChangeEven = {rating: this.rating}; | ||
this.saveOnRatingChange($event); | ||
setHalfStarVisible(): void { | ||
//update halfStarVisible | ||
if (this.showHalfStars) { | ||
//check if custom function is given | ||
if (typeof this.getHalfStarVisible === "function") { | ||
this.halfStarVisible = this.getHalfStarVisible(this.rating); | ||
} else { | ||
this.halfStarVisible = StarRatingComponent._getHalfStarVisible(this.rating); | ||
this.saveOnModelChange(this.rating); | ||
} | ||
} | ||
else { | ||
this.halfStarVisible = false; | ||
} | ||
} | ||
}; | ||
/** | ||
@@ -499,3 +218,3 @@ * onStarClicked | ||
*/ | ||
protected onStarClicked(rating: number): void { | ||
onStarClicked(rating: number): void { | ||
@@ -514,6 +233,10 @@ //fire onClick event | ||
}; | ||
this.onClick.emit(onClickEventObject); | ||
this.saveOnClick(onClickEventObject); | ||
} | ||
/** | ||
* ngOnChanges | ||
* @param changes | ||
*/ | ||
ngOnChanges(changes: any): void { | ||
@@ -534,4 +257,2 @@ | ||
//functions | ||
//boolean | ||
@@ -592,4 +313,14 @@ if (valueChanged('showHalfStars', changes)) { | ||
if (valueChanged('step', changes)) { | ||
this.step = changes['step'].currentValue; | ||
} | ||
} | ||
ngOnInit() { | ||
console.log('ngOnInit'); | ||
this.saveOnModelChange(this.rating); | ||
} | ||
} |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
182361
37
2481
0
443
25